
ADC Niose test Connect four analog signals to your MBED. and then run the Windows app. The four traces are displayed on an oscilloscope like display. I have used a USB HID DEVICE link, so connections to D+, D- are required. The MBED code is otherwise quite basic, So you can modify it to your own test needs. Additionaly, there is a 16 bit count value, in my MBED code Mainly to test if MSB & LSB are correct.
USBMouseKeyboard Class Reference
USBMouseKeyboard example. More...
#include <USBMouseKeyboard.h>
Inherits USBHID.
Public Member Functions | |
USBMouseKeyboard (MOUSE_TYPE mouse_type=REL_MOUSE, uint16_t vendor_id=0x1234, uint16_t product_id=0x0010, uint16_t product_release=0x0001) | |
Constructor. | |
bool | update (int16_t x, int16_t y, uint8_t buttons, int8_t z) |
Write a state of the mouse. | |
bool | move (int16_t x, int16_t y) |
Move the cursor to (x, y) | |
bool | press (uint8_t button) |
Press one or several buttons. | |
bool | release (uint8_t button) |
Release one or several buttons. | |
bool | doubleClick () |
Double click (MOUSE_LEFT) | |
bool | click (uint8_t button) |
Click. | |
bool | scroll (int8_t z) |
Scrolling. | |
bool | keyCode (uint8_t key, uint8_t modifier=0) |
To send a character defined by a modifier(CTRL, SHIFT, ALT) and the key. | |
virtual int | _putc (int c) |
Send a character. | |
bool | mediaControl (MEDIA_KEY key) |
Control media keys. | |
virtual uint8_t * | reportDesc () |
To define the report descriptor. | |
bool | send (HID_REPORT *report) |
Send a Report. | |
bool | read (HID_REPORT *report) |
Read a report. | |
bool | read (uint8_t endpoint, uint8_t *buffer, uint16_t *size, uint16_t maxSize) |
Read a certain endpoint. | |
bool | readNB (HID_REPORT *report) |
Read a report. | |
bool | readNB (uint8_t endpoint, uint8_t *buffer, uint16_t *size, uint16_t maxSize) |
Read a certain endpoint. | |
virtual uint16_t | reportDescLength () |
Get the length of the report descriptor. | |
virtual uint8_t * | stringIproductDesc () |
Get string product descriptor. | |
virtual uint8_t * | stringIinterfaceDesc () |
Get string interface descriptor. | |
virtual uint8_t * | configurationDesc () |
Get configuration descriptor. | |
virtual void | HID_callbackSetReport (HID_REPORT *report) |
HID Report received by SET_REPORT request. | |
virtual bool | USBCallback_request () |
Called by USBDevice on Endpoint0 request. | |
virtual void | USBCallback_requestCompleted () |
Called by USBDevice on Endpoint0 request completion if the 'notify' flag has been set to true. | |
virtual bool | USBCallback_setConfiguration (uint8_t configuration) |
Called by USBDevice layer. | |
bool | configured (void) |
Check if the device is configured. | |
void | connect (void) |
Connect a device. | |
void | disconnect (void) |
Disconnect a device. | |
bool | addEndpoint (uint8_t endpoint, uint32_t maxPacket) |
Add an endpoint. | |
bool | readStart (uint8_t endpoint, uint16_t maxSize) |
Start a reading on a certain endpoint. | |
bool | write (uint8_t endpoint, uint8_t *buffer, uint16_t size, uint16_t maxSize) |
Write a certain endpoint. | |
virtual void | USBCallback_busReset (void) |
Called by USBDevice layer on bus reset. | |
virtual uint8_t * | deviceDesc () |
Get device descriptor. | |
virtual uint8_t * | stringLangidDesc () |
Get string lang id descriptor. | |
virtual uint8_t * | stringImanufacturerDesc () |
Get string manufacturer descriptor. | |
virtual uint8_t * | stringIserialDesc () |
Get string serial descriptor. | |
virtual uint8_t * | stringIConfigurationDesc () |
Get string configuration descriptor. |
Detailed Description
USBMouseKeyboard example.
#include "mbed.h" #include "USBMouseKeyboard.h" USBMouseKeyboard key_mouse; int main(void) { while(1) { key_mouse.move(20, 0); key_mouse.puts("Hello From MBED\r\n"); wait(1); } }
#include "mbed.h" #include "USBMouseKeyboard.h" USBMouseKeyboard key_mouse(ABS_MOUSE); int main(void) { while(1) { key_mouse.move(X_MAX_ABS/2, Y_MAX_ABS/2); key_mouse.puts("Hello from MBED\r\n"); wait(1); } }
Definition at line 56 of file USBMouseKeyboard.h.
Constructor & Destructor Documentation
USBMouseKeyboard | ( | MOUSE_TYPE | mouse_type = REL_MOUSE , |
uint16_t | vendor_id = 0x1234 , |
||
uint16_t | product_id = 0x0010 , |
||
uint16_t | product_release = 0x0001 |
||
) |
Constructor.
- Parameters:
-
mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) vendor_id Your vendor_id (default: 0x1234) product_id Your product_id (default: 0x0001) product_release Your preoduct_release (default: 0x0001)
Definition at line 69 of file USBMouseKeyboard.h.
Member Function Documentation
int _putc | ( | int | c ) | [virtual] |
Send a character.
- Parameters:
-
c character to be sent
- Returns:
- true if there is no error, false otherwise
Definition at line 613 of file USBMouseKeyboard.cpp.
bool addEndpoint | ( | uint8_t | endpoint, |
uint32_t | maxPacket | ||
) | [inherited] |
Add an endpoint.
- Parameters:
-
endpoint endpoint which will be added maxPacket Maximum size of a packet which can be sent for this endpoint
- Returns:
- true if successful, false otherwise
Definition at line 660 of file USBDevice.cpp.
bool click | ( | uint8_t | button ) |
Click.
- Parameters:
-
button state of the buttons ( ex: clic(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 596 of file USBMouseKeyboard.cpp.
uint8_t * configurationDesc | ( | ) | [virtual, inherited] |
Get configuration descriptor.
- Returns:
- pointer to the configuration descriptor
Definition at line 210 of file USBHID.cpp.
bool configured | ( | void | ) | [inherited] |
Check if the device is configured.
- Returns:
- true if configured, false otherwise
Definition at line 637 of file USBDevice.cpp.
void connect | ( | void | ) | [inherited] |
Connect a device.
Definition at line 643 of file USBDevice.cpp.
uint8_t * deviceDesc | ( | ) | [virtual, inherited] |
Get device descriptor.
Warning: this method has to store the length of the report descriptor in reportLength.
- Returns:
- pointer to the device descriptor
Definition at line 836 of file USBDevice.cpp.
void disconnect | ( | void | ) | [inherited] |
Disconnect a device.
Definition at line 649 of file USBDevice.cpp.
bool doubleClick | ( | ) |
Double click (MOUSE_LEFT)
- Returns:
- true if there is no error, false otherwise
Definition at line 589 of file USBMouseKeyboard.cpp.
virtual void HID_callbackSetReport | ( | HID_REPORT * | report ) | [virtual, inherited] |
bool keyCode | ( | uint8_t | key, |
uint8_t | modifier = 0 |
||
) |
To send a character defined by a modifier(CTRL, SHIFT, ALT) and the key.
//To send CTRL + s (save) keyboard.keyCode('s', KEY_CTRL);
- Parameters:
-
modifier bit 0: CTRL, bit 1: SHIFT, bit 2: ALT (default: 0) key character to send
- Returns:
- true if there is no error, false otherwise
Definition at line 617 of file USBMouseKeyboard.cpp.
bool mediaControl | ( | MEDIA_KEY | key ) |
Control media keys.
- Parameters:
-
key media key pressed (KEY_NEXT_TRACK, KEY_PREVIOUS_TRACK, KEY_STOP, KEY_PLAY_PAUSE, KEY_MUTE, KEY_VOLUME_UP, KEY_VOLUME_DOWN)
- Returns:
- true if there is no error, false otherwise
Definition at line 650 of file USBMouseKeyboard.cpp.
bool move | ( | int16_t | x, |
int16_t | y | ||
) |
Move the cursor to (x, y)
- Parameters:
-
x x-axis position y y-axis position
- Returns:
- true if there is no error, false otherwise
Definition at line 581 of file USBMouseKeyboard.cpp.
bool press | ( | uint8_t | button ) |
Press one or several buttons.
- Parameters:
-
button button state (ex: press(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 603 of file USBMouseKeyboard.cpp.
bool read | ( | HID_REPORT * | report ) | [inherited] |
Read a report.
Warning: blocking
- Parameters:
-
report pointer to the report to fill
- Returns:
- true if successful
Definition at line 23 of file USBHID.cpp.
bool read | ( | uint8_t | endpoint, |
uint8_t * | buffer, | ||
uint16_t * | size, | ||
uint16_t | maxSize | ||
) | [inherited] |
Read a certain endpoint.
Before calling this function, USBUSBDevice_readStart must be called.
Warning: blocking
- Parameters:
-
endpoint endpoint which will be read buffer buffer will be filled with the data received size the number of bytes read will be stored in *size maxSize the maximum length that can be read
- Returns:
- true if successful
Definition at line 806 of file USBDevice.cpp.
bool readNB | ( | uint8_t | endpoint, |
uint8_t * | buffer, | ||
uint16_t * | size, | ||
uint16_t | maxSize | ||
) | [inherited] |
Read a certain endpoint.
Warning: non blocking
- Parameters:
-
endpoint endpoint which will be read buffer buffer will be filled with the data received (if data are available) size the number of bytes read will be stored in *size maxSize the maximum length that can be read
- Returns:
- true if successful
Definition at line 822 of file USBDevice.cpp.
bool readNB | ( | HID_REPORT * | report ) | [inherited] |
Read a report.
Warning: non blocking
- Parameters:
-
report pointer to the report to fill
- Returns:
- true if successful
Definition at line 35 of file USBHID.cpp.
bool readStart | ( | uint8_t | endpoint, |
uint16_t | maxSize | ||
) | [inherited] |
Start a reading on a certain endpoint.
You can access the result of the reading by USBDevice_read
- Parameters:
-
endpoint endpoint which will be read maxSize the maximum length that can be read
- Returns:
- true if successful
Definition at line 744 of file USBDevice.cpp.
bool release | ( | uint8_t | button ) |
Release one or several buttons.
- Parameters:
-
button button state (ex: release(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 608 of file USBMouseKeyboard.cpp.
uint8_t * reportDesc | ( | ) | [virtual] |
To define the report descriptor.
Warning: this method has to store the length of the report descriptor in reportLength.
- Returns:
- pointer to the report descriptor
Reimplemented from USBHID.
Definition at line 329 of file USBMouseKeyboard.cpp.
uint16_t reportDescLength | ( | ) | [virtual, inherited] |
Get the length of the report descriptor.
- Returns:
- the length of the report descriptor
Definition at line 47 of file USBHID.cpp.
bool scroll | ( | int8_t | z ) |
Scrolling.
- Parameters:
-
z value of the wheel (>0 to go down, <0 to go up)
- Returns:
- true if there is no error, false otherwise
Definition at line 585 of file USBMouseKeyboard.cpp.
bool send | ( | HID_REPORT * | report ) | [inherited] |
Send a Report.
- Parameters:
-
report Report which will be sent (a report is defined by all data and the length)
- Returns:
- true if successful
Definition at line 17 of file USBHID.cpp.
uint8_t * stringIConfigurationDesc | ( | ) | [virtual, inherited] |
Get string configuration descriptor.
- Returns:
- pointer to the string configuration descriptor
Definition at line 887 of file USBDevice.cpp.
uint8_t * stringIinterfaceDesc | ( | ) | [virtual, inherited] |
Get string interface descriptor.
- Returns:
- pointer to the string interface descriptor
Definition at line 163 of file USBHID.cpp.
uint8_t * stringImanufacturerDesc | ( | ) | [virtual, inherited] |
Get string manufacturer descriptor.
- Returns:
- pointer to the string manufacturer descriptor
Definition at line 869 of file USBDevice.cpp.
uint8_t * stringIproductDesc | ( | ) | [virtual, inherited] |
Get string product descriptor.
- Returns:
- pointer to the string product descriptor
Definition at line 172 of file USBHID.cpp.
uint8_t * stringIserialDesc | ( | ) | [virtual, inherited] |
Get string serial descriptor.
- Returns:
- pointer to the string serial descriptor
Definition at line 878 of file USBDevice.cpp.
uint8_t * stringLangidDesc | ( | ) | [virtual, inherited] |
Get string lang id descriptor.
- Returns:
- pointer to the string lang id descriptor
Definition at line 860 of file USBDevice.cpp.
bool update | ( | int16_t | x, |
int16_t | y, | ||
uint8_t | buttons, | ||
int8_t | z | ||
) |
Write a state of the mouse.
- Parameters:
-
x x-axis position y y-axis position buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) z wheel state (>0 to scroll down, <0 to scroll up)
- Returns:
- true if there is no error, false otherwise
Definition at line 529 of file USBMouseKeyboard.cpp.
virtual void USBCallback_busReset | ( | void | ) | [virtual, inherited] |
Called by USBDevice layer on bus reset.
Warning: Called in ISR context
May be used to reset state
Definition at line 101 of file USBDevice.h.
bool USBCallback_request | ( | ) | [virtual, inherited] |
Called by USBDevice on Endpoint0 request.
Warning: Called in ISR context This is used to handle extensions to standard requests and class specific requests
- Returns:
- true if class handles this request
Definition at line 64 of file USBHID.cpp.
void USBCallback_requestCompleted | ( | ) | [virtual, inherited] |
Called by USBDevice on Endpoint0 request completion if the 'notify' flag has been set to true.
Warning: Called in ISR context
In this case it is used to indicate that a HID report has been received from the host on endpoint 0
Definition at line 139 of file USBHID.cpp.
bool USBCallback_setConfiguration | ( | uint8_t | configuration ) | [virtual, inherited] |
Called by USBDevice layer.
Set configuration of the device. For instance, you can add all endpoints that you need on this function.
- Parameters:
-
configuration Number of the configuration
- Returns:
- true if class handles this request
Definition at line 149 of file USBHID.cpp.
bool write | ( | uint8_t | endpoint, |
uint8_t * | buffer, | ||
uint16_t | size, | ||
uint16_t | maxSize | ||
) | [inherited] |
Write a certain endpoint.
Warning: blocking
- Parameters:
-
endpoint endpoint to write buffer data contained in buffer will be write size the number of bytes to write maxSize the maximum length that can be written on this endpoint
Definition at line 750 of file USBDevice.cpp.
Generated on Tue Jul 12 2022 20:27:21 by
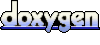