
ADC Niose test Connect four analog signals to your MBED. and then run the Windows app. The four traces are displayed on an oscilloscope like display. I have used a USB HID DEVICE link, so connections to D+, D- are required. The MBED code is otherwise quite basic, So you can modify it to your own test needs. Additionaly, there is a 16 bit count value, in my MBED code Mainly to test if MSB & LSB are correct.
USBDevice.cpp
00001 /* USBDevice.c */ 00002 /* Generic USB device */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 /* Reference: */ 00006 /* Universal Serial Bus Specification Revision 2.0, Chapter 9 "USB Device Framework" */ 00007 00008 #include "stdint.h" 00009 00010 #include "USBEndpoints.h" 00011 #include "USBDevice.h" 00012 #include "USBDescriptor.h" 00013 #include "USBHID_Types.h" 00014 00015 00016 /* Device status */ 00017 #define DEVICE_STATUS_SELF_POWERED (1U<<0) 00018 #define DEVICE_STATUS_REMOTE_WAKEUP (1U<<1) 00019 00020 /* Endpoint status */ 00021 #define ENDPOINT_STATUS_HALT (1U<<0) 00022 00023 /* Standard feature selectors */ 00024 #define DEVICE_REMOTE_WAKEUP (1) 00025 #define ENDPOINT_HALT (0) 00026 00027 /* Macro to convert wIndex endpoint number to physical endpoint number */ 00028 #define WINDEX_TO_PHYSICAL(endpoint) (((endpoint & 0x0f) << 1) + \ 00029 ((endpoint & 0x80) ? 1 : 0)) 00030 00031 00032 bool USBDevice::requestGetDescriptor(void) 00033 { 00034 bool success = false; 00035 00036 switch (DESCRIPTOR_TYPE(transfer.setup.wValue)) 00037 { 00038 case DEVICE_DESCRIPTOR: 00039 if (deviceDesc() != NULL) 00040 { 00041 if ((deviceDesc()[0] == DEVICE_DESCRIPTOR_LENGTH) \ 00042 && (deviceDesc()[1] == DEVICE_DESCRIPTOR)) 00043 { 00044 transfer.remaining = DEVICE_DESCRIPTOR_LENGTH; 00045 transfer.ptr = deviceDesc(); 00046 transfer.direction = DEVICE_TO_HOST; 00047 success = true; 00048 } 00049 } 00050 break; 00051 case CONFIGURATION_DESCRIPTOR: 00052 if (configurationDesc() != NULL) 00053 { 00054 if ((configurationDesc()[0] == CONFIGURATION_DESCRIPTOR_LENGTH) \ 00055 && (configurationDesc()[1] == CONFIGURATION_DESCRIPTOR)) 00056 { 00057 /* Get wTotalLength */ 00058 transfer.remaining = configurationDesc()[2] \ 00059 | (configurationDesc()[3] << 8); 00060 00061 transfer.ptr = configurationDesc(); 00062 transfer.direction = DEVICE_TO_HOST; 00063 success = true; 00064 } 00065 } 00066 break; 00067 case STRING_DESCRIPTOR: 00068 switch (DESCRIPTOR_INDEX(transfer.setup.wValue)) 00069 { 00070 case STRING_OFFSET_LANGID: 00071 transfer.remaining = stringLangidDesc()[0]; 00072 transfer.ptr = stringLangidDesc(); 00073 transfer.direction = DEVICE_TO_HOST; 00074 success = true; 00075 break; 00076 case STRING_OFFSET_IMANUFACTURER: 00077 transfer.remaining = stringImanufacturerDesc()[0]; 00078 transfer.ptr = stringImanufacturerDesc(); 00079 transfer.direction = DEVICE_TO_HOST; 00080 success = true; 00081 break; 00082 case STRING_OFFSET_IPRODUCT: 00083 transfer.remaining = stringIproductDesc()[0]; 00084 transfer.ptr = stringIproductDesc(); 00085 transfer.direction = DEVICE_TO_HOST; 00086 success = true; 00087 break; 00088 case STRING_OFFSET_ISERIAL: 00089 transfer.remaining = stringIserialDesc()[0]; 00090 transfer.ptr = stringIserialDesc(); 00091 transfer.direction = DEVICE_TO_HOST; 00092 success = true; 00093 break; 00094 case STRING_OFFSET_ICONFIGURATION: 00095 transfer.remaining = stringIConfigurationDesc()[0]; 00096 transfer.ptr = stringIConfigurationDesc(); 00097 transfer.direction = DEVICE_TO_HOST; 00098 success = true; 00099 break; 00100 case STRING_OFFSET_IINTERFACE: 00101 transfer.remaining = stringIinterfaceDesc()[0]; 00102 transfer.ptr = stringIinterfaceDesc(); 00103 transfer.direction = DEVICE_TO_HOST; 00104 success = true; 00105 break; 00106 } 00107 break; 00108 case INTERFACE_DESCRIPTOR: 00109 case ENDPOINT_DESCRIPTOR: 00110 /* TODO: Support is optional, not implemented here */ 00111 break; 00112 default: 00113 break; 00114 } 00115 00116 return success; 00117 } 00118 00119 void USBDevice::decodeSetupPacket(uint8_t *data, SETUP_PACKET *packet) 00120 { 00121 /* Fill in the elements of a SETUP_PACKET structure from raw data */ 00122 packet->bmRequestType.dataTransferDirection = (data[0] & 0x80) >> 7; 00123 packet->bmRequestType.Type = (data[0] & 0x60) >> 5; 00124 packet->bmRequestType.Recipient = data[0] & 0x1f; 00125 packet->bRequest = data[1]; 00126 packet->wValue = (data[2] | (uint16_t)data[3] << 8); 00127 packet->wIndex = (data[4] | (uint16_t)data[5] << 8); 00128 packet->wLength = (data[6] | (uint16_t)data[7] << 8); 00129 } 00130 00131 00132 bool USBDevice::controlOut(void) 00133 { 00134 /* Control transfer data OUT stage */ 00135 uint8_t buffer[MAX_PACKET_SIZE_EP0]; 00136 uint32_t packetSize; 00137 00138 /* Check we should be transferring data OUT */ 00139 if (transfer.direction != HOST_TO_DEVICE) 00140 { 00141 return false; 00142 } 00143 00144 /* Read from endpoint */ 00145 packetSize = EP0getReadResult(buffer); 00146 00147 /* Check if transfer size is valid */ 00148 if (packetSize > transfer.remaining) 00149 { 00150 /* Too big */ 00151 return false; 00152 } 00153 00154 /* Update transfer */ 00155 transfer.ptr += packetSize; 00156 transfer.remaining -= packetSize; 00157 00158 /* Check if transfer has completed */ 00159 if (transfer.remaining == 0) 00160 { 00161 /* Transfer completed */ 00162 if (transfer.notify) 00163 { 00164 /* Notify class layer. */ 00165 USBCallback_requestCompleted(); 00166 transfer.notify = false; 00167 } 00168 /* Status stage */ 00169 EP0write(NULL, 0); 00170 } 00171 else 00172 { 00173 EP0read(); 00174 } 00175 00176 return true; 00177 } 00178 00179 bool USBDevice::controlIn(void) 00180 { 00181 /* Control transfer data IN stage */ 00182 uint32_t packetSize; 00183 00184 /* Check if transfer has completed (status stage transactions */ 00185 /* also have transfer.remaining == 0) */ 00186 if (transfer.remaining == 0) 00187 { 00188 if (transfer.zlp) 00189 { 00190 /* Send zero length packet */ 00191 EP0write(NULL, 0); 00192 transfer.zlp = false; 00193 } 00194 00195 /* Transfer completed */ 00196 if (transfer.notify) 00197 { 00198 /* Notify class layer. */ 00199 USBCallback_requestCompleted(); 00200 transfer.notify = false; 00201 } 00202 00203 EP0read(); 00204 00205 /* Completed */ 00206 return true; 00207 } 00208 00209 /* Check we should be transferring data IN */ 00210 if (transfer.direction != DEVICE_TO_HOST) 00211 { 00212 return false; 00213 } 00214 00215 packetSize = transfer.remaining; 00216 00217 if (packetSize > MAX_PACKET_SIZE_EP0) 00218 { 00219 packetSize = MAX_PACKET_SIZE_EP0; 00220 } 00221 00222 /* Write to endpoint */ 00223 EP0write(transfer.ptr, packetSize); 00224 00225 /* Update transfer */ 00226 transfer.ptr += packetSize; 00227 transfer.remaining -= packetSize; 00228 00229 return true; 00230 } 00231 00232 bool USBDevice::requestSetAddress(void) 00233 { 00234 /* Set the device address */ 00235 setAddress(transfer.setup.wValue); 00236 00237 if (transfer.setup.wValue == 0) 00238 { 00239 device.state = DEFAULT; 00240 } 00241 else 00242 { 00243 device.state = ADDRESS; 00244 } 00245 00246 return true; 00247 } 00248 00249 bool USBDevice::requestSetConfiguration(void) 00250 { 00251 00252 device.configuration = transfer.setup.wValue; 00253 /* Set the device configuration */ 00254 if (device.configuration == 0) 00255 { 00256 /* Not configured */ 00257 unconfigureDevice(); 00258 device.state = ADDRESS; 00259 } 00260 else 00261 { 00262 if (USBCallback_setConfiguration(device.configuration)) 00263 { 00264 /* Valid configuration */ 00265 configureDevice(); 00266 device.state = CONFIGURED; 00267 } 00268 else 00269 { 00270 return false; 00271 } 00272 } 00273 00274 return true; 00275 } 00276 00277 bool USBDevice::requestGetConfiguration(void) 00278 { 00279 /* Send the device configuration */ 00280 transfer.ptr = &device.configuration; 00281 transfer.remaining = sizeof(device.configuration); 00282 transfer.direction = DEVICE_TO_HOST; 00283 return true; 00284 } 00285 00286 bool USBDevice::requestGetInterface(void) 00287 { 00288 static uint8_t alternateSetting; 00289 00290 /* Return the selected alternate setting for an interface */ 00291 00292 if (device.state != CONFIGURED) 00293 { 00294 return false; 00295 } 00296 00297 /* TODO: We currently do not support alternate settings */ 00298 /* so always return zero */ 00299 /* TODO: Should check that the interface number is valid */ 00300 alternateSetting = 0; 00301 00302 /* Send the alternate setting */ 00303 transfer.ptr = &alternateSetting; 00304 transfer.remaining = sizeof(alternateSetting); 00305 transfer.direction = DEVICE_TO_HOST; 00306 return true; 00307 } 00308 00309 bool USBDevice::requestSetInterface(void) 00310 { 00311 /* TODO: We currently do not support alternate settings, return false */ 00312 return false; 00313 } 00314 00315 bool USBDevice::requestSetFeature() 00316 { 00317 bool success = false; 00318 00319 if (device.state != CONFIGURED) 00320 { 00321 /* Endpoint or interface must be zero */ 00322 if (transfer.setup.wIndex != 0) 00323 { 00324 return false; 00325 } 00326 } 00327 00328 switch (transfer.setup.bmRequestType.Recipient) 00329 { 00330 case DEVICE_RECIPIENT: 00331 /* TODO: Remote wakeup feature not supported */ 00332 break; 00333 case ENDPOINT_RECIPIENT: 00334 if (transfer.setup.wValue == ENDPOINT_HALT) 00335 { 00336 /* TODO: We should check that the endpoint number is valid */ 00337 stallEndpoint( 00338 WINDEX_TO_PHYSICAL(transfer.setup.wIndex)); 00339 success = true; 00340 } 00341 break; 00342 default: 00343 break; 00344 } 00345 00346 return success; 00347 } 00348 00349 bool USBDevice::requestClearFeature() 00350 { 00351 bool success = false; 00352 00353 if (device.state != CONFIGURED) 00354 { 00355 /* Endpoint or interface must be zero */ 00356 if (transfer.setup.wIndex != 0) 00357 { 00358 return false; 00359 } 00360 } 00361 00362 switch (transfer.setup.bmRequestType.Recipient) 00363 { 00364 case DEVICE_RECIPIENT: 00365 /* TODO: Remote wakeup feature not supported */ 00366 break; 00367 case ENDPOINT_RECIPIENT: 00368 /* TODO: We should check that the endpoint number is valid */ 00369 if (transfer.setup.wValue == ENDPOINT_HALT) 00370 { 00371 unstallEndpoint( 00372 WINDEX_TO_PHYSICAL(transfer.setup.wIndex)); 00373 success = true; 00374 } 00375 break; 00376 default: 00377 break; 00378 } 00379 00380 return success; 00381 } 00382 00383 bool USBDevice::requestGetStatus(void) 00384 { 00385 static uint16_t status; 00386 bool success = false; 00387 00388 if (device.state != CONFIGURED) 00389 { 00390 /* Endpoint or interface must be zero */ 00391 if (transfer.setup.wIndex != 0) 00392 { 00393 return false; 00394 } 00395 } 00396 00397 switch (transfer.setup.bmRequestType.Recipient) 00398 { 00399 case DEVICE_RECIPIENT: 00400 /* TODO: Currently only supports self powered devices */ 00401 status = DEVICE_STATUS_SELF_POWERED; 00402 success = true; 00403 break; 00404 case INTERFACE_RECIPIENT: 00405 status = 0; 00406 success = true; 00407 break; 00408 case ENDPOINT_RECIPIENT: 00409 /* TODO: We should check that the endpoint number is valid */ 00410 if (getEndpointStallState( 00411 WINDEX_TO_PHYSICAL(transfer.setup.wIndex))) 00412 { 00413 status = ENDPOINT_STATUS_HALT; 00414 } 00415 else 00416 { 00417 status = 0; 00418 } 00419 success = true; 00420 break; 00421 default: 00422 break; 00423 } 00424 00425 if (success) 00426 { 00427 /* Send the status */ 00428 transfer.ptr = (uint8_t *)&status; /* Assumes little endian */ 00429 transfer.remaining = sizeof(status); 00430 transfer.direction = DEVICE_TO_HOST; 00431 } 00432 00433 return success; 00434 } 00435 00436 bool USBDevice::requestSetup(void) 00437 { 00438 bool success = false; 00439 00440 /* Process standard requests */ 00441 if ((transfer.setup.bmRequestType.Type == STANDARD_TYPE)) 00442 { 00443 switch (transfer.setup.bRequest) 00444 { 00445 case GET_STATUS: 00446 success = requestGetStatus(); 00447 break; 00448 case CLEAR_FEATURE: 00449 success = requestClearFeature(); 00450 break; 00451 case SET_FEATURE: 00452 success = requestSetFeature(); 00453 break; 00454 case SET_ADDRESS: 00455 success = requestSetAddress(); 00456 break; 00457 case GET_DESCRIPTOR: 00458 success = requestGetDescriptor(); 00459 break; 00460 case SET_DESCRIPTOR: 00461 /* TODO: Support is optional, not implemented here */ 00462 success = false; 00463 break; 00464 case GET_CONFIGURATION: 00465 success = requestGetConfiguration(); 00466 break; 00467 case SET_CONFIGURATION: 00468 success = requestSetConfiguration(); 00469 break; 00470 case GET_INTERFACE: 00471 success = requestGetInterface(); 00472 break; 00473 case SET_INTERFACE: 00474 success = requestSetInterface(); 00475 break; 00476 default: 00477 break; 00478 } 00479 } 00480 00481 return success; 00482 } 00483 00484 bool USBDevice::controlSetup(void) 00485 { 00486 bool success = false; 00487 00488 /* Control transfer setup stage */ 00489 uint8_t buffer[MAX_PACKET_SIZE_EP0]; 00490 00491 EP0setup(buffer); 00492 00493 /* Initialise control transfer state */ 00494 decodeSetupPacket(buffer, &transfer.setup); 00495 transfer.ptr = NULL; 00496 transfer.remaining = 0; 00497 transfer.direction = 0; 00498 transfer.zlp = false; 00499 transfer.notify = false; 00500 00501 /* Process request */ 00502 00503 /* Class / vendor specific */ 00504 success = USBCallback_request(); 00505 00506 if (!success) 00507 { 00508 /* Standard requests */ 00509 if (!requestSetup()) 00510 { 00511 return false; 00512 } 00513 } 00514 00515 /* Check transfer size and direction */ 00516 if (transfer.setup.wLength>0) 00517 { 00518 if (transfer.setup.bmRequestType.dataTransferDirection \ 00519 == DEVICE_TO_HOST) 00520 { 00521 /* IN data stage is required */ 00522 if (transfer.direction != DEVICE_TO_HOST) 00523 { 00524 return false; 00525 } 00526 00527 /* Transfer must be less than or equal to the size */ 00528 /* requested by the host */ 00529 if (transfer.remaining > transfer.setup.wLength) 00530 { 00531 transfer.remaining = transfer.setup.wLength; 00532 } 00533 } 00534 else 00535 { 00536 00537 /* OUT data stage is required */ 00538 if (transfer.direction != HOST_TO_DEVICE) 00539 { 00540 return false; 00541 } 00542 00543 /* Transfer must be equal to the size requested by the host */ 00544 if (transfer.remaining != transfer.setup.wLength) 00545 { 00546 return false; 00547 } 00548 } 00549 } 00550 else 00551 { 00552 /* No data stage; transfer size must be zero */ 00553 if (transfer.remaining != 0) 00554 { 00555 return false; 00556 } 00557 } 00558 00559 /* Data or status stage if applicable */ 00560 if (transfer.setup.wLength>0) 00561 { 00562 if (transfer.setup.bmRequestType.dataTransferDirection \ 00563 == DEVICE_TO_HOST) 00564 { 00565 /* Check if we'll need to send a zero length packet at */ 00566 /* the end of this transfer */ 00567 if (transfer.setup.wLength > transfer.remaining) 00568 { 00569 /* Device wishes to transfer less than host requested */ 00570 if ((transfer.remaining % MAX_PACKET_SIZE_EP0) == 0) 00571 { 00572 /* Transfer is a multiple of EP0 max packet size */ 00573 transfer.zlp = true; 00574 } 00575 } 00576 00577 /* IN stage */ 00578 controlIn(); 00579 } 00580 else 00581 { 00582 /* OUT stage */ 00583 EP0read(); 00584 } 00585 } 00586 else 00587 { 00588 /* Status stage */ 00589 EP0write(NULL, 0); 00590 } 00591 00592 return true; 00593 } 00594 00595 void USBDevice::busReset(void) 00596 { 00597 device.state = DEFAULT; 00598 device.configuration = 0; 00599 device.suspended = false; 00600 00601 /* Call class / vendor specific busReset function */ 00602 USBCallback_busReset(); 00603 } 00604 00605 void USBDevice::EP0setupCallback(void) 00606 { 00607 /* Endpoint 0 setup event */ 00608 if (!controlSetup()) 00609 { 00610 /* Protocol stall */ 00611 EP0stall(); 00612 } 00613 00614 /* Return true if an OUT data stage is expected */ 00615 } 00616 00617 void USBDevice::EP0out(void) 00618 { 00619 /* Endpoint 0 OUT data event */ 00620 if (!controlOut()) 00621 { 00622 /* Protocol stall; this will stall both endpoints */ 00623 EP0stall(); 00624 } 00625 } 00626 00627 void USBDevice::EP0in(void) 00628 { 00629 /* Endpoint 0 IN data event */ 00630 if (!controlIn()) 00631 { 00632 /* Protocol stall; this will stall both endpoints */ 00633 EP0stall(); 00634 } 00635 } 00636 00637 bool USBDevice::configured(void) 00638 { 00639 /* Returns true if device is in the CONFIGURED state */ 00640 return (device.state == CONFIGURED); 00641 } 00642 00643 void USBDevice::connect(void) 00644 { 00645 /* Connect device */ 00646 USBHAL::connect(); 00647 } 00648 00649 void USBDevice::disconnect(void) 00650 { 00651 /* Disconnect device */ 00652 USBHAL::disconnect(); 00653 } 00654 00655 CONTROL_TRANSFER * USBDevice::getTransferPtr(void) 00656 { 00657 return &transfer; 00658 } 00659 00660 bool USBDevice::addEndpoint(uint8_t endpoint, uint32_t maxPacket) 00661 { 00662 return realiseEndpoint(endpoint, maxPacket, 0); 00663 } 00664 00665 bool USBDevice::addRateFeedbackEndpoint(uint8_t endpoint, uint32_t maxPacket) 00666 { 00667 /* For interrupt endpoints only */ 00668 return realiseEndpoint(endpoint, maxPacket, RATE_FEEDBACK_MODE); 00669 } 00670 00671 uint8_t * USBDevice::findDescriptor(uint8_t descriptorType) 00672 { 00673 /* Find a descriptor within the list of descriptors */ 00674 /* following a configuration descriptor. */ 00675 uint16_t wTotalLength; 00676 uint8_t *ptr; 00677 00678 if (configurationDesc() == NULL) 00679 { 00680 return NULL; 00681 } 00682 00683 /* Check this is a configuration descriptor */ 00684 if ((configurationDesc()[0] != CONFIGURATION_DESCRIPTOR_LENGTH) \ 00685 || (configurationDesc()[1] != CONFIGURATION_DESCRIPTOR)) 00686 { 00687 return NULL; 00688 } 00689 00690 wTotalLength = configurationDesc()[2] | (configurationDesc()[3] << 8); 00691 00692 /* Check there are some more descriptors to follow */ 00693 if (wTotalLength <= (CONFIGURATION_DESCRIPTOR_LENGTH+2)) 00694 /* +2 is for bLength and bDescriptorType of next descriptor */ 00695 { 00696 return false; 00697 } 00698 00699 /* Start at first descriptor after the configuration descriptor */ 00700 ptr = &(configurationDesc()[CONFIGURATION_DESCRIPTOR_LENGTH]); 00701 00702 do { 00703 if (ptr[1] /* bDescriptorType */ == descriptorType) 00704 { 00705 /* Found */ 00706 return ptr; 00707 } 00708 00709 /* Skip to next descriptor */ 00710 ptr += ptr[0]; /* bLength */ 00711 } while (ptr < (configurationDesc() + wTotalLength)); 00712 00713 /* Reached end of the descriptors - not found */ 00714 return NULL; 00715 } 00716 00717 void USBDevice::SOF(int frameNumber) 00718 { 00719 } 00720 00721 void USBDevice::connectStateChanged(unsigned int connected) 00722 { 00723 } 00724 00725 void USBDevice::suspendStateChanged(unsigned int suspended) 00726 { 00727 } 00728 00729 00730 USBDevice::USBDevice(uint16_t vendor_id, uint16_t product_id, uint16_t product_release){ 00731 VENDOR_ID = vendor_id; 00732 PRODUCT_ID = product_id; 00733 PRODUCT_RELEASE = product_release; 00734 00735 /* Set initial device state */ 00736 device.state = POWERED; 00737 device.configuration = 0; 00738 device.suspended = false; 00739 00740 connect(); 00741 }; 00742 00743 00744 bool USBDevice::readStart(uint8_t endpoint, uint16_t maxSize) 00745 { 00746 return endpointRead(endpoint, maxSize) == EP_PENDING; 00747 } 00748 00749 00750 bool USBDevice::write(uint8_t endpoint, uint8_t * buffer, uint16_t size, uint16_t maxSize) 00751 { 00752 EP_STATUS result; 00753 00754 if (size > maxSize) 00755 { 00756 return false; 00757 } 00758 00759 /* Block if not configured */ 00760 while (!configured()); 00761 00762 /* Send report */ 00763 result = endpointWrite(endpoint, buffer, size); 00764 00765 if (result != EP_PENDING) 00766 { 00767 return false; 00768 } 00769 00770 /* Wait for completion */ 00771 do { 00772 result = endpointWriteResult(endpoint); 00773 } while ((result == EP_PENDING) && configured()); 00774 00775 return (result == EP_COMPLETED); 00776 } 00777 00778 00779 bool USBDevice::writeNB(uint8_t endpoint, uint8_t * buffer, uint16_t size, uint16_t maxSize) 00780 { 00781 EP_STATUS result; 00782 00783 if (size > maxSize) 00784 { 00785 return false; 00786 } 00787 00788 /* Block if not configured */ 00789 while (!configured()); 00790 00791 /* Send report */ 00792 result = endpointWrite(endpoint, buffer, size); 00793 00794 if (result != EP_PENDING) 00795 { 00796 return false; 00797 } 00798 00799 result = endpointWriteResult(endpoint); 00800 00801 return (result == EP_COMPLETED); 00802 } 00803 00804 00805 00806 bool USBDevice::read(uint8_t endpoint, uint8_t * buffer, uint16_t * size, uint16_t maxSize) 00807 { 00808 EP_STATUS result; 00809 00810 /* Block if not configured */ 00811 while (!configured()); 00812 00813 /* Wait for completion */ 00814 do { 00815 result = endpointReadResult(endpoint, buffer, (uint32_t *)size); 00816 } while ((result == EP_PENDING) && configured()); 00817 00818 return (result == EP_COMPLETED); 00819 } 00820 00821 00822 bool USBDevice::readNB(uint8_t endpoint, uint8_t * buffer, uint16_t * size, uint16_t maxSize) 00823 { 00824 EP_STATUS result; 00825 00826 /* Block if not configured */ 00827 while (!configured()); 00828 00829 result = endpointReadResult(endpoint, buffer, (uint32_t *)size); 00830 00831 return (result == EP_COMPLETED); 00832 } 00833 00834 00835 00836 uint8_t * USBDevice::deviceDesc() { 00837 static uint8_t deviceDescriptor[] = { 00838 DEVICE_DESCRIPTOR_LENGTH, /* bLength */ 00839 DEVICE_DESCRIPTOR, /* bDescriptorType */ 00840 LSB(USB_VERSION_2_0), /* bcdUSB (LSB) */ 00841 MSB(USB_VERSION_2_0), /* bcdUSB (MSB) */ 00842 0x00, /* bDeviceClass */ 00843 0x00, /* bDeviceSubClass */ 00844 0x00, /* bDeviceprotocol */ 00845 MAX_PACKET_SIZE_EP0, /* bMaxPacketSize0 */ 00846 LSB(VENDOR_ID), /* idVendor (LSB) */ 00847 MSB(VENDOR_ID), /* idVendor (MSB) */ 00848 LSB(PRODUCT_ID), /* idProduct (LSB) */ 00849 MSB(PRODUCT_ID), /* idProduct (MSB) */ 00850 LSB(PRODUCT_RELEASE), /* bcdDevice (LSB) */ 00851 MSB(PRODUCT_RELEASE), /* bcdDevice (MSB) */ 00852 STRING_OFFSET_IMANUFACTURER, /* iManufacturer */ 00853 STRING_OFFSET_IPRODUCT, /* iProduct */ 00854 STRING_OFFSET_ISERIAL, /* iSerialNumber */ 00855 0x01 /* bNumConfigurations */ 00856 }; 00857 return deviceDescriptor; 00858 } 00859 00860 uint8_t * USBDevice::stringLangidDesc() { 00861 static uint8_t stringLangidDescriptor[] = { 00862 0x04, /*bLength*/ 00863 STRING_DESCRIPTOR, /*bDescriptorType 0x03*/ 00864 0x09,0x00, /*bString Lang ID - 0x009 - English*/ 00865 }; 00866 return stringLangidDescriptor; 00867 } 00868 00869 uint8_t * USBDevice::stringImanufacturerDesc() { 00870 static uint8_t stringImanufacturerDescriptor[] = { 00871 0x12, /*bLength*/ 00872 STRING_DESCRIPTOR, /*bDescriptorType 0x03*/ 00873 'm',0,'b',0,'e',0,'d',0,'.',0,'o',0,'r',0,'g',0, /*bString iManufacturer - mbed.org*/ 00874 }; 00875 return stringImanufacturerDescriptor; 00876 } 00877 00878 uint8_t * USBDevice::stringIserialDesc() { 00879 static uint8_t stringIserialDescriptor[] = { 00880 0x16, /*bLength*/ 00881 STRING_DESCRIPTOR, /*bDescriptorType 0x03*/ 00882 '0',0,'1',0,'2',0,'3',0,'4',0,'5',0,'6',0,'7',0,'8',0,'9',0, /*bString iSerial - 0123456789*/ 00883 }; 00884 return stringIserialDescriptor; 00885 } 00886 00887 uint8_t * USBDevice::stringIConfigurationDesc() { 00888 static uint8_t stringIconfigurationDescriptor[] = { 00889 0x06, /*bLength*/ 00890 STRING_DESCRIPTOR, /*bDescriptorType 0x03*/ 00891 '0',0,'1',0, /*bString iConfiguration - 01*/ 00892 }; 00893 return stringIconfigurationDescriptor; 00894 } 00895 00896 uint8_t * USBDevice::stringIinterfaceDesc() { 00897 static uint8_t stringIinterfaceDescriptor[] = { 00898 0x08, /*bLength*/ 00899 STRING_DESCRIPTOR, /*bDescriptorType 0x03*/ 00900 'U',0,'S',0,'B',0, /*bString iInterface - USB*/ 00901 }; 00902 return stringIinterfaceDescriptor; 00903 } 00904 00905 uint8_t * USBDevice::stringIproductDesc() { 00906 static uint8_t stringIproductDescriptor[] = { 00907 0x16, /*bLength*/ 00908 STRING_DESCRIPTOR, /*bDescriptorType 0x03*/ 00909 'U',0,'S',0,'B',0,' ',0,'D',0,'E',0,'V',0,'I',0,'C',0,'E',0 /*bString iProduct - USB DEVICE*/ 00910 }; 00911 return stringIproductDescriptor; 00912 }
Generated on Tue Jul 12 2022 20:27:20 by
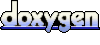