
ADC Niose test Connect four analog signals to your MBED. and then run the Windows app. The four traces are displayed on an oscilloscope like display. I have used a USB HID DEVICE link, so connections to D+, D- are required. The MBED code is otherwise quite basic, So you can modify it to your own test needs. Additionaly, there is a 16 bit count value, in my MBED code Mainly to test if MSB & LSB are correct.
USBMouseKeyboard.h
00001 /* USBMouseKeyboard.h */ 00002 /* USB device example: Keyboard with a relative mouse */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef USBMOUSEKEYBOARD_H 00006 #define USBMOUSEKEYBOARD_H 00007 00008 #define REPORT_ID_KEYBOARD 1 00009 #define REPORT_ID_MOUSE 2 00010 #define REPORT_ID_VOLUME 3 00011 00012 #include "USBMouse.h" 00013 #include "USBKeyboard.h" 00014 #include "Stream.h" 00015 #include "USBHID.h" 00016 00017 /** 00018 * USBMouseKeyboard example 00019 * @code 00020 * 00021 * #include "mbed.h" 00022 * #include "USBMouseKeyboard.h" 00023 * 00024 * USBMouseKeyboard key_mouse; 00025 * 00026 * int main(void) 00027 * { 00028 * while(1) 00029 * { 00030 * key_mouse.move(20, 0); 00031 * key_mouse.puts("Hello From MBED\r\n"); 00032 * wait(1); 00033 * } 00034 * } 00035 * @endcode 00036 * 00037 * 00038 * @code 00039 * 00040 * #include "mbed.h" 00041 * #include "USBMouseKeyboard.h" 00042 * 00043 * USBMouseKeyboard key_mouse(ABS_MOUSE); 00044 * 00045 * int main(void) 00046 * { 00047 * while(1) 00048 * { 00049 * key_mouse.move(X_MAX_ABS/2, Y_MAX_ABS/2); 00050 * key_mouse.puts("Hello from MBED\r\n"); 00051 * wait(1); 00052 * } 00053 * } 00054 * @endcode 00055 */ 00056 class USBMouseKeyboard: public USBHID, public Stream 00057 { 00058 public: 00059 00060 /** 00061 * Constructor 00062 * 00063 * @param mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) 00064 * @param vendor_id Your vendor_id (default: 0x1234) 00065 * @param product_id Your product_id (default: 0x0001) 00066 * @param product_release Your preoduct_release (default: 0x0001) 00067 * 00068 */ 00069 USBMouseKeyboard(MOUSE_TYPE mouse_type = REL_MOUSE, uint16_t vendor_id = 0x1234, uint16_t product_id = 0x0010, uint16_t product_release = 0x0001): 00070 USBHID(vendor_id, product_id, product_release) 00071 { 00072 button = 0; 00073 this->mouse_type = mouse_type; 00074 }; 00075 00076 00077 /** 00078 * Write a state of the mouse 00079 * 00080 * @param x x-axis position 00081 * @param y y-axis position 00082 * @param buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) 00083 * @param z wheel state (>0 to scroll down, <0 to scroll up) 00084 * @returns true if there is no error, false otherwise 00085 */ 00086 bool update(int16_t x, int16_t y, uint8_t buttons, int8_t z); 00087 00088 00089 /** 00090 * Move the cursor to (x, y) 00091 * 00092 * @param x x-axis position 00093 * @param y y-axis position 00094 * @returns true if there is no error, false otherwise 00095 */ 00096 bool move(int16_t x, int16_t y); 00097 00098 /** 00099 * Press one or several buttons 00100 * 00101 * @param button button state (ex: press(MOUSE_LEFT)) 00102 * @returns true if there is no error, false otherwise 00103 */ 00104 bool press(uint8_t button); 00105 00106 /** 00107 * Release one or several buttons 00108 * 00109 * @param button button state (ex: release(MOUSE_LEFT)) 00110 * @returns true if there is no error, false otherwise 00111 */ 00112 bool release(uint8_t button); 00113 00114 /** 00115 * Double click (MOUSE_LEFT) 00116 * 00117 * @returns true if there is no error, false otherwise 00118 */ 00119 bool doubleClick(); 00120 00121 /** 00122 * Click 00123 * 00124 * @param button state of the buttons ( ex: clic(MOUSE_LEFT)) 00125 * @returns true if there is no error, false otherwise 00126 */ 00127 bool click(uint8_t button); 00128 00129 /** 00130 * Scrolling 00131 * 00132 * @param z value of the wheel (>0 to go down, <0 to go up) 00133 * @returns true if there is no error, false otherwise 00134 */ 00135 bool scroll(int8_t z); 00136 00137 /** 00138 * To send a character defined by a modifier(CTRL, SHIFT, ALT) and the key 00139 * 00140 * @code 00141 * //To send CTRL + s (save) 00142 * keyboard.keyCode('s', KEY_CTRL); 00143 * @endcode 00144 * 00145 * @param modifier bit 0: CTRL, bit 1: SHIFT, bit 2: ALT (default: 0) 00146 * @param key character to send 00147 * @returns true if there is no error, false otherwise 00148 */ 00149 bool keyCode(uint8_t key, uint8_t modifier = 0); 00150 00151 /** 00152 * Send a character 00153 * 00154 * @param c character to be sent 00155 * @returns true if there is no error, false otherwise 00156 */ 00157 virtual int _putc(int c); 00158 00159 /** 00160 * Control media keys 00161 * 00162 * @param key media key pressed (KEY_NEXT_TRACK, KEY_PREVIOUS_TRACK, KEY_STOP, KEY_PLAY_PAUSE, KEY_MUTE, KEY_VOLUME_UP, KEY_VOLUME_DOWN) 00163 * @returns true if there is no error, false otherwise 00164 */ 00165 bool mediaControl(MEDIA_KEY key); 00166 00167 /** 00168 * To define the report descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00169 * 00170 * @returns pointer to the report descriptor 00171 */ 00172 virtual uint8_t * reportDesc(); 00173 00174 00175 private: 00176 bool mouseWrite(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00177 MOUSE_TYPE mouse_type; 00178 uint8_t button; 00179 bool mouseSend(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00180 00181 //dummy otherwise it doesn,t compile (we must define all methods of an abstract class) 00182 virtual int _getc() { return -1;} 00183 }; 00184 00185 #endif
Generated on Tue Jul 12 2022 20:27:21 by
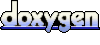