
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
list.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __LIST_H__ 00010 #define __LIST_H__ 00011 00012 /** 00013 * \file 00014 * \brief List Object Type 00015 * 00016 * List object type header. 00017 */ 00018 00019 /** 00020 * List obj 00021 * 00022 * Mutable ordered sequence of objects. Contains ptr to linked list of nodes. 00023 */ 00024 typedef struct PmList_s 00025 { 00026 /** Object descriptor */ 00027 PmObjDesc_t od; 00028 00029 /** List length; number of objs linked */ 00030 uint16_t length; 00031 00032 /** Ptr to linked list of nodes */ 00033 pSeglist_t val; 00034 } PmList_t, 00035 *pPmList_t; 00036 00037 00038 /** 00039 * Allocates a new List object. 00040 * 00041 * If there is not enough memory to allocate the List, 00042 * the return status will indicate an OutOfMemoryError 00043 * that must be passed up to the interpreter. 00044 * Otherwise, a ptr to the list is returned by reference 00045 * and the return status is OK. 00046 * 00047 * @param r_pobj Return; addr of ptr to obj 00048 * @return Return status 00049 */ 00050 PmReturn_t list_new(pPmObj_t *r_pobj); 00051 00052 /** 00053 * Gets the object in the list at the index. 00054 * 00055 * @param plist Ptr to list obj 00056 * @param index Index into list 00057 * @param r_pobj Return by reference; ptr to item 00058 * @return Return status 00059 */ 00060 PmReturn_t list_getItem(pPmObj_t plist, int16_t index, pPmObj_t *r_pobj); 00061 00062 /** 00063 * Sets the item in the list at the index. 00064 * 00065 * @param plist Ptr to list 00066 * @param index Index into list 00067 * @param pobj Ptr to obj to put into list 00068 * @return Return status 00069 */ 00070 PmReturn_t list_setItem(pPmObj_t plist, int16_t index, pPmObj_t pobj); 00071 00072 /** 00073 * Makes a copy of the given list. 00074 * 00075 * Allocate the necessary memory for root and nodes. 00076 * Duplicate ptrs to objs. 00077 * 00078 * @param pobj Ptr to source list 00079 * @param r_pobj Return; Addr of ptr to return obj 00080 * @return Return status 00081 */ 00082 PmReturn_t list_copy(pPmObj_t pobj, pPmObj_t *r_pobj); 00083 00084 /** 00085 * Appends the given obj to the end of the given list. 00086 * 00087 * Allocate the memory for the node. 00088 * Do not copy obj, just reuse ptr. 00089 * 00090 * @param plist Ptr to list 00091 * @param pobj Ptr to item to append 00092 * @return Return status 00093 */ 00094 PmReturn_t list_append(pPmObj_t plist, pPmObj_t pobj); 00095 00096 /** 00097 * Creates a new list with the contents of psrclist 00098 * copied pint number of times. 00099 * This implements the python code "[0,...] * N" 00100 * where the list can be any list and N is an integer. 00101 * 00102 * @param psrclist The source list to replicate 00103 * @param n The integer number of times to replicate it 00104 * @param r_pnewlist Return; new list with its contents set. 00105 * @return Return status 00106 */ 00107 PmReturn_t list_replicate(pPmObj_t psrclist, int16_t n, pPmObj_t *r_pnewlist); 00108 00109 /** 00110 * Inserts the object into the list at the desired index. 00111 * 00112 * @param plist Ptr to list obj 00113 * @param pobj Ptr to obj to insert 00114 * @param index Index of where to insert obj 00115 * @return Return status 00116 */ 00117 PmReturn_t list_insert(pPmObj_t plist, int16_t index, pPmObj_t pobj); 00118 00119 /** 00120 * Removes a given object from the list. 00121 * 00122 * @param plist Ptr to list obj 00123 * @param item Ptr to object to be removed 00124 * @return Return status 00125 */ 00126 PmReturn_t list_remove(pPmObj_t plist, pPmObj_t item); 00127 00128 /** 00129 * Finds the first index of the item that matches pitem. 00130 * Returns an ValueError Exception if the item is not found. 00131 * 00132 * @param plist Ptr to list obj 00133 * @param pitem Ptr to object to be removed 00134 * @param r_index Return by reference; ptr to index (C uint16) 00135 * @return Return status 00136 */ 00137 PmReturn_t list_index(pPmObj_t plist, pPmObj_t pitem, uint16_t *r_index); 00138 00139 /** 00140 * Removes the item at the given index. 00141 * Raises a TypeError if the first argument is not a list. 00142 * Raises an IndexError if the index is out of bounds. 00143 * 00144 * @param plist Ptr to list obj 00145 * @param index Index of item to remove 00146 * @return Return status 00147 */ 00148 PmReturn_t list_delItem(pPmObj_t plist, int16_t index); 00149 00150 #ifdef HAVE_PRINT 00151 /** 00152 * Prints out a list. Uses obj_print() to print elements. 00153 * 00154 * @param pobj Object to print. 00155 * @return Return status 00156 */ 00157 PmReturn_t list_print(pPmObj_t pobj); 00158 #endif /* HAVE_PRINT */ 00159 00160 /** 00161 * Removes all items from the list and zeroes the length. 00162 * 00163 * @param plist List to clear 00164 * @return Return status 00165 */ 00166 PmReturn_t list_clear(pPmObj_t plist); 00167 00168 #endif /* __LIST_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
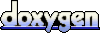