
python-on-a-chip online compiler
obj.h File Reference
Object Type. More...
Go to the source code of this file.
Data Structures | |
struct | PmObj_s |
The abstract empty object type for PyMite. More... | |
struct | PmBoolean_s |
Boolean object. More... | |
Typedefs | |
typedef enum PmType_e | PmType_t |
Object type enum. | |
typedef uint16_t | PmObjDesc_t |
Object Descriptor. | |
typedef struct PmObj_s | PmObj_t |
The abstract empty object type for PyMite. | |
typedef struct PmBoolean_s | PmBoolean_t |
Boolean object. | |
Enumerations | |
enum | PmType_e { , OBJ_TYPE_NON = 0x00, OBJ_TYPE_INT = 0x01, OBJ_TYPE_FLT = 0x02, OBJ_TYPE_STR = 0x03, OBJ_TYPE_TUP = 0x04, OBJ_TYPE_COB = 0x05, OBJ_TYPE_MOD = 0x06, OBJ_TYPE_CLO = 0x07, OBJ_TYPE_FXN = 0x08, OBJ_TYPE_CLI = 0x09, OBJ_TYPE_CIM = 0x0A, OBJ_TYPE_NIM = 0x0B, OBJ_TYPE_NOB = 0x0C, OBJ_TYPE_THR = 0x0D, OBJ_TYPE_BOOL = 0x0F, OBJ_TYPE_CIO = 0x10, OBJ_TYPE_MTH = 0x11 , OBJ_TYPE_LST = 0x12, OBJ_TYPE_DIC = 0x13, OBJ_TYPE_BYA = 0x14 , OBJ_TYPE_BYS = 0x18, OBJ_TYPE_FRM = 0x19, OBJ_TYPE_BLK = 0x1A, OBJ_TYPE_SEG = 0x1B, OBJ_TYPE_SGL = 0x1C, OBJ_TYPE_SQI = 0x1D, OBJ_TYPE_NFM = 0x1E } |
Object type enum. More... | |
Functions | |
PmReturn_t | obj_loadFromImg (PmMemSpace_t memspace, uint8_t const **paddr, pPmObj_t *r_pobj) |
Loads an object from an image in memory. | |
PmReturn_t | obj_loadFromImgObj (pPmObj_t pimg, pPmObj_t *r_pobj) |
Loads a code object from a code image object. | |
int8_t | obj_isFalse (pPmObj_t pobj) |
Finds the boolean value of the given object. | |
PmReturn_t | obj_isIn (pPmObj_t pobj, pPmObj_t pitem) |
Returns the boolean true if the item is in the object. | |
int8_t | obj_compare (pPmObj_t pobj1, pPmObj_t pobj2) |
Compares two objects for equality. | |
PmReturn_t | obj_print (pPmObj_t pobj, uint8_t is_expr_repr, uint8_t is_nested) |
Print an object, thereby using objects helpers. | |
PmReturn_t | obj_repr (pPmObj_t pobj, pPmObj_t *r_pstr) |
Returns by reference a string object that is the human-readable representation of the object. |
Detailed Description
Object Type.
Object type header.
Definition in file obj.h.
Typedef Documentation
typedef struct PmBoolean_s PmBoolean_t |
Boolean object.
typedef uint16_t PmObjDesc_t |
Object Descriptor.
All active PyMite "objects" must have this at the top of their struct. The following is a diagram of the object descriptor:
* MSb LSb * 7 6 5 4 3 2 1 0 * pchunk-> +-+-+-+-+-+-+-+-+ S := Size of the chunk (2 LSbs dropped) * | S |F|M| F := Free bit * +---------+-+-+-+ M := GC Mark bit * | T | S | T := Object type (PyMite specific) * +---------+-----+ * | object data | * ... ... * | end data | * +---------------+ *
The theoretical minimum size of an object descriptor is 2 bytes; however, the effective minimum size must be at least that of the minimum heap descriptor. So on an 8-bit MCU, the minimum size is 8 bytes and on an MCU with 32-bit addresses, the size is 12 bytes.
Object type enum.
These values go in the od_type fields of the obj descriptor. Be sure these values correspond to those in the image creator tool. The hashable types are grouped together for convenience.
WARNING: od_type must be at most 5 bits! (must be < 0x20)
Enumeration Type Documentation
enum PmType_e |
Object type enum.
These values go in the od_type fields of the obj descriptor. Be sure these values correspond to those in the image creator tool. The hashable types are grouped together for convenience.
WARNING: od_type must be at most 5 bits! (must be < 0x20)
- Enumerator:
Function Documentation
int8_t obj_isFalse | ( | pPmObj_t | pobj ) |
PmReturn_t obj_isIn | ( | pPmObj_t | pobj, |
pPmObj_t | pitem | ||
) |
PmReturn_t obj_loadFromImg | ( | PmMemSpace_t | memspace, |
uint8_t const ** | paddr, | ||
pPmObj_t * | r_pobj | ||
) |
Loads an object from an image in memory.
Return pointer to object. Leave add pointing one byte past end of obj.
The following lists the simple object types and their image structures: -None: -type: int8_t - OBJ_TYPE_NON
-Int: -type: int8_t - OBJ_TYPE_INT -value: int32_t - signed integer value
-Float: -type: int8_t - OBJ_TYPE_FLOAT -value: float32_t - 32-bit floating point value
-Slice (is this allowed in img?): -type: int8_t - OBJ_TYPE_SLICE -index1: int16_t - first index. -index2: int16_t - second index.
- Parameters:
-
memspace memory space/type paddr ptr to ptr to obj return by reference: paddr pts to first byte after obj r_pobj Return arg, the loaded object.
- Returns:
- Return status
PmReturn_t obj_loadFromImgObj | ( | pPmObj_t | pimg, |
pPmObj_t * | r_pobj | ||
) |
PmReturn_t obj_print | ( | pPmObj_t | pobj, |
uint8_t | is_expr_repr, | ||
uint8_t | is_nested | ||
) |
Print an object, thereby using objects helpers.
- Parameters:
-
pobj Ptr to object for printing. is_expr_repr Influences the way None and strings are printed. If 0, None is printed, strings are printed. If 1, None is not printed and strings are printed surrounded with single quotes and unprintable characters are escaped. is_nested Influences the way None and strings are printed. If 1, None will be printed and strings will be surrounded with single quotes and escaped. This argument overrides the is_expr_repr argument.
- Returns:
- Return status
PmReturn_t obj_repr | ( | pPmObj_t | pobj, |
pPmObj_t * | r_pstr | ||
) |
Generated on Tue Jul 12 2022 23:13:47 by
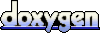