
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
Functions | |
IntStatus | PWM_GetIntStatus (LPC_PWM_TypeDef *PWMx, uint32_t IntFlag) |
Check whether specified interrupt flag in PWM is set or not. | |
void | PWM_ClearIntPending (LPC_PWM_TypeDef *PWMx, uint32_t IntFlag) |
Clear specified PWM Interrupt pending. | |
void | PWM_ConfigStructInit (uint8_t PWMTimerCounterMode, void *PWM_InitStruct) |
Fills each PWM_InitStruct member with its default value:
| |
void | PWM_Init (LPC_PWM_TypeDef *PWMx, uint32_t PWMTimerCounterMode, void *PWM_ConfigStruct) |
Initializes the PWMx peripheral corresponding to the specified parameters in the PWM_ConfigStruct. | |
void | PWM_DeInit (LPC_PWM_TypeDef *PWMx) |
De-initializes the PWM peripheral registers to their default reset values. | |
void | PWM_Cmd (LPC_PWM_TypeDef *PWMx, FunctionalState NewState) |
Enable/Disable PWM peripheral. | |
void | PWM_CounterCmd (LPC_PWM_TypeDef *PWMx, FunctionalState NewState) |
Enable/Disable Counter in PWM peripheral. | |
void | PWM_ResetCounter (LPC_PWM_TypeDef *PWMx) |
Reset Counter in PWM peripheral. | |
void | PWM_ConfigMatch (LPC_PWM_TypeDef *PWMx, PWM_MATCHCFG_Type *PWM_MatchConfigStruct) |
Configures match for PWM peripheral. | |
void | PWM_ConfigCapture (LPC_PWM_TypeDef *PWMx, PWM_CAPTURECFG_Type *PWM_CaptureConfigStruct) |
Configures capture input for PWM peripheral. | |
uint32_t | PWM_GetCaptureValue (LPC_PWM_TypeDef *PWMx, uint8_t CaptureChannel) |
Read value of capture register PWM peripheral. | |
void | PWM_MatchUpdate (LPC_PWM_TypeDef *PWMx, uint8_t MatchChannel,\uint32_t MatchValue, uint8_t UpdateType) |
Update value for each PWM channel with update type option. | |
void | PWM_ChannelConfig (LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, uint8_t ModeOption) |
Configure Edge mode for each PWM channel. | |
void | PWM_ChannelCmd (LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, FunctionalState NewState) |
Enable/Disable PWM channel output. |
Function Documentation
void PWM_ChannelCmd | ( | LPC_PWM_TypeDef * | PWMx, |
uint8_t | PWMChannel, | ||
FunctionalState | NewState | ||
) |
Enable/Disable PWM channel output.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] PWMChannel PWM channel, should be in range from 1 to 6 [in] NewState New State of this function, should be: - ENABLE: Enable this PWM channel output
- DISABLE: Disable this PWM channel output
- Returns:
- None
Definition at line 507 of file lpc17xx_pwm.c.
void PWM_ChannelConfig | ( | LPC_PWM_TypeDef * | PWMx, |
uint8_t | PWMChannel, | ||
uint8_t | ModeOption | ||
) |
Configure Edge mode for each PWM channel.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] PWMChannel PWM channel, should be in range from 2 to 6 [in] ModeOption PWM mode option, should be: - PWM_CHANNEL_SINGLE_EDGE: Single Edge mode
- PWM_CHANNEL_DUAL_EDGE: Dual Edge mode
- Returns:
- None Note: PWM Channel 1 can not be selected for mode option
Definition at line 478 of file lpc17xx_pwm.c.
void PWM_ClearIntPending | ( | LPC_PWM_TypeDef * | PWMx, |
uint32_t | IntFlag | ||
) |
Clear specified PWM Interrupt pending.
- Parameters:
-
[in] PWMx,: PWM peripheral, should be PWM1 [in] IntFlag,: PWM interrupt flag, should be: - PWM_INTSTAT_MR0: Interrupt flag for PWM match channel 0
- PWM_INTSTAT_MR1: Interrupt flag for PWM match channel 1
- PWM_INTSTAT_MR2: Interrupt flag for PWM match channel 2
- PWM_INTSTAT_MR3: Interrupt flag for PWM match channel 3
- PWM_INTSTAT_MR4: Interrupt flag for PWM match channel 4
- PWM_INTSTAT_MR5: Interrupt flag for PWM match channel 5
- PWM_INTSTAT_MR6: Interrupt flag for PWM match channel 6
- PWM_INTSTAT_CAP0: Interrupt flag for capture input 0
- PWM_INTSTAT_CAP1: Interrupt flag for capture input 1
- Returns:
- None
Definition at line 89 of file lpc17xx_pwm.c.
void PWM_Cmd | ( | LPC_PWM_TypeDef * | PWMx, |
FunctionalState | NewState | ||
) |
Enable/Disable PWM peripheral.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] NewState New State of this function, should be: - ENABLE: Enable PWM peripheral
- DISABLE: Disable PWM peripheral
- Returns:
- None
Definition at line 229 of file lpc17xx_pwm.c.
void PWM_ConfigCapture | ( | LPC_PWM_TypeDef * | PWMx, |
PWM_CAPTURECFG_Type * | PWM_CaptureConfigStruct | ||
) |
Configures capture input for PWM peripheral.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] PWM_CaptureConfigStruct Pointer to a PWM_CAPTURECFG_Type structure that contains the configuration information for the specified PWM capture input function.
- Returns:
- None
Definition at line 340 of file lpc17xx_pwm.c.
void PWM_ConfigMatch | ( | LPC_PWM_TypeDef * | PWMx, |
PWM_MATCHCFG_Type * | PWM_MatchConfigStruct | ||
) |
Configures match for PWM peripheral.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] PWM_MatchConfigStruct Pointer to a PWM_MATCHCFG_Type structure that contains the configuration information for the specified PWM match function.
- Returns:
- None
Definition at line 289 of file lpc17xx_pwm.c.
void PWM_ConfigStructInit | ( | uint8_t | PWMTimerCounterMode, |
void * | PWM_InitStruct | ||
) |
Fills each PWM_InitStruct member with its default value:
- If PWMCounterMode = PWM_MODE_TIMER: + PrescaleOption = PWM_TIMER_PRESCALE_USVAL + PrescaleValue = 1
- If PWMCounterMode = PWM_MODE_COUNTER: + CountInputSelect = PWM_COUNTER_PCAP1_0 + CounterOption = PWM_COUNTER_RISING.
- Parameters:
-
[in] PWMTimerCounterMode Timer or Counter mode, should be: - PWM_MODE_TIMER: Counter of PWM peripheral is in Timer mode
- PWM_MODE_COUNTER: Counter of PWM peripheral is in Counter mode
[in] PWM_InitStruct Pointer to structure (PWM_TIMERCFG_Type or PWM_COUNTERCFG_Type) which will be initialized.
- Returns:
- None Note: PWM_InitStruct pointer will be assigned to corresponding structure (PWM_TIMERCFG_Type or PWM_COUNTERCFG_Type) due to PWMTimerCounterMode.
Definition at line 115 of file lpc17xx_pwm.c.
void PWM_CounterCmd | ( | LPC_PWM_TypeDef * | PWMx, |
FunctionalState | NewState | ||
) |
Enable/Disable Counter in PWM peripheral.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] NewState New State of this function, should be: - ENABLE: Enable Counter in PWM peripheral
- DISABLE: Disable Counter in PWM peripheral
- Returns:
- None
Definition at line 253 of file lpc17xx_pwm.c.
void PWM_DeInit | ( | LPC_PWM_TypeDef * | PWMx ) |
De-initializes the PWM peripheral registers to their default reset values.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1
- Returns:
- None
Definition at line 207 of file lpc17xx_pwm.c.
uint32_t PWM_GetCaptureValue | ( | LPC_PWM_TypeDef * | PWMx, |
uint8_t | CaptureChannel | ||
) |
Read value of capture register PWM peripheral.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] CaptureChannel,: capture channel number, should be in range 0 to 1
- Returns:
- Value of capture register
Definition at line 387 of file lpc17xx_pwm.c.
IntStatus PWM_GetIntStatus | ( | LPC_PWM_TypeDef * | PWMx, |
uint32_t | IntFlag | ||
) |
Check whether specified interrupt flag in PWM is set or not.
- Parameters:
-
[in] PWMx,: PWM peripheral, should be PWM1 [in] IntFlag,: PWM interrupt flag, should be: - PWM_INTSTAT_MR0: Interrupt flag for PWM match channel 0
- PWM_INTSTAT_MR1: Interrupt flag for PWM match channel 1
- PWM_INTSTAT_MR2: Interrupt flag for PWM match channel 2
- PWM_INTSTAT_MR3: Interrupt flag for PWM match channel 3
- PWM_INTSTAT_MR4: Interrupt flag for PWM match channel 4
- PWM_INTSTAT_MR5: Interrupt flag for PWM match channel 5
- PWM_INTSTAT_MR6: Interrupt flag for PWM match channel 6
- PWM_INTSTAT_CAP0: Interrupt flag for capture input 0
- PWM_INTSTAT_CAP1: Interrupt flag for capture input 1
- Returns:
- New State of PWM interrupt flag (SET or RESET)
Definition at line 64 of file lpc17xx_pwm.c.
void PWM_Init | ( | LPC_PWM_TypeDef * | PWMx, |
uint32_t | PWMTimerCounterMode, | ||
void * | PWM_ConfigStruct | ||
) |
Initializes the PWMx peripheral corresponding to the specified parameters in the PWM_ConfigStruct.
- Parameters:
-
[in] PWMx PWM peripheral, should be PWM1 [in] PWMTimerCounterMode Timer or Counter mode, should be: - PWM_MODE_TIMER: Counter of PWM peripheral is in Timer mode
- PWM_MODE_COUNTER: Counter of PWM peripheral is in Counter mode
[in] PWM_ConfigStruct Pointer to structure (PWM_TIMERCFG_Type or PWM_COUNTERCFG_Type) which will be initialized.
- Returns:
- None Note: PWM_ConfigStruct pointer will be assigned to corresponding structure (PWM_TIMERCFG_Type or PWM_COUNTERCFG_Type) due to PWMTimerCounterMode.
Definition at line 148 of file lpc17xx_pwm.c.
void PWM_MatchUpdate | ( | LPC_PWM_TypeDef * | PWMx, |
uint8_t | MatchChannel, | ||
\uint32_t | MatchValue, | ||
uint8_t | UpdateType | ||
) |
Update value for each PWM channel with update type option.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1 [in] MatchChannel Match channel [in] MatchValue Match value [in] UpdateType Type of Update, should be: - PWM_MATCH_UPDATE_NOW: The update value will be updated for this channel immediately
- PWM_MATCH_UPDATE_NEXT_RST: The update value will be updated for this channel on next reset by a PWM Match event.
- Returns:
- None
Definition at line 418 of file lpc17xx_pwm.c.
void PWM_ResetCounter | ( | LPC_PWM_TypeDef * | PWMx ) |
Reset Counter in PWM peripheral.
- Parameters:
-
[in] PWMx PWM peripheral selected, should be PWM1
- Returns:
- None
Definition at line 273 of file lpc17xx_pwm.c.
Generated on Tue Jul 12 2022 17:06:03 by
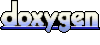