
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_pwm.c
00001 /** 00002 * @file : lpc17xx_pwm.c 00003 * @brief : Contains all functions support for PWM firmware library on LPC17xx 00004 * @version : 1.0 00005 * @date : 22. Apr. 2009 00006 * @author : HieuNguyen 00007 ************************************************************************** 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 /* Peripheral group ----------------------------------------------------------- */ 00021 /** @addtogroup PWM 00022 * @{ 00023 */ 00024 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_pwm.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 /* If this source file built with example, the LPC17xx FW library configuration 00030 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00031 * otherwise the default FW library configuration file must be included instead 00032 */ 00033 #ifdef __BUILD_WITH_EXAMPLE__ 00034 #include "lpc17xx_libcfg.h" 00035 #else 00036 #include "lpc17xx_libcfg_default.h" 00037 #endif /* __BUILD_WITH_EXAMPLE__ */ 00038 00039 00040 #ifdef _PWM 00041 00042 00043 /* Public Functions ----------------------------------------------------------- */ 00044 /** @addtogroup PWM_Public_Functions 00045 * @{ 00046 */ 00047 00048 00049 /*********************************************************************//** 00050 * @brief Check whether specified interrupt flag in PWM is set or not 00051 * @param[in] PWMx: PWM peripheral, should be PWM1 00052 * @param[in] IntFlag: PWM interrupt flag, should be: 00053 * - PWM_INTSTAT_MR0: Interrupt flag for PWM match channel 0 00054 * - PWM_INTSTAT_MR1: Interrupt flag for PWM match channel 1 00055 * - PWM_INTSTAT_MR2: Interrupt flag for PWM match channel 2 00056 * - PWM_INTSTAT_MR3: Interrupt flag for PWM match channel 3 00057 * - PWM_INTSTAT_MR4: Interrupt flag for PWM match channel 4 00058 * - PWM_INTSTAT_MR5: Interrupt flag for PWM match channel 5 00059 * - PWM_INTSTAT_MR6: Interrupt flag for PWM match channel 6 00060 * - PWM_INTSTAT_CAP0: Interrupt flag for capture input 0 00061 * - PWM_INTSTAT_CAP1: Interrupt flag for capture input 1 00062 * @return New State of PWM interrupt flag (SET or RESET) 00063 **********************************************************************/ 00064 IntStatus PWM_GetIntStatus(LPC_PWM_TypeDef *PWMx, uint32_t IntFlag) 00065 { 00066 CHECK_PARAM(PARAM_PWMx(PWMx)); 00067 CHECK_PARAM(PARAM_PWM_INTSTAT(IntFlag)); 00068 00069 return ((PWMx->IR & IntFlag) ? SET : RESET); 00070 } 00071 00072 00073 00074 /*********************************************************************//** 00075 * @brief Clear specified PWM Interrupt pending 00076 * @param[in] PWMx: PWM peripheral, should be PWM1 00077 * @param[in] IntFlag: PWM interrupt flag, should be: 00078 * - PWM_INTSTAT_MR0: Interrupt flag for PWM match channel 0 00079 * - PWM_INTSTAT_MR1: Interrupt flag for PWM match channel 1 00080 * - PWM_INTSTAT_MR2: Interrupt flag for PWM match channel 2 00081 * - PWM_INTSTAT_MR3: Interrupt flag for PWM match channel 3 00082 * - PWM_INTSTAT_MR4: Interrupt flag for PWM match channel 4 00083 * - PWM_INTSTAT_MR5: Interrupt flag for PWM match channel 5 00084 * - PWM_INTSTAT_MR6: Interrupt flag for PWM match channel 6 00085 * - PWM_INTSTAT_CAP0: Interrupt flag for capture input 0 00086 * - PWM_INTSTAT_CAP1: Interrupt flag for capture input 1 00087 * @return None 00088 **********************************************************************/ 00089 void PWM_ClearIntPending(LPC_PWM_TypeDef *PWMx, uint32_t IntFlag) 00090 { 00091 CHECK_PARAM(PARAM_PWMx(PWMx)); 00092 CHECK_PARAM(PARAM_PWM_INTSTAT(IntFlag)); 00093 PWMx->IR = IntFlag; 00094 } 00095 00096 00097 00098 /*****************************************************************************//** 00099 * @brief Fills each PWM_InitStruct member with its default value: 00100 * - If PWMCounterMode = PWM_MODE_TIMER: 00101 * + PrescaleOption = PWM_TIMER_PRESCALE_USVAL 00102 * + PrescaleValue = 1 00103 * - If PWMCounterMode = PWM_MODE_COUNTER: 00104 * + CountInputSelect = PWM_COUNTER_PCAP1_0 00105 * + CounterOption = PWM_COUNTER_RISING 00106 * @param[in] PWMTimerCounterMode Timer or Counter mode, should be: 00107 * - PWM_MODE_TIMER: Counter of PWM peripheral is in Timer mode 00108 * - PWM_MODE_COUNTER: Counter of PWM peripheral is in Counter mode 00109 * @param[in] PWM_InitStruct Pointer to structure (PWM_TIMERCFG_Type or 00110 * PWM_COUNTERCFG_Type) which will be initialized. 00111 * @return None 00112 * Note: PWM_InitStruct pointer will be assigned to corresponding structure 00113 * (PWM_TIMERCFG_Type or PWM_COUNTERCFG_Type) due to PWMTimerCounterMode. 00114 *******************************************************************************/ 00115 void PWM_ConfigStructInit(uint8_t PWMTimerCounterMode, void *PWM_InitStruct) 00116 { 00117 CHECK_PARAM(PARAM_PWM_TC_MODE(PWMTimerCounterMode)); 00118 00119 PWM_TIMERCFG_Type *pTimeCfg = (PWM_TIMERCFG_Type *) PWM_InitStruct; 00120 PWM_COUNTERCFG_Type *pCounterCfg = (PWM_COUNTERCFG_Type *) PWM_InitStruct; 00121 00122 if (PWMTimerCounterMode == PWM_MODE_TIMER ) 00123 { 00124 pTimeCfg->PrescaleOption = PWM_TIMER_PRESCALE_USVAL ; 00125 pTimeCfg->PrescaleValue = 1; 00126 } 00127 else if (PWMTimerCounterMode == PWM_MODE_COUNTER ) 00128 { 00129 pCounterCfg->CountInputSelect = PWM_COUNTER_PCAP1_0 ; 00130 pCounterCfg->CounterOption = PWM_COUNTER_RISING ; 00131 } 00132 } 00133 00134 00135 /*********************************************************************//** 00136 * @brief Initializes the PWMx peripheral corresponding to the specified 00137 * parameters in the PWM_ConfigStruct. 00138 * @param[in] PWMx PWM peripheral, should be PWM1 00139 * @param[in] PWMTimerCounterMode Timer or Counter mode, should be: 00140 * - PWM_MODE_TIMER: Counter of PWM peripheral is in Timer mode 00141 * - PWM_MODE_COUNTER: Counter of PWM peripheral is in Counter mode 00142 * @param[in] PWM_ConfigStruct Pointer to structure (PWM_TIMERCFG_Type or 00143 * PWM_COUNTERCFG_Type) which will be initialized. 00144 * @return None 00145 * Note: PWM_ConfigStruct pointer will be assigned to corresponding structure 00146 * (PWM_TIMERCFG_Type or PWM_COUNTERCFG_Type) due to PWMTimerCounterMode. 00147 **********************************************************************/ 00148 void PWM_Init(LPC_PWM_TypeDef *PWMx, uint32_t PWMTimerCounterMode, void *PWM_ConfigStruct) 00149 { 00150 CHECK_PARAM(PARAM_PWMx(PWMx)); 00151 CHECK_PARAM(PARAM_PWM_TC_MODE(PWMTimerCounterMode)); 00152 00153 PWM_TIMERCFG_Type *pTimeCfg = (PWM_TIMERCFG_Type *)PWM_ConfigStruct; 00154 PWM_COUNTERCFG_Type *pCounterCfg = (PWM_COUNTERCFG_Type *)PWM_ConfigStruct; 00155 uint64_t clkdlycnt=0; 00156 00157 if (PWMx == LPC_PWM1) 00158 { 00159 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCPWM1, ENABLE); 00160 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_PWM1, CLKPWR_PCLKSEL_CCLK_DIV_4); 00161 // Get peripheral clock of PWM1 00162 clkdlycnt = (uint64_t) CLKPWR_GetPCLK (CLKPWR_PCLKSEL_PWM1); 00163 } 00164 00165 // Clear all interrupts pending 00166 PWMx->IR = 0xFF & PWM_IR_BITMASK; 00167 PWMx->TCR = 0x00; 00168 PWMx->CTCR = 0x00; 00169 PWMx->MCR = 0x00; 00170 PWMx->CCR = 0x00; 00171 PWMx->PCR = 0x00; 00172 PWMx->LER = 0x00; 00173 00174 if (PWMTimerCounterMode == PWM_MODE_TIMER ) 00175 { 00176 CHECK_PARAM(PARAM_PWM_TIMER_PRESCALE(pTimeCfg->PrescaleOption)); 00177 00178 /* Absolute prescale value */ 00179 if (pTimeCfg->PrescaleOption == PWM_TIMER_PRESCALE_TICKVAL ) 00180 { 00181 PWMx->PR = pTimeCfg->PrescaleValue - 1; 00182 } 00183 /* uSecond prescale value */ 00184 else 00185 { 00186 clkdlycnt = (clkdlycnt * pTimeCfg->PrescaleValue) / 1000000; 00187 PWMx->PR = ((uint32_t) clkdlycnt) - 1; 00188 } 00189 00190 } 00191 else if (PWMTimerCounterMode == PWM_MODE_COUNTER ) 00192 { 00193 CHECK_PARAM(PARAM_PWM_COUNTER_INPUTSEL(pCounterCfg->CountInputSelect)); 00194 CHECK_PARAM(PARAM_PWM_COUNTER_EDGE(pCounterCfg->CounterOption)); 00195 00196 PWMx->CTCR |= (PWM_CTCR_MODE((uint32_t)pCounterCfg->CounterOption)) \ 00197 | (PWM_CTCR_SELECT_INPUT((uint32_t)pCounterCfg->CountInputSelect)); 00198 } 00199 } 00200 00201 /*********************************************************************//** 00202 * @brief De-initializes the PWM peripheral registers to their 00203 * default reset values. 00204 * @param[in] PWMx PWM peripheral selected, should be PWM1 00205 * @return None 00206 **********************************************************************/ 00207 void PWM_DeInit (LPC_PWM_TypeDef *PWMx) 00208 { 00209 CHECK_PARAM(PARAM_PWMx(PWMx)); 00210 00211 // Disable PWM control (timer, counter and PWM) 00212 PWMx->TCR = 0x00; 00213 00214 if (PWMx == LPC_PWM1) 00215 { 00216 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCPWM1, DISABLE); 00217 } 00218 } 00219 00220 00221 /*********************************************************************//** 00222 * @brief Enable/Disable PWM peripheral 00223 * @param[in] PWMx PWM peripheral selected, should be PWM1 00224 * @param[in] NewState New State of this function, should be: 00225 * - ENABLE: Enable PWM peripheral 00226 * - DISABLE: Disable PWM peripheral 00227 * @return None 00228 **********************************************************************/ 00229 void PWM_Cmd(LPC_PWM_TypeDef *PWMx, FunctionalState NewState) 00230 { 00231 CHECK_PARAM(PARAM_PWMx(PWMx)); 00232 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00233 00234 if (NewState == ENABLE) 00235 { 00236 PWMx->TCR |= PWM_TCR_PWM_ENABLE; 00237 } 00238 else 00239 { 00240 PWMx->TCR &= (~PWM_TCR_PWM_ENABLE) & PWM_TCR_BITMASK; 00241 } 00242 } 00243 00244 00245 /*********************************************************************//** 00246 * @brief Enable/Disable Counter in PWM peripheral 00247 * @param[in] PWMx PWM peripheral selected, should be PWM1 00248 * @param[in] NewState New State of this function, should be: 00249 * - ENABLE: Enable Counter in PWM peripheral 00250 * - DISABLE: Disable Counter in PWM peripheral 00251 * @return None 00252 **********************************************************************/ 00253 void PWM_CounterCmd(LPC_PWM_TypeDef *PWMx, FunctionalState NewState) 00254 { 00255 CHECK_PARAM(PARAM_PWMx(PWMx)); 00256 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00257 if (NewState == ENABLE) 00258 { 00259 PWMx->TCR |= PWM_TCR_COUNTER_ENABLE; 00260 } 00261 else 00262 { 00263 PWMx->TCR &= (~PWM_TCR_COUNTER_ENABLE) & PWM_TCR_BITMASK; 00264 } 00265 } 00266 00267 00268 /*********************************************************************//** 00269 * @brief Reset Counter in PWM peripheral 00270 * @param[in] PWMx PWM peripheral selected, should be PWM1 00271 * @return None 00272 **********************************************************************/ 00273 void PWM_ResetCounter(LPC_PWM_TypeDef *PWMx) 00274 { 00275 CHECK_PARAM(PARAM_PWMx(PWMx)); 00276 PWMx->TCR |= PWM_TCR_COUNTER_RESET; 00277 PWMx->TCR &= (~PWM_TCR_COUNTER_RESET) & PWM_TCR_BITMASK; 00278 } 00279 00280 00281 /*********************************************************************//** 00282 * @brief Configures match for PWM peripheral 00283 * @param[in] PWMx PWM peripheral selected, should be PWM1 00284 * @param[in] PWM_MatchConfigStruct Pointer to a PWM_MATCHCFG_Type structure 00285 * that contains the configuration information for the 00286 * specified PWM match function. 00287 * @return None 00288 **********************************************************************/ 00289 void PWM_ConfigMatch(LPC_PWM_TypeDef *PWMx, PWM_MATCHCFG_Type *PWM_MatchConfigStruct) 00290 { 00291 CHECK_PARAM(PARAM_PWMx(PWMx)); 00292 CHECK_PARAM(PARAM_PWM1_MATCH_CHANNEL(PWM_MatchConfigStruct->MatchChannel)); 00293 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_MatchConfigStruct->IntOnMatch)); 00294 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_MatchConfigStruct->ResetOnMatch)); 00295 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_MatchConfigStruct->StopOnMatch)); 00296 00297 //interrupt on MRn 00298 if (PWM_MatchConfigStruct->IntOnMatch == ENABLE) 00299 { 00300 PWMx->MCR |= PWM_MCR_INT_ON_MATCH(PWM_MatchConfigStruct->MatchChannel); 00301 } 00302 else 00303 { 00304 PWMx->MCR &= (~PWM_MCR_INT_ON_MATCH(PWM_MatchConfigStruct->MatchChannel)) \ 00305 & PWM_MCR_BITMASK; 00306 } 00307 00308 //reset on MRn 00309 if (PWM_MatchConfigStruct->ResetOnMatch == ENABLE) 00310 { 00311 PWMx->MCR |= PWM_MCR_RESET_ON_MATCH(PWM_MatchConfigStruct->MatchChannel); 00312 } 00313 else 00314 { 00315 PWMx->MCR &= (~PWM_MCR_RESET_ON_MATCH(PWM_MatchConfigStruct->MatchChannel)) \ 00316 & PWM_MCR_BITMASK; 00317 } 00318 00319 //stop on MRn 00320 if (PWM_MatchConfigStruct->StopOnMatch == ENABLE) 00321 { 00322 PWMx->MCR |= PWM_MCR_STOP_ON_MATCH(PWM_MatchConfigStruct->MatchChannel); 00323 } 00324 else 00325 { 00326 PWMx->MCR &= (~PWM_MCR_STOP_ON_MATCH(PWM_MatchConfigStruct->MatchChannel)) \ 00327 & PWM_MCR_BITMASK; 00328 } 00329 } 00330 00331 00332 /*********************************************************************//** 00333 * @brief Configures capture input for PWM peripheral 00334 * @param[in] PWMx PWM peripheral selected, should be PWM1 00335 * @param[in] PWM_CaptureConfigStruct Pointer to a PWM_CAPTURECFG_Type structure 00336 * that contains the configuration information for the 00337 * specified PWM capture input function. 00338 * @return None 00339 **********************************************************************/ 00340 void PWM_ConfigCapture(LPC_PWM_TypeDef *PWMx, PWM_CAPTURECFG_Type *PWM_CaptureConfigStruct) 00341 { 00342 CHECK_PARAM(PARAM_PWMx(PWMx)); 00343 CHECK_PARAM(PARAM_PWM1_CAPTURE_CHANNEL(PWM_CaptureConfigStruct->CaptureChannel)); 00344 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_CaptureConfigStruct->FallingEdge)); 00345 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_CaptureConfigStruct->IntOnCaption)); 00346 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_CaptureConfigStruct->RisingEdge)); 00347 00348 if (PWM_CaptureConfigStruct->RisingEdge == ENABLE) 00349 { 00350 PWMx->CCR |= PWM_CCR_CAP_RISING(PWM_CaptureConfigStruct->CaptureChannel); 00351 } 00352 else 00353 { 00354 PWMx->CCR &= (~PWM_CCR_CAP_RISING(PWM_CaptureConfigStruct->CaptureChannel)) \ 00355 & PWM_CCR_BITMASK; 00356 } 00357 00358 if (PWM_CaptureConfigStruct->FallingEdge == ENABLE) 00359 { 00360 PWMx->CCR |= PWM_CCR_CAP_FALLING(PWM_CaptureConfigStruct->CaptureChannel); 00361 } 00362 else 00363 { 00364 PWMx->CCR &= (~PWM_CCR_CAP_FALLING(PWM_CaptureConfigStruct->CaptureChannel)) \ 00365 & PWM_CCR_BITMASK; 00366 } 00367 00368 if (PWM_CaptureConfigStruct->IntOnCaption == ENABLE) 00369 { 00370 PWMx->CCR |= PWM_CCR_INT_ON_CAP(PWM_CaptureConfigStruct->CaptureChannel); 00371 } 00372 else 00373 { 00374 PWMx->CCR &= (~PWM_CCR_INT_ON_CAP(PWM_CaptureConfigStruct->CaptureChannel)) \ 00375 & PWM_CCR_BITMASK; 00376 } 00377 } 00378 00379 00380 /*********************************************************************//** 00381 * @brief Read value of capture register PWM peripheral 00382 * @param[in] PWMx PWM peripheral selected, should be PWM1 00383 * @param[in] CaptureChannel: capture channel number, should be in 00384 * range 0 to 1 00385 * @return Value of capture register 00386 **********************************************************************/ 00387 uint32_t PWM_GetCaptureValue(LPC_PWM_TypeDef *PWMx, uint8_t CaptureChannel) 00388 { 00389 CHECK_PARAM(PARAM_PWMx(PWMx)); 00390 CHECK_PARAM(PARAM_PWM1_CAPTURE_CHANNEL(CaptureChannel)); 00391 00392 switch (CaptureChannel) 00393 { 00394 case 0: 00395 return PWMx->CR0; 00396 00397 case 1: 00398 return PWMx->CR1; 00399 00400 default: 00401 return (0); 00402 } 00403 } 00404 00405 00406 /********************************************************************//** 00407 * @brief Update value for each PWM channel with update type option 00408 * @param[in] PWMx PWM peripheral selected, should be PWM1 00409 * @param[in] MatchChannel Match channel 00410 * @param[in] MatchValue Match value 00411 * @param[in] UpdateType Type of Update, should be: 00412 * - PWM_MATCH_UPDATE_NOW: The update value will be updated for 00413 * this channel immediately 00414 * - PWM_MATCH_UPDATE_NEXT_RST: The update value will be updated for 00415 * this channel on next reset by a PWM Match event. 00416 * @return None 00417 *********************************************************************/ 00418 void PWM_MatchUpdate(LPC_PWM_TypeDef *PWMx, uint8_t MatchChannel, \ 00419 uint32_t MatchValue, uint8_t UpdateType) 00420 { 00421 CHECK_PARAM(PARAM_PWMx(PWMx)); 00422 CHECK_PARAM(PARAM_PWM1_MATCH_CHANNEL(MatchChannel)); 00423 CHECK_PARAM(PARAM_PWM_MATCH_UPDATE(UpdateType)); 00424 00425 switch (MatchChannel) 00426 { 00427 case 0: 00428 PWMx->MR0 = MatchValue; 00429 break; 00430 00431 case 1: 00432 PWMx->MR1 = MatchValue; 00433 break; 00434 00435 case 2: 00436 PWMx->MR2 = MatchValue; 00437 break; 00438 00439 case 3: 00440 PWMx->MR3 = MatchValue; 00441 break; 00442 00443 case 4: 00444 PWMx->MR4 = MatchValue; 00445 break; 00446 00447 case 5: 00448 PWMx->MR5 = MatchValue; 00449 break; 00450 00451 case 6: 00452 PWMx->MR6 = MatchValue; 00453 break; 00454 } 00455 00456 // Write Latch register 00457 PWMx->LER |= PWM_LER_EN_MATCHn_LATCH(MatchChannel); 00458 00459 // In case of update now 00460 if (UpdateType == PWM_MATCH_UPDATE_NOW) 00461 { 00462 PWMx->TCR |= PWM_TCR_COUNTER_RESET; 00463 PWMx->TCR &= (~PWM_TCR_COUNTER_RESET) & PWM_TCR_BITMASK; 00464 } 00465 } 00466 00467 00468 /********************************************************************//** 00469 * @brief Configure Edge mode for each PWM channel 00470 * @param[in] PWMx PWM peripheral selected, should be PWM1 00471 * @param[in] PWMChannel PWM channel, should be in range from 2 to 6 00472 * @param[in] ModeOption PWM mode option, should be: 00473 * - PWM_CHANNEL_SINGLE_EDGE: Single Edge mode 00474 * - PWM_CHANNEL_DUAL_EDGE: Dual Edge mode 00475 * @return None 00476 * Note: PWM Channel 1 can not be selected for mode option 00477 *********************************************************************/ 00478 void PWM_ChannelConfig(LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, uint8_t ModeOption) 00479 { 00480 CHECK_PARAM(PARAM_PWMx(PWMx)); 00481 CHECK_PARAM(PARAM_PWM1_EDGE_MODE_CHANNEL(PWMChannel)); 00482 CHECK_PARAM(PARAM_PWM_CHANNEL_EDGE(ModeOption)); 00483 00484 // Single edge mode 00485 if (ModeOption == PWM_CHANNEL_SINGLE_EDGE ) 00486 { 00487 PWMx->PCR &= (~PWM_PCR_PWMSELn(PWMChannel)) & PWM_PCR_BITMASK; 00488 } 00489 // Double edge mode 00490 else if (PWM_CHANNEL_DUAL_EDGE ) 00491 { 00492 PWMx->PCR |= PWM_PCR_PWMSELn(PWMChannel); 00493 } 00494 } 00495 00496 00497 00498 /********************************************************************//** 00499 * @brief Enable/Disable PWM channel output 00500 * @param[in] PWMx PWM peripheral selected, should be PWM1 00501 * @param[in] PWMChannel PWM channel, should be in range from 1 to 6 00502 * @param[in] NewState New State of this function, should be: 00503 * - ENABLE: Enable this PWM channel output 00504 * - DISABLE: Disable this PWM channel output 00505 * @return None 00506 *********************************************************************/ 00507 void PWM_ChannelCmd(LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, FunctionalState NewState) 00508 { 00509 CHECK_PARAM(PARAM_PWMx(PWMx)); 00510 CHECK_PARAM(PARAM_PWM1_CHANNEL(PWMChannel)); 00511 00512 if (NewState == ENABLE) 00513 { 00514 PWMx->PCR |= PWM_PCR_PWMENAn(PWMChannel); 00515 } 00516 else 00517 { 00518 PWMx->PCR &= (~PWM_PCR_PWMENAn(PWMChannel)) & PWM_PCR_BITMASK; 00519 } 00520 } 00521 00522 /** 00523 * @} 00524 */ 00525 00526 #endif /* _PWM */ 00527 00528 /** 00529 * @} 00530 */ 00531 00532 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
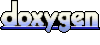