
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
Functions | |
void | ADC_Init (LPC_ADC_TypeDef *ADCx, uint32_t ConvFreq) |
Initial for ADC
| |
void | ADC_DeInit (LPC_ADC_TypeDef *ADCx) |
Close ADC. | |
void | ADC_BurstCmd (LPC_ADC_TypeDef *ADCx, FunctionalState NewState) |
ADC Burst mode setting. | |
void | ADC_PowerdownCmd (LPC_ADC_TypeDef *ADCx, FunctionalState NewState) |
Set AD conversion in power mode. | |
void | ADC_StartCmd (LPC_ADC_TypeDef *ADCx, uint8_t start_mode) |
Set start mode for ADC. | |
void | ADC_EdgeStartConfig (LPC_ADC_TypeDef *ADCx, uint8_t EdgeOption) |
Set Edge start configuration. | |
void | ADC_IntConfig (LPC_ADC_TypeDef *ADCx, ADC_TYPE_INT_OPT IntType, FunctionalState NewState) |
ADC interrupt configuration. | |
void | ADC_ChannelCmd (LPC_ADC_TypeDef *ADCx, uint8_t Channel, FunctionalState NewState) |
Enable/Disable ADC channel number. | |
uint16_t | ADC_ChannelGetData (LPC_ADC_TypeDef *ADCx, uint8_t channel) |
Get ADC result. | |
FlagStatus | ADC_ChannelGetStatus (LPC_ADC_TypeDef *ADCx, uint8_t channel, uint32_t StatusType) |
Get ADC Chanel status from ADC data register. | |
uint16_t | ADC_GlobalGetData (LPC_ADC_TypeDef *ADCx, uint8_t channel) |
Get ADC Data from AD Global register. | |
FlagStatus | ADC_GlobalGetStatus (LPC_ADC_TypeDef *ADCx, uint32_t StatusType) |
Get ADC Chanel status from AD global data register. | |
uint32_t | ADC_GetData (uint32_t channel) |
Get Result conversion from A/D data register. |
Function Documentation
void ADC_BurstCmd | ( | LPC_ADC_TypeDef * | ADCx, |
FunctionalState | NewState | ||
) |
ADC Burst mode setting.
- Parameters:
-
[in] ADCx pointer to ADC [in] NewState - 1: Set Burst mode
- 0: reset Burst mode
- Returns:
- None
Definition at line 140 of file lpc17xx_adc.c.
void ADC_ChannelCmd | ( | LPC_ADC_TypeDef * | ADCx, |
uint8_t | Channel, | ||
FunctionalState | NewState | ||
) |
Enable/Disable ADC channel number.
- Parameters:
-
[in] ADCx pointer to ADC [in] Channel channel number [in] NewState Enable or Disable
- Returns:
- None
Definition at line 220 of file lpc17xx_adc.c.
uint16_t ADC_ChannelGetData | ( | LPC_ADC_TypeDef * | ADCx, |
uint8_t | channel | ||
) |
Get ADC result.
- Parameters:
-
[in] ADCx pointer to ADC [in] channel channel number
- Returns:
- Data conversion
Definition at line 238 of file lpc17xx_adc.c.
FlagStatus ADC_ChannelGetStatus | ( | LPC_ADC_TypeDef * | ADCx, |
uint8_t | channel, | ||
uint32_t | StatusType | ||
) |
Get ADC Chanel status from ADC data register.
- Parameters:
-
[in] ADCx pointer to ADC [in] channel channel number [in] StatusType 0:Burst status 1:Done status
- Returns:
- SET / RESET
Definition at line 258 of file lpc17xx_adc.c.
void ADC_DeInit | ( | LPC_ADC_TypeDef * | ADCx ) |
Close ADC.
- Parameters:
-
[in] ADCx pointer to ADC
- Returns:
- None
Definition at line 88 of file lpc17xx_adc.c.
void ADC_EdgeStartConfig | ( | LPC_ADC_TypeDef * | ADCx, |
uint8_t | EdgeOption | ||
) |
Set Edge start configuration.
- Parameters:
-
[in] ADCx pointer to ADC [in] EdgeOption is ADC_START_ON_RISING and ADC_START_ON_FALLING 0:ADC_START_ON_RISING 1:ADC_START_ON_FALLING
- Returns:
- None
Definition at line 180 of file lpc17xx_adc.c.
uint32_t ADC_GetData | ( | uint32_t | channel ) |
Get Result conversion from A/D data register.
- Parameters:
-
[in] channel number which want to read back the result
- Returns:
- Result of conversion
Definition at line 104 of file lpc17xx_adc.c.
uint16_t ADC_GlobalGetData | ( | LPC_ADC_TypeDef * | ADCx, |
uint8_t | channel | ||
) |
Get ADC Data from AD Global register.
- Parameters:
-
[in] ADCx pointer to ADC [in] channel channel number
- Returns:
- Result of conversion
Definition at line 286 of file lpc17xx_adc.c.
FlagStatus ADC_GlobalGetStatus | ( | LPC_ADC_TypeDef * | ADCx, |
uint32_t | StatusType | ||
) |
Get ADC Chanel status from AD global data register.
- Parameters:
-
[in] ADCx pointer to ADC [in] StatusType 0:Burst status 1:Done status
- Returns:
- SET / RESET
Definition at line 304 of file lpc17xx_adc.c.
void ADC_Init | ( | LPC_ADC_TypeDef * | ADCx, |
uint32_t | ConvFreq | ||
) |
Initial for ADC
- Set bit PCADC
- Set clock for ADC
- Set Clock Frequency.
- Parameters:
-
[in] ADCx pointer to LPC_ADC_TypeDef [in] ConvFreq Clock frequency
- Returns:
- None
Definition at line 57 of file lpc17xx_adc.c.
void ADC_IntConfig | ( | LPC_ADC_TypeDef * | ADCx, |
ADC_TYPE_INT_OPT | IntType, | ||
FunctionalState | NewState | ||
) |
ADC interrupt configuration.
- Parameters:
-
[in] ADCx pointer to ADC [in] IntType [in] NewState,: - SET : enable ADC interrupt
- RESET: disable ADC interrupt
- Returns:
- None
Definition at line 201 of file lpc17xx_adc.c.
void ADC_PowerdownCmd | ( | LPC_ADC_TypeDef * | ADCx, |
FunctionalState | NewState | ||
) |
Set AD conversion in power mode.
- Parameters:
-
[in] ADCx pointer to ADC [in] NewState - 1: AD converter is optional
- 0: AD Converter is in power down mode
- Returns:
- None
Definition at line 160 of file lpc17xx_adc.c.
void ADC_StartCmd | ( | LPC_ADC_TypeDef * | ADCx, |
uint8_t | start_mode | ||
) |
Set start mode for ADC.
- Parameters:
-
[in] ADCx pointer to LPC_ADC_TypeDef [in] start_mode Start mode choose one of modes in 'ADC_START_OPT' enumeration type definition
- Returns:
- None
Definition at line 121 of file lpc17xx_adc.c.
Generated on Tue Jul 12 2022 17:06:02 by
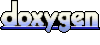