
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_adc.c
00001 /** 00002 * @file : lpc17xx_adc.c 00003 * @brief : Contains all functions support for ADC firmware library on LPC17xx 00004 * @version : 1.0 00005 * @date : 3. April. 2009 00006 * @author : NgaDinh 00007 ************************************************************************** 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 /* Peripheral group ----------------------------------------------------------- */ 00021 /** @addtogroup ADC 00022 * @{ 00023 */ 00024 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_adc.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 /* If this source file built with example, the LPC17xx FW library configuration 00030 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00031 * otherwise the default FW library configuration file must be included instead 00032 */ 00033 #ifdef __BUILD_WITH_EXAMPLE__ 00034 #include "lpc17xx_libcfg.h" 00035 #else 00036 #include "lpc17xx_libcfg_default.h" 00037 #endif /* __BUILD_WITH_EXAMPLE__ */ 00038 00039 00040 #ifdef _ADC 00041 00042 /* Public Functions ----------------------------------------------------------- */ 00043 /** @addtogroup ADC_Public_Functions 00044 * @{ 00045 */ 00046 00047 /*********************************************************************//** 00048 * @brief Initial for ADC 00049 * - Set bit PCADC 00050 * - Set clock for ADC 00051 * - Set Clock Frequency 00052 * 00053 * @param[in] ADCx pointer to LPC_ADC_TypeDef 00054 * @param[in] ConvFreq Clock frequency 00055 * @return None 00056 **********************************************************************/ 00057 void ADC_Init(LPC_ADC_TypeDef *ADCx, uint32_t ConvFreq) 00058 00059 { 00060 uint32_t temp, tmp; 00061 00062 CHECK_PARAM(PARAM_ADCx(ADCx)); 00063 CHECK_PARAM(PARAM_ADC_FREQUENCY(ConvFreq)); 00064 00065 // Turn on power and clock 00066 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCAD, ENABLE); 00067 // Set clock divider for ADC to 4 from CCLK as default 00068 // CLKPWR_SetPCLKDiv(CLKPWR_PCLKSEL_ADC,CLKPWR_PCLKSEL_CCLK_DIV_4); 00069 00070 ADCx->ADCR = 0; 00071 00072 //Enable PDN bit 00073 tmp = ADC_CR_PDN; 00074 // Set clock frequency 00075 temp = CLKPWR_GetPCLK(CLKPWR_PCLKSEL_ADC) ; 00076 temp = (temp /ConvFreq) - 1; 00077 tmp |= ADC_CR_CLKDIV(temp); 00078 00079 ADCx->ADCR = tmp; 00080 } 00081 00082 00083 /*********************************************************************//** 00084 * @brief Close ADC 00085 * @param[in] ADCx pointer to ADC 00086 * @return None 00087 **********************************************************************/ 00088 void ADC_DeInit(LPC_ADC_TypeDef *ADCx) 00089 { 00090 CHECK_PARAM(PARAM_ADCx(ADCx)); 00091 00092 // Clear PDN bit 00093 ADCx->ADCR &= ~ADC_CR_PDN; 00094 // Turn on power and clock 00095 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCAD, DISABLE); 00096 } 00097 00098 00099 /*********************************************************************//** 00100 * @brief Get Result conversion from A/D data register 00101 * @param[in] channel number which want to read back the result 00102 * @return Result of conversion 00103 *********************************************************************/ 00104 uint32_t ADC_GetData(uint32_t channel) 00105 { 00106 uint32_t adc_value; 00107 00108 CHECK_PARAM(PARAM_ADC_CHANNEL_SELECTION(channel)); 00109 00110 adc_value = *(uint32_t *)((&LPC_ADC->ADDR0) + channel); 00111 return ADC_GDR_RESULT(adc_value); 00112 } 00113 00114 /*********************************************************************//** 00115 * @brief Set start mode for ADC 00116 * @param[in] ADCx pointer to LPC_ADC_TypeDef 00117 * @param[in] start_mode Start mode choose one of modes in 00118 * 'ADC_START_OPT' enumeration type definition 00119 * @return None 00120 *********************************************************************/ 00121 void ADC_StartCmd(LPC_ADC_TypeDef *ADCx, uint8_t start_mode) 00122 { 00123 CHECK_PARAM(PARAM_ADCx(ADCx)); 00124 CHECK_PARAM(PARAM_ADC_START_OPT(start_mode)); 00125 00126 ADCx->ADCR &= ~ADC_CR_START_MASK; 00127 ADCx->ADCR |=ADC_CR_START_MODE_SEL((uint32_t)start_mode); 00128 } 00129 00130 00131 /*********************************************************************//** 00132 * @brief ADC Burst mode setting 00133 * 00134 * @param[in] ADCx pointer to ADC 00135 * @param[in] NewState 00136 * - 1: Set Burst mode 00137 * - 0: reset Burst mode 00138 * @return None 00139 **********************************************************************/ 00140 void ADC_BurstCmd(LPC_ADC_TypeDef *ADCx, FunctionalState NewState) 00141 { 00142 CHECK_PARAM(PARAM_ADCx(ADCx)); 00143 00144 ADCx->ADCR &= ~ADC_CR_BURST; 00145 if (NewState){ 00146 ADCx->ADCR |= ADC_CR_BURST; 00147 } 00148 00149 } 00150 00151 /*********************************************************************//** 00152 * @brief Set AD conversion in power mode 00153 * 00154 * @param[in] ADCx pointer to ADC 00155 * @param[in] NewState 00156 * - 1: AD converter is optional 00157 * - 0: AD Converter is in power down mode 00158 * @return None 00159 **********************************************************************/ 00160 void ADC_PowerdownCmd(LPC_ADC_TypeDef *ADCx, FunctionalState NewState) 00161 { 00162 CHECK_PARAM(PARAM_ADCx(ADCx)); 00163 00164 ADCx->ADCR &= ~ADC_CR_PDN; 00165 if (NewState){ 00166 ADCx->ADCR |= ADC_CR_PDN; 00167 } 00168 } 00169 00170 /*********************************************************************//** 00171 * @brief Set Edge start configuration 00172 * 00173 * @param[in] ADCx pointer to ADC 00174 * @param[in] EdgeOption is ADC_START_ON_RISING and ADC_START_ON_FALLING 00175 * 0:ADC_START_ON_RISING 00176 * 1:ADC_START_ON_FALLING 00177 * 00178 * @return None 00179 **********************************************************************/ 00180 void ADC_EdgeStartConfig(LPC_ADC_TypeDef *ADCx, uint8_t EdgeOption) 00181 { 00182 CHECK_PARAM(PARAM_ADCx(ADCx)); 00183 CHECK_PARAM(PARAM_ADC_START_ON_EDGE_OPT(EdgeOption)); 00184 00185 ADCx->ADCR &= ~ADC_CR_EDGE; 00186 if (EdgeOption){ 00187 ADCx->ADCR |= ADC_CR_EDGE; 00188 } 00189 } 00190 00191 /*********************************************************************//** 00192 * @brief ADC interrupt configuration 00193 * @param[in] ADCx pointer to ADC 00194 * @param[in] IntType 00195 * @param[in] NewState: 00196 * - SET : enable ADC interrupt 00197 * - RESET: disable ADC interrupt 00198 * 00199 * @return None 00200 **********************************************************************/ 00201 void ADC_IntConfig (LPC_ADC_TypeDef *ADCx, ADC_TYPE_INT_OPT IntType, FunctionalState NewState) 00202 { 00203 CHECK_PARAM(PARAM_ADCx(ADCx)); 00204 CHECK_PARAM(PARAM_ADC_TYPE_INT_OPT(IntType)); 00205 00206 ADCx->ADINTEN &= ~ADC_INTEN_CH(IntType); 00207 if (NewState){ 00208 ADCx->ADINTEN |= ADC_INTEN_CH(IntType); 00209 } 00210 } 00211 00212 /*********************************************************************//** 00213 * @brief Enable/Disable ADC channel number 00214 * @param[in] ADCx pointer to ADC 00215 * @param[in] Channel channel number 00216 * @param[in] NewState Enable or Disable 00217 * 00218 * @return None 00219 **********************************************************************/ 00220 void ADC_ChannelCmd (LPC_ADC_TypeDef *ADCx, uint8_t Channel, FunctionalState NewState) 00221 { 00222 CHECK_PARAM(PARAM_ADCx(ADCx)); 00223 CHECK_PARAM(PARAM_ADC_CHANNEL_SELECTION(Channel)); 00224 00225 if (NewState == ENABLE) { 00226 ADCx->ADCR |= ADC_CR_CH_SEL(Channel); 00227 } else { 00228 ADCx->ADCR &= ~ADC_CR_CH_SEL(Channel); 00229 } 00230 } 00231 00232 /*********************************************************************//** 00233 * @brief Get ADC result 00234 * @param[in] ADCx pointer to ADC 00235 * @param[in] channel channel number 00236 * @return Data conversion 00237 **********************************************************************/ 00238 uint16_t ADC_ChannelGetData(LPC_ADC_TypeDef *ADCx, uint8_t channel) 00239 { 00240 uint32_t adc_value; 00241 00242 CHECK_PARAM(PARAM_ADCx(ADCx)); 00243 CHECK_PARAM(PARAM_ADC_CHANNEL_SELECTION(channel)); 00244 00245 adc_value = *(uint32_t *) ((&ADCx->ADDR0) + channel); 00246 return ADC_DR_RESULT(adc_value); 00247 } 00248 00249 /*********************************************************************//** 00250 * @brief Get ADC Chanel status from ADC data register 00251 * @param[in] ADCx pointer to ADC 00252 * @param[in] channel channel number 00253 * @param[in] StatusType 00254 * 0:Burst status 00255 * 1:Done status 00256 * @return SET / RESET 00257 **********************************************************************/ 00258 FlagStatus ADC_ChannelGetStatus(LPC_ADC_TypeDef *ADCx, uint8_t channel, uint32_t StatusType) 00259 { 00260 uint32_t temp; 00261 00262 CHECK_PARAM(PARAM_ADCx(ADCx)); 00263 CHECK_PARAM(PARAM_ADC_CHANNEL_SELECTION(channel)); 00264 CHECK_PARAM(PARAM_ADC_DATA_STATUS(StatusType)); 00265 00266 temp = *(uint32_t *) ((&ADCx->ADDR0) + channel); 00267 if (StatusType) { 00268 temp &= ADC_DR_DONE_FLAG; 00269 }else{ 00270 temp &= ADC_DR_OVERRUN_FLAG; 00271 } 00272 if (temp) { 00273 return SET; 00274 } else { 00275 return RESET; 00276 } 00277 00278 } 00279 00280 /*********************************************************************//** 00281 * @brief Get ADC Data from AD Global register 00282 * @param[in] ADCx pointer to ADC 00283 * @param[in] channel channel number 00284 * @return Result of conversion 00285 **********************************************************************/ 00286 uint16_t ADC_GlobalGetData(LPC_ADC_TypeDef *ADCx, uint8_t channel) 00287 { 00288 CHECK_PARAM(PARAM_ADCx(ADCx)); 00289 CHECK_PARAM(PARAM_ADC_CHANNEL_SELECTION(channel)); 00290 00291 //ADCx->ADGDR &= ~ADC_GDR_CH_MASK; 00292 //ADCx->ADGDR |= ADC_GDR_CH(channel); 00293 return (uint16_t)(ADC_GDR_RESULT(ADCx->ADGDR)); 00294 } 00295 00296 /*********************************************************************//** 00297 * @brief Get ADC Chanel status from AD global data register 00298 * @param[in] ADCx pointer to ADC 00299 * @param[in] StatusType 00300 * 0:Burst status 00301 * 1:Done status 00302 * @return SET / RESET 00303 **********************************************************************/ 00304 FlagStatus ADC_GlobalGetStatus(LPC_ADC_TypeDef *ADCx, uint32_t StatusType) 00305 { 00306 uint32_t temp; 00307 00308 CHECK_PARAM(PARAM_ADCx(ADCx)); 00309 CHECK_PARAM(PARAM_ADC_DATA_STATUS(StatusType)); 00310 00311 temp = ADCx->ADGDR; 00312 if (StatusType){ 00313 temp &= ADC_DR_DONE_FLAG; 00314 }else{ 00315 temp &= ADC_DR_OVERRUN_FLAG; 00316 } 00317 if (temp){ 00318 return SET; 00319 }else{ 00320 return RESET; 00321 } 00322 } 00323 00324 /** 00325 * @} 00326 */ 00327 00328 #endif /* _ADC */ 00329 00330 /** 00331 * @} 00332 */ 00333 00334 /* --------------------------------- End Of File ------------------------------ */ 00335
Generated on Tue Jul 12 2022 17:06:02 by
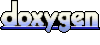