Forked from Aaron Berk's ITG3200 driver class library, customized for my specific application using 9DoF-Stick by Sparkfun.
Fork of ITG3200 by
ITG3200 Class Reference
ITG-3200 triple axis digital gyroscope. More...
#include <ITG3200.h>
Public Types | |
enum | Correction { NoCorrection, OffsetCorrection, Calibration } |
Zero offset correction mode that can be specified when calling getGyroXYZ(). More... | |
Public Member Functions | |
ITG3200 (PinName sda, PinName scl, bool fastmode=false) | |
Constructor. | |
ITG3200 (I2C &i2c) | |
Constructor that accepts external i2c interface object. | |
void | setCalibrationCurve (const float offset[3], const float slope[3]) |
Sets calibration curve parameters. | |
char | getWhoAmI (void) |
Get the identity of the device. | |
void | setWhoAmI (char address) |
Set the address of the device. | |
char | getSampleRateDivider (void) |
Get the sample rate divider. | |
void | setSampleRateDivider (char divider) |
Set the sample rate divider. | |
int | getInternalSampleRate (void) |
Get the internal sample rate. | |
void | setLpBandwidth (char bandwidth) |
Set the low pass filter bandwidth. | |
char | getInterruptConfiguration (void) |
Get the interrupt configuration. | |
void | setInterruptConfiguration (char config) |
Set the interrupt configuration. | |
bool | isPllReady (void) |
Check the ITG_RDY bit of the INT_STATUS register. | |
bool | isRawDataReady (void) |
Check the RAW_DATA_RDY bit of the INT_STATUS register. | |
int | getRawTemperature (void) |
Get the temperature in raw format. | |
float | getTemperature (void) |
Get the temperature of the device. | |
int | getGyroX (void) |
Get the output for the x-axis gyroscope. | |
int | getGyroY (void) |
Get the output for the y-axis gyroscope. | |
int | getGyroZ (void) |
Get the output on the z-axis gyroscope. | |
void | getGyroXYZ (int readings[3], Correction corr=OffsetCorrection) |
Burst read the outputs on the x,y,z-axis gyroscope. | |
void | getGyroXYZDegrees (double readings[3], Correction corr=OffsetCorrection) |
Burst read the outputs on the x,y,z-axis gyroscope and convert them into degrees per second. | |
void | getGyroXYZRadians (double readings[3], Correction corr=OffsetCorrection) |
Burst read the outputs on the x,y,z-axis gyroscope and convert them into degrees per second. | |
char | getPowerManagement (void) |
Get the power management configuration. | |
void | setPowerManagement (char config) |
Set power management configuration. | |
void | calibrate (double time) |
Calibrate the sensor drift by sampling zero offset. | |
I2C & | getI2C () |
Returns the I2C object that this object is using for communication. | |
const int * | getOffset () const |
Returns internal offset values for zero adjusting. | |
Static Public Attributes | |
static const int | I2C_ADDRESS = 0xD0 |
The I2C address that can be passed directly to i2c object (it's already shifted 1 bit left). | |
Protected Member Functions | |
int | getWord (int regi) |
Reads a word (2 bytes) from the sensor via I2C bus. | |
void | getRawGyroXYZ (int readings[3]) |
An internal method to acquire gyro sensor readings before calibration correction. | |
Protected Attributes | |
int | offset [3] |
Offset values that will be subtracted from output. |
Detailed Description
ITG-3200 triple axis digital gyroscope.
Definition at line 87 of file ITG3200.h.
Member Enumeration Documentation
enum Correction |
Zero offset correction mode that can be specified when calling getGyroXYZ().
The device has a major drift in readings depending on ambient temperature. You can measure the temperature with built-in thermometer to correct it, but you must have calibration curves for each axes to do so. Here are the options on how to correct the drift.
- Enumerator:
NoCorrection Do not correct zero offset at all; You would have trouble integrating the values to obtain rotation.
OffsetCorrection Correct the outputs with single-point zero adjust.
Calibration Use calibration curve (actually lines) to correct the outputs. You must provide coefficients with setCalibrationCurve().
Constructor & Destructor Documentation
ITG3200 | ( | PinName | sda, |
PinName | scl, | ||
bool | fastmode = false |
||
) |
Constructor.
Sets FS_SEL to 0x03 for proper opertaion.
- Parameters:
-
sda - mbed pin to use for the SDA I2C line. scl - mbed pin to use for the SCL I2C line. fastmode Sets the internal I2C interface to use 400kHz clock.
Definition at line 39 of file ITG3200.cpp.
ITG3200 | ( | I2C & | i2c ) |
Member Function Documentation
void calibrate | ( | double | time ) |
Calibrate the sensor drift by sampling zero offset.
Be sure to keep the sensor stationary while sampling offset.
Once this function is invoked, following getGyroXYZ*() functions return corrected values.
If the drift value changes over time, you can call this function once in a while to follow it. But don't forget to fix the sensor while calibrating!
- Parameters:
-
time The time span to sample and average offset. Sampling rate is limited, so giving long time to calibrate will improve correction quality.
Definition at line 297 of file ITG3200.cpp.
int getGyroX | ( | void | ) |
void getGyroXYZ | ( | int | readings[3], |
Correction | corr = OffsetCorrection |
||
) |
Burst read the outputs on the x,y,z-axis gyroscope.
Typical sensitivity is 14.375 LSB/(degrees/sec).
- Parameters:
-
readings The output buffer array that has at least 3 length. corr Correction method for returned values.
Definition at line 256 of file ITG3200.cpp.
void getGyroXYZDegrees | ( | double | readings[3], |
Correction | corr = OffsetCorrection |
||
) |
void getGyroXYZRadians | ( | double | readings[3], |
Correction | corr = OffsetCorrection |
||
) |
int getGyroY | ( | void | ) |
int getGyroZ | ( | void | ) |
I2C& getI2C | ( | ) |
int getInternalSampleRate | ( | void | ) |
Get the internal sample rate.
- Returns:
- The internal sample rate in kHz - either 1 or 8.
Definition at line 129 of file ITG3200.cpp.
char getInterruptConfiguration | ( | void | ) |
Get the interrupt configuration.
See datasheet for register contents details.
7 6 5 4 +------+------+--------------+------------------+ | ACTL | OPEN | LATCH_INT_EN | INT_ANYRD_2CLEAR | +------+------+--------------+------------------+
3 2 1 0 +---+------------+------------+---+ | 0 | ITG_RDY_EN | RAW_RDY_EN | 0 | +---+------------+------------+---+
ACTL Logic level for INT output pin; 1 = active low, 0 = active high. OPEN Drive type for INT output pin; 1 = open drain, 0 = push-pull. LATCH_INT_EN Latch mode; 1 = latch until interrupt is cleared, 0 = 50us pulse. INT_ANYRD_2CLEAR Latch clear method; 1 = any register read, 0 = status register read only. ITG_RDY_EN Enable interrupt when device is ready, (PLL ready after changing clock source). RAW_RDY_EN Enable interrupt when data is available. 0 Bits 1 and 3 of the INT_CFG register should be zero.
- Returns:
- the contents of the INT_CFG register.
Definition at line 164 of file ITG3200.cpp.
const int* getOffset | ( | ) | const |
char getPowerManagement | ( | void | ) |
Get the power management configuration.
See the datasheet for register contents details.
7 6 5 4 +---------+-------+---------+---------+ | H_RESET | SLEEP | STBY_XG | STBY_YG | +---------+-------+---------+---------+
3 2 1 0 +---------+----------+----------+----------+ | STBY_ZG | CLK_SEL2 | CLK_SEL1 | CLK_SEL0 | +---------+----------+----------+----------+
H_RESET Reset device and internal registers to the power-up-default settings. SLEEP Enable low power sleep mode. STBY_XG Put gyro X in standby mode (1=standby, 0=normal). STBY_YG Put gyro Y in standby mode (1=standby, 0=normal). STBY_ZG Put gyro Z in standby mode (1=standby, 0=normal). CLK_SEL Select device clock source:
CLK_SEL | Clock Source --------+-------------- 0 Internal oscillator 1 PLL with X Gyro reference 2 PLL with Y Gyro reference 3 PLL with Z Gyro reference 4 PLL with external 32.768kHz reference 5 PLL with external 19.2MHz reference 6 Reserved 7 Reserved
- Returns:
- The contents of the PWR_MGM register.
Definition at line 274 of file ITG3200.cpp.
void getRawGyroXYZ | ( | int | readings[3] ) | [protected] |
An internal method to acquire gyro sensor readings before calibration correction.
Protected for the time being, although there could be cases that raw values are appreciated by the user.
Definition at line 243 of file ITG3200.cpp.
int getRawTemperature | ( | void | ) |
char getSampleRateDivider | ( | void | ) |
Get the sample rate divider.
- Returns:
- The sample rate divider as a number from 0-255.
Definition at line 106 of file ITG3200.cpp.
float getTemperature | ( | void | ) |
Get the temperature of the device.
- Returns:
- The temperature in degrees celsius.
Definition at line 237 of file ITG3200.cpp.
char getWhoAmI | ( | void | ) |
Get the identity of the device.
- Returns:
- The contents of the Who Am I register which contains the I2C address of the device.
Definition at line 82 of file ITG3200.cpp.
int getWord | ( | int | regi ) | [protected] |
Reads a word (2 bytes) from the sensor via I2C bus.
The queried value is assumed big-endian, 2's complement value.
This protected function is added because we shouldn't write getGyroX(), getGyroY() and getGyroZ() independently, but collect common codes.
- Parameters:
-
regi Register address to be read.
Definition at line 225 of file ITG3200.cpp.
bool isPllReady | ( | void | ) |
Check the ITG_RDY bit of the INT_STATUS register.
- Returns:
- True if the ITG_RDY bit is set, corresponding to PLL ready, false if the ITG_RDY bit is not set, corresponding to PLL not ready.
Definition at line 187 of file ITG3200.cpp.
bool isRawDataReady | ( | void | ) |
Check the RAW_DATA_RDY bit of the INT_STATUS register.
- Returns:
- True if the RAW_DATA_RDY bit is set, corresponding to new data in the sensor registers, false if the RAW_DATA_RDY bit is not set, corresponding to no new data yet in the sensor registers.
Definition at line 206 of file ITG3200.cpp.
void setCalibrationCurve | ( | const float | offset[3], |
const float | slope[3] | ||
) |
Sets calibration curve parameters.
- Parameters:
-
offset An array holding calibration curve offsets (0th-order coefficient) for each axis, must have 3 elements. slope An array holding calibration curve slopes (1st-order coefficient) for each axis, must have 3 elements.
Definition at line 71 of file ITG3200.cpp.
void setInterruptConfiguration | ( | char | config ) |
Set the interrupt configuration.
See datasheet for configuration byte details.
7 6 5 4 +------+------+--------------+------------------+ | ACTL | OPEN | LATCH_INT_EN | INT_ANYRD_2CLEAR | +------+------+--------------+------------------+
3 2 1 0 +---+------------+------------+---+ | 0 | ITG_RDY_EN | RAW_RDY_EN | 0 | +---+------------+------------+---+
ACTL Logic level for INT output pin; 1 = active low, 0 = active high. OPEN Drive type for INT output pin; 1 = open drain, 0 = push-pull. LATCH_INT_EN Latch mode; 1 = latch until interrupt is cleared, 0 = 50us pulse. INT_ANYRD_2CLEAR Latch clear method; 1 = any register read, 0 = status register read only. ITG_RDY_EN Enable interrupt when device is ready, (PLL ready after changing clock source). RAW_RDY_EN Enable interrupt when data is available. 0 Bits 1 and 3 of the INT_CFG register should be zero.
- Parameters:
-
config Configuration byte to write to INT_CFG register.
Definition at line 177 of file ITG3200.cpp.
void setLpBandwidth | ( | char | bandwidth ) |
Set the low pass filter bandwidth.
Also used to set the internal sample rate. Pass the define bandwidth codes as a parameter.
256Hz -> 8kHz internal sample rate. Everything else -> 1kHz internal rate.
- Parameters:
-
bandwidth Low pass filter bandwidth code
Definition at line 153 of file ITG3200.cpp.
void setPowerManagement | ( | char | config ) |
Set power management configuration.
See the datasheet for configuration byte details
7 6 5 4 +---------+-------+---------+---------+ | H_RESET | SLEEP | STBY_XG | STBY_YG | +---------+-------+---------+---------+
3 2 1 0 +---------+----------+----------+----------+ | STBY_ZG | CLK_SEL2 | CLK_SEL1 | CLK_SEL0 | +---------+----------+----------+----------+
H_RESET Reset device and internal registers to the power-up-default settings. SLEEP Enable low power sleep mode. STBY_XG Put gyro X in standby mode (1=standby, 0=normal). STBY_YG Put gyro Y in standby mode (1=standby, 0=normal). STBY_ZG Put gyro Z in standby mode (1=standby, 0=normal). CLK_SEL Select device clock source:
CLK_SEL | Clock Source --------+-------------- 0 Internal oscillator 1 PLL with X Gyro reference 2 PLL with Y Gyro reference 3 PLL with Z Gyro reference 4 PLL with external 32.768kHz reference 5 PLL with external 19.2MHz reference 6 Reserved 7 Reserved
- Parameters:
-
config The configuration byte to write to the PWR_MGM register.
Definition at line 287 of file ITG3200.cpp.
void setSampleRateDivider | ( | char | divider ) |
Set the sample rate divider.
Fsample = Finternal / (divider + 1), where Finternal = 1kHz or 8kHz, as decidied by the DLPF_FS register.
- Parameters:
-
The sample rate divider as a number from 0-255.
Definition at line 119 of file ITG3200.cpp.
void setWhoAmI | ( | char | address ) |
Set the address of the device.
- Parameters:
-
address The I2C slave address to write to the Who Am I register on the device.
Definition at line 96 of file ITG3200.cpp.
Field Documentation
const int I2C_ADDRESS = 0xD0 [static] |
Generated on Wed Jul 20 2022 11:54:17 by
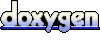