
CLASS for software SPI master class instantiated on any general IO pin. Slow but flexible.
SPI_flex Class Reference
A software Master SPI (Serial Peripheral Interface) class that can be instantiated on any mbed LPC1768 general IO pins (p5...p30). More...
#include <SPI_flex.h>
Public Member Functions | |
SPI_flex (PinName mosi, PinName miso, PinName sclk, PinName cs) | |
Create a software Master SPI bus instance. | |
void | format (unsigned int bits=16, int mode=0) |
Configure the SPI bus data transmission format. | |
void | csactive (bool cs=0) |
Set active state of the SPI bus chip select pin. | |
void | frequency (int Hz=400000) |
Set the SPI bus clock frequency. | |
bool | writebit (bool bit) |
Send a single bit on the SPI bus MOSI pin and read response on MISO pin. | |
uint8_t | writebyte (uint8_t byte) |
Send a byte on the SPI bus MOSI pin and read response on MISO pin. | |
uint16_t | writeword (uint16_t word) |
Send a word on the SPI bus MOSI pin and read response on MISO pin. |
Detailed Description
A software Master SPI (Serial Peripheral Interface) class that can be instantiated on any mbed LPC1768 general IO pins (p5...p30).
Primarily optimized for driving daisy-chained serial display hardware, it provides flexibility beyond on-board SPI hardware capabilities and some automation at the expense of speed. Currently runs full clock speed at about 395kHz. Comment-out code lines testing/setting the automatic chip select for higher speed performance if the chip select feature is not required.
- flexible bit-lengths from 0 .. 4,294,967,295. Not possible with mbed hardware.
- automation of the chip select line which enables all external chips to be loaded with information before latching data in.
- selectable clock polarity and edge trigger (Mode 0 through Mode 3).
- selectable chip select polarity (active-low or active-high).
- latching data in while clock is still active on last bit, **such as required by the Maxim MAX6952. This is not possible with the mbed API, onboard SPI or SSP hardware.
- allows one-shot loading of all daisy-chained hardware registers before latching. Prevents display ghosting effect.
The MAX6952 chip requires the following sequence:
[[http://datasheets.maxim-ic.com/en/ds/MAX6952.pdf]]
- Take CLK low.
- Take CS low. This enables the internal 16-bit shift register.
- Clock 16 bits of data into DIN, D15 first to D0 last, observing the setup and hold times. Bit D15 is low, indicating a write command.
- Take CS high **(while CLK is still high after clocking in the last data bit).
- Take CLK low.
Example:
// Send a byte to a SPI slave, and record the response // Chip select is automatic #include "mbed.h" #include "SPI_flex.h" SPI device(p5, p6, p7, p8); // mosi, miso, sclk, cs int main() { device.frequency(300000); //to slow clock speed if necessary int response = device.writebyte(0xFF); }
Definition at line 65 of file SPI_flex.h.
Constructor & Destructor Documentation
SPI_flex | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs | ||
) |
Create a software Master SPI bus instance.
- Parameters:
-
mosi Master Out Slave In pin miso Master In Slave Out pin sclk Serial Clock out pin cs Chip Select out pin
Definition at line 18 of file SPI_flex.cpp.
Member Function Documentation
void csactive | ( | bool | cs = 0 ) |
Set active state of the SPI bus chip select pin.
Constructor calls by default. Call only if change needed.
- Parameters:
-
cs 0 (default) for active-low, 1 for active-high
Definition at line 35 of file SPI_flex.cpp.
void format | ( | unsigned int | bits = 16 , |
int | mode = 0 |
||
) |
Configure the SPI bus data transmission format.
Constructor calls by default. Call only if change needed.
Automatically controls chip select output pin. Default is 16 bit.
- Parameters:
-
bits Bits per SPI hardware string frame (0 .. 4,294,967,295) mode Clock polarity and phase. Default is Mode 0.
Mode | POL | PHA -----|-----|---- 0 | 0 | 0 1 | 0 | 1 2 | 1 | 0 3 | 1 | 1
Definition at line 27 of file SPI_flex.cpp.
void frequency | ( | int | Hz = 400000 ) |
Set the SPI bus clock frequency.
Is maximum by default. Call only if change needed.
Result will not be exact and may jump in 5Khz increments due to integer rounding.
- Parameters:
-
Hz Hertz 395,000 is approximate maximum (and default speed)
Definition at line 39 of file SPI_flex.cpp.
bool writebit | ( | bool | bit ) |
Send a single bit on the SPI bus MOSI pin and read response on MISO pin.
- Parameters:
-
bit Boolean one or zero, TRUE or FALSE
- Returns:
- Boolean one or zero read from MISO from external hardware
Definition at line 52 of file SPI_flex.cpp.
uint8_t writebyte | ( | uint8_t | byte ) |
Send a byte on the SPI bus MOSI pin and read response on MISO pin.
MSB first out, LSB last out (7:0)
- Parameters:
-
byte An 8-bit value
- Returns:
- 8-bit value read from MISO from external hardware
Definition at line 69 of file SPI_flex.cpp.
uint16_t writeword | ( | uint16_t | word ) |
Send a word on the SPI bus MOSI pin and read response on MISO pin.
MSB first out, LSB last out (15:0)
- Parameters:
-
word A 16-bit value
- Returns:
- 16-bit value read from MISO from external hardware
Definition at line 77 of file SPI_flex.cpp.
Generated on Wed Jul 13 2022 23:44:52 by
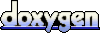