
CLASS for software SPI master class instantiated on any general IO pin. Slow but flexible.
Embed:
(wiki syntax)
Show/hide line numbers
SPI_flex.h
00001 /* Class SPI_flex, Copyright 2014, David T. Mort (http://mbed.org/users/dtmort/) 00002 00003 Licensed under the Apache License, Version 2.0 (the "License"); 00004 you may not use this file except in compliance with the License. 00005 You may obtain a copy of the License at 00006 00007 http://www.apache.org/licenses/LICENSE-2.0 00008 00009 Unless required by applicable law or agreed to in writing, software 00010 distributed under the License is distributed on an "AS IS" BASIS, 00011 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 See the License for the specific language governing permissions and 00013 limitations under the License. 00014 */ 00015 #ifndef MBED_SPI_FLEX_H 00016 #define MBED_SPI_FLEX_H 00017 00018 #include "mbed.h" 00019 00020 /**A software Master SPI (Serial Peripheral Interface) class 00021 * that can be instantiated on any mbed LPC1768 general IO pins (p5...p30). 00022 * 00023 * Primarily optimized for driving daisy-chained serial display hardware, 00024 * it provides flexibility beyond on-board SPI hardware capabilities and some automation 00025 * at the expense of speed. Currently runs full clock speed at about 395kHz. 00026 * Comment-out code lines testing/setting the automatic chip select for higher speed 00027 * performance if the chip select feature is not required. 00028 * - flexible bit-lengths from 0 .. 4,294,967,295. Not possible with mbed hardware. 00029 * - automation of the chip select line which enables 00030 * all external chips to be loaded with information before latching data in. 00031 * - selectable clock polarity and edge trigger (Mode 0 through Mode 3). 00032 * - selectable chip select polarity (active-low or active-high). 00033 * - latching data in while clock is still active on last bit, 00034 * **such as required by the Maxim MAX6952. This is not possible with the mbed API, 00035 * onboard SPI or SSP hardware. 00036 * - allows one-shot loading of all daisy-chained hardware registers before latching. 00037 * Prevents display ghosting effect. 00038 * 00039 * The MAX6952 chip requires the following sequence: 00040 * 00041 * [[http://datasheets.maxim-ic.com/en/ds/MAX6952.pdf]] 00042 * -# Take CLK low. 00043 * -# Take CS low. This enables the internal 16-bit shift register. 00044 * -# Clock 16 bits of data into DIN, D15 first to D0 last, observing the setup and hold times. Bit D15 is low, indicating a write command. 00045 * -# Take CS high **(while CLK is still high after clocking in the last data bit). 00046 * -# Take CLK low. 00047 * 00048 * Example: 00049 * @code 00050 * // Send a byte to a SPI slave, and record the response 00051 * 00052 * // Chip select is automatic 00053 * 00054 * #include "mbed.h" 00055 * #include "SPI_flex.h" 00056 * 00057 * SPI device(p5, p6, p7, p8); // mosi, miso, sclk, cs 00058 * 00059 * int main() { 00060 * device.frequency(300000); //to slow clock speed if necessary 00061 * int response = device.writebyte(0xFF); 00062 * } 00063 * @endcode 00064 */ 00065 class SPI_flex { 00066 00067 public: 00068 /**Create a software Master SPI bus instance 00069 * 00070 * @param mosi Master Out Slave In pin 00071 * @param miso Master In Slave Out pin 00072 * @param sclk Serial Clock out pin 00073 * @param cs Chip Select out pin 00074 */ 00075 SPI_flex(PinName mosi, PinName miso, PinName sclk, PinName cs); 00076 00077 /** Configure the SPI bus data transmission format 00078 * 00079 * Constructor calls by default. Call only if change needed. 00080 * 00081 * Automatically controls chip select output pin. Default is 16 bit. 00082 * 00083 * @param bits Bits per SPI hardware string frame (0 .. 4,294,967,295) 00084 * 00085 * @param mode Clock polarity and phase. Default is Mode 0. 00086 * 00087 @code 00088 Mode | POL | PHA 00089 -----|-----|---- 00090 0 | 0 | 0 00091 1 | 0 | 1 00092 2 | 1 | 0 00093 3 | 1 | 1 00094 @endcode 00095 */ 00096 void format(unsigned int bits=16, int mode=0); 00097 00098 /** Set active state of the SPI bus chip select pin 00099 * 00100 * Constructor calls by default. Call only if change needed. 00101 * 00102 * @param cs 0 (default) for active-low, 1 for active-high 00103 */ 00104 void csactive(bool cs=0); 00105 00106 /** Set the SPI bus clock frequency. 00107 * Is maximum by default. Call only if change needed. 00108 * 00109 * Result will not be exact and may jump in 5Khz increments 00110 * due to integer rounding. 00111 * 00112 * @param Hz Hertz 395,000 is approximate maximum (and default speed) 00113 */ 00114 void frequency(int Hz=400000); 00115 00116 00117 /** Send a single bit on the SPI bus MOSI pin and read response on MISO pin 00118 * 00119 * @param bit Boolean one or zero, TRUE or FALSE 00120 * @returns Boolean one or zero read from MISO from external hardware 00121 */ 00122 bool writebit(bool bit); 00123 00124 /** Send a byte on the SPI bus MOSI pin and read response on MISO pin 00125 * 00126 * MSB first out, LSB last out (7:0) 00127 * 00128 * @param byte An 8-bit value 00129 * @returns 8-bit value read from MISO from external hardware 00130 */ 00131 uint8_t writebyte(uint8_t byte); 00132 00133 /** Send a word on the SPI bus MOSI pin and read response on MISO pin 00134 * 00135 * MSB first out, LSB last out (15:0) 00136 * 00137 * @param word A 16-bit value 00138 * @returns 16-bit value read from MISO from external hardware 00139 */ 00140 uint16_t writeword(uint16_t word); 00141 00142 00143 00144 //protected: 00145 00146 private: 00147 00148 void select(void); //activates chip select sequence, called by writebit() function 00149 void deSelect(void); //deactivates chip select, called by writebit() function 00150 DigitalOut _mosi; //master out slave in, pin 00151 DigitalIn _miso; //master in slave out, pin 00152 DigitalOut _sclk; //serial clock, pin 00153 DigitalOut _cs; //chip select, pin 00154 bool __cs; //chip select active state, operating setup 00155 bool _cpol; //clock polarity, operating setup 00156 bool _cpha; //clock phase, operating setup 00157 int _mode; //mode 0,1,2 or 3, operating setup 00158 unsigned int _deAssert; //counts-up bits sent for chip select, placeholder 00159 unsigned int BitString; //# serialized bits sent to hardware string, controls chip select activation 00160 unsigned int BitStream; //# bits to hardware from source data, not used yet 00161 bool bit_read; //bit value read from miso pin 00162 uint8_t byte_read; //byte value read from miso pin 00163 uint16_t word_read; //word value read from miso pin 00164 int Dcpwi; //Delay clock pulse width inactive, for freqency adjustments 00165 int Dcpwa; //Delay clock pulse width active, for freqency adjustments 00166 00167 }; 00168 #endif
Generated on Wed Jul 13 2022 23:44:52 by
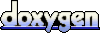