SCA3000 triple axis digital interface accelerometer
Embed:
(wiki syntax)
Show/hide line numbers
SCA3000.h
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 * 00024 * @section DESCRIPTION 00025 * 00026 * SCA3000, triple axis, digital interface, accelerometer. 00027 * 00028 * Datasheet: 00029 * 00030 * http://www.sparkfun.com/datasheets/Sensors/Accelerometer/SCA3000-D01.pdf 00031 */ 00032 00033 #ifndef MBED_SCA3000_H 00034 #define MBED_SCA3000_H 00035 00036 /** 00037 * Includes 00038 */ 00039 #include "mbed.h" 00040 00041 /** 00042 * Defines 00043 */ 00044 //Registers. 00045 #define SCA3000_REVID_REG 0x00 00046 #define SCA3000_STATUS_REG 0x02 00047 #define SCA3000_X_LSB 0x04 00048 #define SCA3000_X_MSB 0x05 00049 #define SCA3000_Y_LSB 0x06 00050 #define SCA3000_Y_MSB 0x07 00051 #define SCA3000_Z_LSB 0x08 00052 #define SCA3000_Z_MSB 0x09 00053 00054 #define SCA3000_SPI_READ 0x00 00055 #define SCA3000_SPI_WRITE 0x02 00056 00057 #define SCA3000_X_AXIS 0x00 00058 #define SCA3000_Y_AXIS 0x01 00059 #define SCA3000_Z_AXIS 0x02 00060 00061 /** 00062 * SCA3000, triple axis, digital interface, accelerometer. 00063 */ 00064 class SCA3000 { 00065 00066 public: 00067 00068 /** 00069 * Constructor. 00070 * 00071 * @param mosi mbed pin to use for MOSI line of SPI interface. 00072 * @param miso mbed pin to use for MISO line of SPI interface. 00073 * @param sck mbed pin to use for SCK line of SPI interface. 00074 * @param cs mbed pin to use for not chip select line of SPI interface. 00075 * @param nr mbed pin to use for the not reset line. 00076 */ 00077 SCA3000(PinName mosi, PinName miso, PinName sck, PinName cs, PinName nr); 00078 00079 /** 00080 * Read the revision ID register on the device. 00081 * 00082 * @return The revision ID number. 00083 */ 00084 int getRevId(void); 00085 00086 /** 00087 * Get the register contents acceleration value for the 00088 * given axis, using the nominal sensitivity values 00089 * found in the datasheet. 00090 * 00091 * @param axis The axis to get acceleration values for. 00092 * 00093 * @return The acceleration on the specified axis in mg. 00094 */ 00095 float getAcceleration(int axis); 00096 00097 /** 00098 * Get the register contents acceleration value for the 00099 * given axis, in counts. 00100 * 00101 * @param axis The axis to get the counts values for. 00102 * 00103 * @return The acceleration on the specified axis in counts. 00104 */ 00105 int getCounts(int axis); 00106 00107 private: 00108 00109 SPI spi_; 00110 DigitalOut nCS_; 00111 DigitalOut nR_; 00112 00113 /** 00114 * Read one byte from a register on the device. 00115 * 00116 * @param address Address of the register to read. 00117 * 00118 * @return The contents of the register address. 00119 */ 00120 int oneByteRead(int address); 00121 00122 /** 00123 * Write one byte to a register on the device. 00124 * 00125 * @param address Address of the register to write to. 00126 * @param data The data to write into the register. 00127 */ 00128 void oneByteWrite(int address, char data); 00129 00130 }; 00131 00132 #endif /* MBED_SCA3000_H */
Generated on Tue Jul 12 2022 23:35:55 by
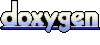