A library to control MX28 servos. It could also be used with the rest of the MX series servos.
Fork of MX28 by
Utilities Class Reference
MX28 utility functions class. More...
#include <Utilities.h>
Static Public Member Functions | |
static uint8_t | GetCheckSum (const uint8_t *data, uint8_t length) |
Get the checksum for a packet. | |
static void | ConvertUInt16ToUInt8Array (uint16_t value, uint8_t *data) |
Convert a UInt16 number to array of chars. | |
static void | ConvertInt16ToUInt8Array (int16_t value, uint8_t *data) |
Convert an Int16 number to array of chars. | |
static void | ConvertUInt32ToUInt8Array (uint32_t value, uint8_t *data) |
Convert a UInt32 number to array of chars. | |
static void | ConvertInt32ToUInt8Array (int32_t value, uint8_t *data) |
Convert an Int32 number to array of chars. | |
static uint16_t | ConvertUInt8ArrayToUInt16 (uint8_t *data) |
Convert an array of char to UInt16. | |
static int16_t | ConvertUInt8ArrayToInt16 (uint8_t *data) |
Convert an array of char to Int16. | |
static int32_t | ConvertUInt8ArrayToInt32 (uint8_t *data) |
Convert an array of char to Int32. |
Detailed Description
MX28 utility functions class.
Definition at line 26 of file Utilities.h.
Member Function Documentation
void ConvertInt16ToUInt8Array | ( | int16_t | value, |
uint8_t * | data | ||
) | [static] |
Convert an Int16 number to array of chars.
- Parameters:
-
value The number value. data The array to store the conversion.
Definition at line 39 of file Utilities.cpp.
void ConvertInt32ToUInt8Array | ( | int32_t | value, |
uint8_t * | data | ||
) | [static] |
Convert an Int32 number to array of chars.
- Parameters:
-
value The number value. data The array to store the conversion.
Definition at line 53 of file Utilities.cpp.
void ConvertUInt16ToUInt8Array | ( | uint16_t | value, |
uint8_t * | data | ||
) | [static] |
Convert a UInt16 number to array of chars.
- Parameters:
-
value The number value. data The array to store the conversion.
Definition at line 33 of file Utilities.cpp.
void ConvertUInt32ToUInt8Array | ( | uint32_t | value, |
uint8_t * | data | ||
) | [static] |
Convert a UInt32 number to array of chars.
- Parameters:
-
value The number value. data The array to store the conversion.
Definition at line 45 of file Utilities.cpp.
int16_t ConvertUInt8ArrayToInt16 | ( | uint8_t * | data ) | [static] |
Convert an array of char to Int16.
- Parameters:
-
data The array containing the chars.
- Returns:
- Int16 converted number.
Definition at line 66 of file Utilities.cpp.
int32_t ConvertUInt8ArrayToInt32 | ( | uint8_t * | data ) | [static] |
Convert an array of char to Int32.
- Parameters:
-
data The array containing the chars.
- Returns:
- Int32 number.
Definition at line 71 of file Utilities.cpp.
uint16_t ConvertUInt8ArrayToUInt16 | ( | uint8_t * | data ) | [static] |
Convert an array of char to UInt16.
- Parameters:
-
data The array containing the chars.
- Returns:
- UInt16 converted number.
Definition at line 61 of file Utilities.cpp.
uint8_t GetCheckSum | ( | const uint8_t * | data, |
uint8_t | length | ||
) | [static] |
Get the checksum for a packet.
- Parameters:
-
data The array of bytes. length The size of the array.
- Returns:
- checksum
Definition at line 21 of file Utilities.cpp.
Generated on Sun Jul 17 2022 08:53:17 by
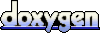