A library to control MX28 servos. It could also be used with the rest of the MX series servos.
Fork of MX28 by
Utilities.cpp
00001 /* Copyright (c) 2012 Georgios Petrou, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "Utilities.h" 00020 00021 uint8_t Utilities::GetCheckSum(const uint8_t *data, uint8_t length) 00022 { 00023 uint8_t checkSum = 0; 00024 00025 for(int i = 0; i < length; i++) 00026 checkSum += data[i]; 00027 00028 checkSum = ~checkSum; 00029 00030 return checkSum; 00031 } 00032 00033 void Utilities::ConvertUInt16ToUInt8Array(uint16_t value, uint8_t* data) 00034 { 00035 data[0] = value; 00036 data[1] = value >> 8; 00037 } 00038 00039 void Utilities::ConvertInt16ToUInt8Array(int16_t value, uint8_t* data) 00040 { 00041 data[0] = value; 00042 data[1] = value >> 8; 00043 } 00044 00045 void Utilities::ConvertUInt32ToUInt8Array(uint32_t value, uint8_t* data) 00046 { 00047 data[0] = value; 00048 data[1] = value >> 8; 00049 data[2] = value >> 16; 00050 data[3] = value >> 24; 00051 } 00052 00053 void Utilities::ConvertInt32ToUInt8Array(int32_t value, uint8_t* data) 00054 { 00055 data[0] = value; 00056 data[1] = value >> 8; 00057 data[2] = value >> 16; 00058 data[3] = value >> 24; 00059 } 00060 00061 uint16_t Utilities::ConvertUInt8ArrayToUInt16(uint8_t* data) 00062 { 00063 return (((uint16_t)data[1]) << 8) | ((uint16_t)data[0]); 00064 } 00065 00066 int16_t Utilities::ConvertUInt8ArrayToInt16(uint8_t* data) 00067 { 00068 return (((int16_t)data[1]) << 8) | ((int16_t)data[0]); 00069 } 00070 00071 int32_t Utilities::ConvertUInt8ArrayToInt32(uint8_t* data) 00072 { 00073 return (((int32_t)data[3]) << 24) | (((int32_t)data[2]) << 16) | (((int32_t)data[1]) << 8) | ((int32_t)data[0]); 00074 } 00075
Generated on Sun Jul 17 2022 08:53:17 by
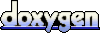