Library to wrapper most of the functions on the MPL3115A2 pressure and temperature sensor.
Dependents: WeatherBalloon4180 WeatherBalloon4180 mbed_rifletool Smart_Watch_4180_Final_Design ... more
MPL3115A2 Class Reference
MPL3115A2 I2C Barometric Pressure and Tempurature Sensor Library This class wraps most of the function in the MPL3115A2 sensor leaving out the FIFO and interrupt system. More...
#include <MPL3115A2.h>
Public Member Functions | |
MPL3115A2 (I2C *i2c, Serial *pc=NULL) | |
Constructs an MPL3115A2 object and associates an I2C and optional Serial debug object. | |
void | init () |
Initializes the sensor, defaulting to Altitude mode. | |
char | whoAmI () |
Queries the value from the WHO_AM_I register (usually equal to 0xC4). | |
Altitude * | readAltitude (Altitude *a) |
Reads Altitude data from the sensor and returns it in the Altitude object passed in. | |
Pressure * | readPressure (Pressure *p) |
Reads Pressure data from the sensor and returns it in the Pressure object passed in. | |
Temperature * | readTemperature (Temperature *t) |
Reads Temperature data from the sensor and returns it in the Temperature object passed in. | |
char | offsetAltitude () |
Returns the altitude offset stored in the sensor. | |
void | setOffsetAltitude (const char offset) |
Sets the altitude offset stored in the sensor. The allowed offset range is from -128 to 127 meters. | |
char | offsetPressure () |
Returns the pressure offset stored in the sensor. | |
void | setOffsetPressure (const char offset) |
Sets the pressure offset stored in the sensor. The allowed offset range is from -128 to 127 where each LSB represents 4 Pa. | |
char | offsetTemperature () |
Returns the temperature offset stored in the sensor. | |
void | setOffsetTemperature (const char offset) |
Sets the temperature offset stored in the sensor. The allowed offset range is from -128 to 127 where each LSB represents 0.0625ºC. | |
void | setModeStandby () |
Puts the sensor into Standby mode. Required when using methods below. | |
void | setModeActive () |
Activates the sensor to start taking measurements. | |
void | setModeBarometer () |
Puts the sensor into barometric mode, be sure to put the sensor in standby mode first. | |
void | setModeAltimeter () |
Puts the sensor into altimeter mode, be sure to put the sensor in standby mode first. | |
void | setOversampleRate (char rate) |
Sets the number of samples from 1 to 128, be sure to put the sensor in standby mode first. | |
void | enableEventFlags () |
Sets all the event flags, be sure to put the sensor in standby mode first. |
Detailed Description
MPL3115A2 I2C Barometric Pressure and Tempurature Sensor Library This class wraps most of the function in the MPL3115A2 sensor leaving out the FIFO and interrupt system.
Definition at line 104 of file MPL3115A2.h.
Constructor & Destructor Documentation
MPL3115A2 | ( | I2C * | i2c, |
Serial * | pc = NULL |
||
) |
Constructs an MPL3115A2 object and associates an I2C and optional Serial debug object.
- Parameters:
-
*i2c The I2C object to use for the sensor. *pc An optional serial debug connection object.
Definition at line 6 of file MPL3115A2.cpp.
Member Function Documentation
void enableEventFlags | ( | ) |
Sets all the event flags, be sure to put the sensor in standby mode first.
Definition at line 159 of file MPL3115A2.cpp.
void init | ( | ) |
Initializes the sensor, defaulting to Altitude mode.
This should be called before using the sensor for the first time.
Definition at line 13 of file MPL3115A2.cpp.
char offsetAltitude | ( | ) |
Returns the altitude offset stored in the sensor.
Definition at line 144 of file MPL3115A2.h.
char offsetPressure | ( | ) |
Returns the pressure offset stored in the sensor.
Definition at line 148 of file MPL3115A2.h.
char offsetTemperature | ( | ) |
Returns the temperature offset stored in the sensor.
Definition at line 152 of file MPL3115A2.h.
Reads Altitude data from the sensor and returns it in the Altitude object passed in.
If no data could be read, the Altitude object is left as is.
- Parameters:
-
a A pointer to an Altitude object that will receive the sensor data.
- Returns:
- The Altitude pointer that was passed in.
Definition at line 47 of file MPL3115A2.cpp.
Reads Pressure data from the sensor and returns it in the Pressure object passed in.
If no data could be read, the Pressure object is left as is.
- Parameters:
-
a A pointer to a Pressure object that will receive the sensor data.
- Returns:
- The Pressure pointer that was passed in.
Definition at line 80 of file MPL3115A2.cpp.
Temperature * readTemperature | ( | Temperature * | t ) |
Reads Temperature data from the sensor and returns it in the Temperature object passed in.
If no data could be read, the Temperature object is left as is.
- Parameters:
-
a A pointer to an Temperature object that will receive the sensor data.
- Returns:
- The Temperature pointer that was passed in.
Definition at line 104 of file MPL3115A2.cpp.
void setModeActive | ( | ) |
Activates the sensor to start taking measurements.
Definition at line 141 of file MPL3115A2.cpp.
void setModeAltimeter | ( | ) |
Puts the sensor into altimeter mode, be sure to put the sensor in standby mode first.
Definition at line 127 of file MPL3115A2.cpp.
void setModeBarometer | ( | ) |
Puts the sensor into barometric mode, be sure to put the sensor in standby mode first.
Definition at line 132 of file MPL3115A2.cpp.
void setModeStandby | ( | ) |
Puts the sensor into Standby mode. Required when using methods below.
Definition at line 137 of file MPL3115A2.cpp.
void setOffsetAltitude | ( | const char | offset ) |
Sets the altitude offset stored in the sensor. The allowed offset range is from -128 to 127 meters.
Definition at line 146 of file MPL3115A2.h.
void setOffsetPressure | ( | const char | offset ) |
Sets the pressure offset stored in the sensor. The allowed offset range is from -128 to 127 where each LSB represents 4 Pa.
Definition at line 150 of file MPL3115A2.h.
void setOffsetTemperature | ( | const char | offset ) |
Sets the temperature offset stored in the sensor. The allowed offset range is from -128 to 127 where each LSB represents 0.0625ºC.
Definition at line 154 of file MPL3115A2.h.
void setOversampleRate | ( | char | rate ) |
Sets the number of samples from 1 to 128, be sure to put the sensor in standby mode first.
Definition at line 146 of file MPL3115A2.cpp.
char whoAmI | ( | ) |
Queries the value from the WHO_AM_I register (usually equal to 0xC4).
- Returns:
- The fixed device ID from the sensor.
Definition at line 118 of file MPL3115A2.h.
Generated on Tue Jul 12 2022 19:49:10 by
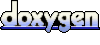