
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
tuple.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __TUPLE_H__ 00010 #define __TUPLE_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief Tuple Object Type 00016 * 00017 * Tuple object type header. 00018 */ 00019 00020 /** 00021 * Tuple obj 00022 * 00023 * Immutable ordered sequence. Contains array of ptrs to objs. 00024 */ 00025 typedef struct PmTuple_s 00026 { 00027 /** Object descriptor */ 00028 PmObjDesc_t od; 00029 00030 /** 00031 * Length of tuple 00032 * I don't expect a tuple to ever exceed 255 elements, 00033 * but if I set this type to int8_t, a 0-element tuple 00034 * is too small to be allocated. 00035 */ 00036 uint16_t length; 00037 00038 /** Array of ptrs to objs */ 00039 pPmObj_t val[1]; 00040 } PmTuple_t, 00041 *pPmTuple_t; 00042 00043 00044 #define tuple_copy(src, dest) tuple_replicate((src), 1, (dest)) 00045 00046 00047 /** 00048 * Creates a Tuple by loading a tuple image from memory. 00049 * 00050 * Obtain space for tuple from the heap. 00051 * Load all objs within the tuple img. 00052 * Leave contents of paddr pointing one byte past end of 00053 * last obj in tuple. 00054 * 00055 * The tuple image has the following structure: 00056 * -type: S8 - OBJ_TYPE_TUPLE 00057 * -length U8 - N number of objects in the tuple. 00058 * N objects follow in the stream. 00059 * 00060 * @param memspace Memory space. 00061 * @param paddr Ptr to ptr to tuple in memspace 00062 * @param r_ptuple Return by reference; new filled tuple 00063 * @return Return status 00064 */ 00065 PmReturn_t tuple_loadFromImg(PmMemSpace_t memspace, 00066 uint8_t const **paddr, pPmObj_t *r_ptuple); 00067 00068 /** 00069 * Allocates space for a new Tuple. Returns a pointer to the tuple. 00070 * 00071 * @param n the number of elements the tuple will contain 00072 * @param r_ptuple Return by ref, ptr to new tuple 00073 * @return Return status 00074 */ 00075 PmReturn_t tuple_new(uint16_t n, pPmObj_t *r_ptuple); 00076 00077 /** 00078 * Replicates a tuple, n number of times to create a new tuple 00079 * 00080 * Copies the pointers (not the objects). 00081 * 00082 * @param ptup Ptr to source tuple. 00083 * @param n Number of times to replicate the tuple. 00084 * @param r_ptuple Return arg; Ptr to new tuple. 00085 * @return Return status 00086 */ 00087 PmReturn_t tuple_replicate(pPmObj_t ptup, int16_t n, pPmObj_t *r_ptuple); 00088 00089 /** 00090 * Gets the object in the tuple at the index. 00091 * 00092 * @param ptup Ptr to tuple obj 00093 * @param index Index into tuple 00094 * @param r_pobj Return by reference; ptr to item 00095 * @return Return status 00096 */ 00097 PmReturn_t tuple_getItem(pPmObj_t ptup, int16_t index, pPmObj_t *r_pobj); 00098 00099 #ifdef HAVE_PRINT 00100 /** 00101 * Prints out a tuple. Uses obj_print() to print elements. 00102 * 00103 * @param pobj Object to print. 00104 * @return Return status 00105 */ 00106 PmReturn_t tuple_print(pPmObj_t pobj); 00107 #endif /* HAVE_PRINT */ 00108 00109 #endif /* __TUPLE_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
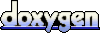