
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
strobj.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __STRING_H__ 00010 #define __STRING_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief String Object Type 00016 * 00017 * String object type header. 00018 */ 00019 00020 00021 /** Set to nonzero to enable string cache. DO NOT REMOVE THE DEFINITION. */ 00022 #define USE_STRING_CACHE 1 00023 00024 00025 /** 00026 * Loads a string from image 00027 * 00028 * @param ms memoryspace paddr points to 00029 * @param paddr address in memoryspace of source string 00030 * @param r_pstring Return by reference; a new string object 00031 * @return Return status 00032 */ 00033 #define string_loadFromImg(ms, paddr, r_pstring) \ 00034 string_create((ms), (paddr), (int16_t)-1, (int16_t)1, (r_pstring)) 00035 00036 /** 00037 * Creates String object from character array in RAM 00038 * 00039 * @param paddr pointer to address of source string 00040 * @param r_pstring Return arg; addr of ptr to string 00041 */ 00042 #define string_new(paddr, r_pstring) \ 00043 string_create(MEMSPACE_RAM, (uint8_t const **)(paddr), 0, (int16_t)1, (r_pstring)) 00044 00045 /** 00046 * Creates String object from character array in RAM which may contain 00047 * embedded null characters. 00048 * 00049 * @param paddr pointer to address of source string 00050 * @param len length of source string 00051 * @param r_pstring Return arg; addr of ptr to string 00052 */ 00053 #define string_newWithLen(paddr, len, r_pstring) \ 00054 string_create(MEMSPACE_RAM, (uint8_t const **)(paddr), (len), (int16_t)1, \ 00055 (r_pstring)) 00056 00057 /** 00058 * Creates String object by replicating an existing C string, n times 00059 * 00060 * @param paddr pointer to address of source string 00061 * @param n number of times to replicate the source string 00062 * @param r_pstring Return arg; addr of ptr to string 00063 */ 00064 #define string_replicate(paddr, n, r_pstring) \ 00065 string_create(MEMSPACE_RAM, (paddr), (uint8_t)0, (n), (r_pstring)) 00066 00067 /*************************************************************** 00068 * Types 00069 **************************************************************/ 00070 00071 /** 00072 * String obj 00073 * 00074 * Null terminated array of chars. 00075 */ 00076 typedef struct PmString_s 00077 { 00078 /** Object descriptor */ 00079 PmObjDesc_t od; 00080 00081 /** Length of string */ 00082 uint16_t length; 00083 00084 #if USE_STRING_CACHE 00085 /** Ptr to next string in cache */ 00086 struct PmString_s *next; 00087 #endif /* USE_STRING_CACHE */ 00088 00089 /** 00090 * Null-term char array 00091 * 00092 * Use length 1 here so that string-alloc function can use 00093 * "sizeof(PmString_t) + len" and there will be room for the null-term 00094 */ 00095 uint8_t val[1]; 00096 } PmString_t, 00097 *pPmString_t; 00098 00099 00100 /*************************************************************** 00101 * Prototypes 00102 **************************************************************/ 00103 00104 /** 00105 * Creates a new String obj. 00106 * If len is less than zero, load from a String image. 00107 * If len is zero, copy from a C string (which has a null terminator) 00108 * If len is positive, copy as many chars as given in the len argument 00109 * A string image has the following structure: 00110 * -type: int8 - OBJ_TYPE_STRING 00111 * -length: uint16 - number of bytes in the string 00112 * -val: uint8[] - array of chars with null term 00113 * 00114 * Returns by reference a ptr to String obj. 00115 * 00116 * Obtain space for String from the heap. 00117 * Copy string from memspace. 00118 * Leave contents of paddr pointing one byte past end of str. 00119 * 00120 * THE PROGRAMMER SHOULD NOT CALL THIS FUNCTION DIRECTLY. 00121 * Instead, use one of the two macros string_loadFromImg() 00122 * or string_new(). 00123 * 00124 * @param memspace memory space where *paddr points 00125 * @param paddr ptr to ptr to null term character array or image. 00126 * @param len length of the C character array 00127 * (use -1 for string images, 0 for C strings) 00128 * @param n Number of times to replicate the given string argument 00129 * @param r_pstring Return by reference; ptr to String obj 00130 * @return Return status 00131 */ 00132 PmReturn_t string_create(PmMemSpace_t memspace, uint8_t const **paddr, 00133 int16_t len, int16_t n, pPmObj_t *r_pstring); 00134 00135 /** 00136 * Creates a new String object from a single character. 00137 * 00138 * @param c The character to become the string 00139 * @param r_pstring Return by reference; ptr to String obj 00140 * @return Return status 00141 */ 00142 PmReturn_t string_newFromChar(uint8_t const c, pPmObj_t *r_pstring); 00143 00144 /** 00145 * Compares two String objects for equality. 00146 * 00147 * @param pstr1 Ptr to first string 00148 * @param pstr2 Ptr to second string 00149 * @return C_SAME if the strings are equivalent, C_DIFFER otherwise 00150 */ 00151 int8_t string_compare(pPmString_t pstr1, pPmString_t pstr2); 00152 00153 #ifdef HAVE_PRINT 00154 /** 00155 * Sends out a string object bytewise. Escaping and framing is configurable 00156 * via is_escaped. 00157 * 00158 * @param pstr Ptr to string object 00159 * @param is_escaped If 0, print out string as is. Otherwise escape unprintable 00160 * characters and surround string with single quotes. 00161 * @return Return status 00162 */ 00163 PmReturn_t string_print(pPmObj_t pstr, uint8_t is_escaped); 00164 #endif /* HAVE_PRINT */ 00165 00166 /** 00167 * Clears the string cache if one exists. 00168 * Called by heap_init() 00169 * 00170 * @return Return status 00171 */ 00172 PmReturn_t string_cacheInit(void); 00173 00174 00175 /** Returns a pointer to the base of the string cache */ 00176 PmReturn_t string_getCache(pPmString_t **r_ppstrcache); 00177 00178 /** 00179 * Returns a new string object that is the concatenation 00180 * of the two given strings. 00181 * 00182 * @param pstr1 First source string 00183 * @param pstr2 Second source string 00184 * @param r_pstring Return arg; ptr to new string object 00185 * @return Return status 00186 */ 00187 PmReturn_t 00188 string_concat(pPmString_t pstr1, pPmString_t pstr2, pPmObj_t *r_pstring); 00189 00190 /** 00191 * Returns a new string object that is created from the given format string 00192 * and the argument(s). 00193 * 00194 * @param pstr Format string object 00195 * @param parg Single argument or tuple of arguments 00196 * @param r_pstring Return arg; ptr to new string object 00197 * @return Return status 00198 */ 00199 PmReturn_t string_format(pPmString_t pstr, pPmObj_t parg, pPmObj_t *r_pstring); 00200 00201 #ifdef HAVE_PRINT 00202 /** 00203 * Prints n bytes, formatting them if is_escaped is true 00204 * 00205 * @param pb Pointer to C bytes 00206 * @param is_escaped Boolean true if string is to be escaped 00207 * @param n Number of bytes to print 00208 * @return Return status 00209 */ 00210 PmReturn_t string_printFormattedBytes(uint8_t *pb, 00211 uint8_t is_escaped, 00212 uint16_t n); 00213 #endif /* HAVE_PRINT */ 00214 00215 #endif /* __STRING_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
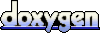