
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
main_nat.cpp
Go to the documentation of this file.
00001 #undef __FILE_ID__ 00002 #define __FILE_ID__ 0x0A 00003 /** 00004 * PyMite usr native function file 00005 * 00006 * automatically created by pmImgCreator.py 00007 * on Fri Mar 15 14:38:03 2013 00008 * 00009 * DO NOT EDIT THIS FILE. 00010 * ANY CHANGES WILL BE LOST. 00011 * 00012 * @file main_nat.cpp 00013 */ 00014 00015 #define __IN_LIBNATIVE_C__ 00016 #include "pm.h" 00017 00018 /* From: mbed.py */ 00019 #include "mbed.h" 00020 #include "NativeClassInterface.h" 00021 #if defined(TARGET_KL25Z) 00022 #include "TSISensor.h" 00023 #endif 00024 00025 #if DEVICE_ANALOGOUT==0 00026 class AnalogOut { 00027 public: 00028 AnalogOut(PinName pin){} 00029 void write(float value){} 00030 void write_u16(unsigned short value){} 00031 float read(){ return 0.0; } 00032 }; 00033 #endif 00034 00035 PmReturn_t 00036 nat_placeholder_func(pPmFrame_t *ppframe) 00037 { 00038 00039 /* 00040 * Use placeholder because an index 00041 * value of zero denotes the stdlib. 00042 * This function should not be called. 00043 */ 00044 PmReturn_t retval; 00045 PM_RAISE(retval, PM_RET_EX_SYS); 00046 return retval; 00047 00048 } 00049 00050 PmReturn_t 00051 nat_01_mbed___init__(pPmFrame_t *ppframe) 00052 { 00053 00054 NativeClassInterface nci; 00055 return nci.init<DigitalOut,PinName>(OBJ_TYPE_STR); 00056 00057 } 00058 00059 PmReturn_t 00060 nat_02_mbed_write(pPmFrame_t *ppframe) 00061 { 00062 00063 NativeClassInterface nci; 00064 return nci.method<DigitalOut,int,&DigitalOut::write>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00065 00066 } 00067 00068 PmReturn_t 00069 nat_03_mbed_read(pPmFrame_t *ppframe) 00070 { 00071 00072 NativeClassInterface nci; 00073 return nci.method<int,DigitalOut,&DigitalOut::read>(OBJ_TYPE_INT); 00074 00075 } 00076 00077 PmReturn_t 00078 nat_04_mbed___init__(pPmFrame_t *ppframe) 00079 { 00080 00081 NativeClassInterface nci; 00082 return nci.init<DigitalIn,PinName>(OBJ_TYPE_STR); 00083 00084 } 00085 00086 PmReturn_t 00087 nat_05_mbed_read(pPmFrame_t *ppframe) 00088 { 00089 00090 NativeClassInterface nci; 00091 return nci.method<int,DigitalIn,&DigitalIn::read>(OBJ_TYPE_INT); 00092 00093 } 00094 00095 PmReturn_t 00096 nat_06_mbed___init__(pPmFrame_t *ppframe) 00097 { 00098 00099 NativeClassInterface nci; 00100 return nci.init<DigitalInOut,PinName>(OBJ_TYPE_STR); 00101 00102 } 00103 00104 PmReturn_t 00105 nat_07_mbed_read(pPmFrame_t *ppframe) 00106 { 00107 00108 NativeClassInterface nci; 00109 return nci.method<int,DigitalInOut,&DigitalInOut::read>(OBJ_TYPE_INT); 00110 00111 } 00112 00113 PmReturn_t 00114 nat_08_mbed_write(pPmFrame_t *ppframe) 00115 { 00116 00117 NativeClassInterface nci; 00118 return nci.method<DigitalInOut,int,&DigitalInOut::write>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00119 00120 } 00121 00122 PmReturn_t 00123 nat_09_mbed_input(pPmFrame_t *ppframe) 00124 { 00125 00126 NativeClassInterface nci; 00127 return nci.method<DigitalInOut,&DigitalInOut::input>(OBJ_TYPE_NON); 00128 00129 } 00130 00131 PmReturn_t 00132 nat_10_mbed_output(pPmFrame_t *ppframe) 00133 { 00134 00135 NativeClassInterface nci; 00136 return nci.method<DigitalInOut,&DigitalInOut::output>(OBJ_TYPE_NON); 00137 00138 } 00139 00140 PmReturn_t 00141 nat_11_mbed___init__(pPmFrame_t *ppframe) 00142 { 00143 00144 NativeClassInterface nci; 00145 return nci.init<AnalogIn,PinName>(OBJ_TYPE_STR); 00146 00147 } 00148 00149 PmReturn_t 00150 nat_12_mbed_read_u16(pPmFrame_t *ppframe) 00151 { 00152 00153 NativeClassInterface nci; 00154 return nci.method<uint16_t,AnalogIn,&AnalogIn::read_u16>(OBJ_TYPE_INT); 00155 00156 } 00157 00158 PmReturn_t 00159 nat_13_mbed_read(pPmFrame_t *ppframe) 00160 { 00161 00162 NativeClassInterface nci; 00163 return nci.method<float,AnalogIn,&AnalogIn::read>(OBJ_TYPE_FLT); 00164 00165 } 00166 00167 PmReturn_t 00168 nat_14_mbed___init__(pPmFrame_t *ppframe) 00169 { 00170 00171 NativeClassInterface nci; 00172 return nci.init<AnalogOut,PinName>(OBJ_TYPE_STR); 00173 00174 } 00175 00176 PmReturn_t 00177 nat_15_mbed_write_u16(pPmFrame_t *ppframe) 00178 { 00179 00180 NativeClassInterface nci; 00181 return nci.method<AnalogOut,uint16_t,&AnalogOut::write_u16>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00182 00183 } 00184 00185 PmReturn_t 00186 nat_16_mbed_write(pPmFrame_t *ppframe) 00187 { 00188 00189 NativeClassInterface nci; 00190 return nci.method<AnalogOut,float,&AnalogOut::write>(OBJ_TYPE_NON, OBJ_TYPE_FLT); 00191 00192 } 00193 00194 PmReturn_t 00195 nat_17_mbed_read(pPmFrame_t *ppframe) 00196 { 00197 00198 NativeClassInterface nci; 00199 return nci.method<float,AnalogOut,&AnalogOut::read>(OBJ_TYPE_FLT); 00200 00201 } 00202 00203 PmReturn_t 00204 nat_18_mbed___init__(pPmFrame_t *ppframe) 00205 { 00206 00207 NativeClassInterface nci; 00208 return nci.init<PwmOut,PinName>(OBJ_TYPE_STR); 00209 00210 } 00211 00212 PmReturn_t 00213 nat_19_mbed_write(pPmFrame_t *ppframe) 00214 { 00215 00216 NativeClassInterface nci; 00217 return nci.method<PwmOut,float,&PwmOut::write>(OBJ_TYPE_NON, OBJ_TYPE_FLT); 00218 00219 } 00220 00221 PmReturn_t 00222 nat_20_mbed_read(pPmFrame_t *ppframe) 00223 { 00224 00225 NativeClassInterface nci; 00226 return nci.method<float,PwmOut,&PwmOut::read>(OBJ_TYPE_FLT); 00227 00228 } 00229 00230 PmReturn_t 00231 nat_21_mbed_period(pPmFrame_t *ppframe) 00232 { 00233 00234 NativeClassInterface nci; 00235 return nci.method<PwmOut,float,&PwmOut::period>(OBJ_TYPE_NON, OBJ_TYPE_FLT); 00236 00237 } 00238 00239 PmReturn_t 00240 nat_22_mbed_period_ms(pPmFrame_t *ppframe) 00241 { 00242 00243 NativeClassInterface nci; 00244 return nci.method<PwmOut,int,&PwmOut::period_ms>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00245 00246 } 00247 00248 PmReturn_t 00249 nat_23_mbed_period_us(pPmFrame_t *ppframe) 00250 { 00251 00252 NativeClassInterface nci; 00253 return nci.method<PwmOut,int,&PwmOut::period_us>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00254 00255 } 00256 00257 PmReturn_t 00258 nat_24_mbed_pulsewidth(pPmFrame_t *ppframe) 00259 { 00260 00261 NativeClassInterface nci; 00262 return nci.method<PwmOut,float,&PwmOut::pulsewidth>(OBJ_TYPE_NON, OBJ_TYPE_FLT); 00263 00264 } 00265 00266 PmReturn_t 00267 nat_25_mbed_pulsewidth_ms(pPmFrame_t *ppframe) 00268 { 00269 00270 NativeClassInterface nci; 00271 return nci.method<PwmOut,int,&PwmOut::pulsewidth_ms>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00272 00273 } 00274 00275 PmReturn_t 00276 nat_26_mbed_pulsewidth_us(pPmFrame_t *ppframe) 00277 { 00278 00279 NativeClassInterface nci; 00280 return nci.method<PwmOut,int,&PwmOut::pulsewidth_us>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00281 00282 } 00283 00284 PmReturn_t 00285 nat_27_mbed___init__(pPmFrame_t *ppframe) 00286 { 00287 00288 NativeClassInterface nci; 00289 return nci.init<Serial,PinName,PinName>(OBJ_TYPE_STR, OBJ_TYPE_STR); 00290 00291 } 00292 00293 PmReturn_t 00294 nat_28_mbed_baud(pPmFrame_t *ppframe) 00295 { 00296 00297 NativeClassInterface nci; 00298 Serial* obj; 00299 nci._load_obj<Serial>(&obj); 00300 obj->baud(nci.argv<int>(1)); 00301 return nci.set_return_value<int>(OBJ_TYPE_INT, 0); 00302 //return nci.method<Serial,int,&Serial::baud>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00303 00304 } 00305 00306 PmReturn_t 00307 nat_29_mbed_readable(pPmFrame_t *ppframe) 00308 { 00309 00310 NativeClassInterface nci; 00311 Serial* obj; 00312 nci._load_obj<Serial>(&obj); 00313 int r = obj->readable(); 00314 return nci.set_return_value<int>(OBJ_TYPE_INT, r); 00315 //return nci.method<int,Serial,&Serial::readable>(OBJ_TYPE_INT); 00316 00317 } 00318 00319 PmReturn_t 00320 nat_30_mbed_writeable(pPmFrame_t *ppframe) 00321 { 00322 00323 NativeClassInterface nci; 00324 Serial* obj; 00325 nci._load_obj<Serial>(&obj); 00326 int r = obj->writeable(); 00327 return nci.set_return_value<int>(OBJ_TYPE_INT, r); 00328 //return nci.method<int,Serial,&Serial::writeable>(OBJ_TYPE_INT); 00329 00330 } 00331 00332 PmReturn_t 00333 nat_31_mbed_putc(pPmFrame_t *ppframe) 00334 { 00335 00336 NativeClassInterface nci; 00337 Serial* obj; 00338 nci._load_obj<Serial>(&obj); 00339 int r = obj->putc(nci.argv<int>(1)); 00340 return nci.set_return_value<int>(OBJ_TYPE_INT, r); 00341 //return nci.method<int,Serial,int,&Serial::putc>(OBJ_TYPE_INT, OBJ_TYPE_STR); 00342 00343 } 00344 00345 PmReturn_t 00346 nat_32_mbed_puts(pPmFrame_t *ppframe) 00347 { 00348 00349 NativeClassInterface nci; 00350 Serial* obj; 00351 nci._load_obj<Serial>(&obj); 00352 int r = obj->puts(nci.argv<const char*>(1)); 00353 return nci.set_return_value<int>(OBJ_TYPE_INT, r); 00354 //return nci.method<int,Serial,char*,&Serial::puts>(OBJ_TYPE_INT, OBJ_TYPE_STR); 00355 00356 } 00357 00358 PmReturn_t 00359 nat_33_mbed_getc(pPmFrame_t *ppframe) 00360 { 00361 00362 NativeClassInterface nci; 00363 Serial* obj; 00364 nci._load_obj<Serial>(&obj); 00365 int r = obj->getc(); 00366 return nci.set_return_value<int>(OBJ_TYPE_STR, r); 00367 //return nci.method<int,Serial,&Serial::getc>(OBJ_TYPE_STR); 00368 00369 } 00370 00371 PmReturn_t 00372 nat_34_mbed___init__(pPmFrame_t *ppframe) 00373 { 00374 00375 NativeClassInterface nci; 00376 return nci.init<SPI,PinName,PinName,PinName>(OBJ_TYPE_STR, OBJ_TYPE_STR, OBJ_TYPE_STR); 00377 00378 } 00379 00380 PmReturn_t 00381 nat_35_mbed_format(pPmFrame_t *ppframe) 00382 { 00383 00384 NativeClassInterface nci; 00385 return nci.method<SPI,int,int,&SPI::format>(OBJ_TYPE_NON, OBJ_TYPE_INT, OBJ_TYPE_INT); 00386 00387 } 00388 00389 PmReturn_t 00390 nat_36_mbed_frequency(pPmFrame_t *ppframe) 00391 { 00392 00393 NativeClassInterface nci; 00394 return nci.method<SPI,int,&SPI::frequency>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00395 00396 } 00397 00398 PmReturn_t 00399 nat_37_mbed_write(pPmFrame_t *ppframe) 00400 { 00401 00402 NativeClassInterface nci; 00403 return nci.method<int,SPI,int,&SPI::write>(OBJ_TYPE_INT, OBJ_TYPE_INT); 00404 00405 } 00406 00407 PmReturn_t 00408 nat_38_mbed___init__(pPmFrame_t *ppframe) 00409 { 00410 00411 NativeClassInterface nci; 00412 return nci.init<I2C,PinName,PinName>(OBJ_TYPE_STR, OBJ_TYPE_STR); 00413 00414 } 00415 00416 PmReturn_t 00417 nat_39_mbed_frequency(pPmFrame_t *ppframe) 00418 { 00419 00420 NativeClassInterface nci; 00421 return nci.method<I2C,int,&I2C::frequency>(OBJ_TYPE_NON, OBJ_TYPE_INT); 00422 00423 } 00424 00425 PmReturn_t 00426 nat_40_mbed_read(pPmFrame_t *ppframe) 00427 { 00428 00429 NativeClassInterface nci; 00430 return nci.method<int,I2C,int,char*,int,bool,&I2C::read>(OBJ_TYPE_INT, 00431 OBJ_TYPE_INT, OBJ_TYPE_STR, OBJ_TYPE_INT, OBJ_TYPE_INT); 00432 00433 } 00434 00435 PmReturn_t 00436 nat_41_mbed_write(pPmFrame_t *ppframe) 00437 { 00438 00439 NativeClassInterface nci; 00440 return nci.method<int,I2C,int,const char*,int,bool,&I2C::write>(OBJ_TYPE_INT, 00441 OBJ_TYPE_INT, OBJ_TYPE_STR, OBJ_TYPE_INT, OBJ_TYPE_INT); 00442 00443 } 00444 00445 PmReturn_t 00446 nat_42_mbed___init__(pPmFrame_t *ppframe) 00447 { 00448 00449 NativeClassInterface nci; 00450 return nci.init<Timer>(); 00451 00452 } 00453 00454 PmReturn_t 00455 nat_43_mbed_start(pPmFrame_t *ppframe) 00456 { 00457 00458 NativeClassInterface nci; 00459 return nci.method<Timer,&Timer::start>(OBJ_TYPE_NON); 00460 00461 } 00462 00463 PmReturn_t 00464 nat_44_mbed_stop(pPmFrame_t *ppframe) 00465 { 00466 00467 NativeClassInterface nci; 00468 return nci.method<Timer,&Timer::stop>(OBJ_TYPE_NON); 00469 00470 } 00471 00472 PmReturn_t 00473 nat_45_mbed_reset(pPmFrame_t *ppframe) 00474 { 00475 00476 NativeClassInterface nci; 00477 return nci.method<Timer,&Timer::reset>(OBJ_TYPE_NON); 00478 00479 } 00480 00481 PmReturn_t 00482 nat_46_mbed_read(pPmFrame_t *ppframe) 00483 { 00484 00485 NativeClassInterface nci; 00486 return nci.method<float,Timer,&Timer::read>(OBJ_TYPE_FLT); 00487 00488 } 00489 00490 PmReturn_t 00491 nat_47_mbed_read_ms(pPmFrame_t *ppframe) 00492 { 00493 00494 NativeClassInterface nci; 00495 return nci.method<int,Timer,&Timer::read_ms>(OBJ_TYPE_INT); 00496 00497 } 00498 00499 PmReturn_t 00500 nat_48_mbed_read_us(pPmFrame_t *ppframe) 00501 { 00502 00503 NativeClassInterface nci; 00504 return nci.method<int,Timer,&Timer::read_us>(OBJ_TYPE_INT); 00505 00506 } 00507 00508 PmReturn_t 00509 nat_49_mbed___init__(pPmFrame_t *ppframe) 00510 { 00511 00512 #if defined(TARGET_KL25Z) 00513 NativeClassInterface nci; 00514 return nci.init<TSISensor>(); 00515 #endif 00516 00517 } 00518 00519 PmReturn_t 00520 nat_50_mbed_readPercentage(pPmFrame_t *ppframe) 00521 { 00522 00523 #if defined(TARGET_KL25Z) 00524 NativeClassInterface nci; 00525 return nci.method<float,TSISensor,&TSISensor::readPercentage>(OBJ_TYPE_FLT); 00526 #endif 00527 00528 } 00529 00530 PmReturn_t 00531 nat_51_mbed_readDistance(pPmFrame_t *ppframe) 00532 { 00533 00534 #if defined(TARGET_KL25Z) 00535 NativeClassInterface nci; 00536 return nci.method<uint8_t,TSISensor,&TSISensor::readDistance>(OBJ_TYPE_INT); 00537 #endif 00538 00539 } 00540 00541 /* Native function lookup table */ 00542 pPmNativeFxn_t const usr_nat_fxn_table[] = 00543 { 00544 nat_placeholder_func, 00545 nat_01_mbed___init__, 00546 nat_02_mbed_write, 00547 nat_03_mbed_read, 00548 nat_04_mbed___init__, 00549 nat_05_mbed_read, 00550 nat_06_mbed___init__, 00551 nat_07_mbed_read, 00552 nat_08_mbed_write, 00553 nat_09_mbed_input, 00554 nat_10_mbed_output, 00555 nat_11_mbed___init__, 00556 nat_12_mbed_read_u16, 00557 nat_13_mbed_read, 00558 nat_14_mbed___init__, 00559 nat_15_mbed_write_u16, 00560 nat_16_mbed_write, 00561 nat_17_mbed_read, 00562 nat_18_mbed___init__, 00563 nat_19_mbed_write, 00564 nat_20_mbed_read, 00565 nat_21_mbed_period, 00566 nat_22_mbed_period_ms, 00567 nat_23_mbed_period_us, 00568 nat_24_mbed_pulsewidth, 00569 nat_25_mbed_pulsewidth_ms, 00570 nat_26_mbed_pulsewidth_us, 00571 nat_27_mbed___init__, 00572 nat_28_mbed_baud, 00573 nat_29_mbed_readable, 00574 nat_30_mbed_writeable, 00575 nat_31_mbed_putc, 00576 nat_32_mbed_puts, 00577 nat_33_mbed_getc, 00578 nat_34_mbed___init__, 00579 nat_35_mbed_format, 00580 nat_36_mbed_frequency, 00581 nat_37_mbed_write, 00582 nat_38_mbed___init__, 00583 nat_39_mbed_frequency, 00584 nat_40_mbed_read, 00585 nat_41_mbed_write, 00586 nat_42_mbed___init__, 00587 nat_43_mbed_start, 00588 nat_44_mbed_stop, 00589 nat_45_mbed_reset, 00590 nat_46_mbed_read, 00591 nat_47_mbed_read_ms, 00592 nat_48_mbed_read_us, 00593 nat_49_mbed___init__, 00594 nat_50_mbed_readPercentage, 00595 nat_51_mbed_readDistance, 00596 };
Generated on Tue Jul 12 2022 23:13:47 by
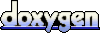