
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
int.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __INT_H__ 00010 #define __INT_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief Integer Object Type 00016 * 00017 * Integer object type header. 00018 */ 00019 00020 /** 00021 * Integer obj 00022 * 00023 * 32b signed integer 00024 */ 00025 typedef struct PmInt_s 00026 { 00027 /** Object descriptor */ 00028 PmObjDesc_t od; 00029 00030 /** Integer value */ 00031 int32_t val; 00032 } PmInt_t, 00033 *pPmInt_t; 00034 00035 00036 /** 00037 * Creates a duplicate Integer object 00038 * 00039 * Created specifically for the index value in FOR_LOOP. 00040 * 00041 * @param pint Pointer to int obj to duplicate. 00042 * @param r_pint Return by ref, ptr to new int 00043 * @return Return status 00044 */ 00045 PmReturn_t int_dup(pPmObj_t pint, pPmObj_t *r_pint); 00046 00047 /** 00048 * Creates a new Integer object 00049 * 00050 * @param val Value to assign int (signed 32-bit). 00051 * @param r_pint Return by ref, ptr to new int 00052 * @return Return status 00053 */ 00054 PmReturn_t int_new(int32_t val, pPmObj_t *r_pint); 00055 00056 /** 00057 * Implements the UNARY_POSITIVE bcode. 00058 * 00059 * Creates a new int with the same value as the given int. 00060 * 00061 * @param pobj Pointer to integer object 00062 * @param r_pint Return by reference, ptr to int 00063 * @return Return status 00064 */ 00065 PmReturn_t int_positive(pPmObj_t pobj, pPmObj_t *r_pint); 00066 00067 /** 00068 * Implements the UNARY_NEGATIVE bcode. 00069 * 00070 * Creates a new int with a value that is the negative of the given int. 00071 * 00072 * @param pobj Pointer to target object 00073 * @param r_pint Return by ref, ptr to int 00074 * @return Return status 00075 */ 00076 PmReturn_t int_negative(pPmObj_t pobj, pPmObj_t *r_pint); 00077 00078 /** 00079 * Implements the UNARY_INVERT bcode. 00080 * 00081 * Creates a new int with a value that is 00082 * the bitwise inversion of the given int. 00083 * 00084 * @param pobj Pointer to integer to invert 00085 * @param r_pint Return by reference; new integer 00086 * @return Return status 00087 */ 00088 PmReturn_t int_bitInvert(pPmObj_t pobj, pPmObj_t *r_pint); 00089 00090 #ifdef HAVE_PRINT 00091 /** 00092 * Sends out an integer object in decimal notation with MSB first. 00093 * The number is preceded with a "-" when necessary. 00094 * 00095 * @param pObj Ptr to int object 00096 * @return Return status 00097 */ 00098 PmReturn_t int_print(pPmObj_t pint); 00099 00100 /** 00101 * Prints the Int object in ascii-coded hexadecimal out the platform output 00102 * 00103 * @param pint Pointer to Int object 00104 */ 00105 PmReturn_t int_printHex(pPmObj_t pint); 00106 #endif /* HAVE_PRINT */ 00107 00108 /** 00109 * Returns by reference an integer that is x raised to the power of y. 00110 * 00111 * @param px The integer base 00112 * @param py The integer exponent 00113 * @param r_pn Return by reference; New integer with value of x ** y 00114 * @return Return status 00115 */ 00116 PmReturn_t int_pow(pPmObj_t px, pPmObj_t py, pPmObj_t *r_pn); 00117 00118 /** 00119 * Returns by reference the result of the selected operation. 00120 * 00121 * @param px The integer numerator 00122 * @param py The integer denominator 00123 * @param op The operator selector. '/' selects division, all else is modulus. 00124 * @param r_pn Return by reference; New integer with value of x / y or x % y. 00125 * @return Return status 00126 */ 00127 PmReturn_t int_divmod(pPmObj_t px, pPmObj_t py, uint8_t op, pPmObj_t *r_pxopy); 00128 00129 #endif /* __INT_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
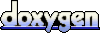