
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
img.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __IMG_H__ 00010 #define __IMG_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief Image header 00016 * 00017 * Created to eliminate a circular include 00018 * among mem, string and obj. 00019 */ 00020 00021 00022 /** The maximum number of paths available in PmImgPaths */ 00023 #define PM_NUM_IMG_PATHS 4 00024 00025 00026 typedef struct PmImgPaths_s 00027 { 00028 PmMemSpace_t memspace[PM_NUM_IMG_PATHS]; 00029 uint8_t const *pimg[PM_NUM_IMG_PATHS]; 00030 uint8_t pathcount; 00031 } 00032 PmImgPaths_t, *pPmImgPaths_t; 00033 00034 00035 /** 00036 * Code image object 00037 * 00038 * A type to hold code images in the heap. 00039 * A code image with an object descriptor at the front. 00040 * Used for storing image objects during ipm; 00041 * the code object keeps a reference to this object. 00042 */ 00043 typedef struct PmCodeImgObj_s 00044 { 00045 /** Object descriptor */ 00046 PmObjDesc_t od; 00047 00048 /** Null-term? char array */ 00049 uint8_t val[1]; 00050 } PmCodeImgObj_t, 00051 *pPmCodeImgObj_t; 00052 00053 00054 /** 00055 * Iterates over all paths in the paths array until the named module is found. 00056 * Returns the memspace,address of the head of the module. 00057 * 00058 * @param pname Pointer to the name of the desired module 00059 * @param r_memspace Return by reference the memory space of the module 00060 * @param r_imgaddr Return by reference the address of the module's image 00061 * @return Return status 00062 */ 00063 PmReturn_t img_findInPaths(pPmObj_t pname, PmMemSpace_t *r_memspace, 00064 uint8_t const **r_imgaddr); 00065 00066 /** 00067 * Appends the given memspace and address to the image path array 00068 * 00069 * @param memspace The memspace 00070 * @param paddr The address 00071 * @return Return status 00072 */ 00073 PmReturn_t img_appendToPath(PmMemSpace_t memspace, uint8_t const * const paddr); 00074 00075 #endif /* __IMG_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
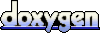