
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
frame.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __FRAME_H__ 00010 #define __FRAME_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief VM Frame 00016 * 00017 * VM frame header. 00018 */ 00019 00020 00021 /** 00022 * The maximum number of local variables a native function can have. 00023 * This defines the length of the locals array in the native frame struct. 00024 */ 00025 #define NATIVE_MAX_NUM_LOCALS 8 00026 00027 00028 /** 00029 * Block Type 00030 * 00031 * Numerical values to put in the 'b_type' field of the tPmBlockType struct. 00032 */ 00033 typedef enum PmBlockType_e 00034 { 00035 /** Invalid block type */ 00036 B_INVALID = 0, 00037 00038 /** Loop type */ 00039 B_LOOP, 00040 00041 /** Try type */ 00042 B_TRY 00043 } PmBlockType_t, *pPmBlockType_t; 00044 00045 00046 /** 00047 * Block 00048 * 00049 * Extra info for loops and trys (others?) 00050 * Frames use linked list of blocks to handle 00051 * nested loops and try-catch blocks. 00052 */ 00053 typedef struct PmBlock_s 00054 { 00055 /** Obligatory obj descriptor */ 00056 PmObjDesc_t od; 00057 00058 /** Ptr to backup stack ptr */ 00059 pPmObj_t *b_sp; 00060 00061 /** Handler fxn obj */ 00062 uint8_t const *b_handler; 00063 00064 /** Block type */ 00065 PmBlockType_t b_type:8; 00066 00067 /** Next block in stack */ 00068 struct PmBlock_s *next; 00069 } PmBlock_t, 00070 *pPmBlock_t; 00071 00072 00073 /** 00074 * Frame 00075 * 00076 * A struct that holds the execution frame of a function, including the stack, 00077 * local vars and pointer to the code object. 00078 * 00079 * This struct doesn't declare the stack. 00080 * frame_new() is responsible for allocating the extra memory 00081 * at the tail of fo_locals[] to hold both the locals and stack. 00082 */ 00083 typedef struct PmFrame_s 00084 { 00085 /** Obligatory obj descriptor */ 00086 PmObjDesc_t od; 00087 00088 /** Ptr to previous frame obj */ 00089 struct PmFrame_s *fo_back; 00090 00091 /** Ptr to fxn obj */ 00092 pPmFunc_t fo_func; 00093 00094 /** Mem space where func's CO comes from */ 00095 PmMemSpace_t fo_memspace:8; 00096 00097 /** Instrxn ptr (pts into memspace) */ 00098 uint8_t const *fo_ip; 00099 00100 /** Linked list of blocks */ 00101 pPmBlock_t fo_blockstack; 00102 00103 /** Local attributes dict (non-fast locals) */ 00104 pPmDict_t fo_attrs; 00105 00106 /** Global attributes dict (pts to root frame's globals */ 00107 pPmDict_t fo_globals; 00108 00109 /** Points to next empty slot in fo_locals (1 past TOS) */ 00110 pPmObj_t *fo_sp; 00111 00112 /** Frame can be an import-frame that handles RETURN differently */ 00113 uint8_t fo_isImport:1; 00114 00115 #ifdef HAVE_CLASSES 00116 /** Flag to indicate class initailzer frame; handle RETURN differently */ 00117 uint8_t fo_isInit:1; 00118 #endif /* HAVE_CLASSES */ 00119 00120 /** Array of local vars and stack (space appended at alloc) */ 00121 pPmObj_t fo_locals[1]; 00122 /* WARNING: Do not put new fields below fo_locals */ 00123 } PmFrame_t, 00124 *pPmFrame_t; 00125 00126 00127 /** 00128 * Native Frame 00129 * 00130 * A struct that holds the execution frame of a native function, 00131 * including the args and single stack slot, and pointer to the code object. 00132 * 00133 * This struct doesn't need an OD because it is only used statically in the 00134 * globals struct. There's only one native frame, the global one. 00135 * This happens because a native function is a leaf node 00136 * in the call tree (a native func can't call python funcs). 00137 */ 00138 typedef struct PmNativeFrame_s 00139 { 00140 /** Object descriptor */ 00141 PmObjDesc_t od; 00142 00143 /** Ptr to previous frame obj */ 00144 struct PmFrame_s *nf_back; 00145 00146 /** Ptr to fxn obj */ 00147 pPmFunc_t nf_func; 00148 00149 /** Single stack slot */ 00150 pPmObj_t nf_stack; 00151 00152 /** Boolean to indicate if the native frame is active */ 00153 uint8_t nf_active; 00154 00155 /** Number of args passed to the native function */ 00156 uint8_t nf_numlocals; 00157 00158 /** Local vars */ 00159 pPmObj_t nf_locals[NATIVE_MAX_NUM_LOCALS]; 00160 } PmNativeFrame_t, 00161 *pPmNativeFrame_t; 00162 00163 00164 /** 00165 * Allocate space for a new frame, fill its fields 00166 * with respect to the given function object. 00167 * Return pointer to the new frame. 00168 * 00169 * @param pfunc ptr to Function object. 00170 * @param r_pobj Return value; the new frame. 00171 * @return Return status. 00172 */ 00173 PmReturn_t frame_new(pPmObj_t pfunc, pPmObj_t *r_pobj); 00174 00175 #endif /* __FRAME_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
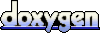