
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
float.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2009 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __FLOAT_H__ 00010 #define __FLOAT_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief Float Object Type 00016 * 00017 * Float object type header. 00018 */ 00019 00020 00021 /** 00022 * Float obj 00023 * 00024 * 32b floating point number 00025 */ 00026 typedef struct PmFloat_s 00027 { 00028 /** Object descriptor */ 00029 PmObjDesc_t od; 00030 00031 /** Float value */ 00032 float val; 00033 } PmFloat_t, *pPmFloat_t; 00034 00035 00036 #ifdef HAVE_FLOAT 00037 00038 /** 00039 * Creates a new Float object 00040 * 00041 * @param f Value to assign float (signed 32-bit). 00042 * @param r_pint Return by ref, ptr to new float 00043 * @return Return status 00044 */ 00045 PmReturn_t float_new(float f, pPmObj_t *r_pf); 00046 00047 /** 00048 * Implements the UNARY_NEGATIVE bcode. 00049 * 00050 * Creates a new float with a value that is the negative of the given float. 00051 * 00052 * @param pobj Pointer to target object 00053 * @param r_pint Return by ref, ptr to float 00054 * @return Return status 00055 */ 00056 PmReturn_t float_negative(pPmObj_t pf, pPmObj_t *r_pf); 00057 00058 /** 00059 * Returns by reference a float that is x op y. 00060 * 00061 * @param px The float left-hand argument 00062 * @param py The float right-hand argument 00063 * @param r_pn The return value of x op y 00064 * @param op The operator (+,-,*,/ and power) 00065 * @return Return status 00066 */ 00067 PmReturn_t float_op(pPmObj_t px, pPmObj_t py, pPmObj_t *r_pn, int8_t op); 00068 00069 /** 00070 * Returns by reference a boolean that is x op y. 00071 * 00072 * @param px The float left-hand argument 00073 * @param py The float right-hand argument 00074 * @param r_pn The return value of x cmp y 00075 * @param cmp The comparison operator 00076 * @return Return status 00077 */ 00078 PmReturn_t float_compare(pPmObj_t px, pPmObj_t py, pPmObj_t *r_pobj, 00079 PmCompare_t cmp); 00080 00081 #ifdef HAVE_PRINT 00082 /** 00083 * Sends out a float object. 00084 * The number is preceded with a "-" when necessary. 00085 * 00086 * @param pObj Ptr to float object 00087 * @return Return status 00088 */ 00089 PmReturn_t float_print(pPmObj_t pf); 00090 00091 #endif /* HAVE_PRINT */ 00092 00093 #endif /* HAVE_FLOAT */ 00094 00095 #endif /* __FLOAT_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
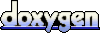