
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
dict.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __DICT_H__ 00010 #define __DICT_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief Dict Object Type 00016 * 00017 * Dict object type header. 00018 */ 00019 00020 00021 /** 00022 * Dict 00023 * 00024 * Contains ptr to two seglists, 00025 * one for keys, the other for values; 00026 * and a length, the number of key/value pairs. 00027 */ 00028 typedef struct PmDict_s 00029 { 00030 /** object descriptor */ 00031 PmObjDesc_t od; 00032 /** number of key,value pairs in the dict */ 00033 uint16_t length; 00034 /** ptr to seglist containing keys */ 00035 pSeglist_t d_keys; 00036 /** ptr to seglist containing values */ 00037 pSeglist_t d_vals; 00038 } PmDict_t, 00039 *pPmDict_t; 00040 00041 00042 /** 00043 * Clears the contents of a dict. 00044 * after this operation, the dict should in the same state 00045 * as if it were just created using dict_new(). 00046 * 00047 * @param pdict ptr to dict to clear. 00048 * @return nothing 00049 */ 00050 PmReturn_t dict_clear(pPmObj_t pdict); 00051 00052 /** 00053 * Gets the value in the dict using the given key. 00054 * 00055 * @param pdict ptr to dict to search 00056 * @param pkey ptr to key obj 00057 * @param r_pobj Return; addr of ptr to obj 00058 * @return Return status 00059 */ 00060 PmReturn_t dict_getItem(pPmObj_t pdict, pPmObj_t pkey, pPmObj_t *r_pobj); 00061 00062 #ifdef HAVE_DEL 00063 /** 00064 * Removes a key and value from the dict. 00065 * Throws TypeError if pdict is not a dict. 00066 * Throws KeyError if pkey does not exist in pdict. 00067 * 00068 * @param pdict Ptr to dict to search 00069 * @param pkey Ptr to key obj 00070 * @return Return status 00071 */ 00072 PmReturn_t dict_delItem(pPmObj_t pdict, pPmObj_t pkey); 00073 #endif /* HAVE_DEL */ 00074 00075 /** 00076 * Allocates space for a new Dict. 00077 * Return a pointer to the dict by reference. 00078 * 00079 * @param r_pdict Return; Addr of ptr to dict 00080 * @return Return status 00081 */ 00082 PmReturn_t dict_new(pPmObj_t *r_pdict); 00083 00084 /** 00085 * Sets a value in the dict using the given key. 00086 * 00087 * If the dict already contains a matching key, the value is 00088 * replaced; otherwise the new key,val pair is inserted 00089 * at the front of the dict (for fast lookup). 00090 * In the later case, the length of the dict is incremented. 00091 * 00092 * @param pdict ptr to dict in which (key,val) will go 00093 * @param pkey ptr to key obj 00094 * @param pval ptr to val obj 00095 * @return Return status 00096 */ 00097 PmReturn_t dict_setItem(pPmObj_t pdict, pPmObj_t pkey, pPmObj_t pval); 00098 00099 #ifdef HAVE_PRINT 00100 /** 00101 * Prints out a dict. Uses obj_print() to print elements. 00102 * 00103 * @param pobj Object to print. 00104 * @return Return status 00105 */ 00106 PmReturn_t dict_print(pPmObj_t pdict); 00107 #endif /* HAVE_PRINT */ 00108 00109 /** 00110 * Updates the destination dict with the key,value pairs from the source dict 00111 * 00112 * @param pdestdict ptr to destination dict in which key,val pairs will go 00113 * @param psourcedict ptr to source dict which has all key,val pairs to copy 00114 * @param omit_underscored Boolean set to true to omit key,val pairs where 00115 * the key starts with an underscore '_'. 00116 * @return Return status 00117 */ 00118 PmReturn_t dict_update(pPmObj_t pdestdict, pPmObj_t psourcedict, 00119 uint8_t omit_underscored); 00120 00121 /** 00122 * Returns C_SAME if the two given dictionaries have the same contents 00123 * 00124 * @param d1 ptr to a dictionary object 00125 * @param d2 ptr to another dictionary object 00126 * @return C_DIFFER or C_SAME 00127 */ 00128 int8_t dict_compare(pPmObj_t d1, pPmObj_t d2); 00129 00130 #endif /* __DICT_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
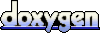