
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
Buttons.cpp
00001 #include "Buttons.h" 00002 #include "PinDetect.h" 00003 00004 PinDetect nextButton(p14); 00005 PinDetect selectButton(p16); // Input selectButton 00006 PinDetect prevButton(p15); 00007 00008 Buttons::Buttons(void): which(0), pressed(false) 00009 { 00010 } 00011 00012 void Buttons::init() 00013 { 00014 00015 // Set up button (plugs into two GPIOs, active low 00016 selectButton.mode(PullUp); 00017 selectButton.setSamplesTillAssert(50); 00018 selectButton.setAssertValue(0); // active low logic 00019 selectButton.setSampleFrequency(50); // us 00020 selectButton.attach_asserted( this, &Buttons::selectPressed ); 00021 00022 nextButton.mode(PullUp); 00023 nextButton.setSamplesTillAssert(50); 00024 nextButton.setAssertValue(0); // active low logic 00025 nextButton.setSampleFrequency(50); // us 00026 nextButton.attach_asserted( this, &Buttons::nextPressed ); 00027 00028 prevButton.mode(PullUp); 00029 prevButton.setSamplesTillAssert(50); 00030 prevButton.setAssertValue(0); // active low logic 00031 prevButton.setSampleFrequency(50); // us 00032 prevButton.attach_asserted( this, &Buttons::prevPressed ); 00033 } 00034 00035 void Buttons::nextPressed() 00036 { 00037 pressed = true; 00038 which = NEXT_BUTTON; 00039 } 00040 00041 void Buttons::prevPressed() 00042 { 00043 pressed = true; 00044 which = PREV_BUTTON; 00045 } 00046 00047 void Buttons::selectPressed() 00048 { 00049 pressed = true; 00050 which = SELECT_BUTTON; 00051 }
Generated on Tue Jul 12 2022 14:09:25 by
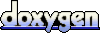