Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
FileLike Class Reference
A file-like object is one that can be opened with fopen by fopen("/name", mode). More...
#include <FileLike.h>
Inherits mbed::Base, and mbed::FileHandle.
Inherited by Stream.
Public Member Functions | |
FileLike (const char *name) | |
FileLike constructor. | |
void | register_object (const char *name) |
Registers this object with the given name, so that it can be looked up with lookup. | |
const char * | name () |
Returns the name of the object. | |
virtual bool | rpc (const char *method, const char *arguments, char *result) |
Call the given method with the given arguments, and write the result into the string pointed to by result. | |
virtual struct rpc_method * | get_rpc_methods () |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). | |
virtual ssize_t | write (const void *buffer, size_t length)=0 |
Write the contents of a buffer to the file. | |
virtual int | close ()=0 |
Close the file. | |
virtual ssize_t | read (void *buffer, size_t length)=0 |
Function read Reads the contents of the file into a buffer. | |
virtual int | isatty ()=0 |
Check if the handle is for a interactive terminal device. | |
virtual off_t | lseek (off_t offset, int whence)=0 |
Move the file position to a given offset from a given location. | |
virtual int | fsync ()=0 |
Flush any buffers associated with the FileHandle, ensuring it is up to date on disk. | |
Static Public Member Functions | |
static bool | rpc (const char *name, const char *method, const char *arguments, char *result) |
Use the lookup function to lookup an object and, if successful, call its rpc method. | |
static Base * | lookup (const char *name, unsigned int len) |
Lookup and return the object that has the given name. | |
template<class C > | |
static void | add_rpc_class () |
Add the class to the list of classes which can have static methods called via rpc (the static methods which can be called are defined by that class' get_rpc_class() static method). |
Detailed Description
A file-like object is one that can be opened with fopen by fopen("/name", mode).
It is intersection of the classes Base and FileHandle.
Definition at line 17 of file FileLike.h.
Constructor & Destructor Documentation
FileLike | ( | const char * | name ) |
FileLike constructor.
- Parameters:
-
name The name to use to open the file.
Definition at line 24 of file FileLike.h.
Member Function Documentation
static void add_rpc_class | ( | ) | [static, inherited] |
virtual int close | ( | ) | [pure virtual, inherited] |
Close the file.
- Returns:
- Zero on success, -1 on error.
virtual int fsync | ( | ) | [pure virtual, inherited] |
Flush any buffers associated with the FileHandle, ensuring it is up to date on disk.
- Returns:
- 0 on success or un-needed, -1 on error
virtual struct rpc_method* get_rpc_methods | ( | ) | [read, virtual, inherited] |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass).
Example
class Example : public Base { int foo(int a, int b) { return a + b; } virtual const struct rpc_method *get_rpc_methods() { static const rpc_method rpc_methods[] = { { "foo", generic_caller<int, Example, int, int, &Example::foo> }, RPC_METHOD_SUPER(Base) }; return rpc_methods; } };
Reimplemented in AnalogIn, AnalogOut, BusIn, BusInOut, BusOut, DigitalIn, DigitalInOut, DigitalOut, PwmOut, Serial, SPI, and Timer.
virtual int isatty | ( | ) | [pure virtual, inherited] |
Check if the handle is for a interactive terminal device.
If so, line buffered behaviour is used by default
- Returns:
- 1 if it is a terminal, 0 otherwise
static Base* lookup | ( | const char * | name, |
unsigned int | len | ||
) | [static, inherited] |
Lookup and return the object that has the given name.
- Parameters:
-
name the name to lookup. len the length of name.
virtual off_t lseek | ( | off_t | offset, |
int | whence | ||
) | [pure virtual, inherited] |
Move the file position to a given offset from a given location.
- Parameters:
-
offset The offset from whence to move to whence SEEK_SET for the start of the file, SEEK_CUR for the current file position, or SEEK_END for the end of the file.
- Returns:
- new file position on success, -1 on failure or unsupported
const char* name | ( | ) | [inherited] |
Returns the name of the object.
- Returns:
- The name of the object, or NULL if it has no name.
virtual ssize_t read | ( | void * | buffer, |
size_t | length | ||
) | [pure virtual, inherited] |
Function read Reads the contents of the file into a buffer.
- Parameters:
-
buffer the buffer to read in to length the number of characters to read
- Returns:
- The number of characters read (zero at end of file) on success, -1 on error.
void register_object | ( | const char * | name ) | [inherited] |
Registers this object with the given name, so that it can be looked up with lookup.
If this object has already been registered, then this just changes the name.
- Parameters:
-
name The name to give the object. If NULL we do nothing.
virtual bool rpc | ( | const char * | method, |
const char * | arguments, | ||
char * | result | ||
) | [virtual, inherited] |
Call the given method with the given arguments, and write the result into the string pointed to by result.
The default implementation calls rpc_methods to determine the supported methods.
- Parameters:
-
method The name of the method to call. arguments A list of arguments separated by spaces. result A pointer to a string to write the result into. May be NULL, in which case nothing is written.
- Returns:
- true if method corresponds to a valid rpc method, or false otherwise.
static bool rpc | ( | const char * | name, |
const char * | method, | ||
const char * | arguments, | ||
char * | result | ||
) | [static, inherited] |
Use the lookup function to lookup an object and, if successful, call its rpc method.
- Returns:
- false if name does not correspond to an object, otherwise the return value of the call to the object's rpc method.
virtual ssize_t write | ( | const void * | buffer, |
size_t | length | ||
) | [pure virtual, inherited] |
Write the contents of a buffer to the file.
- Parameters:
-
buffer the buffer to write from length the number of characters to write
- Returns:
- The number of characters written (possibly 0) on success, -1 on error.
Generated on Tue Jul 12 2022 11:27:31 by
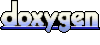