XBee-mbed library http://mbed.org/users/okini3939/notebook/xbee-mbed/
Dependents: device_server_udp led_sender_post XBee_API_ex1 XBee_API_ex2 ... more
XBeeResponse Class Reference
The super class of all XBee responses (RX packets) Users should never attempt to create an instance of this class; instead create an instance of a subclass It is recommend to reuse subclasses to conserve memory. More...
#include <XBee.h>
Inherited by FrameIdResponse, ModemStatusResponse, and RxDataResponse.
Public Member Functions | |
XBeeResponse () | |
Default constructor. | |
uint8_t | getApiId () |
Returns Api Id of the response. | |
uint8_t | getMsbLength () |
Returns the MSB length of the packet. | |
uint8_t | getLsbLength () |
Returns the LSB length of the packet. | |
uint8_t | getChecksum () |
Returns the packet checksum. | |
uint16_t | getFrameDataLength () |
Returns the length of the frame data: all bytes after the api id, and prior to the checksum Note up to release 0.1.2, this was incorrectly including the checksum in the length. | |
uint8_t * | getFrameData () |
Returns the buffer that contains the response. | |
uint16_t | getPacketLength () |
Returns the length of the packet. | |
void | reset () |
Resets the response to default values. | |
void | init () |
Initializes the response. | |
void | getZBTxStatusResponse (XBeeResponse &response) |
Call with instance of ZBTxStatusResponse class only if getApiId() == ZB_TX_STATUS_RESPONSE to populate response. | |
void | getZBRxResponse (XBeeResponse &response) |
Call with instance of ZBRxResponse class only if getApiId() == ZB_RX_RESPONSE to populate response. | |
void | getZBRxIoSampleResponse (XBeeResponse &response) |
Call with instance of ZBRxIoSampleResponse class only if getApiId() == ZB_IO_SAMPLE_RESPONSE to populate response. | |
void | getTxStatusResponse (XBeeResponse &response) |
Call with instance of TxStatusResponse only if getApiId() == TX_STATUS_RESPONSE. | |
void | getRx16Response (XBeeResponse &response) |
Call with instance of Rx16Response only if getApiId() == RX_16_RESPONSE. | |
void | getRx64Response (XBeeResponse &response) |
Call with instance of Rx64Response only if getApiId() == RX_64_RESPONSE. | |
void | getRx16IoSampleResponse (XBeeResponse &response) |
Call with instance of Rx16IoSampleResponse only if getApiId() == RX_16_IO_RESPONSE. | |
void | getRx64IoSampleResponse (XBeeResponse &response) |
Call with instance of Rx64IoSampleResponse only if getApiId() == RX_64_IO_RESPONSE. | |
void | getAtCommandResponse (XBeeResponse &responses) |
Call with instance of AtCommandResponse only if getApiId() == AT_COMMAND_RESPONSE. | |
void | getRemoteAtCommandResponse (XBeeResponse &response) |
Call with instance of RemoteAtCommandResponse only if getApiId() == REMOTE_AT_COMMAND_RESPONSE. | |
void | getModemStatusResponse (XBeeResponse &response) |
Call with instance of ModemStatusResponse only if getApiId() == MODEM_STATUS_RESPONSE. | |
bool | isAvailable () |
Returns true if the response has been successfully parsed and is complete and ready for use. | |
bool | isError () |
Returns true if the response contains errors. | |
uint8_t | getErrorCode () |
Returns an error code, or zero, if successful. |
Detailed Description
The super class of all XBee responses (RX packets) Users should never attempt to create an instance of this class; instead create an instance of a subclass It is recommend to reuse subclasses to conserve memory.
Definition at line 159 of file XBee.h.
Constructor & Destructor Documentation
XBeeResponse | ( | ) |
Member Function Documentation
void getAtCommandResponse | ( | XBeeResponse & | responses ) |
Call with instance of AtCommandResponse only if getApiId() == AT_COMMAND_RESPONSE.
uint8_t getErrorCode | ( | ) |
uint8_t * getFrameData | ( | ) |
Returns the buffer that contains the response.
Starts with byte that follows API ID and includes all bytes prior to the checksum Length is specified by getFrameDataLength() Note: Unlike Digi's definition of the frame data, this does not start with the API ID.. The reason for this is all responses include an API ID, whereas my frame data includes only the API specific data.
uint16_t getFrameDataLength | ( | ) |
uint8_t getLsbLength | ( | ) |
void getModemStatusResponse | ( | XBeeResponse & | response ) |
Call with instance of ModemStatusResponse only if getApiId() == MODEM_STATUS_RESPONSE.
uint8_t getMsbLength | ( | ) |
uint16_t getPacketLength | ( | ) |
void getRemoteAtCommandResponse | ( | XBeeResponse & | response ) |
Call with instance of RemoteAtCommandResponse only if getApiId() == REMOTE_AT_COMMAND_RESPONSE.
void getRx16IoSampleResponse | ( | XBeeResponse & | response ) |
Call with instance of Rx16IoSampleResponse only if getApiId() == RX_16_IO_RESPONSE.
void getRx16Response | ( | XBeeResponse & | response ) |
Call with instance of Rx16Response only if getApiId() == RX_16_RESPONSE.
void getRx64IoSampleResponse | ( | XBeeResponse & | response ) |
Call with instance of Rx64IoSampleResponse only if getApiId() == RX_64_IO_RESPONSE.
void getRx64Response | ( | XBeeResponse & | response ) |
Call with instance of Rx64Response only if getApiId() == RX_64_RESPONSE.
void getTxStatusResponse | ( | XBeeResponse & | response ) |
Call with instance of TxStatusResponse only if getApiId() == TX_STATUS_RESPONSE.
void getZBRxIoSampleResponse | ( | XBeeResponse & | response ) |
Call with instance of ZBRxIoSampleResponse class only if getApiId() == ZB_IO_SAMPLE_RESPONSE to populate response.
void getZBRxResponse | ( | XBeeResponse & | response ) |
Call with instance of ZBRxResponse class only if getApiId() == ZB_RX_RESPONSE to populate response.
void getZBTxStatusResponse | ( | XBeeResponse & | response ) |
Call with instance of ZBTxStatusResponse class only if getApiId() == ZB_TX_STATUS_RESPONSE to populate response.
bool isAvailable | ( | ) |
bool isError | ( | ) |
Generated on Tue Jul 12 2022 18:07:39 by
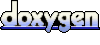