I2C interface for LG1300L IMU
Dependents: LG1300L_Hello_World LG1300L_Hello_World_LCD
LG1300L Class Reference
CruizCore XG1300L IMU interface Used for communicating with an XG1300L IMU over I2C. More...
#include <LG1300L.h>
Public Member Functions | |
LG1300L (I2C &i2c, int ACC_SETTING) | |
Create an IMU readout interface. | |
float | GetAngle () |
Returns a float containing the current Accumulated Angle. | |
float | GetROT () |
Returns a float containing the current ROT. | |
float | GetACC_X () |
Returns a float containing the current acceleration on the X axis. | |
float | GetACC_Y () |
Returns a float containing the current acceleration on the Y axis. | |
float | GetACC_Z () |
Returns a float containing the current acceleration on the Z axis. |
Detailed Description
CruizCore XG1300L IMU interface Used for communicating with an XG1300L IMU over I2C.
*#include "mbed.h" *#include "TextLCD.h" *#include "LG1300L.h" *TextLCD lcd(p15, p16, p17, p18, p19, p20); // rs, e, d4-d7 *Serial pc(USBTX, USBRX); // tx, rx *I2C i2c(p28,p27); *DigitalOut LED (LED1); *int main() { LG1300L IMU(i2c, 2); while(1) { float angle = IMU.GetAngle(); float ROT= IMU.GetROT(); float X_ACC = IMU.GetACC_X(); float Y_ACC = IMU. GetACC_Y(); float Z_ACC = IMU. GetACC_Z(); pc.printf("///////////////////////////////////\n"); pc.printf("//ANGLE: %f\n", angle); pc.printf("//ROT: %f\n", ROT); pc.printf("//X-Axis: %f\n", X_ACC ); pc.printf("//Y-Axis: %f\n", Y_ACC ); pc.printf("//Z-axis: %f\n", Z_ACC ); pc.printf("///////////////////////////////////\n"); wait(1); } *}
Definition at line 43 of file LG1300L.h.
Constructor & Destructor Documentation
LG1300L | ( | I2C & | i2c, |
int | ACC_SETTING | ||
) |
Create an IMU readout interface.
- Parameters:
-
i2c The instantiated I2C bus to be used ACC_SETTING the desired initialization range for the
- Note:
- Create an IMU readout interface accelerometers, choices are +-2G, +-4G, or +-8G
Definition at line 6 of file LG1300L.cpp.
Member Function Documentation
float GetACC_X | ( | ) |
Returns a float containing the current acceleration on the X axis.
- Returns:
- the acceleration on the X_Axis
Definition at line 67 of file LG1300L.cpp.
float GetACC_Y | ( | ) |
Returns a float containing the current acceleration on the Y axis.
- Returns:
- the acceleration on the Y_Axis
Definition at line 77 of file LG1300L.cpp.
float GetACC_Z | ( | ) |
Returns a float containing the current acceleration on the Z axis.
- Returns:
- the acceleration on the Z_Axis
Definition at line 88 of file LG1300L.cpp.
float GetAngle | ( | ) |
Returns a float containing the current Accumulated Angle.
- Returns:
- the Accumulated Angle
Definition at line 44 of file LG1300L.cpp.
float GetROT | ( | ) |
Returns a float containing the current ROT.
- Returns:
- the Rate of Turn
Definition at line 56 of file LG1300L.cpp.
Generated on Tue Jul 12 2022 14:05:29 by
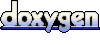