
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_spi.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_spi.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for SPI firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 3. April. 2009 00007 * @author : HieuNguyen 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup SPI 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_SPI_H_ 00028 #define LPC17XX_SPI_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 /* Private Macros ------------------------------------------------------------- */ 00041 /** @defgroup SPI_Private_Macros 00042 * @{ 00043 */ 00044 00045 /*********************************************************************//** 00046 * Macro defines for SPI Control Register 00047 **********************************************************************/ 00048 /** @defgroup SPI_REGISTER_BIT_DEFINITION 00049 * @{ 00050 */ 00051 00052 /** Bit enable, the SPI controller sends and receives the number 00053 * of bits selected by bits 11:8 */ 00054 #define SPI_SPCR_BIT_EN ((uint32_t)(1<<2)) 00055 /** Clock phase control bit */ 00056 #define SPI_SPCR_CPHA_SECOND ((uint32_t)(1<<3)) 00057 /** Clock polarity control bit */ 00058 #define SPI_SPCR_CPOL_LOW ((uint32_t)(1<<4)) 00059 /** SPI master mode enable */ 00060 #define SPI_SPCR_MSTR ((uint32_t)(1<<5)) 00061 /** LSB enable bit */ 00062 #define SPI_SPCR_LSBF ((uint32_t)(1<<6)) 00063 /** SPI interrupt enable bit */ 00064 #define SPI_SPCR_SPIE ((uint32_t)(1<<7)) 00065 /** When bit 2 of this register is 1, this field controls the 00066 number of bits per transfer */ 00067 #define SPI_SPCR_BITS(n) ((n==0) ? ((uint32_t)0) : ((uint32_t)((n&0x0F)<<8))) 00068 /** SPI Control bit mask */ 00069 #define SPI_SPCR_BITMASK ((uint32_t)(0xFFC)) 00070 00071 00072 /*********************************************************************//** 00073 * Macro defines for SPI Status Register 00074 **********************************************************************/ 00075 /** Slave abort */ 00076 #define SPI_SPSR_ABRT ((uint32_t)(1<<3)) 00077 /** Mode fault */ 00078 #define SPI_SPSR_MODF ((uint32_t)(1<<4)) 00079 /** Read overrun */ 00080 #define SPI_SPSR_ROVR ((uint32_t)(1<<5)) 00081 /** Write collision */ 00082 #define SPI_SPSR_WCOL ((uint32_t)(1<<6)) 00083 /** SPI transfer complete flag */ 00084 #define SPI_SPSR_SPIF ((uint32_t)(1<<7)) 00085 /** SPI Status bit mask */ 00086 #define SPI_SPSR_BITMASK ((uint32_t)(0xF8)) 00087 00088 00089 /*********************************************************************//** 00090 * Macro defines for SPI Data Register 00091 **********************************************************************/ 00092 /** SPI Data low bit-mask */ 00093 #define SPI_SPDR_LO_MASK ((uint32_t)(0xFF)) 00094 /** SPI Data high bit-mask */ 00095 #define SPI_SPDR_HI_MASK ((uint32_t)(0xFF00)) 00096 /** SPI Data bit-mask */ 00097 #define SPI_SPDR_BITMASK ((uint32_t)(0xFFFF)) 00098 00099 00100 /*********************************************************************//** 00101 * Macro defines for SPI Clock Counter Register 00102 **********************************************************************/ 00103 /** SPI clock counter setting */ 00104 #define SPI_SPCCR_COUNTER(n) ((uint32_t)(n&0xFF)) 00105 /** SPI clock counter bit-mask */ 00106 #define SPI_SPCCR_BITMASK ((uint32_t)(0xFF)) 00107 00108 00109 /*********************************************************************** 00110 * Macro defines for SPI Test Control Register 00111 **********************************************************************/ 00112 /** SPI Test bit */ 00113 #define SPI_SPTCR_TEST_MASK ((uint32_t)(0xFE)) 00114 /** SPI Test register bit mask */ 00115 #define SPI_SPTCR_BITMASK ((uint32_t)(0xFE)) 00116 00117 00118 00119 /*********************************************************************//** 00120 * Macro defines for SPI Test Status Register 00121 **********************************************************************/ 00122 /** Slave abort */ 00123 #define SPI_SPTSR_ABRT ((uint32_t)(1<<3)) 00124 /** Mode fault */ 00125 #define SPI_SPTSR_MODF ((uint32_t)(1<<4)) 00126 /** Read overrun */ 00127 #define SPI_SPTSR_ROVR ((uint32_t)(1<<5)) 00128 /** Write collision */ 00129 #define SPI_SPTSR_WCOL ((uint32_t)(1<<6)) 00130 /** SPI transfer complete flag */ 00131 #define SPI_SPTSR_SPIF ((uint32_t)(1<<7)) 00132 /** SPI Status bit mask */ 00133 #define SPI_SPTSR_MASKBIT ((uint32_t)(0xF8)) 00134 00135 00136 00137 /*********************************************************************//** 00138 * Macro defines for SPI Interrupt Register 00139 **********************************************************************/ 00140 /** SPI interrupt flag */ 00141 #define SPI_SPINT_INTFLAG ((uint32_t)(1<<0)) 00142 /** SPI interrupt register bit mask */ 00143 #define SPI_SPINT_BITMASK ((uint32_t)(0x01)) 00144 00145 /** 00146 * @} 00147 */ 00148 00149 /** 00150 * @} 00151 */ 00152 00153 00154 /* Public Types --------------------------------------------------------------- */ 00155 /** @defgroup SPI_Public_Types 00156 * @{ 00157 */ 00158 00159 /** @brief SPI configuration structure */ 00160 typedef struct { 00161 uint32_t Databit; /** Databit number, should be SPI_DATABIT_x, 00162 where x is in range from 8 - 16 */ 00163 uint32_t CPHA; /** Clock phase, should be: 00164 - SPI_CPHA_FIRST: first clock edge 00165 - SPI_CPHA_SECOND: second clock edge */ 00166 uint32_t CPOL; /** Clock polarity, should be: 00167 - SPI_CPOL_HI: high level 00168 - SPI_CPOL_LO: low level */ 00169 uint32_t Mode; /** SPI mode, should be: 00170 - SPI_MASTER_MODE: Master mode 00171 - SPI_SLAVE_MODE: Slave mode */ 00172 uint32_t DataOrder; /** Data order, should be: 00173 - SPI_DATA_MSB_FIRST: MSB first 00174 - SPI_DATA_LSB_FIRST: LSB first */ 00175 uint32_t ClockRate; /** Clock rate,in Hz, should not exceed 00176 (SPI peripheral clock)/8 */ 00177 } SPI_CFG_Type; 00178 00179 00180 /** 00181 * @brief SPI Transfer Type definitions 00182 */ 00183 typedef enum { 00184 SPI_TRANSFER_POLLING = 0, /**< Polling transfer */ 00185 SPI_TRANSFER_INTERRUPT /**< Interrupt transfer */ 00186 } SPI_TRANSFER_Type; 00187 00188 /** 00189 * @brief SPI Data configuration structure definitions 00190 */ 00191 typedef struct { 00192 void *tx_data; /**< Pointer to transmit data */ 00193 void *rx_data; /**< Pointer to transmit data */ 00194 uint32_t length; /**< Length of transfer data */ 00195 uint32_t counter; /**< Data counter index */ 00196 uint32_t status; /**< Current status of SPI activity */ 00197 void (*callback)(void); /**< Pointer to Call back function when transmission complete 00198 used in interrupt transfer mode */ 00199 } SPI_DATA_SETUP_Type; 00200 00201 /** 00202 * @} 00203 */ 00204 00205 00206 /* Public Macros -------------------------------------------------------------- */ 00207 /** @defgroup SPI_Public_Macros 00208 * @{ 00209 */ 00210 00211 /** Macro to determine if it is valid SPI port number */ 00212 #define PARAM_SPIx(n) (((uint32_t *)n)==((uint32_t *)LPC_SPI)) 00213 00214 /*********************************************************************//** 00215 * SPI configuration parameter defines 00216 **********************************************************************/ 00217 /** Clock phase control bit */ 00218 #define SPI_CPHA_FIRST ((uint32_t)(0)) 00219 #define SPI_CPHA_SECOND SPI_SPCR_CPHA_SECOND 00220 #define PARAM_SPI_CPHA(n) ((n==SPI_CPHA_FIRST) || (n==SPI_CPHA_SECOND)) 00221 00222 /** Clock polarity control bit */ 00223 #define SPI_CPOL_HI ((uint32_t)(0)) 00224 #define SPI_CPOL_LO SPI_SPCR_CPOL_LOW 00225 #define PARAM_SPI_CPOL(n) ((n==SPI_CPOL_HI) || (n==SPI_CPOL_LO)) 00226 00227 /** SPI master mode enable */ 00228 #define SPI_SLAVE_MODE ((uint32_t)(0)) 00229 #define SPI_MASTER_MODE SPI_SPCR_MSTR 00230 #define PARAM_SPI_MODE(n) ((n==SPI_SLAVE_MODE) || (n==SPI_MASTER_MODE)) 00231 00232 /** LSB enable bit */ 00233 #define SPI_DATA_MSB_FIRST ((uint32_t)(0)) 00234 #define SPI_DATA_LSB_FIRST SPI_SPCR_LSBF 00235 #define PARAM_SPI_DATA_ORDER(n) ((n==SPI_DATA_MSB_FIRST) || (n==SPI_DATA_LSB_FIRST)) 00236 00237 /** SPI data bit number defines */ 00238 #define SPI_DATABIT_16 SPI_SPCR_BITS(0) /*!< Databit number = 16 */ 00239 #define SPI_DATABIT_8 SPI_SPCR_BITS(0x08) /*!< Databit number = 8 */ 00240 #define SPI_DATABIT_9 SPI_SPCR_BITS(0x09) /*!< Databit number = 9 */ 00241 #define SPI_DATABIT_10 SPI_SPCR_BITS(0x0A) /*!< Databit number = 10 */ 00242 #define SPI_DATABIT_11 SPI_SPCR_BITS(0x0B) /*!< Databit number = 11 */ 00243 #define SPI_DATABIT_12 SPI_SPCR_BITS(0x0C) /*!< Databit number = 12 */ 00244 #define SPI_DATABIT_13 SPI_SPCR_BITS(0x0D) /*!< Databit number = 13 */ 00245 #define SPI_DATABIT_14 SPI_SPCR_BITS(0x0E) /*!< Databit number = 14 */ 00246 #define SPI_DATABIT_15 SPI_SPCR_BITS(0x0F) /*!< Databit number = 15 */ 00247 #define PARAM_SPI_DATABIT(n) ((n==SPI_DATABIT_16) || (n==SPI_DATABIT_8) \ 00248 || (n==SPI_DATABIT_9) || (n==SPI_DATABIT_10) \ 00249 || (n==SPI_DATABIT_11) || (n==SPI_DATABIT_12) \ 00250 || (n==SPI_DATABIT_13) || (n==SPI_DATABIT_14) \ 00251 || (n==SPI_DATABIT_15)) 00252 00253 00254 /*********************************************************************//** 00255 * SPI Status Flag defines 00256 **********************************************************************/ 00257 /** Slave abort */ 00258 #define SPI_STAT_ABRT SPI_SPSR_ABRT 00259 /** Mode fault */ 00260 #define SPI_STAT_MODF SPI_SPSR_MODF 00261 /** Read overrun */ 00262 #define SPI_STAT_ROVR SPI_SPSR_ROVR 00263 /** Write collision */ 00264 #define SPI_STAT_WCOL SPI_SPSR_WCOL 00265 /** SPI transfer complete flag */ 00266 #define SPI_STAT_SPIF SPI_SPSR_SPIF 00267 #define PARAM_SPI_STAT(n) ((n==SPI_STAT_ABRT) || (n==SPI_STAT_MODF) \ 00268 || (n==SPI_STAT_ROVR) || (n==SPI_STAT_WCOL) \ 00269 || (n==SPI_STAT_SPIF)) 00270 00271 00272 /* SPI Status Implementation definitions */ 00273 #define SPI_STAT_DONE (1UL<<8) /**< Done */ 00274 #define SPI_STAT_ERROR (1UL<<9) /**< Error */ 00275 00276 /** 00277 * @} 00278 */ 00279 00280 00281 /* Public Functions ----------------------------------------------------------- */ 00282 /** @defgroup SPI_Public_Functions 00283 * @{ 00284 */ 00285 00286 void SPI_SetClock (LPC_SPI_TypeDef *SPIx, uint32_t target_clock); 00287 void SPI_DeInit(LPC_SPI_TypeDef *SPIx); 00288 void SPI_Init(LPC_SPI_TypeDef *SPIx, SPI_CFG_Type *SPI_ConfigStruct); 00289 void SPI_ConfigStructInit(SPI_CFG_Type *SPI_InitStruct); 00290 void SPI_SendData(LPC_SPI_TypeDef *SPIx, uint16_t Data); 00291 uint16_t SPI_ReceiveData(LPC_SPI_TypeDef *SPIx); 00292 int32_t SPI_ReadWrite (LPC_SPI_TypeDef *SPIx, SPI_DATA_SETUP_Type *dataCfg, SPI_TRANSFER_Type xfType); 00293 void SPI_IntCmd(LPC_SPI_TypeDef *SPIx, FunctionalState NewState); 00294 IntStatus SPI_GetIntStatus (LPC_SPI_TypeDef *SPIx); 00295 void SPI_ClearIntPending(LPC_SPI_TypeDef *SPIx); 00296 uint32_t SPI_GetStatus(LPC_SPI_TypeDef *SPIx); 00297 FlagStatus SPI_CheckStatus (uint32_t inputSPIStatus, uint8_t SPIStatus); 00298 void SPI_StdIntHandler(void); 00299 00300 /** 00301 * @} 00302 */ 00303 00304 #ifdef __cplusplus 00305 } 00306 #endif 00307 00308 #endif /* LPC17XX_SPI_H_ */ 00309 00310 /** 00311 * @} 00312 */ 00313 00314 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
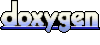