
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
Functions | |
void | SPI_SetClock (LPC_SPI_TypeDef *SPIx, uint32_t target_clock) |
Setup clock rate for SPI device. | |
void | SPI_DeInit (LPC_SPI_TypeDef *SPIx) |
De-initializes the SPIx peripheral registers to their default reset values. | |
void | SPI_Init (LPC_SPI_TypeDef *SPIx, SPI_CFG_Type *SPI_ConfigStruct) |
Initializes the SPIx peripheral according to the specified parameters in the UART_ConfigStruct. | |
void | SPI_ConfigStructInit (SPI_CFG_Type *SPI_InitStruct) |
Fills each SPI_InitStruct member with its default value:
| |
void | SPI_SendData (LPC_SPI_TypeDef *SPIx, uint16_t Data) |
Transmit a single data through SPIx peripheral. | |
uint16_t | SPI_ReceiveData (LPC_SPI_TypeDef *SPIx) |
Receive a single data from SPIx peripheral. | |
void | SPI_IntCmd (LPC_SPI_TypeDef *SPIx, FunctionalState NewState) |
Enable or disable SPIx interrupt. | |
IntStatus | SPI_GetIntStatus (LPC_SPI_TypeDef *SPIx) |
Checks whether the SPI interrupt flag is set or not. | |
void | SPI_ClearIntPending (LPC_SPI_TypeDef *SPIx) |
Clear SPI interrupt flag. | |
uint32_t | SPI_GetStatus (LPC_SPI_TypeDef *SPIx) |
Get current value of SPI Status register in SPIx peripheral. | |
FlagStatus | SPI_CheckStatus (uint32_t inputSPIStatus, uint8_t SPIStatus) |
Checks whether the specified SPI Status flag is set or not via inputSPIStatus parameter. | |
void | SPI_StdIntHandler (void) |
Standard SPI Interrupt handler. | |
int32_t | SPI_ReadWrite (LPC_SPI_TypeDef *SPIx, SPI_DATA_SETUP_Type *dataCfg,\SPI_TRANSFER_Type xfType) |
SPI Read write data function. |
Function Documentation
FlagStatus SPI_CheckStatus | ( | uint32_t | inputSPIStatus, |
uint8_t | SPIStatus | ||
) |
Checks whether the specified SPI Status flag is set or not via inputSPIStatus parameter.
- Parameters:
-
[in] inputSPIStatus Value to check status of each flag type. This value is the return value from SPI_GetStatus(). [in] SPIStatus Specifies the SPI status flag to check, should be one of the following: - SPI_STAT_ABRT: Slave abort.
- SPI_STAT_MODF: Mode fault.
- SPI_STAT_ROVR: Read overrun.
- SPI_STAT_WCOL: Write collision.
- SPI_STAT_SPIF: SPI transfer complete.
- Returns:
- The new state of SPIStatus (SET or RESET)
Definition at line 532 of file lpc17xx_spi.c.
void SPI_ClearIntPending | ( | LPC_SPI_TypeDef * | SPIx ) |
Clear SPI interrupt flag.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI
- Returns:
- None
Definition at line 489 of file lpc17xx_spi.c.
void SPI_ConfigStructInit | ( | SPI_CFG_Type * | SPI_InitStruct ) |
Fills each SPI_InitStruct member with its default value:
- CPHA = SPI_CPHA_FIRST
- CPOL = SPI_CPOL_HI
- ClockRate = 1000000
- DataOrder = SPI_DATA_MSB_FIRST
- Databit = SPI_DATABIT_8
- Mode = SPI_MASTER_MODE.
- Parameters:
-
[in] SPI_InitStruct Pointer to a SPI_CFG_Type structure which will be initialized.
- Returns:
- None
Definition at line 280 of file lpc17xx_spi.c.
void SPI_DeInit | ( | LPC_SPI_TypeDef * | SPIx ) |
De-initializes the SPIx peripheral registers to their default reset values.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI
- Returns:
- None
Definition at line 210 of file lpc17xx_spi.c.
IntStatus SPI_GetIntStatus | ( | LPC_SPI_TypeDef * | SPIx ) |
Checks whether the SPI interrupt flag is set or not.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI
- Returns:
- The new state of SPI Interrupt Flag (SET or RESET)
Definition at line 476 of file lpc17xx_spi.c.
uint32_t SPI_GetStatus | ( | LPC_SPI_TypeDef * | SPIx ) |
Get current value of SPI Status register in SPIx peripheral.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI
- Returns:
- Current value of SPI Status register in SPI peripheral. Note: The return value of this function must be used with SPI_CheckStatus() to determine current flag status corresponding to each SPI status type. Because some flags in SPI Status register will be cleared after reading, the next reading SPI Status register could not be correct. So this function used to read SPI status register in one time only, then the return value used to check all flags.
Definition at line 509 of file lpc17xx_spi.c.
void SPI_Init | ( | LPC_SPI_TypeDef * | SPIx, |
SPI_CFG_Type * | SPI_ConfigStruct | ||
) |
Initializes the SPIx peripheral according to the specified parameters in the UART_ConfigStruct.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI [in] SPI_ConfigStruct Pointer to a SPI_CFG_Type structure that contains the configuration information for the specified SPI peripheral.
- Returns:
- None
Definition at line 231 of file lpc17xx_spi.c.
void SPI_IntCmd | ( | LPC_SPI_TypeDef * | SPIx, |
FunctionalState | NewState | ||
) |
Enable or disable SPIx interrupt.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI [in] NewState New state of specified UART interrupt type, should be: - ENALBE: Enable this SPI interrupt.
- DISALBE: Disable this SPI interrupt.
- Returns:
- None
Definition at line 455 of file lpc17xx_spi.c.
int32_t SPI_ReadWrite | ( | LPC_SPI_TypeDef * | SPIx, |
SPI_DATA_SETUP_Type * | dataCfg, | ||
\SPI_TRANSFER_Type | xfType | ||
) |
SPI Read write data function.
- Parameters:
-
[in] SPIx Pointer to SPI peripheral, should be SPI [in] dataCfg Pointer to a SPI_DATA_SETUP_Type structure that contains specified information about transmit data configuration. [in] xfType Transfer type, should be: - SPI_TRANSFER_POLLING: Polling mode
- SPI_TRANSFER_INTERRUPT: Interrupt mode
- Returns:
- Actual Data length has been transferred in polling mode. In interrupt mode, always return (0) Return (-1) if error. Note: This function can be used in both master and slave mode.
Definition at line 332 of file lpc17xx_spi.c.
uint16_t SPI_ReceiveData | ( | LPC_SPI_TypeDef * | SPIx ) |
Receive a single data from SPIx peripheral.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI
- Returns:
- Data received (16-bit long)
Definition at line 311 of file lpc17xx_spi.c.
void SPI_SendData | ( | LPC_SPI_TypeDef * | SPIx, |
uint16_t | Data | ||
) |
Transmit a single data through SPIx peripheral.
- Parameters:
-
[in] SPIx SPI peripheral selected, should be SPI [in] Data Data to transmit (must be 16 or 8-bit long, this depend on SPI data bit number configured)
- Returns:
- none
Definition at line 297 of file lpc17xx_spi.c.
void SPI_SetClock | ( | LPC_SPI_TypeDef * | SPIx, |
uint32_t | target_clock | ||
) |
Setup clock rate for SPI device.
- Parameters:
-
[in] SPIx SPI peripheral definition, should be SPI [in] target_clock : clock of SPI (Hz)
- Returns:
- None
Definition at line 173 of file lpc17xx_spi.c.
void SPI_StdIntHandler | ( | void | ) |
Standard SPI Interrupt handler.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 544 of file lpc17xx_spi.c.
Generated on Tue Jul 12 2022 17:06:03 by
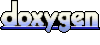