
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_pwm.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_pwm.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for PWM firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 22. Apr. 2009 00007 * @author : HieuNguyen 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup PWM 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_PWM_H_ 00028 #define LPC17XX_PWM_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Private Macros ------------------------------------------------------------- */ 00042 /** @defgroup PWM_Private_Macros 00043 * @{ 00044 */ 00045 00046 /** @defgroup PWM_REGISTER_BIT_DEFINITIONS 00047 * @{ 00048 */ 00049 00050 /********************************************************************** 00051 * IR register definitions 00052 **********************************************************************/ 00053 /** Interrupt flag for PWM match channel for 6 channel */ 00054 #define PWM_IR_PWMMRn(n) ((uint32_t)((n<4)?(1<<n):(1<<(n+4)))) 00055 /** Interrupt flag for capture input */ 00056 #define PWM_IR_PWMCAPn(n) ((uint32_t)(1<<(n+4))) 00057 /** IR register mask */ 00058 #define PWM_IR_BITMASK ((uint32_t)(0x0000073F)) 00059 00060 00061 /********************************************************************** 00062 * TCR register definitions 00063 **********************************************************************/ 00064 /** TCR register mask */ 00065 #define PWM_TCR_BITMASK ((uint32_t)(0x0000000B)) 00066 #define PWM_TCR_COUNTER_ENABLE ((uint32_t)(1<<0)) /*!< PWM Counter Enable */ 00067 #define PWM_TCR_COUNTER_RESET ((uint32_t)(1<<1)) /*!< PWM Counter Reset */ 00068 #define PWM_TCR_PWM_ENABLE ((uint32_t)(1<<3)) /*!< PWM Enable */ 00069 00070 00071 /********************************************************************** 00072 * CTCR register definitions 00073 **********************************************************************/ 00074 /** CTCR register mask */ 00075 #define PWM_CTCR_BITMASK ((uint32_t)(0x0000000F)) 00076 /** PWM Counter-Timer Mode */ 00077 #define PWM_CTCR_MODE(n) ((uint32_t)(n&0x03)) 00078 /** PWM Capture input select */ 00079 #define PWM_CTCR_SELECT_INPUT(n) ((uint32_t)((n&0x03)<<2)) 00080 00081 00082 /********************************************************************** 00083 * MCR register definitions 00084 **********************************************************************/ 00085 /** MCR register mask */ 00086 #define PWM_MCR_BITMASK ((uint32_t)(0x001FFFFF)) 00087 /** generate a PWM interrupt when a MATCHn occurs */ 00088 #define PWM_MCR_INT_ON_MATCH(n) ((uint32_t)(1<<(((n&0x7)<<1)+(n&0x07)))) 00089 /** reset the PWM when a MATCHn occurs */ 00090 #define PWM_MCR_RESET_ON_MATCH(n) ((uint32_t)(1<<(((n&0x7)<<1)+(n&0x07)+1))) 00091 /** stop the PWM when a MATCHn occurs */ 00092 #define PWM_MCR_STOP_ON_MATCH(n) ((uint32_t)(1<<(((n&0x7)<<1)+(n&0x07)+2))) 00093 00094 00095 /********************************************************************** 00096 * CCR register definitions 00097 **********************************************************************/ 00098 /** CCR register mask */ 00099 #define PWM_CCR_BITMASK ((uint32_t)(0x0000003F)) 00100 /** PCAPn is rising edge sensitive */ 00101 #define PWM_CCR_CAP_RISING(n) ((uint32_t)(1<<(((n&0x2)<<1)+(n&0x1)))) 00102 /** PCAPn is falling edge sensitive */ 00103 #define PWM_CCR_CAP_FALLING(n) ((uint32_t)(1<<(((n&0x2)<<1)+(n&0x1)+1))) 00104 /** PWM interrupt is generated on a PCAP event */ 00105 #define PWM_CCR_INT_ON_CAP(n) ((uint32_t)(1<<(((n&0x2)<<1)+(n&0x1)+2))) 00106 00107 00108 /********************************************************************** 00109 * PCR register definitions 00110 **********************************************************************/ 00111 /** PCR register mask */ 00112 #define PWM_PCR_BITMASK (uint32_t)0x00007E7C 00113 /** PWM output n is a single edge controlled output */ 00114 #define PWM_PCR_PWMSELn(n) ((uint32_t)(((n&0x7)<2) ? 0 : (1<<n))) 00115 /** enable PWM output n */ 00116 #define PWM_PCR_PWMENAn(n) ((uint32_t)(((n&0x7)<1) ? 0 : (1<<(n+8)))) 00117 00118 00119 /********************************************************************** 00120 * LER register definitions 00121 **********************************************************************/ 00122 /** LER register mask*/ 00123 #define PWM_LER_BITMASK ((uint32_t)(0x0000007F)) 00124 /** PWM MATCHn register update control */ 00125 #define PWM_LER_EN_MATCHn_LATCH(n) ((uint32_t)((n<7) ? (1<<n) : 0)) 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** 00132 * @} 00133 */ 00134 00135 00136 /* Public Types --------------------------------------------------------------- */ 00137 /** @defgroup PWM_Public_Types 00138 * @{ 00139 */ 00140 00141 /** @brief Configuration structure in PWM TIMER mode */ 00142 typedef struct { 00143 00144 uint8_t PrescaleOption; /**< Prescale option, should be: 00145 - PWM_TIMER_PRESCALE_TICKVAL: Prescale in absolute value 00146 - PWM_TIMER_PRESCALE_USVAL: Prescale in microsecond value 00147 */ 00148 uint8_t Reserved[3]; 00149 uint32_t PrescaleValue; /**< Prescale value, 32-bit long, should be matched 00150 with PrescaleOption 00151 */ 00152 } PWM_TIMERCFG_Type; 00153 00154 /** @brief Configuration structure in PWM COUNTER mode */ 00155 typedef struct { 00156 00157 uint8_t CounterOption; /**< Counter Option, should be: 00158 - PWM_COUNTER_RISING: Rising Edge 00159 - PWM_COUNTER_FALLING: Falling Edge 00160 - PWM_COUNTER_ANY: Both rising and falling mode 00161 */ 00162 uint8_t CountInputSelect; /**< Counter input select, should be: 00163 - PWM_COUNTER_PCAP1_0: PWM Counter input selected is PCAP1.0 pin 00164 - PWM_COUNTER_PCAP1_1: PWM Counter input selected is PCAP1.1 pin 00165 */ 00166 uint8_t Reserved[2]; 00167 } PWM_COUNTERCFG_Type; 00168 00169 /** @brief PWM Match channel configuration structure */ 00170 typedef struct { 00171 uint8_t MatchChannel; /**< Match channel, should be in range 00172 from 0..6 */ 00173 uint8_t IntOnMatch; /**< Interrupt On match, should be: 00174 - ENABLE: Enable this function. 00175 - DISABLE: Disable this function. 00176 */ 00177 uint8_t StopOnMatch; /**< Stop On match, should be: 00178 - ENABLE: Enable this function. 00179 - DISABLE: Disable this function. 00180 */ 00181 uint8_t ResetOnMatch; /**< Reset On match, should be: 00182 - ENABLE: Enable this function. 00183 - DISABLE: Disable this function. 00184 */ 00185 } PWM_MATCHCFG_Type; 00186 00187 00188 /** @brief PWM Capture Input configuration structure */ 00189 typedef struct { 00190 uint8_t CaptureChannel; /**< Capture channel, should be in range 00191 from 0..1 */ 00192 uint8_t RisingEdge; /**< caption rising edge, should be: 00193 - ENABLE: Enable rising edge. 00194 - DISABLE: Disable this function. 00195 */ 00196 uint8_t FallingEdge; /**< caption falling edge, should be: 00197 - ENABLE: Enable falling edge. 00198 - DISABLE: Disable this function. 00199 */ 00200 uint8_t IntOnCaption; /**< Interrupt On caption, should be: 00201 - ENABLE: Enable interrupt function. 00202 - DISABLE: Disable this function. 00203 */ 00204 } PWM_CAPTURECFG_Type; 00205 00206 00207 /** Macro to determine if it is valid PWM peripheral */ 00208 #define PARAM_PWMx(n) (((uint32_t *)n)==((uint32_t *)LPC_PWM1)) 00209 00210 #define PARAM_PWM1_MATCH_CHANNEL(n) ((n<=6)) 00211 #define PARAM_PWM1_CHANNEL(n) ((n>=1) && (n<=6)) 00212 #define PARAM_PWM1_EDGE_MODE_CHANNEL(n) ((n>=2) && (n<=6)) 00213 #define PARAM_PWM1_CAPTURE_CHANNEL(n) ((n==0) || (n==1)) 00214 00215 00216 /* Timer/Counter in PWM configuration type definition -----------------------------------*/ 00217 00218 /** @brief PMW TC mode select option */ 00219 typedef enum { 00220 PWM_MODE_TIMER = 0, /*!< PWM using Timer mode */ 00221 PWM_MODE_COUNTER , /*!< PWM using Counter mode */ 00222 } PWM_TC_MODE_OPT; 00223 00224 #define PARAM_PWM_TC_MODE(n) ((n==PWM_MODE_TIMER) || (n==PWM_MODE_COUNTER)) 00225 00226 00227 /** @brief PWM Timer/Counter prescale option */ 00228 typedef enum 00229 { 00230 PWM_TIMER_PRESCALE_TICKVAL = 0, /*!< Prescale in absolute value */ 00231 PWM_TIMER_PRESCALE_USVAL /*!< Prescale in microsecond value */ 00232 } PWM_TIMER_PRESCALE_OPT; 00233 00234 #define PARAM_PWM_TIMER_PRESCALE(n) ((n==PWM_TIMER_PRESCALE_TICKVAL) || (n==PWM_TIMER_PRESCALE_USVAL)) 00235 00236 00237 /** @brief PWM Input Select in counter mode */ 00238 typedef enum { 00239 PWM_COUNTER_PCAP1_0 = 0, /*!< PWM Counter input selected is PCAP1.0 pin */ 00240 PWM_COUNTER_PCAP1_1 /*!< PWM counter input selected is CAP1.1 pin */ 00241 } PWM_COUNTER_INPUTSEL_OPT; 00242 00243 #define PARAM_PWM_COUNTER_INPUTSEL(n) ((n==PWM_COUNTER_PCAP1_0) || (n==PWM_COUNTER_PCAP1_1)) 00244 00245 /** @brief PWM Input Edge Option in counter mode */ 00246 typedef enum { 00247 PWM_COUNTER_RISING = 1, /*!< Rising edge mode */ 00248 PWM_COUNTER_FALLING = 2, /*!< Falling edge mode */ 00249 PWM_COUNTER_ANY = 3 /*!< Both rising and falling mode */ 00250 } PWM_COUNTER_EDGE_OPT; 00251 00252 #define PARAM_PWM_COUNTER_EDGE(n) ((n==PWM_COUNTER_RISING) || (n==PWM_COUNTER_FALLING) \ 00253 || (n==PWM_COUNTER_ANY)) 00254 00255 00256 /* PWM configuration type definition ----------------------------------------------------- */ 00257 /** @brief PWM operating mode options */ 00258 typedef enum { 00259 PWM_CHANNEL_SINGLE_EDGE , /*!< PWM Channel Single edge mode */ 00260 PWM_CHANNEL_DUAL_EDGE /*!< PWM Channel Dual edge mode */ 00261 } PWM_CHANNEL_EDGE_OPT; 00262 00263 #define PARAM_PWM_CHANNEL_EDGE(n) ((n==PWM_CHANNEL_SINGLE_EDGE) || (n==PWM_CHANNEL_DUAL_EDGE)) 00264 00265 00266 /** @brief PWM update type */ 00267 typedef enum { 00268 PWM_MATCH_UPDATE_NOW = 0, /**< PWM Match Channel Update Now */ 00269 PWM_MATCH_UPDATE_NEXT_RST /**< PWM Match Channel Update on next 00270 PWM Counter resetting */ 00271 } PWM_MATCH_UPDATE_OPT; 00272 00273 #define PARAM_PWM_MATCH_UPDATE(n) ((n==PWM_MATCH_UPDATE_NOW) || (n==PWM_MATCH_UPDATE_NEXT_RST)) 00274 00275 00276 /** @brief PWM interrupt status type definition ----------------------------------------------------- */ 00277 /** @brief PWM Interrupt status type */ 00278 typedef enum 00279 { 00280 PWM_INTSTAT_MR0 = PWM_IR_PWMMRn(0), /**< Interrupt flag for PWM match channel 0 */ 00281 PWM_INTSTAT_MR1 = PWM_IR_PWMMRn(1), /**< Interrupt flag for PWM match channel 1 */ 00282 PWM_INTSTAT_MR2 = PWM_IR_PWMMRn(2), /**< Interrupt flag for PWM match channel 2 */ 00283 PWM_INTSTAT_MR3 = PWM_IR_PWMMRn(3), /**< Interrupt flag for PWM match channel 3 */ 00284 PWM_INTSTAT_CAP0 = PWM_IR_PWMCAPn(0), /**< Interrupt flag for capture input 0 */ 00285 PWM_INTSTAT_CAP1 = PWM_IR_PWMCAPn(1), /**< Interrupt flag for capture input 1 */ 00286 PWM_INTSTAT_MR4 = PWM_IR_PWMMRn(4), /**< Interrupt flag for PWM match channel 4 */ 00287 PWM_INTSTAT_MR6 = PWM_IR_PWMMRn(5), /**< Interrupt flag for PWM match channel 5 */ 00288 PWM_INTSTAT_MR5 = PWM_IR_PWMMRn(6), /**< Interrupt flag for PWM match channel 6 */ 00289 }PWM_INTSTAT_TYPE; 00290 00291 #define PARAM_PWM_INTSTAT(n) ((n==PWM_INTSTAT_MR0) || (n==PWM_INTSTAT_MR1) || (n==PWM_INTSTAT_MR2) \ 00292 || (n==PWM_INTSTAT_MR3) || (n==PWM_INTSTAT_MR4) || (n==PWM_INTSTAT_MR5) \ 00293 || (n==PWM_INTSTAT_MR6) || (n==PWM_INTSTAT_CAP0) || (n==PWM_INTSTAT_CAP1)) 00294 00295 /** 00296 * @} 00297 */ 00298 00299 00300 /* Public Functions ----------------------------------------------------------- */ 00301 /** @defgroup PWM_Public_Functions 00302 * @{ 00303 */ 00304 00305 void PWM_PinConfig(LPC_PWM_TypeDef *PWMx, uint8_t PWM_Channel, uint8_t PinselOption); 00306 IntStatus PWM_GetIntStatus(LPC_PWM_TypeDef *PWMx, uint32_t IntFlag); 00307 void PWM_ClearIntPending(LPC_PWM_TypeDef *PWMx, uint32_t IntFlag); 00308 void PWM_ConfigStructInit(uint8_t PWMTimerCounterMode, void *PWM_InitStruct); 00309 void PWM_Init(LPC_PWM_TypeDef *PWMx, uint32_t PWMTimerCounterMode, void *PWM_ConfigStruct); 00310 void PWM_DeInit (LPC_PWM_TypeDef *PWMx); 00311 void PWM_Cmd(LPC_PWM_TypeDef *PWMx, FunctionalState NewState); 00312 void PWM_CounterCmd(LPC_PWM_TypeDef *PWMx, FunctionalState NewState); 00313 void PWM_ResetCounter(LPC_PWM_TypeDef *PWMx); 00314 void PWM_ConfigMatch(LPC_PWM_TypeDef *PWMx, PWM_MATCHCFG_Type *PWM_MatchConfigStruct); 00315 void PWM_ConfigCapture(LPC_PWM_TypeDef *PWMx, PWM_CAPTURECFG_Type *PWM_CaptureConfigStruct); 00316 uint32_t PWM_GetCaptureValue(LPC_PWM_TypeDef *PWMx, uint8_t CaptureChannel); 00317 void PWM_MatchUpdate(LPC_PWM_TypeDef *PWMx, uint8_t MatchChannel, \ 00318 uint32_t MatchValue, uint8_t UpdateType); 00319 void PWM_ChannelConfig(LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, uint8_t ModeOption); 00320 void PWM_ChannelCmd(LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, FunctionalState NewState); 00321 00322 /** 00323 * @} 00324 */ 00325 00326 #ifdef __cplusplus 00327 } 00328 #endif 00329 00330 #endif /* LPC17XX_PWM_H_ */ 00331 00332 /** 00333 * @} 00334 */ 00335 00336 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
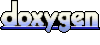