
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_i2c.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_i2c.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for I2C firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 13. Apr. 2009 00007 * @author : HieuNguyen 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup I2C 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_I2C_H_ 00028 #define LPC17XX_I2C_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Private Macros ------------------------------------------------------------- */ 00042 /** @defgroup I2C_Private_Macros 00043 * @{ 00044 */ 00045 00046 /** @defgroup I2C_REGISTER_BIT_DEFINITIONS 00047 * @{ 00048 */ 00049 00050 /*******************************************************************//** 00051 * I2C Control Set register description 00052 *********************************************************************/ 00053 #define I2C_I2CONSET_AA ((0x04)) /*!< Assert acknowledge flag */ 00054 #define I2C_I2CONSET_SI ((0x08)) /*!< I2C interrupt flag */ 00055 #define I2C_I2CONSET_STO ((0x10)) /*!< STOP flag */ 00056 #define I2C_I2CONSET_STA ((0x20)) /*!< START flag */ 00057 #define I2C_I2CONSET_I2EN ((0x40)) /*!< I2C interface enable */ 00058 00059 00060 /*******************************************************************//** 00061 * I2C Control Clear register description 00062 *********************************************************************/ 00063 /** Assert acknowledge Clear bit */ 00064 #define I2C_I2CONCLR_AAC ((1<<2)) 00065 /** I2C interrupt Clear bit */ 00066 #define I2C_I2CONCLR_SIC ((1<<3)) 00067 /** START flag Clear bit */ 00068 #define I2C_I2CONCLR_STAC ((1<<5)) 00069 /** I2C interface Disable bit */ 00070 #define I2C_I2CONCLR_I2ENC ((1<<6)) 00071 00072 00073 /********************************************************************//** 00074 * I2C Status Code definition (I2C Status register) 00075 *********************************************************************/ 00076 /* Return Code in I2C status register */ 00077 #define I2C_STAT_CODE_BITMASK ((0xF8)) 00078 00079 /* I2C return status code definitions ----------------------------- */ 00080 00081 /** No relevant information */ 00082 #define I2C_I2STAT_NO_INF ((0xF8)) 00083 00084 /* Master transmit mode -------------------------------------------- */ 00085 /** A start condition has been transmitted */ 00086 #define I2C_I2STAT_M_TX_START ((0x08)) 00087 /** A repeat start condition has been transmitted */ 00088 #define I2C_I2STAT_M_TX_RESTART ((0x10)) 00089 /** SLA+W has been transmitted, ACK has been received */ 00090 #define I2C_I2STAT_M_TX_SLAW_ACK ((0x18)) 00091 /** SLA+W has been transmitted, NACK has been received */ 00092 #define I2C_I2STAT_M_TX_SLAW_NACK ((0x20)) 00093 /** Data has been transmitted, ACK has been received */ 00094 #define I2C_I2STAT_M_TX_DAT_ACK ((0x28)) 00095 /** Data has been transmitted, NACK has been received */ 00096 #define I2C_I2STAT_M_TX_DAT_NACK ((0x30)) 00097 /** Arbitration lost in SLA+R/W or Data bytes */ 00098 #define I2C_I2STAT_M_TX_ARB_LOST ((0x38)) 00099 00100 /* Master receive mode -------------------------------------------- */ 00101 /** A start condition has been transmitted */ 00102 #define I2C_I2STAT_M_RX_START ((0x08)) 00103 /** A repeat start condition has been transmitted */ 00104 #define I2C_I2STAT_M_RX_RESTART ((0x10)) 00105 /** Arbitration lost */ 00106 #define I2C_I2STAT_M_RX_ARB_LOST ((0x38)) 00107 /** SLA+R has been transmitted, ACK has been received */ 00108 #define I2C_I2STAT_M_RX_SLAR_ACK ((0x40)) 00109 /** SLA+R has been transmitted, NACK has been received */ 00110 #define I2C_I2STAT_M_RX_SLAR_NACK ((0x48)) 00111 /** Data has been received, ACK has been returned */ 00112 #define I2C_I2STAT_M_RX_DAT_ACK ((0x50)) 00113 /** Data has been received, NACK has been return */ 00114 #define I2C_I2STAT_M_RX_DAT_NACK ((0x58)) 00115 00116 /* Slave receive mode -------------------------------------------- */ 00117 /** Own slave address has been received, ACK has been returned */ 00118 #define I2C_I2STAT_S_RX_SLAW_ACK ((0x60)) 00119 00120 /** Arbitration lost in SLA+R/W as master */ 00121 #define I2C_I2STAT_S_RX_ARB_LOST_M_SLA ((0x68)) 00122 /** Own SLA+W has been received, ACK returned */ 00123 //#define I2C_I2STAT_S_RX_SLAW_ACK ((0x68)) 00124 00125 /** General call address has been received, ACK has been returned */ 00126 #define I2C_I2STAT_S_RX_GENCALL_ACK ((0x70)) 00127 00128 /** Arbitration lost in SLA+R/W (GENERAL CALL) as master */ 00129 #define I2C_I2STAT_S_RX_ARB_LOST_M_GENCALL ((0x78)) 00130 /** General call address has been received, ACK has been returned */ 00131 //#define I2C_I2STAT_S_RX_GENCALL_ACK ((0x78)) 00132 00133 /** Previously addressed with own SLV address; 00134 * Data has been received, ACK has been return */ 00135 #define I2C_I2STAT_S_RX_PRE_SLA_DAT_ACK ((0x80)) 00136 /** Previously addressed with own SLA; 00137 * Data has been received and NOT ACK has been return */ 00138 #define I2C_I2STAT_S_RX_PRE_SLA_DAT_NACK ((0x88)) 00139 /** Previously addressed with General Call; 00140 * Data has been received and ACK has been return */ 00141 #define I2C_I2STAT_S_RX_PRE_GENCALL_DAT_ACK ((0x90)) 00142 /** Previously addressed with General Call; 00143 * Data has been received and NOT ACK has been return */ 00144 #define I2C_I2STAT_S_RX_PRE_GENCALL_DAT_NACK ((0x98)) 00145 /** A STOP condition or repeated START condition has 00146 * been received while still addressed as SLV/REC 00147 * (Slave Receive) or SLV/TRX (Slave Transmit) */ 00148 #define I2C_I2STAT_S_RX_STA_STO_SLVREC_SLVTRX ((0xA0)) 00149 00150 /** Slave transmit mode */ 00151 /** Own SLA+R has been received, ACK has been returned */ 00152 #define I2C_I2STAT_S_TX_SLAR_ACK ((0xA8)) 00153 00154 /** Arbitration lost in SLA+R/W as master */ 00155 #define I2C_I2STAT_S_TX_ARB_LOST_M_SLA ((0xB0)) 00156 /** Own SLA+R has been received, ACK has been returned */ 00157 //#define I2C_I2STAT_S_TX_SLAR_ACK ((0xB0)) 00158 00159 /** Data has been transmitted, ACK has been received */ 00160 #define I2C_I2STAT_S_TX_DAT_ACK ((0xB8)) 00161 /** Data has been transmitted, NACK has been received */ 00162 #define I2C_I2STAT_S_TX_DAT_NACK ((0xC0)) 00163 /** Last data byte in I2DAT has been transmitted (AA = 0); 00164 ACK has been received */ 00165 #define I2C_I2STAT_S_TX_LAST_DAT_ACK ((0xC8)) 00166 00167 /** Time out in case of using I2C slave mode */ 00168 #define I2C_SLAVE_TIME_OUT 0x10000UL 00169 00170 /********************************************************************//** 00171 * I2C Data register definition 00172 *********************************************************************/ 00173 /** Mask for I2DAT register*/ 00174 #define I2C_I2DAT_BITMASK ((0xFF)) 00175 00176 /** Idle data value will be send out in slave mode in case of the actual 00177 * expecting data requested from the master is greater than its sending data 00178 * length that can be supported */ 00179 #define I2C_I2DAT_IDLE_CHAR (0xFF) 00180 00181 00182 /********************************************************************//** 00183 * I2C Monitor mode control register description 00184 *********************************************************************/ 00185 #define I2C_I2MMCTRL_MM_ENA ((1<<0)) /**< Monitor mode enable */ 00186 #define I2C_I2MMCTRL_ENA_SCL ((1<<1)) /**< SCL output enable */ 00187 #define I2C_I2MMCTRL_MATCH_ALL ((1<<2)) /**< Select interrupt register match */ 00188 #define I2C_I2MMCTRL_BITMASK ((0x07)) /**< Mask for I2MMCTRL register */ 00189 00190 00191 /********************************************************************//** 00192 * I2C Data buffer register description 00193 *********************************************************************/ 00194 /** I2C Data buffer register bit mask */ 00195 #define I2DATA_BUFFER_BITMASK ((0xFF)) 00196 00197 00198 /********************************************************************//** 00199 * I2C Slave Address registers definition 00200 *********************************************************************/ 00201 /** General Call enable bit */ 00202 #define I2C_I2ADR_GC ((1<<0)) 00203 /** I2C Slave Address registers bit mask */ 00204 #define I2C_I2ADR_BITMASK ((0xFF)) 00205 00206 00207 /********************************************************************//** 00208 * I2C Mask Register definition 00209 *********************************************************************/ 00210 /** I2C Mask Register mask field */ 00211 #define I2C_I2MASK_MASK(n) ((n&0xFE)) 00212 00213 00214 /********************************************************************//** 00215 * I2C SCL HIGH duty cycle Register definition 00216 *********************************************************************/ 00217 /** I2C SCL HIGH duty cycle Register bit mask */ 00218 #define I2C_I2SCLH_BITMASK ((0xFFFF)) 00219 00220 00221 /********************************************************************//** 00222 * I2C SCL LOW duty cycle Register definition 00223 *********************************************************************/ 00224 /** I2C SCL LOW duty cycle Register bit mask */ 00225 #define I2C_I2SCLL_BITMASK ((0xFFFF)) 00226 00227 /** 00228 * @} 00229 */ 00230 00231 /** 00232 * @} 00233 */ 00234 00235 00236 00237 /* Public Types --------------------------------------------------------------- */ 00238 /** @defgroup I2C_Public_Types 00239 * @{ 00240 */ 00241 00242 /** @brief I2C Own slave address setting structure */ 00243 typedef struct { 00244 uint8_t SlaveAddrChannel; /**< Slave Address channel in I2C control, 00245 should be in range from 0..3 00246 */ 00247 uint8_t SlaveAddr_7bit; /**< Value of 7-bit slave address */ 00248 uint8_t GeneralCallState; /**< Enable/Disable General Call Functionality 00249 when I2C control being in Slave mode, should be: 00250 - ENABLE: Enable General Call function. 00251 - DISABLE: Disable General Call function. 00252 */ 00253 uint8_t SlaveAddrMaskValue; /**< Any bit in this 8-bit value (bit 7:1) 00254 which is set to '1' will cause an automatic compare on 00255 the corresponding bit of the received address when it 00256 is compared to the SlaveAddr_7bit value associated with this 00257 mask register. In other words, bits in SlaveAddr_7bit value 00258 which are masked are not taken into account in determining 00259 an address match 00260 */ 00261 } I2C_OWNSLAVEADDR_CFG_Type; 00262 00263 00264 /** @brief Master transfer setup data structure definitions */ 00265 typedef struct 00266 { 00267 uint32_t sl_addr7bit; /**< Slave address in 7bit mode */ 00268 uint8_t* tx_data; /**< Pointer to Transmit data - NULL if data transmit 00269 is not used */ 00270 uint32_t tx_length; /**< Transmit data length - 0 if data transmit 00271 is not used*/ 00272 uint32_t tx_count; /**< Current Transmit data counter */ 00273 uint8_t* rx_data; /**< Pointer to Receive data - NULL if data receive 00274 is not used */ 00275 uint32_t rx_length; /**< Receive data length - 0 if data receive is 00276 not used */ 00277 uint32_t rx_count; /**< Current Receive data counter */ 00278 uint32_t retransmissions_max; /**< Max Re-Transmission value */ 00279 uint32_t retransmissions_count; /**< Current Re-Transmission counter */ 00280 uint32_t status; /**< Current status of I2C activity */ 00281 void (*callback)(void); /**< Pointer to Call back function when transmission complete 00282 used in interrupt transfer mode */ 00283 } I2C_M_SETUP_Type; 00284 00285 00286 /** @brief Slave transfer setup data structure definitions */ 00287 typedef struct 00288 { 00289 uint8_t* tx_data; 00290 uint32_t tx_length; 00291 uint32_t tx_count; 00292 uint8_t* rx_data; 00293 uint32_t rx_length; 00294 uint32_t rx_count; 00295 uint32_t status; 00296 void (*callback)(void); 00297 } I2C_S_SETUP_Type; 00298 00299 /** 00300 * @brief Transfer option type definitions 00301 */ 00302 typedef enum { 00303 I2C_TRANSFER_POLLING = 0, /**< Transfer in polling mode */ 00304 I2C_TRANSFER_INTERRUPT /**< Transfer in interrupt mode */ 00305 } I2C_TRANSFER_OPT_Type; 00306 00307 00308 /** 00309 * @} 00310 */ 00311 00312 00313 /* Public Macros -------------------------------------------------------------- */ 00314 /** @defgroup I2C_Public_Macros 00315 * @{ 00316 */ 00317 00318 #define PARAM_I2C_SLAVEADDR_CH(n) ((n<=3)) 00319 00320 /** Macro to determine if it is valid SSP port number */ 00321 #define PARAM_I2Cx(n) ((((uint32_t *)n)==((uint32_t *)LPC_I2C0)) \ 00322 || (((uint32_t *)n)==((uint32_t *)LPC_I2C1)) \ 00323 || (((uint32_t *)n)==((uint32_t *)LPC_I2C2))) 00324 00325 /* I2C status values */ 00326 #define I2C_SETUP_STATUS_ARBF (1<<8) /**< Arbitration false */ 00327 #define I2C_SETUP_STATUS_NOACKF (1<<9) /**< No ACK returned */ 00328 #define I2C_SETUP_STATUS_DONE (1<<10) /**< Status DONE */ 00329 00330 00331 /*********************************************************************//** 00332 * I2C monitor control configuration defines 00333 **********************************************************************/ 00334 #define I2C_MONITOR_CFG_SCL_OUTPUT I2C_I2MMCTRL_ENA_SCL /**< SCL output enable */ 00335 #define I2C_MONITOR_CFG_MATCHALL I2C_I2MMCTRL_MATCH_ALL /**< Select interrupt register match */ 00336 00337 #define PARAM_I2C_MONITOR_CFG(n) ((n==I2C_MONITOR_CFG_SCL_OUTPUT) || (I2C_MONITOR_CFG_MATCHALL)) 00338 00339 /** 00340 * @} 00341 */ 00342 00343 00344 /* Public Functions ----------------------------------------------------------- */ 00345 /** @defgroup I2C_Public_Functions 00346 * @{ 00347 */ 00348 00349 void I2C_SetClock (LPC_I2C_TypeDef *I2Cx, uint32_t target_clock); 00350 void I2C_DeInit(LPC_I2C_TypeDef* I2Cx); 00351 void I2C_Init(LPC_I2C_TypeDef *I2Cx, uint32_t clockrate); 00352 void I2C_Cmd(LPC_I2C_TypeDef* I2Cx, FunctionalState NewState); 00353 00354 Status I2C_MasterTransferData(LPC_I2C_TypeDef *I2Cx, \ 00355 I2C_M_SETUP_Type *TransferCfg, I2C_TRANSFER_OPT_Type Opt); 00356 Status I2C_SlaveTransferData(LPC_I2C_TypeDef *I2Cx, \ 00357 I2C_S_SETUP_Type *TransferCfg, I2C_TRANSFER_OPT_Type Opt); 00358 00359 void I2C_SetOwnSlaveAddr(LPC_I2C_TypeDef *I2Cx, I2C_OWNSLAVEADDR_CFG_Type *OwnSlaveAddrConfigStruct); 00360 uint8_t I2C_GetLastStatusCode(LPC_I2C_TypeDef* I2Cx); 00361 00362 void I2C_MonitorModeConfig(LPC_I2C_TypeDef *I2Cx, uint32_t MonitorCfgType, FunctionalState NewState); 00363 void I2C_MonitorModeCmd(LPC_I2C_TypeDef *I2Cx, FunctionalState NewState); 00364 uint8_t I2C_MonitorGetDatabuffer(LPC_I2C_TypeDef *I2Cx); 00365 00366 void I2C0_StdIntHandler(void); 00367 void I2C1_StdIntHandler(void); 00368 void I2C2_StdIntHandler(void); 00369 00370 00371 /** 00372 * @} 00373 */ 00374 00375 00376 #ifdef __cplusplus 00377 } 00378 #endif 00379 00380 #endif /* LPC17XX_I2C_H_ */ 00381 00382 /** 00383 * @} 00384 */ 00385 00386 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
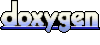