
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
Functions | |
void | I2C_SetClock (LPC_I2C_TypeDef *I2Cx, uint32_t target_clock) |
Setup clock rate for I2C peripheral. | |
void | I2C_DeInit (LPC_I2C_TypeDef *I2Cx) |
De-initializes the I2C peripheral registers to their default reset values. | |
void | I2C_Init (LPC_I2C_TypeDef *I2Cx, uint32_t clockrate) |
Initializes the I2Cx peripheral with specified parameter. | |
void | I2C_Cmd (LPC_I2C_TypeDef *I2Cx, FunctionalState NewState) |
Enable or disable I2C peripheral's operation. | |
void | I2C_SetOwnSlaveAddr (LPC_I2C_TypeDef *I2Cx, I2C_OWNSLAVEADDR_CFG_Type *OwnSlaveAddrConfigStruct) |
Set Own slave address in I2C peripheral corresponding to parameter specified in OwnSlaveAddrConfigStruct. | |
void | I2C_MonitorModeConfig (LPC_I2C_TypeDef *I2Cx, uint32_t MonitorCfgType, FunctionalState NewState) |
Configures functionality in I2C monitor mode. | |
void | I2C_MonitorModeCmd (LPC_I2C_TypeDef *I2Cx, FunctionalState NewState) |
Enable/Disable I2C monitor mode. | |
uint8_t | I2C_MonitorGetDatabuffer (LPC_I2C_TypeDef *I2Cx) |
Get data from I2C data buffer in monitor mode. | |
void | I2C0_StdIntHandler (void) |
Standard Interrupt handler for I2C0 peripheral. | |
void | I2C1_StdIntHandler (void) |
Standard Interrupt handler for I2C1 peripheral. | |
void | I2C2_StdIntHandler (void) |
Standard Interrupt handler for I2C2 peripheral. | |
Status | I2C_MasterTransferData (LPC_I2C_TypeDef *I2Cx, I2C_M_SETUP_Type *TransferCfg,\I2C_TRANSFER_OPT_Type Opt) |
Transmit and Receive data in master mode. | |
Status | I2C_SlaveTransferData (LPC_I2C_TypeDef *I2Cx, I2C_S_SETUP_Type *TransferCfg,\I2C_TRANSFER_OPT_Type Opt) |
Receive and Transmit data in slave mode. |
Function Documentation
void I2C0_StdIntHandler | ( | void | ) |
Standard Interrupt handler for I2C0 peripheral.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 1271 of file lpc17xx_i2c.c.
void I2C1_StdIntHandler | ( | void | ) |
Standard Interrupt handler for I2C1 peripheral.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 1281 of file lpc17xx_i2c.c.
void I2C2_StdIntHandler | ( | void | ) |
Standard Interrupt handler for I2C2 peripheral.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 1291 of file lpc17xx_i2c.c.
void I2C_Cmd | ( | LPC_I2C_TypeDef * | I2Cx, |
FunctionalState | NewState | ||
) |
Enable or disable I2C peripheral's operation.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] NewState New State of I2Cx peripheral's operation
- Returns:
- none
Definition at line 723 of file lpc17xx_i2c.c.
void I2C_DeInit | ( | LPC_I2C_TypeDef * | I2Cx ) |
De-initializes the I2C peripheral registers to their default reset values.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2
- Returns:
- None
Definition at line 645 of file lpc17xx_i2c.c.
void I2C_Init | ( | LPC_I2C_TypeDef * | I2Cx, |
uint32_t | clockrate | ||
) |
Initializes the I2Cx peripheral with specified parameter.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] clockrate Target clock rate value to initialized I2C peripheral
- Returns:
- None
Definition at line 677 of file lpc17xx_i2c.c.
Status I2C_MasterTransferData | ( | LPC_I2C_TypeDef * | I2Cx, |
I2C_M_SETUP_Type * | TransferCfg, | ||
\I2C_TRANSFER_OPT_Type | Opt | ||
) |
Transmit and Receive data in master mode.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] TransferCfg Pointer to a I2C_M_SETUP_Type structure that contains specified information about the configuration for master transfer. [in] Opt a I2C_TRANSFER_OPT_Type type that selected for interrupt or polling mode.
- Returns:
- SUCCESS or ERROR
Note:
- In case of using I2C to transmit data only, either transmit length set to 0 or transmit data pointer set to NULL.
- In case of using I2C to receive data only, either receive length set to 0 or receive data pointer set to NULL.
- In case of using I2C to transmit followed by receive data, transmit length, transmit data pointer, receive length and receive data pointer should be set corresponding.
Definition at line 758 of file lpc17xx_i2c.c.
uint8_t I2C_MonitorGetDatabuffer | ( | LPC_I2C_TypeDef * | I2Cx ) |
Get data from I2C data buffer in monitor mode.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2
- Returns:
- None Note: In monitor mode, the I2C module may lose the ability to stretch the clock (stall the bus) if the ENA_SCL bit is not set. This means that the processor will have a limited amount of time to read the contents of the data received on the bus. If the processor reads the I2DAT shift register, as it ordinarily would, it could have only one bit-time to respond to the interrupt before the received data is overwritten by new data.
Definition at line 1260 of file lpc17xx_i2c.c.
void I2C_MonitorModeCmd | ( | LPC_I2C_TypeDef * | I2Cx, |
FunctionalState | NewState | ||
) |
Enable/Disable I2C monitor mode.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] NewState New State of this function, should be: - ENABLE: Enable monitor mode.
- DISABLE: Disable monitor mode.
- Returns:
- None
Definition at line 1232 of file lpc17xx_i2c.c.
void I2C_MonitorModeConfig | ( | LPC_I2C_TypeDef * | I2Cx, |
uint32_t | MonitorCfgType, | ||
FunctionalState | NewState | ||
) |
Configures functionality in I2C monitor mode.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] MonitorCfgType Monitor Configuration type, should be: - I2C_MONITOR_CFG_SCL_OUTPUT: I2C module can 'stretch' the clock line (hold it low) until it has had time to respond to an I2C interrupt.
- I2C_MONITOR_CFG_MATCHALL: When this bit is set to '1' and the I2C is in monitor mode, an interrupt will be generated on ANY address received.
[in] NewState New State of this function, should be: - ENABLE: Enable this function.
- DISABLE: Disable this function.
- Returns:
- None
Definition at line 1207 of file lpc17xx_i2c.c.
void I2C_SetClock | ( | LPC_I2C_TypeDef * | I2Cx, |
uint32_t | target_clock | ||
) |
Setup clock rate for I2C peripheral.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] target_clock : clock of SSP (Hz)
- Returns:
- None
Definition at line 613 of file lpc17xx_i2c.c.
void I2C_SetOwnSlaveAddr | ( | LPC_I2C_TypeDef * | I2Cx, |
I2C_OWNSLAVEADDR_CFG_Type * | OwnSlaveAddrConfigStruct | ||
) |
Set Own slave address in I2C peripheral corresponding to parameter specified in OwnSlaveAddrConfigStruct.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] OwnSlaveAddrConfigStruct Pointer to a I2C_OWNSLAVEADDR_CFG_Type structure that contains the configuration information for the specified I2C slave address.
- Returns:
- None
Definition at line 1157 of file lpc17xx_i2c.c.
Status I2C_SlaveTransferData | ( | LPC_I2C_TypeDef * | I2Cx, |
I2C_S_SETUP_Type * | TransferCfg, | ||
\I2C_TRANSFER_OPT_Type | Opt | ||
) |
Receive and Transmit data in slave mode.
- Parameters:
-
[in] I2Cx I2C peripheral selected, should be I2C0, I2C1 or I2C2 [in] TransferCfg Pointer to a I2C_S_SETUP_Type structure that contains specified information about the configuration for master transfer. [in] Opt I2C_TRANSFER_OPT_Type type that selected for interrupt or polling mode.
- Returns:
- SUCCESS or ERROR
Note: The mode of slave's operation depends on the command sent from master on the I2C bus. If the master send a SLA+W command, this sub-routine will use receive data length and receive data pointer. If the master send a SLA+R command, this sub-routine will use transmit data length and transmit data pointer. If the master issue an repeat start command or a stop command, the slave will enable an time out condition, during time out condition, if there's no activity on I2C bus, the slave will exit, otherwise (i.e. the master send a SLA+R/W), the slave then switch to relevant operation mode. The time out should be used because the return status code can not show difference from stop and repeat start command in slave operation. In case of the expected data length from master is greater than data length that slave can support:
- In case of reading operation (from master): slave will return I2C_I2DAT_IDLE_CHAR value.
- In case of writing operation (from master): slave will ignore remain data from master.
Definition at line 968 of file lpc17xx_i2c.c.
Generated on Tue Jul 12 2022 17:06:02 by
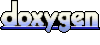