
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_gpio.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_gpio.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for GPIO firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 23. Apr. 2009 00007 * @author : HieuNguyen 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup GPIO 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_GPIO_H_ 00028 #define LPC17XX_GPIO_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Public Types --------------------------------------------------------------- */ 00042 /** @defgroup GPIO_Public_Types 00043 * @{ 00044 */ 00045 00046 /** 00047 * @brief Fast GPIO port byte type definition 00048 */ 00049 typedef struct { 00050 __IO uint8_t FIODIR[4]; /**< FIO direction register in byte-align */ 00051 uint32_t RESERVED0[3]; /**< Reserved */ 00052 __IO uint8_t FIOMASK[4]; /**< FIO mask register in byte-align */ 00053 __IO uint8_t FIOPIN[4]; /**< FIO pin register in byte align */ 00054 __IO uint8_t FIOSET[4]; /**< FIO set register in byte-align */ 00055 __O uint8_t FIOCLR[4]; /**< FIO clear register in byte-align */ 00056 } GPIO_Byte_TypeDef; 00057 00058 00059 /** 00060 * @brief Fast GPIO port half-word type definition 00061 */ 00062 typedef struct { 00063 __IO uint16_t FIODIRL; /**< FIO direction register lower halfword part */ 00064 __IO uint16_t FIODIRU; /**< FIO direction register upper halfword part */ 00065 uint32_t RESERVED0[3]; /**< Reserved */ 00066 __IO uint16_t FIOMASKL; /**< FIO mask register lower halfword part */ 00067 __IO uint16_t FIOMASKU; /**< FIO mask register upper halfword part */ 00068 __IO uint16_t FIOPINL; /**< FIO pin register lower halfword part */ 00069 __IO uint16_t FIOPINU; /**< FIO pin register upper halfword part */ 00070 __IO uint16_t FIOSETL; /**< FIO set register lower halfword part */ 00071 __IO uint16_t FIOSETU; /**< FIO set register upper halfword part */ 00072 __O uint16_t FIOCLRL; /**< FIO clear register lower halfword part */ 00073 __O uint16_t FIOCLRU; /**< FIO clear register upper halfword part */ 00074 } GPIO_HalfWord_TypeDef; 00075 00076 00077 /** 00078 * @} 00079 */ 00080 00081 00082 /* Public Macros -------------------------------------------------------------- */ 00083 /** @defgroup GPIO_Public_Macros 00084 * @{ 00085 */ 00086 00087 /** Fast GPIO port 0 byte accessible definition */ 00088 #define GPIO0_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO0_BASE)) 00089 /** Fast GPIO port 1 byte accessible definition */ 00090 #define GPIO1_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO1_BASE)) 00091 /** Fast GPIO port 2 byte accessible definition */ 00092 #define GPIO2_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO2_BASE)) 00093 /** Fast GPIO port 3 byte accessible definition */ 00094 #define GPIO3_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO3_BASE)) 00095 /** Fast GPIO port 4 byte accessible definition */ 00096 #define GPIO4_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO4_BASE)) 00097 00098 00099 00100 /** Fast GPIO port 0 half-word accessible definition */ 00101 #define GPIO0_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO0_BASE)) 00102 /** Fast GPIO port 1 half-word accessible definition */ 00103 #define GPIO1_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO1_BASE)) 00104 /** Fast GPIO port 2 half-word accessible definition */ 00105 #define GPIO2_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO2_BASE)) 00106 /** Fast GPIO port 3 half-word accessible definition */ 00107 #define GPIO3_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO3_BASE)) 00108 /** Fast GPIO port 4 half-word accessible definition */ 00109 #define GPIO4_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO4_BASE)) 00110 00111 00112 /** 00113 * @} 00114 */ 00115 00116 00117 /* Public Functions ----------------------------------------------------------- */ 00118 /** @defgroup GPIO_Public_Functions 00119 * @{ 00120 */ 00121 00122 /* GPIO style ------------------------------- */ 00123 void GPIO_SetDir(uint8_t portNum, uint32_t bitValue, uint8_t dir); 00124 void GPIO_SetValue(uint8_t portNum, uint32_t bitValue); 00125 void GPIO_ClearValue(uint8_t portNum, uint32_t bitValue); 00126 uint32_t GPIO_ReadValue(uint8_t portNum); 00127 00128 /* FIO (word-accessible) style ------------------------------- */ 00129 void FIO_SetDir(uint8_t portNum, uint32_t bitValue, uint8_t dir); 00130 void FIO_SetValue(uint8_t portNum, uint32_t bitValue); 00131 void FIO_ClearValue(uint8_t portNum, uint32_t bitValue); 00132 uint32_t FIO_ReadValue(uint8_t portNum); 00133 void FIO_SetMask(uint8_t portNum, uint32_t bitValue, uint8_t maskValue); 00134 00135 /* FIO (halfword-accessible) style ------------------------------- */ 00136 void FIO_HalfWordSetDir(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t dir); 00137 void FIO_HalfWordSetMask(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t maskValue); 00138 void FIO_HalfWordSetValue(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue); 00139 void FIO_HalfWordClearValue(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue); 00140 uint16_t FIO_HalfWordReadValue(uint8_t portNum, uint8_t halfwordNum); 00141 00142 /* FIO (byte-accessible) style ------------------------------- */ 00143 void FIO_ByteSetDir(uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t dir); 00144 void FIO_ByteSetMask(uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t maskValue); 00145 void FIO_ByteSetValue(uint8_t portNum, uint8_t byteNum, uint8_t bitValue); 00146 void FIO_ByteClearValue(uint8_t portNum, uint8_t byteNum, uint8_t bitValue); 00147 uint8_t FIO_ByteReadValue(uint8_t portNum, uint8_t byteNum); 00148 00149 00150 00151 /** 00152 * @} 00153 */ 00154 00155 00156 #ifdef __cplusplus 00157 } 00158 #endif 00159 00160 #endif /* LPC17XX_GPIO_H_ */ 00161 00162 /** 00163 * @} 00164 */ 00165 00166 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
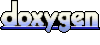