
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
Functions | |
void | GPIO_SetDir (uint8_t portNum, uint32_t bitValue, uint8_t dir) |
Set Direction for GPIO port. | |
void | GPIO_SetValue (uint8_t portNum, uint32_t bitValue) |
Set Value for bits that have output direction on GPIO port. | |
void | GPIO_ClearValue (uint8_t portNum, uint32_t bitValue) |
Clear Value for bits that have output direction on GPIO port. | |
uint32_t | GPIO_ReadValue (uint8_t portNum) |
Read Current state on port pin that have input direction of GPIO. | |
void | FIO_SetDir (uint8_t portNum, uint32_t bitValue, uint8_t dir) |
The same with GPIO_SetDir() | |
void | FIO_SetValue (uint8_t portNum, uint32_t bitValue) |
The same with GPIO_SetValue() | |
void | FIO_ClearValue (uint8_t portNum, uint32_t bitValue) |
The same with GPIO_ClearValue() | |
uint32_t | FIO_ReadValue (uint8_t portNum) |
The same with GPIO_ReadValue() | |
void | FIO_SetMask (uint8_t portNum, uint32_t bitValue, uint8_t maskValue) |
Set mask value for bits in FIO port. | |
void | FIO_HalfWordSetDir (uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t dir) |
Set direction for FIO port in halfword accessible style. | |
void | FIO_HalfWordSetMask (uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t maskValue) |
Set mask value for bits in FIO port in halfword accessible style. | |
void | FIO_HalfWordSetValue (uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue) |
Set bits for FIO port in halfword accessible style. | |
void | FIO_HalfWordClearValue (uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue) |
Clear bits for FIO port in halfword accessible style. | |
uint16_t | FIO_HalfWordReadValue (uint8_t portNum, uint8_t halfwordNum) |
Read Current state on port pin that have input direction of GPIO in halfword accessible style. | |
void | FIO_ByteSetDir (uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t dir) |
Set direction for FIO port in byte accessible style. | |
void | FIO_ByteSetMask (uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t maskValue) |
Set mask value for bits in FIO port in byte accessible style. | |
void | FIO_ByteSetValue (uint8_t portNum, uint8_t byteNum, uint8_t bitValue) |
Set bits for FIO port in byte accessible style. | |
void | FIO_ByteClearValue (uint8_t portNum, uint8_t byteNum, uint8_t bitValue) |
Clear bits for FIO port in byte accessible style. | |
uint8_t | FIO_ByteReadValue (uint8_t portNum, uint8_t byteNum) |
Read Current state on port pin that have input direction of GPIO in byte accessible style. |
Function Documentation
void FIO_ByteClearValue | ( | uint8_t | portNum, |
uint8_t | byteNum, | ||
uint8_t | bitValue | ||
) |
Clear bits for FIO port in byte accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] byteNum Byte part number, should be in range from 0 to 3 [in] bitValue Value that contains all bits in to clear, in range from 0 to 0xFF.
- Returns:
- None
Note:
- For all bits that has been set as input direction, this function will not effect.
- For all remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 616 of file lpc17xx_gpio.c.
uint8_t FIO_ByteReadValue | ( | uint8_t | portNum, |
uint8_t | byteNum | ||
) |
Read Current state on port pin that have input direction of GPIO in byte accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] byteNum Byte part number, should be in range from 0 to 3
- Returns:
- Current value of FIO port pin of specified byte part. Note: Return value contain state of each port pin (bit) on that FIO regardless its direction is input or output.
Definition at line 636 of file lpc17xx_gpio.c.
void FIO_ByteSetDir | ( | uint8_t | portNum, |
uint8_t | byteNum, | ||
uint8_t | bitValue, | ||
uint8_t | dir | ||
) |
Set direction for FIO port in byte accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] byteNum Byte part number, should be in range from 0 to 3 [in] bitValue Value that contains all bits in to set direction, in range from 0 to 0xFF. [in] dir Direction value, should be: - 0: Input.
- 1: Output.
- Returns:
- None
Note: All remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 519 of file lpc17xx_gpio.c.
void FIO_ByteSetMask | ( | uint8_t | portNum, |
uint8_t | byteNum, | ||
uint8_t | bitValue, | ||
uint8_t | maskValue | ||
) |
Set mask value for bits in FIO port in byte accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] byteNum Byte part number, should be in range from 0 to 3 [in] bitValue Value that contains all bits in to set mask, in range from 0 to 0xFF. [in] maskValue Mask value contains state value for each bit: - 0: not mask.
- 1: mask.
- Returns:
- None
Note:
- All remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
- After executing this function, in mask register, value '0' on each bit enables an access to the corresponding physical pin via a read or write access, while value '1' on bit (masked) that corresponding pin will not be changed with write access and if read, will not be reflected in the updated pin.
Definition at line 557 of file lpc17xx_gpio.c.
void FIO_ByteSetValue | ( | uint8_t | portNum, |
uint8_t | byteNum, | ||
uint8_t | bitValue | ||
) |
Set bits for FIO port in byte accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] byteNum Byte part number, should be in range from 0 to 3 [in] bitValue Value that contains all bits in to set, in range from 0 to 0xFF.
- Returns:
- None
Note:
- For all bits that has been set as input direction, this function will not effect.
- For all remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 591 of file lpc17xx_gpio.c.
void FIO_ClearValue | ( | uint8_t | portNum, |
uint32_t | bitValue | ||
) |
The same with GPIO_ClearValue()
Definition at line 274 of file lpc17xx_gpio.c.
void FIO_HalfWordClearValue | ( | uint8_t | portNum, |
uint8_t | halfwordNum, | ||
uint16_t | bitValue | ||
) |
Clear bits for FIO port in halfword accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] halfwordNum HalfWord part number, should be 0 (lower) or 1(upper) [in] bitValue Value that contains all bits in to clear, in range from 0 to 0xFFFF.
- Returns:
- None
Note:
- For all bits that has been set as input direction, this function will not effect.
- For all remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 461 of file lpc17xx_gpio.c.
uint16_t FIO_HalfWordReadValue | ( | uint8_t | portNum, |
uint8_t | halfwordNum | ||
) |
Read Current state on port pin that have input direction of GPIO in halfword accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] halfwordNum HalfWord part number, should be 0 (lower) or 1(upper)
- Returns:
- Current value of FIO port pin of specified halfword. Note: Return value contain state of each port pin (bit) on that FIO regardless its direction is input or output.
Definition at line 486 of file lpc17xx_gpio.c.
void FIO_HalfWordSetDir | ( | uint8_t | portNum, |
uint8_t | halfwordNum, | ||
uint16_t | bitValue, | ||
uint8_t | dir | ||
) |
Set direction for FIO port in halfword accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] halfwordNum HalfWord part number, should be 0 (lower) or 1(upper) [in] bitValue Value that contains all bits in to set direction, in range from 0 to 0xFFFF. [in] dir Direction value, should be: - 0: Input.
- 1: Output.
- Returns:
- None
Note: All remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 338 of file lpc17xx_gpio.c.
void FIO_HalfWordSetMask | ( | uint8_t | portNum, |
uint8_t | halfwordNum, | ||
uint16_t | bitValue, | ||
uint8_t | maskValue | ||
) |
Set mask value for bits in FIO port in halfword accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] halfwordNum HalfWord part number, should be 0 (lower) or 1(upper) [in] bitValue Value that contains all bits in to set, in range from 0 to 0xFFFF. [in] maskValue Mask value contains state value for each bit: - 0: not mask.
- 1: mask.
- Returns:
- None
Note:
- All remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
- After executing this function, in mask register, value '0' on each bit enables an access to the corresponding physical pin via a read or write access, while value '1' on bit (masked) that corresponding pin will not be changed with write access and if read, will not be reflected in the updated pin.
Definition at line 387 of file lpc17xx_gpio.c.
void FIO_HalfWordSetValue | ( | uint8_t | portNum, |
uint8_t | halfwordNum, | ||
uint16_t | bitValue | ||
) |
Set bits for FIO port in halfword accessible style.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] halfwordNum HalfWord part number, should be 0 (lower) or 1(upper) [in] bitValue Value that contains all bits in to set, in range from 0 to 0xFFFF.
- Returns:
- None
Note:
- For all bits that has been set as input direction, this function will not effect.
- For all remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 431 of file lpc17xx_gpio.c.
uint32_t FIO_ReadValue | ( | uint8_t | portNum ) |
The same with GPIO_ReadValue()
Definition at line 282 of file lpc17xx_gpio.c.
void FIO_SetDir | ( | uint8_t | portNum, |
uint32_t | bitValue, | ||
uint8_t | dir | ||
) |
The same with GPIO_SetDir()
Definition at line 258 of file lpc17xx_gpio.c.
void FIO_SetMask | ( | uint8_t | portNum, |
uint32_t | bitValue, | ||
uint8_t | maskValue | ||
) |
Set mask value for bits in FIO port.
- Parameters:
-
[in] portNum Port number, in range from 0 to 4 [in] bitValue Value that contains all bits in to set, in range from 0 to 0xFFFFFFFF. [in] maskValue Mask value contains state value for each bit: - 0: not mask.
- 1: mask.
- Returns:
- None
Note:
- All remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
- After executing this function, in mask register, value '0' on each bit enables an access to the corresponding physical pin via a read or write access, while value '1' on bit (masked) that corresponding pin will not be changed with write access and if read, will not be reflected in the updated pin.
Definition at line 306 of file lpc17xx_gpio.c.
void FIO_SetValue | ( | uint8_t | portNum, |
uint32_t | bitValue | ||
) |
The same with GPIO_SetValue()
Definition at line 266 of file lpc17xx_gpio.c.
void GPIO_ClearValue | ( | uint8_t | portNum, |
uint32_t | bitValue | ||
) |
Clear Value for bits that have output direction on GPIO port.
- Parameters:
-
[in] portNum Port number value, should be in range from 0 to 4 [in] bitValue Value that contains all bits on GPIO to clear, in range from 0 to 0xFFFFFFFF. example: value 0x5 to clear bit 0 and bit 1.
- Returns:
- None
Note:
- For all bits that has been set as input direction, this function will not effect.
- For all remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 224 of file lpc17xx_gpio.c.
uint32_t GPIO_ReadValue | ( | uint8_t | portNum ) |
Read Current state on port pin that have input direction of GPIO.
- Parameters:
-
[in] portNum Port number to read value, in range from 0 to 4
- Returns:
- Current value of GPIO port.
Note: Return value contain state of each port pin (bit) on that GPIO regardless its direction is input or output.
Definition at line 241 of file lpc17xx_gpio.c.
void GPIO_SetDir | ( | uint8_t | portNum, |
uint32_t | bitValue, | ||
uint8_t | dir | ||
) |
Set Direction for GPIO port.
- Parameters:
-
[in] portNum Port Number value, should be in range from 0 to 4 [in] bitValue Value that contains all bits to set direction, in range from 0 to 0xFFFFFFFF. example: value 0x5 to set direction for bit 0 and bit 1. [in] dir Direction value, should be: - 0: Input.
- 1: Output.
- Returns:
- None
Note: All remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 170 of file lpc17xx_gpio.c.
void GPIO_SetValue | ( | uint8_t | portNum, |
uint32_t | bitValue | ||
) |
Set Value for bits that have output direction on GPIO port.
- Parameters:
-
[in] portNum Port number value, should be in range from 0 to 4 [in] bitValue Value that contains all bits on GPIO to set, in range from 0 to 0xFFFFFFFF. example: value 0x5 to set bit 0 and bit 1.
- Returns:
- None
Note:
- For all bits that has been set as input direction, this function will not effect.
- For all remaining bits that are not activated in bitValue (value '0') will not be effected by this function.
Definition at line 201 of file lpc17xx_gpio.c.
Generated on Tue Jul 12 2022 17:06:02 by
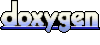