
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_can.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_can.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for CAN firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 1.June.2009 00007 * @author : NguyenCao 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup CAN 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_CAN_H_ 00028 #define LPC17XX_CAN_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 #ifdef __cplusplus 00035 extern "C" 00036 { 00037 #endif 00038 00039 00040 /* Private Macros ------------------------------------------------------------- */ 00041 /** @defgroup CAN_Private_Macros 00042 * @{ 00043 */ 00044 00045 #define ID_11 1 00046 #define MAX_HW_FULLCAN_OBJ 64 00047 #define MAX_SW_FULLCAN_OBJ 32 00048 00049 00050 /** @defgroup CAN_REGISTER_BIT_DEFINITION 00051 * @{ 00052 */ 00053 00054 /** CAN Reset mode */ 00055 #define CAN_MOD_RM ((uint32_t)(1)) 00056 /** CAN Listen Only Mode */ 00057 #define CAN_MOD_LOM ((uint32_t)(1<<1)) 00058 /** CAN Self Test mode */ 00059 #define CAN_MOD_STM ((uint32_t)(1<<2)) 00060 /** CAN Transmit Priority mode */ 00061 #define CAN_MOD_TPM ((uint32_t)(1<<3)) 00062 /** CAN Sleep mode */ 00063 #define CAN_MOD_SM ((uint32_t)(1<<4)) 00064 /** CAN Receive Polarity mode */ 00065 #define CAN_MOD_RPM ((uint32_t)(1<<5)) 00066 /** CAN Test mode */ 00067 #define CAN_MOD_TM ((uint32_t)(1<<7)) 00068 /*********************************************************************//** 00069 * Macro defines for CAN Command Register 00070 **********************************************************************/ 00071 /** CAN Transmission Request */ 00072 #define CAN_CMR_TR ((uint32_t)(1)) 00073 /** CAN Abort Transmission */ 00074 #define CAN_CMR_AT ((uint32_t)(1<<1)) 00075 /** CAN Release Receive Buffer */ 00076 #define CAN_CMR_RRB ((uint32_t)(1<<2)) 00077 /** CAN Clear Data Overrun */ 00078 #define CAN_CMR_CDO ((uint32_t)(1<<3)) 00079 /** CAN Self Reception Request */ 00080 #define CAN_CMR_SRR ((uint32_t)(1<<4)) 00081 /** CAN Select Tx Buffer 1 */ 00082 #define CAN_CMR_STB1 ((uint32_t)(1<<5)) 00083 /** CAN Select Tx Buffer 2 */ 00084 #define CAN_CMR_STB2 ((uint32_t)(1<<6)) 00085 /** CAN Select Tx Buffer 3 */ 00086 #define CAN_CMR_STB3 ((uint32_t)(1<<7)) 00087 00088 /*********************************************************************//** 00089 * Macro defines for CAN Global Status Register 00090 **********************************************************************/ 00091 /** CAN Receive Buffer Status */ 00092 #define CAN_GSR_RBS ((uint32_t)(1)) 00093 /** CAN Data Overrun Status */ 00094 #define CAN_GSR_DOS ((uint32_t)(1<<1)) 00095 /** CAN Transmit Buffer Status */ 00096 #define CAN_GSR_TBS ((uint32_t)(1<<2)) 00097 /** CAN Transmit Complete Status */ 00098 #define CAN_GSR_TCS ((uint32_t)(1<<3)) 00099 /** CAN Receive Status */ 00100 #define CAN_GSR_RS ((uint32_t)(1<<4)) 00101 /** CAN Transmit Status */ 00102 #define CAN_GSR_TS ((uint32_t)(1<<5)) 00103 /** CAN Error Status */ 00104 #define CAN_GSR_ES ((uint32_t)(1<<6)) 00105 /** CAN Bus Status */ 00106 #define CAN_GSR_BS ((uint32_t)(1<<7)) 00107 /** CAN Current value of the Rx Error Counter */ 00108 #define CAN_GSR_RXERR(n) ((uint32_t)((n&0xFF)<<16)) 00109 /** CAN Current value of the Tx Error Counter */ 00110 #define CAN_GSR_TXERR(n) ((uint32_t)(n&0xFF)<<24)) 00111 00112 /*********************************************************************//** 00113 * Macro defines for CAN Interrupt and Capture Register 00114 **********************************************************************/ 00115 /** CAN Receive Interrupt */ 00116 #define CAN_ICR_RI ((uint32_t)(1)) 00117 /** CAN Transmit Interrupt 1 */ 00118 #define CAN_ICR_TI1 ((uint32_t)(1<<1)) 00119 /** CAN Error Warning Interrupt */ 00120 #define CAN_ICR_EI ((uint32_t)(1<<2)) 00121 /** CAN Data Overrun Interrupt */ 00122 #define CAN_ICR_DOI ((uint32_t)(1<<3)) 00123 /** CAN Wake-Up Interrupt */ 00124 #define CAN_ICR_WUI ((uint32_t)(1<<4)) 00125 /** CAN Error Passive Interrupt */ 00126 #define CAN_ICR_EPI ((uint32_t)(1<<5)) 00127 /** CAN Arbitration Lost Interrupt */ 00128 #define CAN_ICR_ALI ((uint32_t)(1<<6)) 00129 /** CAN Bus Error Interrupt */ 00130 #define CAN_ICR_BEI ((uint32_t)(1<<7)) 00131 /** CAN ID Ready Interrupt */ 00132 #define CAN_ICR_IDI ((uint32_t)(1<<8)) 00133 /** CAN Transmit Interrupt 2 */ 00134 #define CAN_ICR_TI2 ((uint32_t)(1<<9)) 00135 /** CAN Transmit Interrupt 3 */ 00136 #define CAN_ICR_TI3 ((uint32_t)(1<<10)) 00137 /** CAN Error Code Capture */ 00138 #define CAN_ICR_ERRBIT(n) ((uint32_t)((n&0x1F)<<16)) 00139 /** CAN Error Direction */ 00140 #define CAN_ICR_ERRDIR ((uint32_t)(1<<21)) 00141 /** CAN Error Capture */ 00142 #define CAN_ICR_ERRC(n) ((uint32_t)((n&0x3)<<22)) 00143 /** CAN Arbitration Lost Capture */ 00144 #define CAN_ICR_ALCBIT(n) ((uint32_t)((n&0xFF)<<24)) 00145 00146 /*********************************************************************//** 00147 * Macro defines for CAN Interrupt Enable Register 00148 **********************************************************************/ 00149 /** CAN Receive Interrupt Enable */ 00150 #define CAN_IER_RIE ((uint32_t)(1)) 00151 /** CAN Transmit Interrupt Enable for buffer 1 */ 00152 #define CAN_IER_TIE1 ((uint32_t)(1<<1)) 00153 /** CAN Error Warning Interrupt Enable */ 00154 #define CAN_IER_EIE ((uint32_t)(1<<2)) 00155 /** CAN Data Overrun Interrupt Enable */ 00156 #define CAN_IER_DOIE ((uint32_t)(1<<3)) 00157 /** CAN Wake-Up Interrupt Enable */ 00158 #define CAN_IER_WUIE ((uint32_t)(1<<4)) 00159 /** CAN Error Passive Interrupt Enable */ 00160 #define CAN_IER_EPIE ((uint32_t)(1<<5)) 00161 /** CAN Arbitration Lost Interrupt Enable */ 00162 #define CAN_IER_ALIE ((uint32_t)(1<<6)) 00163 /** CAN Bus Error Interrupt Enable */ 00164 #define CAN_IER_BEIE ((uint32_t)(1<<7)) 00165 /** CAN ID Ready Interrupt Enable */ 00166 #define CAN_IER_IDIE ((uint32_t)(1<<8)) 00167 /** CAN Transmit Enable Interrupt for Buffer 2 */ 00168 #define CAN_IER_TIE2 ((uint32_t)(1<<9)) 00169 /** CAN Transmit Enable Interrupt for Buffer 3 */ 00170 #define CAN_IER_TIE3 ((uint32_t)(1<<10)) 00171 00172 /*********************************************************************//** 00173 * Macro defines for CAN Bus Timing Register 00174 **********************************************************************/ 00175 /** CAN Baudrate Prescaler */ 00176 #define CAN_BTR_BRP(n) ((uint32_t)(n&0x3FF)) 00177 /** CAN Synchronization Jump Width */ 00178 #define CAN_BTR_SJM(n) ((uint32_t)((n&0x3)<<14)) 00179 /** CAN Time Segment 1 */ 00180 #define CAN_BTR_TESG1(n) ((uint32_t)(n&0xF)<<16)) 00181 /** CAN Time Segment 2 */ 00182 #define CAN_BTR_TESG2(n) ((uint32_t)(n&0xF)<<20)) 00183 /** CAN Sampling */ 00184 #define CAN_BTR_SAM(n) ((uint32_t)(1<<23)) 00185 00186 /*********************************************************************//** 00187 * Macro defines for CAN Error Warning Limit Register 00188 **********************************************************************/ 00189 /** CAN Error Warning Limit */ 00190 #define CAN_EWL_EWL(n) ((uint32_t)(n&0xFF)) 00191 00192 /*********************************************************************//** 00193 * Macro defines for CAN Status Register 00194 **********************************************************************/ 00195 /** CAN Receive Buffer Status */ 00196 #define CAN_SR_RBS ((uint32_t)(1)) 00197 /** CAN Data Overrun Status */ 00198 #define CAN_SR_DOS ((uint32_t)(1<<1)) 00199 /** CAN Transmit Buffer Status 1 */ 00200 #define CAN_SR_TBS1 ((uint32_t)(1<<2)) 00201 /** CAN Transmission Complete Status of Buffer 1 */ 00202 #define CAN_SR_TCS1 ((uint32_t)(1<<3)) 00203 /** CAN Receive Status */ 00204 #define CAN_SR_RS ((uint32_t)(1<<4)) 00205 /** CAN Transmit Status 1 */ 00206 #define CAN_SR_TS1 ((uint32_t)(1<<5)) 00207 /** CAN Error Status */ 00208 #define CAN_SR_ES ((uint32_t)(1<<6)) 00209 /** CAN Bus Status */ 00210 #define CAN_SR_BS ((uint32_t)(1<<7)) 00211 /** CAN Transmit Buffer Status 2 */ 00212 #define CAN_SR_TBS2 ((uint32_t)(1<<10)) 00213 /** CAN Transmission Complete Status of Buffer 2 */ 00214 #define CAN_SR_TCS2 ((uint32_t)(1<<11)) 00215 /** CAN Transmit Status 2 */ 00216 #define CAN_SR_TS2 ((uint32_t)(1<<13)) 00217 /** CAN Transmit Buffer Status 2 */ 00218 #define CAN_SR_TBS3 ((uint32_t)(1<<18)) 00219 /** CAN Transmission Complete Status of Buffer 2 */ 00220 #define CAN_SR_TCS3 ((uint32_t)(1<<19)) 00221 /** CAN Transmit Status 2 */ 00222 #define CAN_SR_TS3 ((uint32_t)(1<<21)) 00223 00224 /*********************************************************************//** 00225 * Macro defines for CAN Receive Frame Status Register 00226 **********************************************************************/ 00227 /** CAN ID Index */ 00228 #define CAN_RFS_ID_INDEX(n) ((uint32_t)(n&0x3FF)) 00229 /** CAN Bypass */ 00230 #define CAN_RFS_BP ((uint32_t)(1<<10)) 00231 /** CAN Data Length Code */ 00232 #define CAN_RFS_DLC(n) ((uint32_t)((n&0xF)<<16) 00233 /** CAN Remote Transmission Request */ 00234 #define CAN_RFS_RTR ((uint32_t)(1<<30)) 00235 /** CAN control 11 bit or 29 bit Identifier */ 00236 #define CAN_RFS_FF ((uint32_t)(1<<31)) 00237 00238 /*********************************************************************//** 00239 * Macro defines for CAN Receive Identifier Register 00240 **********************************************************************/ 00241 /** CAN 11 bit Identifier */ 00242 #define CAN_RID_ID_11(n) ((uint32_t)(n&0x7FF)) 00243 /** CAN 29 bit Identifier */ 00244 #define CAN_RID_ID_29(n) ((uint32_t)(n&0x1FFFFFFF)) 00245 00246 /*********************************************************************//** 00247 * Macro defines for CAN Receive Data A Register 00248 **********************************************************************/ 00249 /** CAN Receive Data 1 */ 00250 #define CAN_RDA_DATA1(n) ((uint32_t)(n&0xFF)) 00251 /** CAN Receive Data 2 */ 00252 #define CAN_RDA_DATA2(n) ((uint32_t)((n&0xFF)<<8)) 00253 /** CAN Receive Data 3 */ 00254 #define CAN_RDA_DATA3(n) ((uint32_t)((n&0xFF)<<16)) 00255 /** CAN Receive Data 4 */ 00256 #define CAN_RDA_DATA4(n) ((uint32_t)((n&0xFF)<<24)) 00257 00258 /*********************************************************************//** 00259 * Macro defines for CAN Receive Data B Register 00260 **********************************************************************/ 00261 /** CAN Receive Data 5 */ 00262 #define CAN_RDB_DATA5(n) ((uint32_t)(n&0xFF)) 00263 /** CAN Receive Data 6 */ 00264 #define CAN_RDB_DATA6(n) ((uint32_t)((n&0xFF)<<8)) 00265 /** CAN Receive Data 7 */ 00266 #define CAN_RDB_DATA7(n) ((uint32_t)((n&0xFF)<<16)) 00267 /** CAN Receive Data 8 */ 00268 #define CAN_RDB_DATA8(n) ((uint32_t)((n&0xFF)<<24)) 00269 00270 /*********************************************************************//** 00271 * Macro defines for CAN Transmit Frame Information Register 00272 **********************************************************************/ 00273 /** CAN Priority */ 00274 #define CAN_TFI_PRIO(n) ((uint32_t)(n&0xFF)) 00275 /** CAN Data Length Code */ 00276 #define CAN_TFI_DLC(n) ((uint32_t)((n&0xF)<<16)) 00277 /** CAN Remote Frame Transmission */ 00278 #define CAN_TFI_RTR ((uint32_t)(1<<30)) 00279 /** CAN control 11-bit or 29-bit Identifier */ 00280 #define CAN_TFI_FF ((uint32_t)(1<<31)) 00281 00282 /*********************************************************************//** 00283 * Macro defines for CAN Transmit Identifier Register 00284 **********************************************************************/ 00285 /** CAN 11-bit Identifier */ 00286 #define CAN_TID_ID11(n) ((uint32_t)(n&0x7FF)) 00287 /** CAN 11-bit Identifier */ 00288 #define CAN_TID_ID29(n) ((uint32_t)(n&0x1FFFFFFF)) 00289 00290 /*********************************************************************//** 00291 * Macro defines for CAN Transmit Data A Register 00292 **********************************************************************/ 00293 /** CAN Transmit Data 1 */ 00294 #define CAN_TDA_DATA1(n) ((uint32_t)(n&0xFF)) 00295 /** CAN Transmit Data 2 */ 00296 #define CAN_TDA_DATA2(n) ((uint32_t)((n&0xFF)<<8)) 00297 /** CAN Transmit Data 3 */ 00298 #define CAN_TDA_DATA3(n) ((uint32_t)((n&0xFF)<<16)) 00299 /** CAN Transmit Data 4 */ 00300 #define CAN_TDA_DATA4(n) ((uint32_t)((n&0xFF)<<24)) 00301 00302 /*********************************************************************//** 00303 * Macro defines for CAN Transmit Data B Register 00304 **********************************************************************/ 00305 /** CAN Transmit Data 5 */ 00306 #define CAN_TDA_DATA5(n) ((uint32_t)(n&0xFF)) 00307 /** CAN Transmit Data 6 */ 00308 #define CAN_TDA_DATA6(n) ((uint32_t)((n&0xFF)<<8)) 00309 /** CAN Transmit Data 7 */ 00310 #define CAN_TDA_DATA7(n) ((uint32_t)((n&0xFF)<<16)) 00311 /** CAN Transmit Data 8 */ 00312 #define CAN_TDA_DATA8(n) ((uint32_t)((n&0xFF)<<24)) 00313 00314 /*********************************************************************//** 00315 * Macro defines for CAN Sleep Clear Register 00316 **********************************************************************/ 00317 /** CAN1 Sleep mode */ 00318 #define CAN1SLEEPCLR ((uint32_t)(1<<1)) 00319 /** CAN2 Sleep Mode */ 00320 #define CAN2SLEEPCLR ((uint32_t)(1<<2)) 00321 00322 /*********************************************************************//** 00323 * Macro defines for CAN Wake up Flags Register 00324 **********************************************************************/ 00325 /** CAN1 Sleep mode */ 00326 #define CAN_WAKEFLAGES_CAN1WAKE ((uint32_t)(1<<1)) 00327 /** CAN2 Sleep Mode */ 00328 #define CAN_WAKEFLAGES_CAN2WAKE ((uint32_t)(1<<2)) 00329 00330 /*********************************************************************//** 00331 * Macro defines for Central transmit Status Register 00332 **********************************************************************/ 00333 /** CAN Transmit 1 */ 00334 #define CAN_TSR_TS1 ((uint32_t)(1)) 00335 /** CAN Transmit 2 */ 00336 #define CAN_TSR_TS2 ((uint32_t)(1<<1)) 00337 /** CAN Transmit Buffer Status 1 */ 00338 #define CAN_TSR_TBS1 ((uint32_t)(1<<8)) 00339 /** CAN Transmit Buffer Status 2 */ 00340 #define CAN_TSR_TBS2 ((uint32_t)(1<<9)) 00341 /** CAN Transmission Complete Status 1 */ 00342 #define CAN_TSR_TCS1 ((uint32_t)(1<<16)) 00343 /** CAN Transmission Complete Status 2 */ 00344 #define CAN_TSR_TCS2 ((uint32_t)(1<<17)) 00345 00346 /*********************************************************************//** 00347 * Macro defines for Central Receive Status Register 00348 **********************************************************************/ 00349 /** CAN Receive Status 1 */ 00350 #define CAN_RSR_RS1 ((uint32_t)(1)) 00351 /** CAN Receive Status 1 */ 00352 #define CAN_RSR_RS2 ((uint32_t)(1<<1)) 00353 /** CAN Receive Buffer Status 1*/ 00354 #define CAN_RSR_RB1 ((uint32_t)(1<<8)) 00355 /** CAN Receive Buffer Status 2*/ 00356 #define CAN_RSR_RB2 ((uint32_t)(1<<9)) 00357 /** CAN Data Overrun Status 1 */ 00358 #define CAN_RSR_DOS1 ((uint32_t)(1<<16)) 00359 /** CAN Data Overrun Status 1 */ 00360 #define CAN_RSR_DOS2 ((uint32_t)(1<<17)) 00361 00362 /*********************************************************************//** 00363 * Macro defines for Central Miscellaneous Status Register 00364 **********************************************************************/ 00365 /** Same CAN Error Status in CAN1GSR */ 00366 #define CAN_MSR_E1 ((uint32_t)(1)) 00367 /** Same CAN Error Status in CAN2GSR */ 00368 #define CAN_MSR_E2 ((uint32_t)(1<<1)) 00369 /** Same CAN Bus Status in CAN1GSR */ 00370 #define CAN_MSR_BS1 ((uint32_t)(1<<8)) 00371 /** Same CAN Bus Status in CAN2GSR */ 00372 #define CAN_MSR_BS2 ((uint32_t)(1<<9)) 00373 00374 /*********************************************************************//** 00375 * Macro defines for Acceptance Filter Mode Register 00376 **********************************************************************/ 00377 /** CAN Acceptance Filter Off mode */ 00378 #define CAN_AFMR_AccOff ((uint32_t)(1)) 00379 /** CAN Acceptance File Bypass mode */ 00380 #define CAN_AFMR_AccBP ((uint32_t)(1<<1)) 00381 /** FullCAN Mode Enhancements */ 00382 #define CAN_AFMR_eFCAN ((uint32_t)(1<<2)) 00383 00384 /*********************************************************************//** 00385 * Macro defines for Standard Frame Individual Start Address Register 00386 **********************************************************************/ 00387 /** The start address of the table of individual Standard Identifier */ 00388 #define CAN_STT_sa(n) ((uint32_t)((n&1FF)<<2)) 00389 00390 /*********************************************************************//** 00391 * Macro defines for Standard Frame Group Start Address Register 00392 **********************************************************************/ 00393 /** The start address of the table of grouped Standard Identifier */ 00394 #define CAN_SFF_GRP_sa(n) ((uint32_t)((n&3FF)<<2)) 00395 00396 /*********************************************************************//** 00397 * Macro defines for Extended Frame Start Address Register 00398 **********************************************************************/ 00399 /** The start address of the table of individual Extended Identifier */ 00400 #define CAN_EFF_sa(n) ((uint32_t)((n&1FF)<<2)) 00401 00402 /*********************************************************************//** 00403 * Macro defines for Extended Frame Group Start Address Register 00404 **********************************************************************/ 00405 /** The start address of the table of grouped Extended Identifier */ 00406 #define CAN_Eff_GRP_sa(n) ((uint32_t)((n&3FF)<<2)) 00407 00408 /*********************************************************************//** 00409 * Macro defines for End Of AF Table Register 00410 **********************************************************************/ 00411 /** The End of Table of AF LookUp Table */ 00412 #define CAN_EndofTable(n) ((uint32_t)((n&3FF)<<2)) 00413 00414 /*********************************************************************//** 00415 * Macro defines for LUT Error Address Register 00416 **********************************************************************/ 00417 /** CAN Look-Up Table Error Address */ 00418 #define CAN_LUTerrAd(n) ((uint32_t)((n&1FF)<<2)) 00419 00420 /*********************************************************************//** 00421 * Macro defines for LUT Error Register 00422 **********************************************************************/ 00423 /** CAN Look-Up Table Error */ 00424 #define CAN_LUTerr ((uint32_t)(1)) 00425 00426 /*********************************************************************//** 00427 * Macro defines for Global FullCANInterrupt Enable Register 00428 **********************************************************************/ 00429 /** Global FullCANInterrupt Enable */ 00430 #define CAN_FCANIE ((uint32_t)(1)) 00431 00432 /*********************************************************************//** 00433 * Macro defines for FullCAN Interrupt and Capture Register 0 00434 **********************************************************************/ 00435 /** FullCAN Interrupt and Capture (0-31)*/ 00436 #define CAN_FCANIC0_IntPnd(n) ((uint32_t)(1<<n)) 00437 00438 /*********************************************************************//** 00439 * Macro defines for FullCAN Interrupt and Capture Register 1 00440 **********************************************************************/ 00441 /** FullCAN Interrupt and Capture (0-31)*/ 00442 #define CAN_FCANIC1_IntPnd(n) ((uint32_t)(1<<(n-32))) 00443 00444 /** 00445 * @} 00446 */ 00447 00448 /** 00449 * @} 00450 */ 00451 00452 00453 /* Public Types --------------------------------------------------------------- */ 00454 /** @defgroup CAN_Public_Types 00455 * @{ 00456 */ 00457 00458 /** CAN configuration structure */ 00459 /*********************************************************************** 00460 * CAN device configuration commands (IOCTL commands and arguments) 00461 **********************************************************************/ 00462 /** 00463 * @brief CAN ID format definition 00464 */ 00465 typedef enum { 00466 STD_ID_FORMAT = 0, /**< Use standard ID format (11 bit ID) */ 00467 EXT_ID_FORMAT = 1 /**< Use extended ID format (29 bit ID) */ 00468 } CAN_ID_FORMAT_Type; 00469 00470 /** 00471 * @brief AFLUT Entry type definition 00472 */ 00473 typedef enum { 00474 FULLCAN_ENTRY = 0, 00475 EXPLICIT_STANDARD_ENTRY, 00476 GROUP_STANDARD_ENTRY, 00477 EXPLICIT_EXTEND_ENTRY, 00478 GROUP_EXTEND_ENTRY, 00479 } AFLUT_ENTRY_Type; 00480 00481 /** 00482 * @brief Symbolic names for type of CAN message 00483 */ 00484 typedef enum { 00485 DATA_FRAME = 0, /**< Data frame */ 00486 REMOTE_FRAME = 1 /**< Remote frame */ 00487 } CAN_FRAME_Type; 00488 00489 /** 00490 * @brief CAN Control status definition 00491 */ 00492 typedef enum { 00493 CANCTRL_GLOBAL_STS = 0, /**< CAN Global Status */ 00494 CANCTRL_INT_CAP, /**< CAN Interrupt and Capture */ 00495 CANCTRL_ERR_WRN, /**< CAN Error Warning Limit */ 00496 CANCTRL_STS /**< CAN Control Status */ 00497 } CAN_CTRL_STS_Type; 00498 00499 /** 00500 * @brief Central CAN status type definition 00501 */ 00502 typedef enum { 00503 CANCR_TX_STS = 0, /**< Central CAN Tx Status */ 00504 CANCR_RX_STS, /**< Central CAN Rx Status */ 00505 CANCR_MS /**< Central CAN Miscellaneous Status */ 00506 } CAN_CR_STS_Type; 00507 00508 /** 00509 * @brief CAN interrupt enable type definition 00510 */ 00511 typedef enum { 00512 CANINT_RIE = 0, /**< CAN Receiver Interrupt Enable */ 00513 CANINT_TIE1, /**< CAN Transmit Interrupt Enable */ 00514 CANINT_EIE, /**< CAN Error Warning Interrupt Enable */ 00515 CANINT_DOIE, /**< CAN Data Overrun Interrupt Enable */ 00516 CANINT_WUIE, /**< CAN Wake-Up Interrupt Enable */ 00517 CANINT_EPIE, /**< CAN Error Passive Interrupt Enable */ 00518 CANINT_ALIE, /**< CAN Arbitration Lost Interrupt Enable */ 00519 CANINT_BEIE, /**< CAN Bus Error Inter rupt Enable */ 00520 CANINT_IDIE, /**< CAN ID Ready Interrupt Enable */ 00521 CANINT_TIE2, /**< CAN Transmit Interrupt Enable for Buffer2 */ 00522 CANINT_TIE3, /**< CAN Transmit Interrupt Enable for Buffer3 */ 00523 CANINT_FCE /**< FullCAN Interrupt Enable */ 00524 } CAN_INT_EN_Type; 00525 00526 /** 00527 * @brief Acceptance Filter Mode type definition 00528 */ 00529 typedef enum { 00530 CAN_Normal = 0, /**< Normal Mode */ 00531 CAN_AccOff, /**< Acceptance Filter Off Mode */ 00532 CAN_AccBP, /**< Acceptance Fileter Bypass Mode */ 00533 CAN_eFCAN /**< FullCAN Mode Enhancement */ 00534 } CAN_AFMODE_Type; 00535 00536 /** 00537 * @brief CAN Mode Type definition 00538 */ 00539 typedef enum { 00540 CAN_OPERATING_MODE = 0, /**< Operating Mode */ 00541 CAN_RESET_MODE, /**< Reset Mode */ 00542 CAN_LISTENONLY_MODE, /**< Listen Only Mode */ 00543 CAN_SELFTEST_MODE, /**< Seft Test Mode */ 00544 CAN_TXPRIORITY_MODE, /**< Transmit Priority Mode */ 00545 CAN_SLEEP_MODE, /**< Sleep Mode */ 00546 CAN_RXPOLARITY_MODE, /**< Receive Polarity Mode */ 00547 CAN_TEST_MODE /**< Test Mode */ 00548 } CAN_MODE_Type; 00549 00550 /** 00551 * @brief Error values that functions can return 00552 */ 00553 typedef enum { 00554 CAN_OK = 1, /**< No error */ 00555 CAN_OBJECTS_FULL_ERROR, /**< No more rx or tx objects available */ 00556 CAN_FULL_OBJ_NOT_RCV, /**< Full CAN object not received */ 00557 CAN_NO_RECEIVE_DATA, /**< No have receive data available */ 00558 CAN_AF_ENTRY_ERROR, /**< Entry load in AFLUT is unvalid */ 00559 CAN_CONFLICT_ID_ERROR, /**< Conflict ID occur */ 00560 CAN_ENTRY_NOT_EXIT_ERROR /**< Entry remove outo AFLUT is not exit */ 00561 } CAN_ERROR; 00562 00563 /** 00564 * @brief Pin Configuration structure 00565 */ 00566 typedef struct { 00567 uint8_t RD; /**< Serial Inputs, from CAN transceivers, should be: 00568 ** For CAN1: 00569 - CAN_RD1_P0_0: RD pin is on P0.0 00570 - CAN_RD1_P0_21 : RD pin is on P0.21 00571 ** For CAN2: 00572 - CAN_RD2_P0_4: RD pin is on P0.4 00573 - CAN_RD2_P2_7: RD pin is on P2.7 00574 */ 00575 uint8_t TD; /**< Serial Outputs, To CAN transceivers, should be: 00576 ** For CAN1: 00577 - CAN_TD1_P0_1: TD pin is on P0.1 00578 - CAN_TD1_P0_22: TD pin is on P0.22 00579 ** For CAN2: 00580 - CAN_TD2_P0_5: TD pin is on P0.5 00581 - CAN_TD2_P2_8: TD pin is on P2.8 00582 */ 00583 } CAN_PinCFG_Type; 00584 00585 /** 00586 * @brief CAN message object structure 00587 */ 00588 typedef struct { 00589 uint32_t id; /**< 29 bit identifier, it depend on "format" value 00590 - if format = STD_ID_FORMAT, id should be 11 bit identifier 00591 - if format = EXT_ID_FORMAT, id should be 29 bit identifier 00592 */ 00593 uint8_t dataA[4]; /**< Data field A */ 00594 uint8_t dataB[4]; /**< Data field B */ 00595 uint8_t len; /**< Length of data field in bytes, should be: 00596 - 0000b-0111b: 0-7 bytes 00597 - 1xxxb: 8 bytes 00598 */ 00599 uint8_t format; /**< Identifier Format, should be: 00600 - STD_ID_FORMAT: Standard ID - 11 bit format 00601 - EXT_ID_FORMAT: Extended ID - 29 bit format 00602 */ 00603 uint8_t type; /**< Remote Frame transmission, should be: 00604 - DATA_FRAME: the number of data bytes called out by the DLC 00605 field are send from the CANxTDA and CANxTDB registers 00606 - REMOTE_FRAME: Remote Frame is sent 00607 */ 00608 } CAN_MSG_Type; 00609 00610 /** 00611 * @brief FullCAN Entry structure 00612 */ 00613 typedef struct { 00614 uint8_t controller; /**< CAN Controller, should be: 00615 - CAN1_CTRL: CAN1 Controller 00616 - CAN2_CTRL: CAN2 Controller 00617 */ 00618 uint8_t disable; /**< Disable bit, should be: 00619 - MSG_ENABLE: disable bit = 0 00620 - MSG_DISABLE: disable bit = 1 00621 */ 00622 uint16_t id_11; /**< Standard ID, should be 11-bit value */ 00623 } FullCAN_Entry; 00624 00625 /** 00626 * @brief Standard ID Frame Format Entry structure 00627 */ 00628 typedef struct { 00629 uint8_t controller; /**< CAN Controller, should be: 00630 - CAN1_CTRL: CAN1 Controller 00631 - CAN2_CTRL: CAN2 Controller 00632 */ 00633 uint8_t disable; /**< Disable bit, should be: 00634 - MSG_ENABLE: disable bit = 0 00635 - MSG_DISABLE: disable bit = 1 00636 */ 00637 uint16_t id_11; /**< Standard ID, should be 11-bit value */ 00638 } SFF_Entry; 00639 00640 /** 00641 * @brief Group of Standard ID Frame Format Entry structure 00642 */ 00643 typedef struct { 00644 uint8_t controller1; /**< First CAN Controller, should be: 00645 - CAN1_CTRL: CAN1 Controller 00646 - CAN2_CTRL: CAN2 Controller 00647 */ 00648 uint8_t disable1; /**< First Disable bit, should be: 00649 - MSG_ENABLE: disable bit = 0) 00650 - MSG_DISABLE: disable bit = 1 00651 */ 00652 uint16_t lowerID; /**< ID lower bound, should be 11-bit value */ 00653 uint8_t controller2; /**< Second CAN Controller, should be: 00654 - CAN1_CTRL: CAN1 Controller 00655 - CAN2_CTRL: CAN2 Controller 00656 */ 00657 uint8_t disable2; /**< Second Disable bit, should be: 00658 - MSG_ENABLE: disable bit = 0 00659 - MSG_DISABLE: disable bit = 1 00660 */ 00661 uint16_t upperID; /**< ID upper bound, should be 11-bit value and 00662 equal or greater than lowerID 00663 */ 00664 } SFF_GPR_Entry; 00665 00666 /** 00667 * @brief Extended ID Frame Format Entry structure 00668 */ 00669 typedef struct { 00670 uint8_t controller; /**< CAN Controller, should be: 00671 - CAN1_CTRL: CAN1 Controller 00672 - CAN2_CTRL: CAN2 Controller 00673 */ 00674 uint32_t ID_29; /**< Extend ID, shoud be 29-bit value */ 00675 } EFF_Entry; 00676 00677 00678 /** 00679 * @brief Group of Extended ID Frame Format Entry structure 00680 */ 00681 typedef struct { 00682 uint8_t controller1; /**< First CAN Controller, should be: 00683 - CAN1_CTRL: CAN1 Controller 00684 - CAN2_CTRL: CAN2 Controller 00685 */ 00686 uint8_t controller2; /**< Second Disable bit, should be: 00687 - MSG_ENABLE: disable bit = 0(default) 00688 - MSG_DISABLE: disable bit = 1 00689 */ 00690 uint32_t lowerEID; /**< Extended ID lower bound, should be 29-bit value */ 00691 uint32_t upperEID; /**< Extended ID upper bound, should be 29-bit value */ 00692 } EFF_GPR_Entry; 00693 00694 00695 /** 00696 * @brief Acceptance Filter Section Table structure 00697 */ 00698 typedef struct { 00699 FullCAN_Entry* FullCAN_Sec; /**< The pointer point to FullCAN_Entry */ 00700 uint8_t FC_NumEntry; /**< FullCAN Entry Number */ 00701 SFF_Entry* SFF_Sec; /**< The pointer point to SFF_Entry */ 00702 uint8_t SFF_NumEntry; /**< Standard ID Entry Number */ 00703 SFF_GPR_Entry* SFF_GPR_Sec; /**< The pointer point to SFF_GPR_Entry */ 00704 uint8_t SFF_GPR_NumEntry; /**< Group Standard ID Entry Number */ 00705 EFF_Entry* EFF_Sec; /**< The pointer point to EFF_Entry */ 00706 uint8_t EFF_NumEntry; /**< Extended ID Entry Number */ 00707 EFF_GPR_Entry* EFF_GPR_Sec; /**< The pointer point to EFF_GPR_Entry */ 00708 uint8_t EFF_GPR_NumEntry; /**< Group Extended ID Entry Number */ 00709 } AF_SectionDef; 00710 00711 /** 00712 * @brief CAN call-back function type definitions 00713 */ 00714 typedef void ( fnCANCbs_Type)(); 00715 00716 00717 /** 00718 * @} 00719 */ 00720 00721 00722 /* Public Macros -------------------------------------------------------------- */ 00723 /** @defgroup CAN_Public_Macros 00724 * @{ 00725 */ 00726 00727 /** Macro to determine if it is valid CAN peripheral */ 00728 #define PARAM_CANx(x) ((((uint32_t*)x)==((uint32_t *)LPC_CAN1)) \ 00729 ||(((uint32_t*)x)==((uint32_t *)LPC_CAN2))) 00730 00731 #define PARAM_CANAFx(x) (((uint32_t*)x)== ((uint32_t*)LPC_CANAF)) 00732 #define PARAM_CANAFRAMx(x) (((uint32_t*)x)== (uint32_t*)LPC_CANAF_RAM) 00733 #define PARAM_CANCRx(x) (((uint32_t*)x)==((uint32_t*)LPC_CANCR)) 00734 00735 /** Macro to check Data to send valid */ 00736 #define PARAM_I2S_DATA(data) ((data <= 0xFFFFFFFF)) 00737 #define PRAM_I2S_FREQ(freq) ((freq>=16000)&&(freq <= 96000)) 00738 00739 /** Macro to check Pin Selection value */ 00740 #define PARAM_RD1_PIN(n) ((n==CAN_RD1_P0_0)||(n==CAN_RD1_P0_21)) 00741 #define PARAM_TD1_PIN(n) ((n==CAN_TD1_P0_1)||(n==CAN_TD1_P0_22)) 00742 #define PARAM_RD2_PIN(n) ((n==CAN_RD2_P0_4)||(n==CAN_RD2_P2_7)) 00743 #define PARAM_TD2_PIN(n) ((n==CAN_TD2_P0_5)||(n==CAN_TD2_P2_8)) 00744 00745 /** Macro to check Frame Identifier */ 00746 #define PARAM_ID_11(n) ((n>>11)==0) /*-- 11 bit --*/ 00747 #define PARAM_ID_29(n) ((n>>29)==0) /*-- 29 bit --*/ 00748 00749 #define PARAM_DLC(n) ((n>>4)==0) /*-- 4 bit --*/ 00750 #define PARAM_ID_FORMAT(n) ((n==STD_ID_FORMAT)||(n==EXT_ID_FORMAT)) 00751 #define PARAM_GRP_ID(x, y) ((x<=y)) 00752 #define PARAM_FRAME_TYPE(n) ((n==DATA_FRAME)||(n==REMOTE_FRAME)) 00753 00754 /** Macro to check Control/Central Status type parameter */ 00755 #define PARAM_CTRL_STS_TYPE(n) ((n==CANCTRL_GLOBAL_STS)||(n==CANCTRL_INT_CAP) \ 00756 ||(n==CANCTRL_ERR_WRN)||(n==CANCTRL_STS)) 00757 #define PARAM_CR_STS_TYPE(n) ((n==CANCR_TX_STS)||(n==CANCR_RX_STS) \ 00758 ||(n==CANCR_MS)) 00759 /** Macro to check AF Mode type parameter */ 00760 #define PARAM_AFMODE_TYPE(n) ((n==CAN_Normal)||(n==CAN_AccOff) \ 00761 ||(n==CAN_AccBP)||(n==CAN_eFCAN)) 00762 /** Macro to check Operation Mode */ 00763 #define PARAM_MODE_TYPE(n) ((n==CAN_OPERATING_MODE)||(n==CAN_RESET_MODE) \ 00764 ||(n==CAN_LISTENONLY_MODE)||(n==CAN_SELFTEST_MODE) \ 00765 ||(n==CAN_TXPRIORITY_MODE)||(n==CAN_SLEEP_MODE) \ 00766 ||(n==CAN_RXPOLARITY_MODE)||(n==CAN_TEST_MODE)) 00767 00768 /** Macro define for struct AF_Section parameter */ 00769 #define CAN1_CTRL ((uint8_t)(0)) 00770 #define CAN2_CTRL ((uint8_t)(1)) 00771 #define PARAM_CTRL(n) ((n==CAN1_CTRL)|(n==CAN2_CTRL)) 00772 00773 #define MSG_ENABLE ((uint8_t)(0)) 00774 #define MSG_DISABLE ((uint8_t)(1)) 00775 #define PARAM_MSG_DISABLE(n) ((n==MSG_ENABLE)|(n==MSG_DISABLE)) 00776 00777 /**Macro to check Interrupt Type parameter */ 00778 #define PARAM_INT_EN_TYPE(n) ((n==CANINT_RIE)||(n==CANINT_TIE1) \ 00779 ||(n==CANINT_EIE)||(n==CANINT_DOIE) \ 00780 ||(n==CANINT_WUIE)||(n==CANINT_EPIE) \ 00781 ||(n==CANINT_ALIE)||(n==CANINT_BEIE) \ 00782 ||(n==CANINT_IDIE)||(n==CANINT_TIE2) \ 00783 ||(n==CANINT_TIE3)||(n==CANINT_FCE)) 00784 00785 /** Macro to check AFLUT Entry type */ 00786 #define PARAM_AFLUT_ENTRY_TYPE(n) ((n==FULLCAN_ENTRY)||(n==EXPLICIT_STANDARD_ENTRY)\ 00787 ||(n==GROUP_STANDARD_ENTRY)||(n==EXPLICIT_EXTEND_ENTRY) \ 00788 ||(n==GROUP_EXTEND_ENTRY)) 00789 #define PARAM_POSITION(n) ((n<512)) 00790 00791 /** CAN function pin selection defines */ 00792 #define CAN_RD1_P0_0 ((uint8_t)(0)) 00793 #define CAN_RD1_P0_21 ((uint8_t)(1)) 00794 #define CAN_TD1_P0_1 ((uint8_t)(0)) 00795 #define CAN_TD1_P0_22 ((uint8_t)(1)) 00796 00797 #define CAN_RD2_P0_4 ((uint8_t)(0)) 00798 #define CAN_RD2_P2_7 ((uint8_t)(1)) 00799 #define CAN_TD2_P0_5 ((uint8_t)(0)) 00800 #define CAN_TD2_P2_8 ((uint8_t)(1)) 00801 00802 00803 /** 00804 * @} 00805 */ 00806 00807 00808 /* Public Functions ----------------------------------------------------------- */ 00809 /** @defgroup CAN_Public_Functions 00810 * @{ 00811 */ 00812 00813 void CAN_Init(LPC_CAN_TypeDef *CANx, uint32_t baudrate); 00814 void CAN_DeInit(LPC_CAN_TypeDef *CANx); 00815 00816 Status CAN_SendMsg(LPC_CAN_TypeDef *CANx, CAN_MSG_Type *CAN_Msg); 00817 Status CAN_ReceiveMsg(LPC_CAN_TypeDef *CANx, CAN_MSG_Type *CAN_Msg); 00818 CAN_ERROR FCAN_ReadObj(LPC_CANAF_TypeDef* CANAFx, CAN_MSG_Type *CAN_Msg); 00819 00820 uint32_t CAN_GetCTRLStatus(LPC_CAN_TypeDef* CANx, CAN_CTRL_STS_Type arg); 00821 uint32_t CAN_GetCRStatus(LPC_CANCR_TypeDef* CANCRx, CAN_CR_STS_Type arg); 00822 void CAN_ModeConfig(LPC_CAN_TypeDef* CANx, CAN_MODE_Type mode, 00823 FunctionalState NewState); 00824 void CAN_SetBaudRate(LPC_CAN_TypeDef *CANx, uint32_t baudrate); 00825 00826 void CAN_SetAFMode(LPC_CANAF_TypeDef* CANAFx, CAN_AFMODE_Type AFmode); 00827 CAN_ERROR CAN_SetupAFLUT(LPC_CANAF_TypeDef* CANAFx, AF_SectionDef* AFSection); 00828 CAN_ERROR CAN_LoadFullCANEntry(LPC_CAN_TypeDef* CANx, uint16_t ID); 00829 CAN_ERROR CAN_LoadExplicitEntry(LPC_CAN_TypeDef* CANx, uint32_t ID, 00830 CAN_ID_FORMAT_Type format); 00831 CAN_ERROR CAN_LoadGroupEntry(LPC_CAN_TypeDef* CANx, uint32_t lowerID, 00832 uint32_t upperID, CAN_ID_FORMAT_Type format); 00833 CAN_ERROR CAN_RemoveEntry(AFLUT_ENTRY_Type EntryType, uint16_t position); 00834 00835 void CAN_SetupCBS(CAN_INT_EN_Type arg, fnCANCbs_Type* pnCANCbs); 00836 void CAN_IRQCmd(LPC_CAN_TypeDef* CANx, CAN_INT_EN_Type arg, 00837 FunctionalState NewState); 00838 void CAN_IntHandler(LPC_CAN_TypeDef* CANx); 00839 00840 /** 00841 * @} 00842 */ 00843 00844 00845 #ifdef __cplusplus 00846 } 00847 #endif 00848 00849 #endif /* LPC17XX_CAN_H_ */ 00850 00851 /** 00852 * @} 00853 */ 00854 00855 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
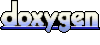