
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
Functions | |
void | CAN_Init (LPC_CAN_TypeDef *CANx, uint32_t baudrate) |
Initialize CAN peripheral with given baudrate. | |
void | CAN_DeInit (LPC_CAN_TypeDef *CANx) |
CAN deInit. | |
Status | CAN_SendMsg (LPC_CAN_TypeDef *CANx, CAN_MSG_Type *CAN_Msg) |
Send message data. | |
Status | CAN_ReceiveMsg (LPC_CAN_TypeDef *CANx, CAN_MSG_Type *CAN_Msg) |
Receive message data. | |
CAN_ERROR | FCAN_ReadObj (LPC_CANAF_TypeDef *CANAFx, CAN_MSG_Type *CAN_Msg) |
Receive FullCAN Object. | |
uint32_t | CAN_GetCTRLStatus (LPC_CAN_TypeDef *CANx, CAN_CTRL_STS_Type arg) |
Get CAN Control Status. | |
uint32_t | CAN_GetCRStatus (LPC_CANCR_TypeDef *CANCRx, CAN_CR_STS_Type arg) |
Get CAN Central Status. | |
void | CAN_ModeConfig (LPC_CAN_TypeDef *CANx, CAN_MODE_Type mode, FunctionalState NewState) |
Enable/Disable CAN Mode. | |
void | CAN_SetBaudRate (LPC_CAN_TypeDef *CANx, uint32_t baudrate) |
Setting CAN baud rate (bps) | |
void | CAN_SetAFMode (LPC_CANAF_TypeDef *CANAFx, CAN_AFMODE_Type AFMode) |
Setting Acceptance Filter mode. | |
CAN_ERROR | CAN_SetupAFLUT (LPC_CANAF_TypeDef *CANAFx, AF_SectionDef *AFSection) |
Setup Acceptance Filter Look-Up Table. | |
CAN_ERROR | CAN_LoadFullCANEntry (LPC_CAN_TypeDef *CANx, uint16_t id) |
Load FullCAN entry into AFLUT. | |
CAN_ERROR | CAN_LoadExplicitEntry (LPC_CAN_TypeDef *CANx, uint32_t id, CAN_ID_FORMAT_Type format) |
Add Explicit ID into AF Look-Up Table dynamically. | |
CAN_ERROR | CAN_RemoveEntry (AFLUT_ENTRY_Type EntryType, uint16_t position) |
Remove AFLUT entry (FullCAN entry and Explicit Standard entry) | |
void | CAN_SetupCBS (CAN_INT_EN_Type arg, fnCANCbs_Type *pnCANCbs) |
Install interrupt call-back function. | |
void | CAN_IRQCmd (LPC_CAN_TypeDef *CANx, CAN_INT_EN_Type arg, FunctionalState NewState) |
Enable/Disable CAN Interrupt. | |
void | CAN_IntHandler (LPC_CAN_TypeDef *CANx) |
Standard CAN interrupt handler, this function will check all interrupt status of CAN channels, then execute the call back function if they're already installed. | |
CAN_ERROR | CAN_LoadGroupEntry (LPC_CAN_TypeDef *CANx, uint32_t lowerID,\uint32_t upperID, CAN_ID_FORMAT_Type format) |
Load Group entry into AFLUT. |
Function Documentation
void CAN_DeInit | ( | LPC_CAN_TypeDef * | CANx ) |
CAN deInit.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
- Returns:
- void
Definition at line 233 of file lpc17xx_can.c.
uint32_t CAN_GetCRStatus | ( | LPC_CANCR_TypeDef * | CANCRx, |
CAN_CR_STS_Type | arg | ||
) |
Get CAN Central Status.
- Parameters:
-
[in] CANCRx point to LPC_CANCR_TypeDef [in] arg,: type of CAN status to get from CAN Central status register Should be: - CANCR_TX_STS: Central CAN Tx Status
- CANCR_RX_STS: Central CAN Rx Status
- CANCR_MS: Central CAN Miscellaneous Status
- Returns:
- Current Central Status that you want to get value
Definition at line 1686 of file lpc17xx_can.c.
uint32_t CAN_GetCTRLStatus | ( | LPC_CAN_TypeDef * | CANx, |
CAN_CTRL_STS_Type | arg | ||
) |
Get CAN Control Status.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] arg,: type of CAN status to get from CAN status register Should be: - CANCTRL_GLOBAL_STS: CAN Global Status
- CANCTRL_INT_CAP: CAN Interrupt and Capture
- CANCTRL_ERR_WRN: CAN Error Warning Limit
- CANCTRL_STS: CAN Control Status
- Returns:
- Current Control Status that you want to get value
Definition at line 1656 of file lpc17xx_can.c.
void CAN_Init | ( | LPC_CAN_TypeDef * | CANx, |
uint32_t | baudrate | ||
) |
Initialize CAN peripheral with given baudrate.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] baudrate,: the value of CAN baudrate will be set (bps)
- Returns:
- void
Definition at line 175 of file lpc17xx_can.c.
void CAN_IntHandler | ( | LPC_CAN_TypeDef * | CANx ) |
Standard CAN interrupt handler, this function will check all interrupt status of CAN channels, then execute the call back function if they're already installed.
- Parameters:
-
[in] CANx point to CAN peripheral selected, should be: CAN1 or CAN2
- Returns:
- None
Definition at line 1893 of file lpc17xx_can.c.
void CAN_IRQCmd | ( | LPC_CAN_TypeDef * | CANx, |
CAN_INT_EN_Type | arg, | ||
FunctionalState | NewState | ||
) |
Enable/Disable CAN Interrupt.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] arg,: type of CAN interrupt that you want to enable/disable Should be: - CANINT_RIE: CAN Receiver Interrupt Enable
- CANINT_TIE1: CAN Transmit Interrupt Enable
- CANINT_EIE: CAN Error Warning Interrupt Enable
- CANINT_DOIE: CAN Data Overrun Interrupt Enable
- CANINT_WUIE: CAN Wake-Up Interrupt Enable
- CANINT_EPIE: CAN Error Passive Interrupt Enable
- CANINT_ALIE: CAN Arbitration Lost Interrupt Enable
- CANINT_BEIE: CAN Bus Error Interrupt Enable
- CANINT_IDIE: CAN ID Ready Interrupt Enable
- CANINT_TIE2: CAN Transmit Interrupt Enable for Buffer2
- CANINT_TIE3: CAN Transmit Interrupt Enable for Buffer3
- CANINT_FCE: FullCAN Interrupt Enable
[in] NewState,: New state of this function, should be: - ENABLE
- DISABLE
- Returns:
- none
Definition at line 1727 of file lpc17xx_can.c.
CAN_ERROR CAN_LoadExplicitEntry | ( | LPC_CAN_TypeDef * | CANx, |
uint32_t | id, | ||
CAN_ID_FORMAT_Type | format | ||
) |
Add Explicit ID into AF Look-Up Table dynamically.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] id,: The ID of entry will be added [in] format,: is the type of ID Frame Format, should be: - STD_ID_FORMAT: 11-bit ID value
- EXT_ID_FORMAT: 29-bit ID value
- Returns:
- CAN Error, could be:
- CAN_OBJECTS_FULL_ERROR: No more rx or tx objects available
- CAN_ID_EXIT_ERROR: ID exited in table
- CAN_OK: ID is added into table successfully
Definition at line 525 of file lpc17xx_can.c.
CAN_ERROR CAN_LoadFullCANEntry | ( | LPC_CAN_TypeDef * | CANx, |
uint16_t | id | ||
) |
Load FullCAN entry into AFLUT.
- Parameters:
-
[in] CANx,: CAN peripheral selected, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] id,: identifier of entry that will be added
- Returns:
- CAN_ERROR, could be:
- CAN_OK: loading is successful
- CAN_ID_EXIT_ERROR: ID exited in FullCAN Section
- CAN_OBJECTS_FULL_ERROR: no more space available
Definition at line 734 of file lpc17xx_can.c.
CAN_ERROR CAN_LoadGroupEntry | ( | LPC_CAN_TypeDef * | CANx, |
uint32_t | lowerID, | ||
\uint32_t | upperID, | ||
CAN_ID_FORMAT_Type | format | ||
) |
Load Group entry into AFLUT.
- Parameters:
-
[in] CANx,: CAN peripheral selected, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] lowerID,upperID,: lower and upper identifier of entry [in] format,: type of ID format, should be: - STD_ID_FORMAT: Standard ID format (11-bit value)
- EXT_ID_FORMAT: Extended ID format (29-bit value)
- Returns:
- CAN_ERROR, could be:
- CAN_OK: loading is successful
- CAN_CONFLICT_ID_ERROR: Conflict ID occurs
- CAN_OBJECTS_FULL_ERROR: no more space available
Definition at line 920 of file lpc17xx_can.c.
void CAN_ModeConfig | ( | LPC_CAN_TypeDef * | CANx, |
CAN_MODE_Type | mode, | ||
FunctionalState | NewState | ||
) |
Enable/Disable CAN Mode.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] mode,: type of CAN mode that you want to enable/disable, should be: - CAN_OPERATING_MODE: Normal Operating Mode
- CAN_RESET_MODE: Reset Mode
- CAN_LISTENONLY_MODE: Listen Only Mode
- CAN_SELFTEST_MODE: Self Test Mode
- CAN_TXPRIORITY_MODE: Transmit Priority Mode
- CAN_SLEEP_MODE: Sleep Mode
- CAN_RXPOLARITY_MODE: Receive Polarity Mode
- CAN_TEST_MODE: Test Mode
[in] NewState,: New State of this function, should be: - ENABLE
- DISABLE
- Returns:
- none
Definition at line 1829 of file lpc17xx_can.c.
Status CAN_ReceiveMsg | ( | LPC_CAN_TypeDef * | CANx, |
CAN_MSG_Type * | CAN_Msg | ||
) |
Receive message data.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] CAN_Msg point to the CAN_MSG_Type Struct, it will contain received message information such as: ID, DLC, RTR, ID Format
- Returns:
- Status:
- SUCCESS: receive message successfully
- ERROR: receive message unsuccessfully
Definition at line 1499 of file lpc17xx_can.c.
CAN_ERROR CAN_RemoveEntry | ( | AFLUT_ENTRY_Type | EntryType, |
uint16_t | position | ||
) |
Remove AFLUT entry (FullCAN entry and Explicit Standard entry)
- Parameters:
-
[in] EntryType,: the type of entry that want to remove, should be: - FULLCAN_ENTRY
- EXPLICIT_STANDARD_ENTRY
- GROUP_STANDARD_ENTRY
- EXPLICIT_EXTEND_ENTRY
- GROUP_EXTEND_ENTRY
[in] position,: the position of this entry in its section Note: the first position is 0
- Returns:
- CAN_ERROR, could be:
- CAN_OK: removing is successful
- CAN_ENTRY_NOT_EXIT_ERROR: entry want to remove is not exit
Definition at line 1100 of file lpc17xx_can.c.
Status CAN_SendMsg | ( | LPC_CAN_TypeDef * | CANx, |
CAN_MSG_Type * | CAN_Msg | ||
) |
Send message data.
- Parameters:
-
[in] CANx pointer to LPC_CAN_TypeDef, should be: - CAN1: CAN 1
- CAN2: CAN 2
[in] CAN_Msg point to the CAN_MSG_Type Structure, it contains message information such as: ID, DLC, RTR, ID Format
- Returns:
- Status:
- SUCCESS: send message successfully
- ERROR: send message unsuccessfully
Definition at line 1340 of file lpc17xx_can.c.
void CAN_SetAFMode | ( | LPC_CANAF_TypeDef * | CANAFx, |
CAN_AFMODE_Type | AFMode | ||
) |
Setting Acceptance Filter mode.
- Parameters:
-
[in] CANAFx point to LPC_CANAF_TypeDef object, should be: CANAF [in] AFMode,: type of AF mode that you want to set, should be: - CAN_Normal: Normal mode
- CAN_AccOff: Acceptance Filter Off Mode
- CAN_AccBP: Acceptance Fileter Bypass Mode
- CAN_eFCAN: FullCAN Mode Enhancement
- Returns:
- none
Definition at line 1788 of file lpc17xx_can.c.
void CAN_SetBaudRate | ( | LPC_CAN_TypeDef * | CANx, |
uint32_t | baudrate | ||
) |
Setting CAN baud rate (bps)
- Parameters:
-
[in] CANx point to LPC_CAN_TypeDef object, should be: - CAN1
- CAN2
[in] baudrate is the baud rate value will be set
- Returns:
- None
Definition at line 119 of file lpc17xx_can.c.
CAN_ERROR CAN_SetupAFLUT | ( | LPC_CANAF_TypeDef * | CANAFx, |
AF_SectionDef * | AFSection | ||
) |
Setup Acceptance Filter Look-Up Table.
- Parameters:
-
[in] CANAFx pointer to LPC_CANAF_TypeDef, should be: CANAF [in] AFSection the pointer to AF_SectionDef struct It contain information about 5 sections will be install in AFLUT
- Returns:
- CAN Error could be:
- CAN_OBJECTS_FULL_ERROR: No more rx or tx objects available
- CAN_AF_ENTRY_ERROR: table error-violation of ascending numerical order
- CAN_OK: ID is added into table successfully
Definition at line 258 of file lpc17xx_can.c.
void CAN_SetupCBS | ( | CAN_INT_EN_Type | arg, |
fnCANCbs_Type * | pnCANCbs | ||
) |
Install interrupt call-back function.
- Parameters:
-
[in] arg,: CAN interrupt type, should be: - CANINT_RIE: CAN Receiver Interrupt Enable
- CANINT_TIE1: CAN Transmit Interrupt Enable
- CANINT_EIE: CAN Error Warning Interrupt Enable
- CANINT_DOIE: CAN Data Overrun Interrupt Enable
- CANINT_WUIE: CAN Wake-Up Interrupt Enable
- CANINT_EPIE: CAN Error Passive Interrupt Enable
- CANINT_ALIE: CAN Arbitration Lost Interrupt Enable
- CANINT_BEIE: CAN Bus Error Interrupt Enable
- CANINT_IDIE: CAN ID Ready Interrupt Enable
- CANINT_TIE2: CAN Transmit Interrupt Enable for Buffer2
- CANINT_TIE3: CAN Transmit Interrupt Enable for Buffer3
- CANINT_FCE: FullCAN Interrupt Enable
- Parameters:
-
[in] pnCANCbs,: pointer point to call-back function
- Returns:
- None
Definition at line 1773 of file lpc17xx_can.c.
CAN_ERROR FCAN_ReadObj | ( | LPC_CANAF_TypeDef * | CANAFx, |
CAN_MSG_Type * | CAN_Msg | ||
) |
Receive FullCAN Object.
- Parameters:
-
[in] CANAFx,: CAN Acceptance Filter register, should be LPC_CANAF [in] CAN_Msg point to the CAN_MSG_Type Struct, it will contain received message information such as: ID, DLC, RTR, ID Format
- Returns:
- CAN_ERROR, could be:
- CAN_FULL_OBJ_NOT_RCV: FullCAN Object is not be received
- CAN_OK: Received FullCAN Object successful
Definition at line 1565 of file lpc17xx_can.c.
Generated on Tue Jul 12 2022 17:06:02 by
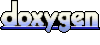