
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_adc.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_adc.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for ADC firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 3. April. 2009 00007 * @author : NgaDinh 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup ADC 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_ADC_H_ 00028 #define LPC17XX_ADC_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Private Macros ------------------------------------------------------------- */ 00042 /** @defgroup ADC_Private_Macros ADC_Private_Macros 00043 * @{ 00044 */ 00045 00046 00047 /** @defgroup group3 ADC_REGISTER_BIT_DEFINITIONS 00048 * @{ 00049 */ 00050 00051 /*********************************************************************//** 00052 * Macro defines for ADC control register 00053 **********************************************************************/ 00054 /** Selects which of the AD0.0:7 pins is (are) to be sampled and converted */ 00055 #define ADC_CR_CH_SEL(n) ((1UL << n)) 00056 /** The APB clock (PCLK) is divided by (this value plus one) 00057 * to produce the clock for the A/D */ 00058 #define ADC_CR_CLKDIV(n) ((n<<8)) 00059 /** Repeated conversions A/D enable bit */ 00060 #define ADC_CR_BURST ((1UL<<16)) 00061 /** ADC convert in power down mode */ 00062 #define ADC_CR_PDN ((1UL<<21)) 00063 /** Start mask bits */ 00064 #define ADC_CR_START_MASK ((7UL<<24)) 00065 /** Select Start Mode */ 00066 #define ADC_CR_START_MODE_SEL(SEL) ((SEL<<24)) 00067 /** Start conversion now */ 00068 #define ADC_CR_START_NOW ((1UL<<24)) 00069 /** Start conversion when the edge selected by bit 27 occurs on P2.10/EINT0 */ 00070 #define ADC_CR_START_EINT0 ((2UL<<24)) 00071 /** Start conversion when the edge selected by bit 27 occurs on P1.27/CAP0.1 */ 00072 #define ADC_CR_START_CAP01 ((3UL<<24)) 00073 /** Start conversion when the edge selected by bit 27 occurs on MAT0.1 */ 00074 #define ADC_CR_START_MAT01 ((4UL<<24)) 00075 /** Start conversion when the edge selected by bit 27 occurs on MAT0.3 */ 00076 #define ADC_CR_START_MAT03 ((5UL<<24)) 00077 /** Start conversion when the edge selected by bit 27 occurs on MAT1.0 */ 00078 #define ADC_CR_START_MAT10 ((6UL<<24)) 00079 /** Start conversion when the edge selected by bit 27 occurs on MAT1.1 */ 00080 #define ADC_CR_START_MAT11 ((7UL<<24)) 00081 /** Start conversion on a falling edge on the selected CAP/MAT signal */ 00082 #define ADC_CR_EDGE ((1UL<<27)) 00083 00084 /*********************************************************************//** 00085 * Macro defines for ADC Global Data register 00086 **********************************************************************/ 00087 /** When DONE is 1, this field contains result value of ADC conversion */ 00088 #define ADC_GDR_RESULT(n) (((n>>4)&0xFFF)) 00089 /** These bits contain the channel from which the LS bits were converted */ 00090 #define ADC_GDR_CH(n) (((n>>24)&0x7)) 00091 /** This bit is 1 in burst mode if the results of one or 00092 * more conversions was (were) lost */ 00093 #define ADC_GDR_OVERRUN_FLAG ((1UL<<30)) 00094 /** This bit is set to 1 when an A/D conversion completes */ 00095 #define ADC_GDR_DONE_FLAG ((1UL<<31)) 00096 00097 /** This bits is used to mask for Channel */ 00098 #define ADC_GDR_CH_MASK ((7UL<<24)) 00099 /*********************************************************************//** 00100 * Macro defines for ADC Interrupt register 00101 **********************************************************************/ 00102 /** These bits allow control over which A/D channels generate 00103 * interrupts for conversion completion */ 00104 #define ADC_INTEN_CH(n) ((1UL<<n)) 00105 /** When 1, enables the global DONE flag in ADDR to generate an interrupt */ 00106 #define ADC_INTEN_GLOBAL ((1UL<<8)) 00107 00108 /*********************************************************************//** 00109 * Macro defines for ADC Data register 00110 **********************************************************************/ 00111 /** When DONE is 1, this field contains result value of ADC conversion */ 00112 #define ADC_DR_RESULT(n) (((n>>4)&0xFFF)) 00113 /** These bits mirror the OVERRRUN status flags that appear in the 00114 * result register for each A/D channel */ 00115 #define ADC_DR_OVERRUN_FLAG ((1UL<<30)) 00116 /** This bit is set to 1 when an A/D conversion completes. It is cleared 00117 * when this register is read */ 00118 #define ADC_DR_DONE_FLAG ((1UL<<31)) 00119 00120 /*********************************************************************//** 00121 * Macro defines for ADC Status register 00122 **********************************************************************/ 00123 /** These bits mirror the DONE status flags that appear in the result 00124 * register for each A/D channel */ 00125 #define ADC_STAT_CH_DONE_FLAG(n) ((n&0xFF)) 00126 /** These bits mirror the OVERRRUN status flags that appear in the 00127 * result register for each A/D channel */ 00128 #define ADC_STAT_CH_OVERRUN_FLAG(n) (((n>>8)&0xFF)) 00129 /** This bit is the A/D interrupt flag */ 00130 #define ADC_STAT_INT_FLAG ((1UL<<16)) 00131 00132 /*********************************************************************//** 00133 * Macro defines for ADC Trim register 00134 **********************************************************************/ 00135 /** Offset trim bits for ADC operation */ 00136 #define ADC_ADCOFFS(n) (((n&0xF)<<4)) 00137 /** Written to boot code*/ 00138 #define ADC_TRIM(n) (((n&0xF)<<8)) 00139 00140 /** 00141 * @} 00142 */ 00143 00144 /** 00145 * @} 00146 */ 00147 00148 00149 /* Public Types --------------------------------------------------------------- */ 00150 /** @defgroup ADC_Public_Types 00151 * @{ 00152 */ 00153 00154 /*********************************************************************//** 00155 * @brief ADC enumeration 00156 **********************************************************************/ 00157 /** @brief Channel Selection */ 00158 typedef enum 00159 { 00160 ADC_CHANNEL_0 = 0, /*!< Channel 0 */ 00161 ADC_CHANNEL_1 , /*!< Channel 1 */ 00162 ADC_CHANNEL_2 , /*!< Channel 2 */ 00163 ADC_CHANNEL_3 , /*!< Channel 3 */ 00164 ADC_CHANNEL_4 , /*!< Channel 4 */ 00165 ADC_CHANNEL_5 , /*!< Channel 5 */ 00166 ADC_CHANNEL_6 , /*!< Channel 6 */ 00167 ADC_CHANNEL_7 /*!< Channel 7 */ 00168 }ADC_CHANNEL_SELECTION; 00169 00170 00171 00172 /** @brief Type of start option */ 00173 00174 /** @brief Type of start option */ 00175 00176 typedef enum 00177 { 00178 ADC_START_CONTINUOUS =0, /*!< Continuous mode */ 00179 ADC_START_NOW , /*!< Start conversion now */ 00180 ADC_START_ON_EINT0 , /*!< Start conversion when the edge selected 00181 * by bit 27 occurs on P2.10/EINT0 */ 00182 ADC_START_ON_CAP01 , /*!< Start conversion when the edge selected 00183 * by bit 27 occurs on P1.27/CAP0.1 */ 00184 ADC_START_ON_MAT01 , /*!< Start conversion when the edge selected 00185 * by bit 27 occurs on MAT0.1 */ 00186 ADC_START_ON_MAT03 , /*!< Start conversion when the edge selected 00187 * by bit 27 occurs on MAT0.3 */ 00188 ADC_START_ON_MAT10 , /*!< Start conversion when the edge selected 00189 * by bit 27 occurs on MAT1.0 */ 00190 ADC_START_ON_MAT11 /*!< Start conversion when the edge selected 00191 * by bit 27 occurs on MAT1.1 */ 00192 } ADC_START_OPT; 00193 00194 00195 /** @brief Type of edge when start conversion on the selected CAP/MAT signal */ 00196 00197 typedef enum 00198 { 00199 ADC_START_ON_RISING = 0, /*!< Start conversion on a rising edge 00200 *on the selected CAP/MAT signal */ 00201 ADC_START_ON_FALLING /*!< Start conversion on a falling edge 00202 *on the selected CAP/MAT signal */ 00203 } ADC_START_ON_EDGE_OPT; 00204 00205 /** @brief* ADC type interrupt enum */ 00206 typedef enum 00207 { 00208 ADC_ADINTEN0 = 0, /*!< Interrupt channel 0 */ 00209 ADC_ADINTEN1 , /*!< Interrupt channel 1 */ 00210 ADC_ADINTEN2 , /*!< Interrupt channel 2 */ 00211 ADC_ADINTEN3 , /*!< Interrupt channel 3 */ 00212 ADC_ADINTEN4 , /*!< Interrupt channel 4 */ 00213 ADC_ADINTEN5 , /*!< Interrupt channel 5 */ 00214 ADC_ADINTEN6 , /*!< Interrupt channel 6 */ 00215 ADC_ADINTEN7 , /*!< Interrupt channel 7 */ 00216 ADC_ADGINTEN /*!< Individual channel/global flag done generate an interrupt */ 00217 }ADC_TYPE_INT_OPT; 00218 00219 /** Macro to determine if it is valid interrupt type */ 00220 #define PARAM_ADC_TYPE_INT_OPT(OPT) ((OPT == ADC_ADINTEN0)||(OPT == ADC_ADINTEN1)\ 00221 ||(OPT == ADC_ADINTEN2)||(OPT == ADC_ADINTEN3)\ 00222 ||(OPT == ADC_ADINTEN4)||(OPT == ADC_ADINTEN5)\ 00223 ||(OPT == ADC_ADINTEN6)||(OPT == ADC_ADINTEN7)\ 00224 ||(OPT == ADC_ADGINTEN)) 00225 00226 00227 /** @brief ADC Data status */ 00228 typedef enum 00229 { 00230 ADC_DATA_BURST = 0, /*Burst bit*/ 00231 ADC_DATA_DONE /*Done bit*/ 00232 }ADC_DATA_STATUS; 00233 00234 00235 #define PARAM_ADC_START_ON_EDGE_OPT(OPT) ((OPT == ADC_START_ON_RISING)||(OPT == ADC_START_ON_FALLING)) 00236 00237 #define PARAM_ADC_DATA_STATUS(OPT) ((OPT== ADC_DATA_BURST)||(OPT== ADC_DATA_DONE)) 00238 00239 #define PARAM_ADC_FREQUENCY(FRE) (FRE <= 13000000 ) 00240 00241 #define PARAM_ADC_CHANNEL_SELECTION(SEL) ((SEL == ADC_CHANNEL_0)||(ADC_CHANNEL_1)\ 00242 ||(SEL == ADC_CHANNEL_2)|(ADC_CHANNEL_3)\ 00243 ||(SEL == ADC_CHANNEL_4)||(ADC_CHANNEL_5)\ 00244 ||(SEL == ADC_CHANNEL_6)||(ADC_CHANNEL_7)) 00245 00246 #define PARAM_ADC_START_OPT(OPT) ((OPT == ADC_START_CONTINUOUS)||(OPT == ADC_START_NOW)\ 00247 ||(OPT == ADC_START_ON_EINT0)||(OPT == ADC_START_ON_CAP01)\ 00248 ||(OPT == ADC_START_ON_MAT01)||(OPT == ADC_START_ON_MAT03)\ 00249 ||(OPT == ADC_START_ON_MAT10)||(OPT == ADC_START_ON_MAT11)) 00250 00251 #define PARAM_ADC_TYPE_INT_OPT(OPT) ((OPT == ADC_ADINTEN0)||(OPT == ADC_ADINTEN1)\ 00252 ||(OPT == ADC_ADINTEN2)||(OPT == ADC_ADINTEN3)\ 00253 ||(OPT == ADC_ADINTEN4)||(OPT == ADC_ADINTEN5)\ 00254 ||(OPT == ADC_ADINTEN6)||(OPT == ADC_ADINTEN7)\ 00255 ||(OPT == ADC_ADGINTEN)) 00256 00257 #define PARAM_ADCx(n) (((uint32_t *)n)==((uint32_t *)LPC_ADC)) 00258 00259 /** 00260 * @} 00261 */ 00262 00263 00264 00265 /* Public Functions ----------------------------------------------------------- */ 00266 /** @defgroup ADC_Public_Functions 00267 * @{ 00268 */ 00269 00270 void ADC_Init(LPC_ADC_TypeDef *ADCx, uint32_t ConvFreq); 00271 void ADC_DeInit(LPC_ADC_TypeDef *ADCx); 00272 void ADC_BurstCmd(LPC_ADC_TypeDef *ADCx, FunctionalState NewState); 00273 void ADC_PowerdownCmd(LPC_ADC_TypeDef *ADCx, FunctionalState NewState); 00274 void ADC_StartCmd(LPC_ADC_TypeDef *ADCx, uint8_t start_mode); 00275 void ADC_EdgeStartConfig(LPC_ADC_TypeDef *ADCx, uint8_t EdgeOption); 00276 void ADC_IntConfig (LPC_ADC_TypeDef *ADCx, ADC_TYPE_INT_OPT IntType, FunctionalState NewState); 00277 void ADC_ChannelCmd (LPC_ADC_TypeDef *ADCx, uint8_t Channel, FunctionalState NewState); 00278 uint16_t ADC_ChannelGetData(LPC_ADC_TypeDef *ADCx, uint8_t channel); 00279 FlagStatus ADC_ChannelGetStatus(LPC_ADC_TypeDef *ADCx, uint8_t channel, uint32_t StatusType); 00280 uint16_t ADC_GlobalGetData(LPC_ADC_TypeDef *ADCx, uint8_t channel); 00281 FlagStatus ADC_GlobalGetStatus(LPC_ADC_TypeDef *ADCx, uint32_t StatusType); 00282 00283 /** 00284 * @} 00285 */ 00286 00287 00288 #ifdef __cplusplus 00289 } 00290 #endif 00291 00292 00293 #endif /* LPC17XX_ADC_H_ */ 00294 00295 /** 00296 * @} 00297 */ 00298 00299 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
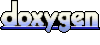