Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
socket.h
00001 /***************************************************************************** 00002 * 00003 * socket.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __SOCKET_H__ 00036 #define __SOCKET_H__ 00037 00038 #include "evnt_handler.h" 00039 00040 //***************************************************************************** 00041 // 00042 //! \addtogroup socket 00043 //! @{ 00044 // 00045 //***************************************************************************** 00046 00047 /** CC3000 Host driver - Socket API 00048 * 00049 */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 // BEWARE! When using send-non-blocking, always check the return value. 00056 // If -2, nothing was actually sent and you have to retry if you still want the data. 00057 //#define SEND_NON_BLOCKING 00058 00059 //Enable this flag if and only if you must comply with BSD socket 00060 //close() function 00061 #ifdef _API_USE_BSD_CLOSE 00062 #define close(sd) closesocket(sd) 00063 #endif 00064 00065 //Enable this flag if and only if you must comply with BSD socket read() and 00066 //write() functions 00067 #ifdef _API_USE_BSD_READ_WRITE 00068 #define read(sd, buf, len, flags) recv(sd, buf, len, flags) 00069 #define write(sd, buf, len, flags) send(sd, buf, len, flags) 00070 #endif 00071 00072 #define SOCKET_OPEN_PARAMS_LEN (12) 00073 #define SOCKET_CLOSE_PARAMS_LEN (4) 00074 #define SOCKET_ACCEPT_PARAMS_LEN (4) 00075 #define SOCKET_BIND_PARAMS_LEN (20) 00076 #define SOCKET_LISTEN_PARAMS_LEN (8) 00077 #define SOCKET_GET_HOST_BY_NAME_PARAMS_LEN (9) 00078 #define SOCKET_CONNECT_PARAMS_LEN (20) 00079 #define SOCKET_SELECT_PARAMS_LEN (44) 00080 #define SOCKET_SET_SOCK_OPT_PARAMS_LEN (20) 00081 #define SOCKET_GET_SOCK_OPT_PARAMS_LEN (12) 00082 #define SOCKET_RECV_FROM_PARAMS_LEN (12) 00083 #define SOCKET_SENDTO_PARAMS_LEN (24) 00084 #define SOCKET_MDNS_ADVERTISE_PARAMS_LEN (12) 00085 #define SOCKET_MAX_FREE_BUFFERS (6) 00086 00087 //#define NULL 0 00088 00089 // The legnth of arguments for the SEND command: sd + buff_offset + len + flags, 00090 // while size of each parameter is 32 bit - so the total length is 16 bytes; 00091 00092 #define HCI_CMND_SEND_ARG_LENGTH (16) 00093 #define SELECT_TIMEOUT_MIN_MICRO_SECONDS 5000 00094 #define HEADERS_SIZE_DATA (SPI_HEADER_SIZE + 5) 00095 #define SIMPLE_LINK_HCI_CMND_TRANSPORT_HEADER_SIZE (SPI_HEADER_SIZE + SIMPLE_LINK_HCI_CMND_HEADER_SIZE) 00096 #define MDNS_DEVICE_SERVICE_MAX_LENGTH (32) 00097 00098 00099 #define HOSTNAME_MAX_LENGTH (230) // 230 bytes + header shouldn't exceed 8 bit value 00100 00101 //--------- Address Families -------- 00102 00103 #define AF_INET 2 00104 #define AF_INET6 23 00105 00106 //------------ Socket Types ------------ 00107 00108 #define SOCK_STREAM 1 00109 #define SOCK_DGRAM 2 00110 #define SOCK_RAW 3 // Raw sockets allow new IPv4 protocols to be implemented in user space. A raw socket receives or sends the raw datagram not including link level headers 00111 #define SOCK_RDM 4 00112 #define SOCK_SEQPACKET 5 00113 00114 //----------- Socket Protocol ---------- 00115 00116 #define IPPROTO_IP 0 // dummy for IP 00117 #define IPPROTO_ICMP 1 // control message protocol 00118 #define IPPROTO_IPV4 IPPROTO_IP // IP inside IP 00119 #define IPPROTO_TCP 6 // tcp 00120 #define IPPROTO_UDP 17 // user datagram protocol 00121 #define IPPROTO_IPV6 41 // IPv6 in IPv6 00122 #define IPPROTO_NONE 59 // No next header 00123 #define IPPROTO_RAW 255 // raw IP packet 00124 #define IPPROTO_MAX 256 00125 00126 //----------- Socket retunr codes ----------- 00127 00128 #define SOC_ERROR (-1) // error 00129 #define SOC_IN_PROGRESS (-2) // socket in progress 00130 00131 //----------- Socket Options ----------- 00132 #define SOL_SOCKET 0xffff // socket level 00133 #define SOCKOPT_RECV_TIMEOUT 1 // optname to configure recv and recvfromtimeout 00134 #define SOCKOPT_NONBLOCK 2 // accept non block mode set SOCK_ON or SOCK_OFF (default block mode ) 00135 #define SOCK_ON 0 // socket non-blocking mode is enabled 00136 #define SOCK_OFF 1 // socket blocking mode is enabled 00137 00138 #define TCP_NODELAY 0x0001 00139 #define TCP_BSDURGENT 0x7000 00140 00141 #define MAX_PACKET_SIZE 1500 00142 #define MAX_LISTEN_QUEUE 4 00143 00144 #define IOCTL_SOCKET_EVENTMASK 00145 00146 #define ENOBUFS 55 // No buffer space available 00147 00148 #define __FD_SETSIZE 32 00149 00150 #define ASIC_ADDR_LEN 8 00151 00152 #define NO_QUERY_RECIVED -3 00153 00154 00155 typedef struct _in_addr_t 00156 { 00157 unsigned long s_addr; // load with inet_aton() 00158 } in_addr; 00159 00160 /*typedef struct _sockaddr_t 00161 { 00162 unsigned short int sa_family; 00163 unsigned char sa_data[14]; 00164 } sockaddr;*/ 00165 00166 typedef struct _sockaddr_in_t 00167 { 00168 short sin_family; // e.g. AF_INET 00169 unsigned short sin_port; // e.g. htons(3490) 00170 in_addr sin_addr; // see struct in_addr, below 00171 char sin_zero[8]; // zero this if you want to 00172 } sockaddr_in; 00173 00174 typedef unsigned long socklen_t; 00175 00176 // The fd_set member is required to be an array of longs. 00177 typedef long int __fd_mask; 00178 00179 // It's easier to assume 8-bit bytes than to get CHAR_BIT. 00180 #define __NFDBITS (8 * sizeof (__fd_mask)) 00181 #define __FDELT(d) ((d) / __NFDBITS) 00182 #define __FDMASK(d) ((__fd_mask) 1 << ((d) % __NFDBITS)) 00183 00184 // fd_set for select and pselect. 00185 typedef struct 00186 { 00187 __fd_mask fds_bits[__FD_SETSIZE / __NFDBITS]; 00188 #define __FDS_BITS(set) ((set)->fds_bits) 00189 } fd_set; 00190 00191 // We don't use `memset' because this would require a prototype and 00192 // the array isn't too big. 00193 #define __FD_ZERO(set) \ 00194 do { \ 00195 unsigned int __i; \ 00196 fd_set *__arr = (set); \ 00197 for (__i = 0; __i < sizeof (fd_set) / sizeof (__fd_mask); ++__i) \ 00198 __FDS_BITS (__arr)[__i] = 0; \ 00199 } while (0) 00200 #define __FD_SET(d, set) (__FDS_BITS (set)[__FDELT (d)] |= __FDMASK (d)) 00201 #define __FD_CLR(d, set) (__FDS_BITS (set)[__FDELT (d)] &= ~__FDMASK (d)) 00202 #define __FD_ISSET(d, set) (__FDS_BITS (set)[__FDELT (d)] & __FDMASK (d)) 00203 00204 // Access macros for 'fd_set'. 00205 #define FD_SET(fd, fdsetp) __FD_SET (fd, fdsetp) 00206 #define FD_CLR(fd, fdsetp) __FD_CLR (fd, fdsetp) 00207 #define FD_ISSET(fd, fdsetp) __FD_ISSET (fd, fdsetp) 00208 #define FD_ZERO(fdsetp) __FD_ZERO (fdsetp) 00209 00210 //Use in case of Big Endian only 00211 00212 #define htonl(A) ((((unsigned long)(A) & 0xff000000) >> 24) | \ 00213 (((unsigned long)(A) & 0x00ff0000) >> 8) | \ 00214 (((unsigned long)(A) & 0x0000ff00) << 8) | \ 00215 (((unsigned long)(A) & 0x000000ff) << 24)) 00216 00217 #define ntohl htonl 00218 00219 //Use in case of Big Endian only 00220 #define htons(A) ((((unsigned long)(A) & 0xff00) >> 8) | \ 00221 (((unsigned long)(A) & 0x00ff) << 8)) 00222 00223 00224 #define ntohs htons 00225 00226 // mDNS port - 5353 mDNS multicast address - 224.0.0.251 00227 #define SET_mDNS_ADD(sockaddr) sockaddr.sa_data[0] = 0x14; \ 00228 sockaddr.sa_data[1] = 0xe9; \ 00229 sockaddr.sa_data[2] = 0xe0; \ 00230 sockaddr.sa_data[3] = 0x0; \ 00231 sockaddr.sa_data[4] = 0x0; \ 00232 sockaddr.sa_data[5] = 0xfb; 00233 00234 00235 //***************************************************************************** 00236 // 00237 // Prototypes for the APIs. 00238 // 00239 //***************************************************************************** 00240 00241 /** 00242 * HostFlowControlConsumeBuff. 00243 * if SEND_NON_BLOCKING is not defined - block until a free buffer is available,\n 00244 * otherwise return the status of the available buffers.\n 00245 * @param sd socket descriptor 00246 * @return 0 in case there are buffers available, \n 00247 * -1 in case of bad socket\n 00248 * -2 if there are no free buffers present (only when SEND_NON_BLOCKING is enabled)\n 00249 */ 00250 int HostFlowControlConsumeBuff(int sd); 00251 00252 /** 00253 * create an endpoint for communication. 00254 * The socket function creates a socket that is bound to a specific transport service provider.\n 00255 * This function is called by the application layer to obtain a socket handle.\n 00256 * @param domain selects the protocol family which will be used for\n 00257 * communication. On this version only AF_INET is supported\n 00258 * @param type specifies the communication semantics. On this version\n 00259 * only SOCK_STREAM, SOCK_DGRAM, SOCK_RAW are supported\n 00260 * @param protocol specifies a particular protocol to be used with the\n 00261 * socket IPPROTO_TCP, IPPROTO_UDP or IPPROTO_RAW are supported.\n 00262 * @return On success, socket handle that is used for consequent socket operations\n 00263 * On error, -1 is returned.\n 00264 */ 00265 extern int socket(long domain, long type, long protocol); 00266 00267 /** 00268 * The socket function closes a created socket. 00269 * @param sd socket handle. 00270 * @return On success, zero is returned. On error, -1 is returned. 00271 */ 00272 extern long closesocket(long sd); 00273 00274 /** 00275 * accept a connection on a socket. 00276 * This function is used with connection-based socket types\n 00277 * (SOCK_STREAM). It extracts the first connection request on the\n 00278 * queue of pending connections, creates a new connected socket, and\n 00279 * returns a new file descriptor referring to that socket.\n 00280 * The newly created socket is not in the listening state.\n 00281 * The original socket sd is unaffected by this call.\n 00282 * The argument sd is a socket that has been created with socket(),\n 00283 * bound to a local address with bind(), and is listening for \n 00284 * connections after a listen(). The argument addr is a pointer \n 00285 * to a sockaddr structure. This structure is filled in with the \n 00286 * address of the peer socket, as known to the communications layer.\n 00287 * The exact format of the address returned addr is determined by the \n 00288 * socket's address family. The addrlen argument is a value-result\n 00289 * argument: it should initially contain the size of the structure\n 00290 * pointed to by addr, on return it will contain the actual\n 00291 * length (in bytes) of the address returned.\n 00292 * @param[in] sd socket descriptor (handle)\n 00293 * @param[out] addr the argument addr is a pointer to a sockaddr structure\n 00294 * This structure is filled in with the address of the \n 00295 * peer socket, as known to the communications layer. \n 00296 * determined. The exact format of the address returned \n 00297 * addr is by the socket's address sockaddr. \n 00298 * On this version only AF_INET is supported.\n 00299 * This argument returns in network order.\n 00300 * @param[out] addrlen the addrlen argument is a value-result argument: \n 00301 * it should initially contain the size of the structure\n 00302 * pointed to by addr.\n 00303 * @return For socket in blocking mode:\n 00304 * - On success, socket handle. on failure negative\n 00305 * For socket in non-blocking mode:\n 00306 * - On connection establishment, socket handle\n 00307 * - On connection pending, SOC_IN_PROGRESS (-2)\n 00308 * - On failure, SOC_ERROR (-1)\n 00309 * @sa socket ; bind ; listen 00310 */ 00311 extern long accept(long sd, sockaddr *addr, socklen_t *addrlen); 00312 00313 /** 00314 * assign a name to a socket. 00315 * This function gives the socket the local address addr.\n 00316 * addr is addrlen bytes long. Traditionally, this is called when a \n 00317 * socket is created with socket, it exists in a name space (address \n 00318 * family) but has no name assigned.\n 00319 * It is necessary to assign a local address before a SOCK_STREAM\n 00320 * socket may receive connections.\n 00321 * @param[in] sd socket descriptor (handle) 00322 * @param[out] addr specifies the destination address. On this version\n 00323 * only AF_INET is supported.\n 00324 * @param[out] addrlen contains the size of the structure pointed to by addr.\n 00325 * @return On success, zero is returned.\n 00326 * On error, -1 is returned.\n 00327 * @sa socket ; accept ; listen 00328 */ 00329 extern long bind(long sd, const sockaddr *addr, long addrlen); 00330 00331 /** 00332 * listen for connections on a socket. 00333 * The willingness to accept incoming connections and a queue\n 00334 * limit for incoming connections are specified with listen(),\n 00335 * and then the connections are accepted with accept.\n 00336 * The listen() call applies only to sockets of type SOCK_STREAM\n 00337 * The backlog parameter defines the maximum length the queue of\n 00338 * pending connections may grow to. \n 00339 * @param[in] sd socket descriptor (handle) 00340 * @param[in] backlog specifies the listen queue depth. On this version\n 00341 * backlog is not supported.\n 00342 * @return On success, zero is returned.\n 00343 * On error, -1 is returned.\n 00344 * @sa socket ; accept ; bind 00345 * @note On this version, backlog is not supported 00346 */ 00347 extern long listen(long sd, long backlog); 00348 00349 /** 00350 * Get host IP by name.\n 00351 * Obtain the IP Address of machine on network\n 00352 * @param[in] hostname host name 00353 * @param[in] usNameLen name length 00354 * @param[out] out_ip_addr This parameter is filled in with host IP address.\n 00355 * In case that host name is not resolved, \n 00356 * out_ip_addr is zero.\n 00357 * @return On success, positive is returned.\n 00358 * On error, negative is returned by its name.\n 00359 * @note On this version, only blocking mode is supported. Also note that\n 00360 * The function requires DNS server to be configured prior to its usage.\n 00361 */ 00362 #ifndef CC3000_TINY_DRIVER 00363 extern int gethostbyname(char * hostname, unsigned short usNameLen, unsigned long* out_ip_addr); 00364 #endif 00365 00366 00367 /** 00368 * initiate a connection on a socket. 00369 * Function connects the socket referred to by the socket descriptor\n 00370 * sd, to the address specified by addr. The addrlen argument \n 00371 * specifies the size of addr. The format of the address in addr is \n 00372 * determined by the address space of the socket. If it is of type \n 00373 * SOCK_DGRAM, this call specifies the peer with which the socket is \n 00374 * to be associated; this address is that to which datagrams are to be\n 00375 * sent, and the only address from which datagrams are to be received. \n 00376 * If the socket is of type SOCK_STREAM, this call attempts to make a \n 00377 * connection to another socket. The other socket is specified by \n 00378 * address, which is an address in the communications space of the\n 00379 * socket. Note that the function implements only blocking behavior \n 00380 * thus the caller will be waiting either for the connection \n 00381 * establishment or for the connection establishment failure.\n 00382 * @param[in] sd socket descriptor (handle) 00383 * @param[in] addr specifies the destination addr. On this version\n 00384 * only AF_INET is supported.\n 00385 * @param[out] addrlen contains the size of the structure pointed to by addr 00386 * @return On success, zero is returned.\n 00387 On error, -1 is returned\n 00388 * @sa socket 00389 */ 00390 extern long connect(long sd, const sockaddr *addr, long addrlen); 00391 00392 /** 00393 * Monitor socket activity. 00394 * Select allow a program to monitor multiple file descriptors,\n 00395 * waiting until one or more of the file descriptors become \n 00396 *"ready" for some class of I/O operation \n 00397 * @param[in] nfds the highest-numbered file descriptor in any of the\n 00398 * three sets, plus 1. \n 00399 * @param[out] writesds socket descriptors list for write monitoring\n 00400 * @param[out] readsds socket descriptors list for read monitoring\n 00401 * @param[out] exceptsds socket descriptors list for exception monitoring\n 00402 * @param[in] timeout is an upper bound on the amount of time elapsed\n 00403 * before select() returns. Null means infinity \n 00404 * timeout. The minimum timeout is 5 milliseconds,\n 00405 * less than 5 milliseconds will be set\n 00406 * automatically to 5 milliseconds.\n 00407 * @return On success, select() returns the number of file descriptors\n 00408 * contained in the three returned descriptor sets (that is, the\n 00409 * total number of bits that are set in readfds, writefds,\n 00410 * exceptfds) which may be zero if the timeout expires before\n 00411 * anything interesting happens.\n 00412 * On error, -1 is returned.\n 00413 * *readsds - return the sockets on which Read request will\n 00414 * return without delay with valid data.\n 00415 * *writesds - return the sockets on which Write request \n 00416 * will return without delay.\n 00417 * *exceptsds - return the sockets which closed recently.\n 00418 * @Note If the timeout value set to less than 5ms it will automatically\n 00419 * change to 5ms to prevent overload of the system\n 00420 * @sa socket 00421 */ 00422 extern int select(long nfds, fd_set *readsds, fd_set *writesds, fd_set *exceptsds, struct timeval *timeout); 00423 00424 /** 00425 * set socket options. 00426 * This function manipulate the options associated with a socket.\n 00427 * Options may exist at multiple protocol levels; they are always\n 00428 * present at the uppermost socket level.\n 00429 * When manipulating socket options the level at which the option \n 00430 * resides and the name of the option must be specified.\n 00431 * To manipulate options at the socket level, level is specified as\n 00432 * SOL_SOCKET. To manipulate options at any other level the protocol \n 00433 * number of the appropriate protocol controlling the option is \n 00434 * supplied. For example, to indicate that an option is to be \n 00435 * interpreted by the TCP protocol, level should be set to the \n 00436 * protocol number of TCP; \n 00437 * The parameters optval and optlen are used to access optval - \n 00438 * use for setsockopt(). For getsockopt() they identify a buffer\n 00439 * in which the value for the requested option(s) are to \n 00440 * be returned. For getsockopt(), optlen is a value-result \n 00441 * parameter, initially containing the size of the buffer \n 00442 * pointed to by option_value, and modified on return to \n 00443 * indicate the actual size of the value returned. If no option \n 00444 * value is to be supplied or returned, option_value may be NULL.\n 00445 * @param[in] sd socket handle 00446 * @param[in] level defines the protocol level for this option 00447 * @param[in] optname defines the option name to Interrogate 00448 * @param[in] optval specifies a value for the option 00449 * @param[in] optlen specifies the length of the option value 00450 * @return On success, zero is returned.\n 00451 On error, -1 is returned\n 00452 * 00453 * @Note On this version the following two socket options are enabled:\n 00454 * The only protocol level supported in this version is SOL_SOCKET (level).\n 00455 * 1. SOCKOPT_RECV_TIMEOUT (optname)\n 00456 * SOCKOPT_RECV_TIMEOUT configures recv and recvfrom timeout in milliseconds.\n 00457 * In that case optval should be pointer to unsigned long.\n 00458 * 2. SOCKOPT_NONBLOCK (optname). sets the socket non-blocking mode on or off.\n 00459 * In that case optval should be SOCK_ON or SOCK_OFF (optval).\n 00460 * @sa getsockopt 00461 */ 00462 #ifndef CC3000_TINY_DRIVER 00463 extern int setsockopt(long sd, long level, long optname, const void *optval, socklen_t optlen); 00464 #endif 00465 00466 /** 00467 * get socket options. 00468 * This function manipulate the options associated with a socket.\n 00469 * Options may exist at multiple protocol levels; they are always\n 00470 * present at the uppermost socket level.\n 00471 * When manipulating socket options the level at which the option \n 00472 * resides and the name of the option must be specified. \n 00473 * To manipulate options at the socket level, level is specified as \n 00474 * SOL_SOCKET. To manipulate options at any other level the protocol \n 00475 * number of the appropriate protocol controlling the option is \n 00476 * supplied. For example, to indicate that an option is to be \n 00477 * interpreted by the TCP protocol, level should be set to the \n 00478 * protocol number of TCP; \n 00479 * The parameters optval and optlen are used to access optval -\n 00480 * use for setsockopt(). For getsockopt() they identify a buffer\n 00481 * in which the value for the requested option(s) are to \n 00482 * be returned. For getsockopt(), optlen is a value-result \n 00483 * parameter, initially containing the size of the buffer \n 00484 * pointed to by option_value, and modified on return to \n 00485 * indicate the actual size of the value returned. If no option \n 00486 * value is to be supplied or returned, option_value may be NULL.\n 00487 * @param[in] sd socket handle 00488 * @param[in] level defines the protocol level for this option 00489 * @param[in] optname defines the option name to Interrogate 00490 * @param[out] optval specifies a value for the option 00491 * @param[out] optlen specifies the length of the option value 00492 * @return On success, zero is returned. On error, -1 is returned 00493 * 00494 * @Note On this version the following two socket options are enabled:\n 00495 * The only protocol level supported in this version is SOL_SOCKET (level).\n 00496 * 1. SOCKOPT_RECV_TIMEOUT (optname)\n 00497 * SOCKOPT_RECV_TIMEOUT configures recv and recvfrom timeout in milliseconds.\n 00498 * In that case optval should be pointer to unsigned long.\n 00499 * 2. SOCKOPT_NONBLOCK (optname). sets the socket non-blocking mode on or off.\n 00500 * In that case optval should be SOCK_ON or SOCK_OFF (optval).\n 00501 * @sa setsockopt 00502 */ 00503 extern int getsockopt(long sd, long level, long optname, void *optval, socklen_t *optlen); 00504 00505 /** 00506 * Read data from socket (simple_link_recv). 00507 * Return the length of the message on successful completion.\n 00508 * If a message is too long to fit in the supplied buffer, excess bytes may\n 00509 * be discarded depending on the type of socket the message is received from.\n 00510 * @param sd socket handle 00511 * @param buf read buffer 00512 * @param len buffer length 00513 * @param flags indicates blocking or non-blocking operation 00514 * @param from pointer to an address structure indicating source address 00515 * @param fromlen source address structure size 00516 * @return Return the number of bytes received, or -1 if an error occurred 00517 */ 00518 int simple_link_recv(long sd, void *buf, long len, long flags, sockaddr *from, socklen_t *fromlen, long opcode); 00519 00520 /** 00521 * Receive a message from a connection-mode socket. 00522 * @param[in] sd socket handle 00523 * @param[out] buf Points to the buffer where the message should be stored 00524 * @param[in] len Specifies the length in bytes of the buffer pointed to \n 00525 * by the buffer argument.\n 00526 * @param[in] flags Specifies the type of message reception. \n 00527 * On this version, this parameter is not supported.\n 00528 * @return Return the number of bytes received, or -1 if an error occurred 00529 * @sa recvfrom 00530 * @Note On this version, only blocking mode is supported. 00531 */ 00532 extern int recv(long sd, void *buf, long len, long flags); 00533 00534 /** 00535 * read data from socket (recvfrom). 00536 * Receives a message from a connection-mode or connectionless-mode socket.\n 00537 * Note that raw sockets are not supported.\n 00538 * @param[in] sd socket handle 00539 * @param[out] buf Points to the buffer where the message should be stored 00540 * @param[in] len Specifies the length in bytes of the buffer pointed to \n 00541 * by the buffer argument.\n 00542 * @param[in] flags Specifies the type of message reception.\n 00543 * On this version, this parameter is not supported.\n 00544 * @param[in] from pointer to an address structure indicating the source\n 00545 * address: sockaddr. On this version only AF_INET is\n 00546 * supported.\n 00547 * @param[in] fromlen source address structure size 00548 * @return Return the number of bytes received, or -1 if an error occurred 00549 * @sa recv 00550 * @Note On this version, only blocking mode is supported. 00551 */ 00552 extern int recvfrom(long sd, void *buf, long len, long flags, sockaddr *from, socklen_t *fromlen); 00553 00554 /** 00555 * Transmit a message to another socket (simple_link_send). 00556 * @param sd socket handle 00557 * @param buf write buffer 00558 * @param len buffer length 00559 * @param flags On this version, this parameter is not supported 00560 * @param to pointer to an address structure indicating destination address 00561 * @param tolen destination address structure size 00562 * @return Return the number of bytes transmitted, or -1 if an error\n 00563 * occurred, or -2 in case there are no free buffers available\n 00564 * (only when SEND_NON_BLOCKING is enabled)\n 00565 */ 00566 int simple_link_send(long sd, const void *buf, long len, long flags, const sockaddr *to, long tolen, long opcode); 00567 00568 /** 00569 * Transmit a message to another socket (send). 00570 * @param sd socket handle 00571 * @param buf Points to a buffer containing the message to be sent 00572 * @param len message size in bytes 00573 * @param flags On this version, this parameter is not supported 00574 * @return Return the number of bytes transmitted, or -1 if an\n 00575 * error occurred\n 00576 * @Note On this version, only blocking mode is supported. 00577 * @sa sendto 00578 */ 00579 extern int send(long sd, const void *buf, long len, long flags); 00580 00581 /** 00582 * Transmit a message to another socket (sendto). 00583 * @param sd socket handle 00584 * @param buf Points to a buffer containing the message to be sent 00585 * @param len message size in bytes 00586 * @param flags On this version, this parameter is not supported 00587 * @param to pointer to an address structure indicating the destination\n 00588 * address: sockaddr. On this version only AF_INET is\n 00589 * supported.\n 00590 * @param tolen destination address structure size 00591 * @return Return the number of bytes transmitted, or -1 if an error occurred 00592 * @Note On this version, only blocking mode is supported. 00593 * @sa send 00594 */ 00595 extern int sendto(long sd, const void *buf, long len, long flags, const sockaddr *to, socklen_t tolen); 00596 00597 /** 00598 * Set CC3000 in mDNS advertiser mode in order to advertise itself. 00599 * @param[in] mdnsEnabled flag to enable/disable the mDNS feature 00600 * @param[in] deviceServiceName Service name as part of the published\n 00601 * canonical domain name\n 00602 * @param[in] deviceServiceNameLength Length of the service name 00603 * @return On success, zero is returned,\n 00604 * return SOC_ERROR if socket was not opened successfully, or if an error occurred.\n 00605 */ 00606 extern int mdnsAdvertiser(unsigned short mdnsEnabled, char * deviceServiceName, unsigned short deviceServiceNameLength); 00607 00608 00609 #ifdef __cplusplus 00610 } 00611 #endif // __cplusplus 00612 00613 //***************************************************************************** 00614 // 00615 // Close the Doxygen group. 00616 //! @} 00617 // 00618 //***************************************************************************** 00619 00620 #endif // __SOCKET_H__ 00621 00622 00623 00624 00625
Generated on Wed Jul 13 2022 18:31:31 by
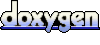