Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
Socket
Functions | |
int | HostFlowControlConsumeBuff (int sd) |
HostFlowControlConsumeBuff. | |
int | socket (long domain, long type, long protocol) |
create an endpoint for communication. | |
long | closesocket (long sd) |
The socket function closes a created socket. | |
long | accept (long sd, sockaddr *addr, socklen_t *addrlen) |
accept a connection on a socket. | |
long | bind (long sd, const sockaddr *addr, long addrlen) |
assign a name to a socket. | |
long | listen (long sd, long backlog) |
listen for connections on a socket. | |
int | gethostbyname (char *hostname, unsigned short usNameLen, unsigned long *out_ip_addr) |
Get host IP by name. | |
long | connect (long sd, const sockaddr *addr, long addrlen) |
initiate a connection on a socket. | |
int | select (long nfds, fd_set *readsds, fd_set *writesds, fd_set *exceptsds, struct timeval *timeout) |
Monitor socket activity. | |
int | setsockopt (long sd, long level, long optname, const void *optval, socklen_t optlen) |
set socket options. | |
int | getsockopt (long sd, long level, long optname, void *optval, socklen_t *optlen) |
get socket options. | |
int | simple_link_recv (long sd, void *buf, long len, long flags, sockaddr *from, socklen_t *fromlen, long opcode) |
Read data from socket (simple_link_recv). | |
int | recv (long sd, void *buf, long len, long flags) |
Receive a message from a connection-mode socket. | |
int | recvfrom (long sd, void *buf, long len, long flags, sockaddr *from, socklen_t *fromlen) |
read data from socket (recvfrom). | |
int | simple_link_send (long sd, const void *buf, long len, long flags, const sockaddr *to, long tolen, long opcode) |
Transmit a message to another socket (simple_link_send). | |
int | send (long sd, const void *buf, long len, long flags) |
Transmit a message to another socket (send). | |
int | sendto (long sd, const void *buf, long len, long flags, const sockaddr *to, socklen_t tolen) |
Transmit a message to another socket (sendto). | |
int | mdnsAdvertiser (unsigned short mdnsEnabled, char *deviceServiceName, unsigned short deviceServiceNameLength) |
Set CC3000 in mDNS advertiser mode in order to advertise itself. |
Function Documentation
long accept | ( | long | sd, |
sockaddr * | addr, | ||
socklen_t * | addrlen | ||
) |
accept a connection on a socket.
This function is used with connection-based socket types
(SOCK_STREAM). It extracts the first connection request on the
queue of pending connections, creates a new connected socket, and
returns a new file descriptor referring to that socket.
The newly created socket is not in the listening state.
The original socket sd is unaffected by this call.
The argument sd is a socket that has been created with socket(),
bound to a local address with bind(), and is listening for
connections after a listen(). The argument addr is a pointer
to a sockaddr structure. This structure is filled in with the
address of the peer socket, as known to the communications layer.
The exact format of the address returned addr is determined by the
socket's address family. The addrlen argument is a value-result
argument: it should initially contain the size of the structure
pointed to by addr, on return it will contain the actual
length (in bytes) of the address returned.
- Parameters:
-
[in] sd socket descriptor (handle)
[out] addr the argument addr is a pointer to a sockaddr structure
This structure is filled in with the address of the
peer socket, as known to the communications layer.
determined. The exact format of the address returned
addr is by the socket's address sockaddr.
On this version only AF_INET is supported.
This argument returns in network order.
[out] addrlen the addrlen argument is a value-result argument:
it should initially contain the size of the structure
pointed to by addr.
- Returns:
- For socket in blocking mode:
- On success, socket handle. on failure negative
For socket in non-blocking mode:
- On connection establishment, socket handle
- On connection pending, SOC_IN_PROGRESS (-2)
- On failure, SOC_ERROR (-1)
- On success, socket handle. on failure negative
Definition at line 147 of file socket.cpp.
long bind | ( | long | sd, |
const sockaddr * | addr, | ||
long | addrlen | ||
) |
assign a name to a socket.
This function gives the socket the local address addr.
addr is addrlen bytes long. Traditionally, this is called when a
socket is created with socket, it exists in a name space (address
family) but has no name assigned.
It is necessary to assign a local address before a SOCK_STREAM
socket may receive connections.
- Parameters:
-
[in] sd socket descriptor (handle) [out] addr specifies the destination address. On this version
only AF_INET is supported.
[out] addrlen contains the size of the structure pointed to by addr.
- Returns:
- On success, zero is returned.
On error, -1 is returned.
Definition at line 187 of file socket.cpp.
long closesocket | ( | long | sd ) |
The socket function closes a created socket.
- Parameters:
-
sd socket handle.
- Returns:
- On success, zero is returned. On error, -1 is returned.
Definition at line 118 of file socket.cpp.
long connect | ( | long | sd, |
const sockaddr * | addr, | ||
long | addrlen | ||
) |
initiate a connection on a socket.
Function connects the socket referred to by the socket descriptor
sd, to the address specified by addr. The addrlen argument
specifies the size of addr. The format of the address in addr is
determined by the address space of the socket. If it is of type
SOCK_DGRAM, this call specifies the peer with which the socket is
to be associated; this address is that to which datagrams are to be
sent, and the only address from which datagrams are to be received.
If the socket is of type SOCK_STREAM, this call attempts to make a
connection to another socket. The other socket is specified by
address, which is an address in the communications space of the
socket. Note that the function implements only blocking behavior
thus the caller will be waiting either for the connection
establishment or for the connection establishment failure.
- Parameters:
-
[in] sd socket descriptor (handle) [in] addr specifies the destination addr. On this version
only AF_INET is supported.
[out] addrlen contains the size of the structure pointed to by addr
- Returns:
- On success, zero is returned.
On error, -1 is returned
- See also:
- socket
Definition at line 277 of file socket.cpp.
int gethostbyname | ( | char * | hostname, |
unsigned short | usNameLen, | ||
unsigned long * | out_ip_addr | ||
) |
Get host IP by name.
Obtain the IP Address of machine on network
- Parameters:
-
[in] hostname host name [in] usNameLen name length [out] out_ip_addr This parameter is filled in with host IP address.
In case that host name is not resolved,
out_ip_addr is zero.
- Returns:
- On success, positive is returned.
On error, negative is returned by its name.
- Note:
- On this version, only blocking mode is supported. Also note that
The function requires DNS server to be configured prior to its usage.
Definition at line 241 of file socket.cpp.
int getsockopt | ( | long | sd, |
long | level, | ||
long | optname, | ||
void * | optval, | ||
socklen_t * | optlen | ||
) |
get socket options.
This function manipulate the options associated with a socket.
Options may exist at multiple protocol levels; they are always
present at the uppermost socket level.
When manipulating socket options the level at which the option
resides and the name of the option must be specified.
To manipulate options at the socket level, level is specified as
SOL_SOCKET. To manipulate options at any other level the protocol
number of the appropriate protocol controlling the option is
supplied. For example, to indicate that an option is to be
interpreted by the TCP protocol, level should be set to the
protocol number of TCP;
The parameters optval and optlen are used to access optval -
use for setsockopt(). For getsockopt() they identify a buffer
in which the value for the requested option(s) are to
be returned. For getsockopt(), optlen is a value-result
parameter, initially containing the size of the buffer
pointed to by option_value, and modified on return to
indicate the actual size of the value returned. If no option
value is to be supplied or returned, option_value may be NULL.
- Parameters:
-
[in] sd socket handle [in] level defines the protocol level for this option [in] optname defines the option name to Interrogate [out] optval specifies a value for the option [out] optlen specifies the length of the option value
- Returns:
- On success, zero is returned. On error, -1 is returned
On this version the following two socket options are enabled:
The only protocol level supported in this version is SOL_SOCKET (level).
1. SOCKOPT_RECV_TIMEOUT (optname)
SOCKOPT_RECV_TIMEOUT configures recv and recvfrom timeout in milliseconds.
In that case optval should be pointer to unsigned long.
2. SOCKOPT_NONBLOCK (optname). sets the socket non-blocking mode on or off.
In that case optval should be SOCK_ON or SOCK_OFF (optval).
- See also:
- setsockopt
Definition at line 416 of file socket.cpp.
int HostFlowControlConsumeBuff | ( | int | sd ) |
HostFlowControlConsumeBuff.
if SEND_NON_BLOCKING is not defined - block until a free buffer is available,
otherwise return the status of the available buffers.
- Parameters:
-
sd socket descriptor
- Returns:
- 0 in case there are buffers available,
-1 in case of bad socket
-2 if there are no free buffers present (only when SEND_NON_BLOCKING is enabled)
Definition at line 38 of file socket.cpp.
long listen | ( | long | sd, |
long | backlog | ||
) |
listen for connections on a socket.
The willingness to accept incoming connections and a queue
limit for incoming connections are specified with listen(),
and then the connections are accepted with accept.
The listen() call applies only to sockets of type SOCK_STREAM
The backlog parameter defines the maximum length the queue of
pending connections may grow to.
- Parameters:
-
[in] sd socket descriptor (handle) [in] backlog specifies the listen queue depth. On this version
backlog is not supported.
- Returns:
- On success, zero is returned.
On error, -1 is returned.
- Note:
- On this version, backlog is not supported
Definition at line 216 of file socket.cpp.
int mdnsAdvertiser | ( | unsigned short | mdnsEnabled, |
char * | deviceServiceName, | ||
unsigned short | deviceServiceNameLength | ||
) |
Set CC3000 in mDNS advertiser mode in order to advertise itself.
- Parameters:
-
[in] mdnsEnabled flag to enable/disable the mDNS feature [in] deviceServiceName Service name as part of the published
canonical domain name
[in] deviceServiceNameLength Length of the service name
- Returns:
- On success, zero is returned,
return SOC_ERROR if socket was not opened successfully, or if an error occurred.
Definition at line 586 of file socket.cpp.
int recv | ( | long | sd, |
void * | buf, | ||
long | len, | ||
long | flags | ||
) |
Receive a message from a connection-mode socket.
- Parameters:
-
[in] sd socket handle [out] buf Points to the buffer where the message should be stored [in] len Specifies the length in bytes of the buffer pointed to
by the buffer argument.
[in] flags Specifies the type of message reception.
On this version, this parameter is not supported.
- Returns:
- Return the number of bytes received, or -1 if an error occurred
- See also:
- recvfrom On this version, only blocking mode is supported.
Definition at line 482 of file socket.cpp.
int recvfrom | ( | long | sd, |
void * | buf, | ||
long | len, | ||
long | flags, | ||
sockaddr * | from, | ||
socklen_t * | fromlen | ||
) |
read data from socket (recvfrom).
Receives a message from a connection-mode or connectionless-mode socket.
Note that raw sockets are not supported.
- Parameters:
-
[in] sd socket handle [out] buf Points to the buffer where the message should be stored [in] len Specifies the length in bytes of the buffer pointed to
by the buffer argument.
[in] flags Specifies the type of message reception.
On this version, this parameter is not supported.
[in] from pointer to an address structure indicating the source
address: sockaddr. On this version only AF_INET is
supported.
[in] fromlen source address structure size
- Returns:
- Return the number of bytes received, or -1 if an error occurred
- See also:
- recv On this version, only blocking mode is supported.
Definition at line 488 of file socket.cpp.
int select | ( | long | nfds, |
fd_set * | readsds, | ||
fd_set * | writesds, | ||
fd_set * | exceptsds, | ||
struct timeval * | timeout | ||
) |
Monitor socket activity.
Select allow a program to monitor multiple file descriptors,
waiting until one or more of the file descriptors become
"ready" for some class of I/O operation
- Parameters:
-
[in] nfds the highest-numbered file descriptor in any of the
three sets, plus 1.
[out] writesds socket descriptors list for write monitoring
[out] readsds socket descriptors list for read monitoring
[out] exceptsds socket descriptors list for exception monitoring
[in] timeout is an upper bound on the amount of time elapsed
before select() returns. Null means infinity
timeout. The minimum timeout is 5 milliseconds,
less than 5 milliseconds will be set
automatically to 5 milliseconds.
- Returns:
- On success, select() returns the number of file descriptors
contained in the three returned descriptor sets (that is, the
total number of bits that are set in readfds, writefds,
exceptfds) which may be zero if the timeout expires before
anything interesting happens.
On error, -1 is returned.
*readsds - return the sockets on which Read request will
return without delay with valid data.
*writesds - return the sockets on which Write request
will return without delay.
*exceptsds - return the sockets which closed recently.
If the timeout value set to less than 5ms it will automatically
change to 5ms to prevent overload of the system
- See also:
- socket
Definition at line 305 of file socket.cpp.
int send | ( | long | sd, |
const void * | buf, | ||
long | len, | ||
long | flags | ||
) |
Transmit a message to another socket (send).
- Parameters:
-
sd socket handle buf Points to a buffer containing the message to be sent len message size in bytes flags On this version, this parameter is not supported
- Returns:
- Return the number of bytes transmitted, or -1 if an
error occurred
On this version, only blocking mode is supported.
- See also:
- sendto
Definition at line 574 of file socket.cpp.
int sendto | ( | long | sd, |
const void * | buf, | ||
long | len, | ||
long | flags, | ||
const sockaddr * | to, | ||
socklen_t | tolen | ||
) |
Transmit a message to another socket (sendto).
- Parameters:
-
sd socket handle buf Points to a buffer containing the message to be sent len message size in bytes flags On this version, this parameter is not supported to pointer to an address structure indicating the destination
address: sockaddr. On this version only AF_INET is
supported.
tolen destination address structure size
- Returns:
- Return the number of bytes transmitted, or -1 if an error occurred On this version, only blocking mode is supported.
- See also:
- send
Definition at line 580 of file socket.cpp.
int setsockopt | ( | long | sd, |
long | level, | ||
long | optname, | ||
const void * | optval, | ||
socklen_t | optlen | ||
) |
set socket options.
This function manipulate the options associated with a socket.
Options may exist at multiple protocol levels; they are always
present at the uppermost socket level.
When manipulating socket options the level at which the option
resides and the name of the option must be specified.
To manipulate options at the socket level, level is specified as
SOL_SOCKET. To manipulate options at any other level the protocol
number of the appropriate protocol controlling the option is
supplied. For example, to indicate that an option is to be
interpreted by the TCP protocol, level should be set to the
protocol number of TCP;
The parameters optval and optlen are used to access optval -
use for setsockopt(). For getsockopt() they identify a buffer
in which the value for the requested option(s) are to
be returned. For getsockopt(), optlen is a value-result
parameter, initially containing the size of the buffer
pointed to by option_value, and modified on return to
indicate the actual size of the value returned. If no option
value is to be supplied or returned, option_value may be NULL.
- Parameters:
-
[in] sd socket handle [in] level defines the protocol level for this option [in] optname defines the option name to Interrogate [in] optval specifies a value for the option [in] optlen specifies the length of the option value
- Returns:
- On success, zero is returned.
On error, -1 is returned
On this version the following two socket options are enabled:
The only protocol level supported in this version is SOL_SOCKET (level).
1. SOCKOPT_RECV_TIMEOUT (optname)
SOCKOPT_RECV_TIMEOUT configures recv and recvfrom timeout in milliseconds.
In that case optval should be pointer to unsigned long.
2. SOCKOPT_NONBLOCK (optname). sets the socket non-blocking mode on or off.
In that case optval should be SOCK_ON or SOCK_OFF (optval).
- See also:
- getsockopt
Definition at line 381 of file socket.cpp.
int simple_link_recv | ( | long | sd, |
void * | buf, | ||
long | len, | ||
long | flags, | ||
sockaddr * | from, | ||
socklen_t * | fromlen, | ||
long | opcode | ||
) |
Read data from socket (simple_link_recv).
Return the length of the message on successful completion.
If a message is too long to fit in the supplied buffer, excess bytes may
be discarded depending on the type of socket the message is received from.
- Parameters:
-
sd socket handle buf read buffer len buffer length flags indicates blocking or non-blocking operation from pointer to an address structure indicating source address fromlen source address structure size
- Returns:
- Return the number of bytes received, or -1 if an error occurred
Definition at line 449 of file socket.cpp.
int simple_link_send | ( | long | sd, |
const void * | buf, | ||
long | len, | ||
long | flags, | ||
const sockaddr * | to, | ||
long | tolen, | ||
long | opcode | ||
) |
Transmit a message to another socket (simple_link_send).
- Parameters:
-
sd socket handle buf write buffer len buffer length flags On this version, this parameter is not supported to pointer to an address structure indicating destination address tolen destination address structure size
- Returns:
- Return the number of bytes transmitted, or -1 if an error
occurred, or -2 in case there are no free buffers available
(only when SEND_NON_BLOCKING is enabled)
Definition at line 494 of file socket.cpp.
int socket | ( | long | domain, |
long | type, | ||
long | protocol | ||
) |
create an endpoint for communication.
The socket function creates a socket that is bound to a specific transport service provider.
This function is called by the application layer to obtain a socket handle.
- Parameters:
-
domain selects the protocol family which will be used for
communication. On this version only AF_INET is supported
type specifies the communication semantics. On this version
only SOCK_STREAM, SOCK_DGRAM, SOCK_RAW are supported
protocol specifies a particular protocol to be used with the
socket IPPROTO_TCP, IPPROTO_UDP or IPPROTO_RAW are supported.
- Returns:
- On success, socket handle that is used for consequent socket operations
On error, -1 is returned.
Definition at line 89 of file socket.cpp.
Generated on Wed Jul 13 2022 18:31:31 by
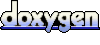