Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
Security
Functions | |
void | expandKey (unsigned char *expandedKey, unsigned char *key) |
expand a 16 bytes key for AES128 implementation. | |
unsigned char | galois_mul2 (unsigned char value) |
multiply by 2 in the galois field. | |
void | aes_encr (unsigned char *state, unsigned char *expandedKey) |
internal implementation of AES128 encryption. | |
void | aes_decr (unsigned char *state, unsigned char *expandedKey) |
internal implementation of AES128 decryption. | |
void | aes_encrypt (unsigned char *state, unsigned char *key) |
AES128 encryption. | |
void | aes_decrypt (unsigned char *state, unsigned char *key) |
AES128 decryption. | |
signed long | aes_read_key (unsigned char *key) |
Read the AES128 key from fileID #12 in EEPROM. | |
signed long | aes_write_key (unsigned char *key) |
Write the AES128 key to fileID #12 in EEPROM. |
Function Documentation
void aes_decr | ( | unsigned char * | state, |
unsigned char * | expandedKey | ||
) |
internal implementation of AES128 decryption.
straightforward aes decryption implementation
the order of substeps is the exact reverse of decryption
inverse functions:
- addRoundKey is its own inverse
- rsbox is inverse of sbox
- rightshift instead of leftshift
- invMixColumns = barreto + mixColumns
no further subfunctions to save cycles for function calls
no structuring with "for (....)" to save cycles
- Parameters:
-
[in] expandedKey expanded AES128 key in\out] state 16 bytes of cipher text and plain text
- Returns:
- none
Definition at line 189 of file security.cpp.
void aes_decrypt | ( | unsigned char * | state, |
unsigned char * | key | ||
) |
AES128 decryption.
Given AES128 key and 16 bytes cipher text, plain text of 16 bytes is computed.
The AES implementation is in mode ECB (Electronic Code Book).
- Parameters:
-
[in] key AES128 key of size 16 bytes in\out] state 16 bytes of cipher text and plain text
- Returns:
- none
Definition at line 326 of file security.cpp.
void aes_encr | ( | unsigned char * | state, |
unsigned char * | expandedKey | ||
) |
internal implementation of AES128 encryption.
straight forward aes encryption implementation
first the group of operations
- addRoundKey
- subbytes
- shiftrows
- mixcolums
is executed 9 times, after this addroundkey to finish the 9th
round, after that the 10th round without mixcolums
no further subfunctions to save cycles for function calls
no structuring with "for (....)" to save cycles.
- Parameters:
-
[in] expandedKey expanded AES128 key in/out] state 16 bytes of plain text and cipher text
- Returns:
- none
Definition at line 82 of file security.cpp.
void aes_encrypt | ( | unsigned char * | state, |
unsigned char * | key | ||
) |
AES128 encryption.
Given AES128 key and 16 bytes plain text, cipher text of 16 bytes is computed.
The AES implementation is in mode ECB (Electronic Code Book).
- Parameters:
-
[in] key AES128 key of size 16 bytes in\out] state 16 bytes of plain text and cipher text
- Returns:
- none
Definition at line 317 of file security.cpp.
signed long aes_read_key | ( | unsigned char * | key ) |
Read the AES128 key from fileID #12 in EEPROM.
- Parameters:
-
[out] key AES128 key of size 16 bytes
- Returns:
- 0 on success, error otherwise.
Definition at line 334 of file security.cpp.
signed long aes_write_key | ( | unsigned char * | key ) |
Write the AES128 key to fileID #12 in EEPROM.
- Parameters:
-
[out] key AES128 key of size 16 bytes
- Returns:
- on success 0, error otherwise.
Definition at line 344 of file security.cpp.
void expandKey | ( | unsigned char * | expandedKey, |
unsigned char * | key | ||
) |
expand a 16 bytes key for AES128 implementation.
- Parameters:
-
key AES128 key - 16 bytes expandedKey expanded AES128 key
- Returns:
- none
Definition at line 42 of file security.cpp.
unsigned char galois_mul2 | ( | unsigned char | value ) |
multiply by 2 in the galois field.
- Parameters:
-
value argument to multiply
- Returns:
- multiplied argument
Definition at line 71 of file security.cpp.
Generated on Wed Jul 13 2022 18:31:31 by
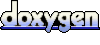