This is a fork of the mbed port of axTLS
Dependents: TLS_axTLS-Example HTTPSClientExample
crypto.h File Reference
Go to the source code of this file.
Functions | |
void | AES_set_key (AES_CTX *ctx, const uint8_t *key, const uint8_t *iv, AES_MODE mode) |
Set up AES with the key/iv and cipher size. | |
void | AES_cbc_encrypt (AES_CTX *ctx, const uint8_t *msg, uint8_t *out, int length) |
Encrypt a byte sequence (with a block size 16) using the AES cipher. | |
void | AES_cbc_decrypt (AES_CTX *ks, const uint8_t *in, uint8_t *out, int length) |
Decrypt a byte sequence (with a block size 16) using the AES cipher. | |
void | AES_convert_key (AES_CTX *ctx) |
Change a key for decryption. | |
void | RC4_setup (RC4_CTX *s, const uint8_t *key, int length) |
An implementation of the RC4/ARC4 algorithm. | |
void | RC4_crypt (RC4_CTX *s, const uint8_t *msg, uint8_t *data, int length) |
Perform the encrypt/decrypt operation (can use it for either since this is a stream cipher). | |
void | SHA1_Init (SHA1_CTX *) |
Initialize the SHA1 context. | |
void | SHA1_Update (SHA1_CTX *, const uint8_t *msg, int len) |
Accepts an array of octets as the next portion of the message. | |
void | SHA1_Final (uint8_t *digest, SHA1_CTX *) |
Return the 160-bit message digest into the user's array. | |
EXP_FUNC void STDCALL | MD5_Init (MD5_CTX *) |
MD5 initialization - begins an MD5 operation, writing a new ctx. | |
EXP_FUNC void STDCALL | MD5_Update (MD5_CTX *, const uint8_t *msg, int len) |
Accepts an array of octets as the next portion of the message. | |
EXP_FUNC void STDCALL | MD5_Final (uint8_t *digest, MD5_CTX *) |
Return the 128-bit message digest into the user's array. | |
void | hmac_md5 (const uint8_t *msg, int length, const uint8_t *key, int key_len, uint8_t *digest) |
HMAC implementation - This code was originally taken from RFC2104 See http://www.ietf.org/rfc/rfc2104.txt and http://www.faqs.org/rfcs/rfc2202.html. | |
void | hmac_sha1 (const uint8_t *msg, int length, const uint8_t *key, int key_len, uint8_t *digest) |
Perform HMAC-SHA1 NOTE: does not handle keys larger than the block size. | |
void | RSA_free (RSA_CTX *ctx) |
Free up any RSA context resources. | |
int | RSA_decrypt (const RSA_CTX *ctx, const uint8_t *in_data, uint8_t *out_data, int is_decryption) |
Use PKCS1.5 for decryption/verification. | |
bigint * | RSA_private (const RSA_CTX *c, bigint *bi_msg) |
Performs m = c^d mod n. | |
bigint * | RSA_public (const RSA_CTX *c, bigint *bi_msg) |
Performs c = m^e mod n. | |
int | RSA_encrypt (const RSA_CTX *ctx, const uint8_t *in_data, uint16_t in_len, uint8_t *out_data, int is_signing) |
Use PKCS1.5 for encryption/signing. | |
void | RSA_print (const RSA_CTX *ctx) |
Used for diagnostics. | |
EXP_FUNC void STDCALL | RNG_initialize (void) |
Initialise the Random Number Generator engine. | |
EXP_FUNC void STDCALL | RNG_custom_init (const uint8_t *seed_buf, int size) |
If no /dev/urandom, then initialise the RNG with something interesting. | |
EXP_FUNC void STDCALL | RNG_terminate (void) |
Terminate the RNG engine. | |
EXP_FUNC void STDCALL | get_random (int num_rand_bytes, uint8_t *rand_data) |
Set a series of bytes with a random number. | |
void | get_random_NZ (int num_rand_bytes, uint8_t *rand_data) |
Set a series of bytes with a random number. |
Detailed Description
Definition in file crypto.h.
Function Documentation
void AES_cbc_decrypt | ( | AES_CTX * | ks, |
const uint8_t * | in, | ||
uint8_t * | out, | ||
int | length | ||
) |
void AES_cbc_encrypt | ( | AES_CTX * | ctx, |
const uint8_t * | msg, | ||
uint8_t * | out, | ||
int | length | ||
) |
void AES_convert_key | ( | AES_CTX * | ctx ) |
void AES_set_key | ( | AES_CTX * | ctx, |
const uint8_t * | key, | ||
const uint8_t * | iv, | ||
AES_MODE | mode | ||
) |
EXP_FUNC void STDCALL get_random | ( | int | num_rand_bytes, |
uint8_t * | rand_data | ||
) |
Set a series of bytes with a random number.
Individual bytes can be 0
Definition at line 164 of file crypto_misc.c.
void get_random_NZ | ( | int | num_rand_bytes, |
uint8_t * | rand_data | ||
) |
Set a series of bytes with a random number.
Individual bytes are not zero.
Definition at line 214 of file crypto_misc.c.
void hmac_md5 | ( | const uint8_t * | msg, |
int | length, | ||
const uint8_t * | key, | ||
int | key_len, | ||
uint8_t * | digest | ||
) |
HMAC implementation - This code was originally taken from RFC2104 See http://www.ietf.org/rfc/rfc2104.txt and http://www.faqs.org/rfcs/rfc2202.html.
Perform HMAC-MD5 NOTE: does not handle keys larger than the block size.
void hmac_sha1 | ( | const uint8_t * | msg, |
int | length, | ||
const uint8_t * | key, | ||
int | key_len, | ||
uint8_t * | digest | ||
) |
EXP_FUNC void STDCALL MD5_Final | ( | uint8_t * | digest, |
MD5_CTX * | |||
) |
EXP_FUNC void STDCALL MD5_Init | ( | MD5_CTX * | ) |
EXP_FUNC void STDCALL MD5_Update | ( | MD5_CTX * | , |
const uint8_t * | msg, | ||
int | len | ||
) |
void RC4_crypt | ( | RC4_CTX * | ctx, |
const uint8_t * | msg, | ||
uint8_t * | out, | ||
int | length | ||
) |
void RC4_setup | ( | RC4_CTX * | ctx, |
const uint8_t * | key, | ||
int | length | ||
) |
EXP_FUNC void STDCALL RNG_custom_init | ( | const uint8_t * | seed_buf, |
int | size | ||
) |
If no /dev/urandom, then initialise the RNG with something interesting.
Definition at line 139 of file crypto_misc.c.
EXP_FUNC void STDCALL RNG_initialize | ( | void | ) |
Initialise the Random Number Generator engine.
- On Win32 use the platform SDK's crypto engine.
- On Linux use /dev/urandom
- If none of these work then use a custom RNG.
Definition at line 109 of file crypto_misc.c.
EXP_FUNC void STDCALL RNG_terminate | ( | void | ) |
Terminate the RNG engine.
Definition at line 152 of file crypto_misc.c.
int RSA_decrypt | ( | const RSA_CTX * | ctx, |
const uint8_t * | in_data, | ||
uint8_t * | out_data, | ||
int | is_decryption | ||
) |
Use PKCS1.5 for decryption/verification.
- Parameters:
-
ctx [in] The context in_data [in] The data to encrypt (must be < modulus size-11) out_data [out] The encrypted data. is_decryption [in] Decryption or verify operation.
- Returns:
- The number of bytes that were originally encrypted. -1 on error.
int RSA_encrypt | ( | const RSA_CTX * | ctx, |
const uint8_t * | in_data, | ||
uint16_t | in_len, | ||
uint8_t * | out_data, | ||
int | is_signing | ||
) |
Use PKCS1.5 for encryption/signing.
void RSA_free | ( | RSA_CTX * | ctx ) |
void SHA1_Final | ( | uint8_t * | digest, |
SHA1_CTX * | |||
) |
Generated on Wed Jul 13 2022 19:30:08 by
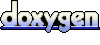