Helloworld program for the S25FL216K flash memory in combination with USBFileSystem
Dependencies: S25FL216K_USBFileSystem mbed
main.cpp
00001 #include "mbed.h" 00002 #include "Flash_USBFileSystem.h" 00003 00004 DigitalOut myled(LED1); 00005 FlashUSB flash(PTD6, PTD7, PTB11, PTE4); 00006 00007 void usbCallback(bool available) 00008 { 00009 if (available) { 00010 FILE *fp = fopen("/USB/in.txt", "r"); 00011 char buffer[100]; 00012 fgets (buffer, 100, fp); 00013 printf("%s\r\n", buffer); 00014 fclose(fp); 00015 } 00016 } 00017 00018 int main() 00019 { 00020 flash.attachUSB(&usbCallback); 00021 00022 wait(0.1); 00023 printf("Hello World!\r\n"); 00024 00025 FILE *fp = fopen("/USB/in.txt", "w"); 00026 00027 if(fp == NULL) { 00028 printf("Could not open file, assuming unformatted disk!\r\n"); 00029 printf("Formatting disk!\r\n"); 00030 flash.format(); 00031 printf("Disk formatted!\r\n"); 00032 printf("Reset your device!\r\n"); 00033 while(1); 00034 } else { 00035 wait(0.2); 00036 fprintf(fp, "Type your text here!"); 00037 fclose(fp); 00038 } 00039 00040 //Connect USB 00041 flash.connect(); 00042 flash.usbMode(1); //Disconnect USB when files are locally written 00043 00044 char buffer[101]; 00045 printf("Type your text here! (100 max length)\n"); 00046 while(1) { 00047 gets(buffer); 00048 myled = !myled; 00049 00050 //Open file for write, keep trying until we succeed 00051 //This is needed because if USB happens to be writing it will fail 00052 while(1) { 00053 fp = fopen("/USB/out.txt", "w"); 00054 if (fp != NULL) 00055 break; 00056 } 00057 fprintf(fp, buffer); 00058 fclose(fp); 00059 00060 } 00061 00062 }
Generated on Mon Jul 18 2022 14:36:21 by
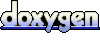