CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_math.h
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_math.h 00009 * 00010 * Description: Public header file for CMSIS DSP Library 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 /** 00042 \mainpage CMSIS DSP Software Library 00043 * 00044 * <b>Introduction</b> 00045 * 00046 * This user manual describes the CMSIS DSP software library, 00047 * a suite of common signal processing functions for use on Cortex-M processor based devices. 00048 * 00049 * The library is divided into a number of functions each covering a specific category: 00050 * - Basic math functions 00051 * - Fast math functions 00052 * - Complex math functions 00053 * - Filters 00054 * - Matrix functions 00055 * - Transforms 00056 * - Motor control functions 00057 * - Statistical functions 00058 * - Support functions 00059 * - Interpolation functions 00060 * 00061 * The library has separate functions for operating on 8-bit integers, 16-bit integers, 00062 * 32-bit integer and 32-bit floating-point values. 00063 * 00064 * <b>Using the Library</b> 00065 * 00066 * The library installer contains prebuilt versions of the libraries in the <code>Lib</code> folder. 00067 * - arm_cortexM4lf_math.lib (Little endian and Floating Point Unit on Cortex-M4) 00068 * - arm_cortexM4bf_math.lib (Big endian and Floating Point Unit on Cortex-M4) 00069 * - arm_cortexM4l_math.lib (Little endian on Cortex-M4) 00070 * - arm_cortexM4b_math.lib (Big endian on Cortex-M4) 00071 * - arm_cortexM3l_math.lib (Little endian on Cortex-M3) 00072 * - arm_cortexM3b_math.lib (Big endian on Cortex-M3) 00073 * - arm_cortexM0l_math.lib (Little endian on Cortex-M0) 00074 * - arm_cortexM0b_math.lib (Big endian on Cortex-M3) 00075 * 00076 * The library functions are declared in the public file <code>arm_math.h</code> which is placed in the <code>Include</code> folder. 00077 * Simply include this file and link the appropriate library in the application and begin calling the library functions. The Library supports single 00078 * public header file <code> arm_math.h</code> for Cortex-M4/M3/M0 with little endian and big endian. Same header file will be used for floating point unit(FPU) variants. 00079 * Define the appropriate pre processor MACRO ARM_MATH_CM4 or ARM_MATH_CM3 or 00080 * ARM_MATH_CM0 or ARM_MATH_CM0PLUS depending on the target processor in the application. 00081 * 00082 * <b>Examples</b> 00083 * 00084 * The library ships with a number of examples which demonstrate how to use the library functions. 00085 * 00086 * <b>Toolchain Support</b> 00087 * 00088 * The library has been developed and tested with MDK-ARM version 4.60. 00089 * The library is being tested in GCC and IAR toolchains and updates on this activity will be made available shortly. 00090 * 00091 * <b>Building the Library</b> 00092 * 00093 * The library installer contains project files to re build libraries on MDK Tool chain in the <code>CMSIS\\DSP_Lib\\Source\\ARM</code> folder. 00094 * - arm_cortexM0b_math.uvproj 00095 * - arm_cortexM0l_math.uvproj 00096 * - arm_cortexM3b_math.uvproj 00097 * - arm_cortexM3l_math.uvproj 00098 * - arm_cortexM4b_math.uvproj 00099 * - arm_cortexM4l_math.uvproj 00100 * - arm_cortexM4bf_math.uvproj 00101 * - arm_cortexM4lf_math.uvproj 00102 * 00103 * 00104 * The project can be built by opening the appropriate project in MDK-ARM 4.60 chain and defining the optional pre processor MACROs detailed above. 00105 * 00106 * <b>Pre-processor Macros</b> 00107 * 00108 * Each library project have differant pre-processor macros. 00109 * 00110 * - UNALIGNED_SUPPORT_DISABLE: 00111 * 00112 * Define macro UNALIGNED_SUPPORT_DISABLE, If the silicon does not support unaligned memory access 00113 * 00114 * - ARM_MATH_BIG_ENDIAN: 00115 * 00116 * Define macro ARM_MATH_BIG_ENDIAN to build the library for big endian targets. By default library builds for little endian targets. 00117 * 00118 * - ARM_MATH_MATRIX_CHECK: 00119 * 00120 * Define macro ARM_MATH_MATRIX_CHECK for checking on the input and output sizes of matrices 00121 * 00122 * - ARM_MATH_ROUNDING: 00123 * 00124 * Define macro ARM_MATH_ROUNDING for rounding on support functions 00125 * 00126 * - ARM_MATH_CMx: 00127 * 00128 * Define macro ARM_MATH_CM4 for building the library on Cortex-M4 target, ARM_MATH_CM3 for building library on Cortex-M3 target 00129 * and ARM_MATH_CM0 for building library on cortex-M0 target, ARM_MATH_CM0PLUS for building library on cortex-M0+ target. 00130 * 00131 * - __FPU_PRESENT: 00132 * 00133 * Initialize macro __FPU_PRESENT = 1 when building on FPU supported Targets. Enable this macro for M4bf and M4lf libraries 00134 * 00135 * <b>Copyright Notice</b> 00136 * 00137 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00138 */ 00139 00140 00141 /** 00142 * @defgroup groupMath Basic Math Functions 00143 */ 00144 00145 /** 00146 * @defgroup groupFastMath Fast Math Functions 00147 * This set of functions provides a fast approximation to sine, cosine, and square root. 00148 * As compared to most of the other functions in the CMSIS math library, the fast math functions 00149 * operate on individual values and not arrays. 00150 * There are separate functions for Q15, Q31, and floating-point data. 00151 * 00152 */ 00153 00154 /** 00155 * @defgroup groupCmplxMath Complex Math Functions 00156 * This set of functions operates on complex data vectors. 00157 * The data in the complex arrays is stored in an interleaved fashion 00158 * (real, imag, real, imag, ...). 00159 * In the API functions, the number of samples in a complex array refers 00160 * to the number of complex values; the array contains twice this number of 00161 * real values. 00162 */ 00163 00164 /** 00165 * @defgroup groupFilters Filtering Functions 00166 */ 00167 00168 /** 00169 * @defgroup groupMatrix Matrix Functions 00170 * 00171 * This set of functions provides basic matrix math operations. 00172 * The functions operate on matrix data structures. For example, 00173 * the type 00174 * definition for the floating-point matrix structure is shown 00175 * below: 00176 * <pre> 00177 * typedef struct 00178 * { 00179 * uint16_t numRows; // number of rows of the matrix. 00180 * uint16_t numCols; // number of columns of the matrix. 00181 * float32_t *pData; // points to the data of the matrix. 00182 * } arm_matrix_instance_f32; 00183 * </pre> 00184 * There are similar definitions for Q15 and Q31 data types. 00185 * 00186 * The structure specifies the size of the matrix and then points to 00187 * an array of data. The array is of size <code>numRows X numCols</code> 00188 * and the values are arranged in row order. That is, the 00189 * matrix element (i, j) is stored at: 00190 * <pre> 00191 * pData[i*numCols + j] 00192 * </pre> 00193 * 00194 * \par Init Functions 00195 * There is an associated initialization function for each type of matrix 00196 * data structure. 00197 * The initialization function sets the values of the internal structure fields. 00198 * Refer to the function <code>arm_mat_init_f32()</code>, <code>arm_mat_init_q31()</code> 00199 * and <code>arm_mat_init_q15()</code> for floating-point, Q31 and Q15 types, respectively. 00200 * 00201 * \par 00202 * Use of the initialization function is optional. However, if initialization function is used 00203 * then the instance structure cannot be placed into a const data section. 00204 * To place the instance structure in a const data 00205 * section, manually initialize the data structure. For example: 00206 * <pre> 00207 * <code>arm_matrix_instance_f32 S = {nRows, nColumns, pData};</code> 00208 * <code>arm_matrix_instance_q31 S = {nRows, nColumns, pData};</code> 00209 * <code>arm_matrix_instance_q15 S = {nRows, nColumns, pData};</code> 00210 * </pre> 00211 * where <code>nRows</code> specifies the number of rows, <code>nColumns</code> 00212 * specifies the number of columns, and <code>pData</code> points to the 00213 * data array. 00214 * 00215 * \par Size Checking 00216 * By default all of the matrix functions perform size checking on the input and 00217 * output matrices. For example, the matrix addition function verifies that the 00218 * two input matrices and the output matrix all have the same number of rows and 00219 * columns. If the size check fails the functions return: 00220 * <pre> 00221 * ARM_MATH_SIZE_MISMATCH 00222 * </pre> 00223 * Otherwise the functions return 00224 * <pre> 00225 * ARM_MATH_SUCCESS 00226 * </pre> 00227 * There is some overhead associated with this matrix size checking. 00228 * The matrix size checking is enabled via the \#define 00229 * <pre> 00230 * ARM_MATH_MATRIX_CHECK 00231 * </pre> 00232 * within the library project settings. By default this macro is defined 00233 * and size checking is enabled. By changing the project settings and 00234 * undefining this macro size checking is eliminated and the functions 00235 * run a bit faster. With size checking disabled the functions always 00236 * return <code>ARM_MATH_SUCCESS</code>. 00237 */ 00238 00239 /** 00240 * @defgroup groupTransforms Transform Functions 00241 */ 00242 00243 /** 00244 * @defgroup groupController Controller Functions 00245 */ 00246 00247 /** 00248 * @defgroup groupStats Statistics Functions 00249 */ 00250 /** 00251 * @defgroup groupSupport Support Functions 00252 */ 00253 00254 /** 00255 * @defgroup groupInterpolation Interpolation Functions 00256 * These functions perform 1- and 2-dimensional interpolation of data. 00257 * Linear interpolation is used for 1-dimensional data and 00258 * bilinear interpolation is used for 2-dimensional data. 00259 */ 00260 00261 /** 00262 * @defgroup groupExamples Examples 00263 */ 00264 #ifndef _ARM_MATH_H 00265 #define _ARM_MATH_H 00266 00267 #define __CMSIS_GENERIC /* disable NVIC and Systick functions */ 00268 00269 #if defined (ARM_MATH_CM4) 00270 #include "core_cm4.h" 00271 #elif defined (ARM_MATH_CM3) 00272 #include "core_cm3.h" 00273 #elif defined (ARM_MATH_CM0) 00274 #include "core_cm0.h" 00275 #define ARM_MATH_CM0_FAMILY 00276 #elif defined (ARM_MATH_CM0PLUS) 00277 #include "core_cm0plus.h" 00278 #define ARM_MATH_CM0_FAMILY 00279 #else 00280 #include "ARMCM4.h" 00281 #warning "Define either ARM_MATH_CM4 OR ARM_MATH_CM3...By Default building on ARM_MATH_CM4....." 00282 #endif 00283 00284 #undef __CMSIS_GENERIC /* enable NVIC and Systick functions */ 00285 #include "string.h" 00286 #include "math.h" 00287 #ifdef __cplusplus 00288 extern "C" 00289 { 00290 #endif 00291 00292 00293 /** 00294 * @brief Macros required for reciprocal calculation in Normalized LMS 00295 */ 00296 00297 #define DELTA_Q31 (0x100) 00298 #define DELTA_Q15 0x5 00299 #define INDEX_MASK 0x0000003F 00300 #ifndef PI 00301 #define PI 3.14159265358979f 00302 #endif 00303 00304 /** 00305 * @brief Macros required for SINE and COSINE Fast math approximations 00306 */ 00307 00308 #define TABLE_SIZE 256 00309 #define TABLE_SPACING_Q31 0x800000 00310 #define TABLE_SPACING_Q15 0x80 00311 00312 /** 00313 * @brief Macros required for SINE and COSINE Controller functions 00314 */ 00315 /* 1.31(q31) Fixed value of 2/360 */ 00316 /* -1 to +1 is divided into 360 values so total spacing is (2/360) */ 00317 #define INPUT_SPACING 0xB60B61 00318 00319 /** 00320 * @brief Macro for Unaligned Support 00321 */ 00322 #ifndef UNALIGNED_SUPPORT_DISABLE 00323 #define ALIGN4 00324 #else 00325 #if defined (__GNUC__) 00326 #define ALIGN4 __attribute__((aligned(4))) 00327 #else 00328 #define ALIGN4 __align(4) 00329 #endif 00330 #endif /* #ifndef UNALIGNED_SUPPORT_DISABLE */ 00331 00332 /** 00333 * @brief Error status returned by some functions in the library. 00334 */ 00335 00336 typedef enum 00337 { 00338 ARM_MATH_SUCCESS = 0, /**< No error */ 00339 ARM_MATH_ARGUMENT_ERROR = -1, /**< One or more arguments are incorrect */ 00340 ARM_MATH_LENGTH_ERROR = -2, /**< Length of data buffer is incorrect */ 00341 ARM_MATH_SIZE_MISMATCH = -3, /**< Size of matrices is not compatible with the operation. */ 00342 ARM_MATH_NANINF = -4, /**< Not-a-number (NaN) or infinity is generated */ 00343 ARM_MATH_SINGULAR = -5, /**< Generated by matrix inversion if the input matrix is singular and cannot be inverted. */ 00344 ARM_MATH_TEST_FAILURE = -6 /**< Test Failed */ 00345 } arm_status; 00346 00347 /** 00348 * @brief 8-bit fractional data type in 1.7 format. 00349 */ 00350 typedef int8_t q7_t; 00351 00352 /** 00353 * @brief 16-bit fractional data type in 1.15 format. 00354 */ 00355 typedef int16_t q15_t; 00356 00357 /** 00358 * @brief 32-bit fractional data type in 1.31 format. 00359 */ 00360 typedef int32_t q31_t; 00361 00362 /** 00363 * @brief 64-bit fractional data type in 1.63 format. 00364 */ 00365 typedef int64_t q63_t; 00366 00367 /** 00368 * @brief 32-bit floating-point type definition. 00369 */ 00370 typedef float float32_t; 00371 00372 /** 00373 * @brief 64-bit floating-point type definition. 00374 */ 00375 typedef double float64_t; 00376 00377 /** 00378 * @brief definition to read/write two 16 bit values. 00379 */ 00380 #if defined __CC_ARM 00381 #define __SIMD32_TYPE int32_t __packed 00382 #define CMSIS_UNUSED __attribute__((unused)) 00383 #elif defined __ICCARM__ 00384 #define CMSIS_UNUSED 00385 #define __SIMD32_TYPE int32_t __packed 00386 #elif defined __GNUC__ 00387 #define __SIMD32_TYPE int32_t 00388 #define CMSIS_UNUSED __attribute__((unused)) 00389 #else 00390 #error Unknown compiler 00391 #endif 00392 00393 #define __SIMD32(addr) (*(__SIMD32_TYPE **) & (addr)) 00394 #define __SIMD32_CONST(addr) ((__SIMD32_TYPE *)(addr)) 00395 00396 #define _SIMD32_OFFSET(addr) (*(__SIMD32_TYPE *) (addr)) 00397 00398 #define __SIMD64(addr) (*(int64_t **) & (addr)) 00399 00400 #if defined (ARM_MATH_CM3) || defined (ARM_MATH_CM0_FAMILY) 00401 /** 00402 * @brief definition to pack two 16 bit values. 00403 */ 00404 #define __PKHBT(ARG1, ARG2, ARG3) ( (((int32_t)(ARG1) << 0) & (int32_t)0x0000FFFF) | \ 00405 (((int32_t)(ARG2) << ARG3) & (int32_t)0xFFFF0000) ) 00406 #define __PKHTB(ARG1, ARG2, ARG3) ( (((int32_t)(ARG1) << 0) & (int32_t)0xFFFF0000) | \ 00407 (((int32_t)(ARG2) >> ARG3) & (int32_t)0x0000FFFF) ) 00408 00409 #endif 00410 00411 00412 /** 00413 * @brief definition to pack four 8 bit values. 00414 */ 00415 #ifndef ARM_MATH_BIG_ENDIAN 00416 00417 #define __PACKq7(v0,v1,v2,v3) ( (((int32_t)(v0) << 0) & (int32_t)0x000000FF) | \ 00418 (((int32_t)(v1) << 8) & (int32_t)0x0000FF00) | \ 00419 (((int32_t)(v2) << 16) & (int32_t)0x00FF0000) | \ 00420 (((int32_t)(v3) << 24) & (int32_t)0xFF000000) ) 00421 #else 00422 00423 #define __PACKq7(v0,v1,v2,v3) ( (((int32_t)(v3) << 0) & (int32_t)0x000000FF) | \ 00424 (((int32_t)(v2) << 8) & (int32_t)0x0000FF00) | \ 00425 (((int32_t)(v1) << 16) & (int32_t)0x00FF0000) | \ 00426 (((int32_t)(v0) << 24) & (int32_t)0xFF000000) ) 00427 00428 #endif 00429 00430 00431 /** 00432 * @brief Clips Q63 to Q31 values. 00433 */ 00434 static __INLINE q31_t clip_q63_to_q31( 00435 q63_t x) 00436 { 00437 return ((q31_t) (x >> 32) != ((q31_t) x >> 31)) ? 00438 ((0x7FFFFFFF ^ ((q31_t) (x >> 63)))) : (q31_t) x; 00439 } 00440 00441 /** 00442 * @brief Clips Q63 to Q15 values. 00443 */ 00444 static __INLINE q15_t clip_q63_to_q15( 00445 q63_t x) 00446 { 00447 return ((q31_t) (x >> 32) != ((q31_t) x >> 31)) ? 00448 ((0x7FFF ^ ((q15_t) (x >> 63)))) : (q15_t) (x >> 15); 00449 } 00450 00451 /** 00452 * @brief Clips Q31 to Q7 values. 00453 */ 00454 static __INLINE q7_t clip_q31_to_q7( 00455 q31_t x) 00456 { 00457 return ((q31_t) (x >> 24) != ((q31_t) x >> 23)) ? 00458 ((0x7F ^ ((q7_t) (x >> 31)))) : (q7_t) x; 00459 } 00460 00461 /** 00462 * @brief Clips Q31 to Q15 values. 00463 */ 00464 static __INLINE q15_t clip_q31_to_q15( 00465 q31_t x) 00466 { 00467 return ((q31_t) (x >> 16) != ((q31_t) x >> 15)) ? 00468 ((0x7FFF ^ ((q15_t) (x >> 31)))) : (q15_t) x; 00469 } 00470 00471 /** 00472 * @brief Multiplies 32 X 64 and returns 32 bit result in 2.30 format. 00473 */ 00474 00475 static __INLINE q63_t mult32x64( 00476 q63_t x, 00477 q31_t y) 00478 { 00479 return ((((q63_t) (x & 0x00000000FFFFFFFF) * y) >> 32) + 00480 (((q63_t) (x >> 32) * y))); 00481 } 00482 00483 00484 #if defined (ARM_MATH_CM0_FAMILY) && defined ( __CC_ARM ) 00485 #define __CLZ __clz 00486 #elif defined (ARM_MATH_CM0_FAMILY) && ((defined (__ICCARM__)) ||(defined (__GNUC__)) || defined (__TASKING__) ) 00487 00488 static __INLINE uint32_t __CLZ( 00489 q31_t data); 00490 00491 00492 static __INLINE uint32_t __CLZ( 00493 q31_t data) 00494 { 00495 uint32_t count = 0; 00496 uint32_t mask = 0x80000000; 00497 00498 while((data & mask) == 0) 00499 { 00500 count += 1u; 00501 mask = mask >> 1u; 00502 } 00503 00504 return (count); 00505 00506 } 00507 00508 #endif 00509 00510 /** 00511 * @brief Function to Calculates 1/in (reciprocal) value of Q31 Data type. 00512 */ 00513 00514 static __INLINE uint32_t arm_recip_q31( 00515 q31_t in, 00516 q31_t * dst, 00517 q31_t * pRecipTable) 00518 { 00519 00520 uint32_t out, tempVal; 00521 uint32_t index, i; 00522 uint32_t signBits; 00523 00524 if(in > 0) 00525 { 00526 signBits = __CLZ(in) - 1; 00527 } 00528 else 00529 { 00530 signBits = __CLZ(-in) - 1; 00531 } 00532 00533 /* Convert input sample to 1.31 format */ 00534 in = in << signBits; 00535 00536 /* calculation of index for initial approximated Val */ 00537 index = (uint32_t) (in >> 24u); 00538 index = (index & INDEX_MASK); 00539 00540 /* 1.31 with exp 1 */ 00541 out = pRecipTable[index]; 00542 00543 /* calculation of reciprocal value */ 00544 /* running approximation for two iterations */ 00545 for (i = 0u; i < 2u; i++) 00546 { 00547 tempVal = (q31_t) (((q63_t) in * out) >> 31u); 00548 tempVal = 0x7FFFFFFF - tempVal; 00549 /* 1.31 with exp 1 */ 00550 //out = (q31_t) (((q63_t) out * tempVal) >> 30u); 00551 out = (q31_t) clip_q63_to_q31(((q63_t) out * tempVal) >> 30u); 00552 } 00553 00554 /* write output */ 00555 *dst = out; 00556 00557 /* return num of signbits of out = 1/in value */ 00558 return (signBits + 1u); 00559 00560 } 00561 00562 /** 00563 * @brief Function to Calculates 1/in (reciprocal) value of Q15 Data type. 00564 */ 00565 static __INLINE uint32_t arm_recip_q15( 00566 q15_t in, 00567 q15_t * dst, 00568 q15_t * pRecipTable) 00569 { 00570 00571 uint32_t out = 0, tempVal = 0; 00572 uint32_t index = 0, i = 0; 00573 uint32_t signBits = 0; 00574 00575 if(in > 0) 00576 { 00577 signBits = __CLZ(in) - 17; 00578 } 00579 else 00580 { 00581 signBits = __CLZ(-in) - 17; 00582 } 00583 00584 /* Convert input sample to 1.15 format */ 00585 in = in << signBits; 00586 00587 /* calculation of index for initial approximated Val */ 00588 index = in >> 8; 00589 index = (index & INDEX_MASK); 00590 00591 /* 1.15 with exp 1 */ 00592 out = pRecipTable[index]; 00593 00594 /* calculation of reciprocal value */ 00595 /* running approximation for two iterations */ 00596 for (i = 0; i < 2; i++) 00597 { 00598 tempVal = (q15_t) (((q31_t) in * out) >> 15); 00599 tempVal = 0x7FFF - tempVal; 00600 /* 1.15 with exp 1 */ 00601 out = (q15_t) (((q31_t) out * tempVal) >> 14); 00602 } 00603 00604 /* write output */ 00605 *dst = out; 00606 00607 /* return num of signbits of out = 1/in value */ 00608 return (signBits + 1); 00609 00610 } 00611 00612 00613 /* 00614 * @brief C custom defined intrinisic function for only M0 processors 00615 */ 00616 #if defined(ARM_MATH_CM0_FAMILY) 00617 00618 static __INLINE q31_t __SSAT( 00619 q31_t x, 00620 uint32_t y) 00621 { 00622 int32_t posMax, negMin; 00623 uint32_t i; 00624 00625 posMax = 1; 00626 for (i = 0; i < (y - 1); i++) 00627 { 00628 posMax = posMax * 2; 00629 } 00630 00631 if(x > 0) 00632 { 00633 posMax = (posMax - 1); 00634 00635 if(x > posMax) 00636 { 00637 x = posMax; 00638 } 00639 } 00640 else 00641 { 00642 negMin = -posMax; 00643 00644 if(x < negMin) 00645 { 00646 x = negMin; 00647 } 00648 } 00649 return (x); 00650 00651 00652 } 00653 00654 #endif /* end of ARM_MATH_CM0_FAMILY */ 00655 00656 00657 00658 /* 00659 * @brief C custom defined intrinsic function for M3 and M0 processors 00660 */ 00661 #if defined (ARM_MATH_CM3) || defined (ARM_MATH_CM0_FAMILY) 00662 00663 /* 00664 * @brief C custom defined QADD8 for M3 and M0 processors 00665 */ 00666 static __INLINE q31_t __QADD8( 00667 q31_t x, 00668 q31_t y) 00669 { 00670 00671 q31_t sum; 00672 q7_t r, s, t, u; 00673 00674 r = (q7_t) x; 00675 s = (q7_t) y; 00676 00677 r = __SSAT((q31_t) (r + s), 8); 00678 s = __SSAT(((q31_t) (((x << 16) >> 24) + ((y << 16) >> 24))), 8); 00679 t = __SSAT(((q31_t) (((x << 8) >> 24) + ((y << 8) >> 24))), 8); 00680 u = __SSAT(((q31_t) ((x >> 24) + (y >> 24))), 8); 00681 00682 sum = 00683 (((q31_t) u << 24) & 0xFF000000) | (((q31_t) t << 16) & 0x00FF0000) | 00684 (((q31_t) s << 8) & 0x0000FF00) | (r & 0x000000FF); 00685 00686 return sum; 00687 00688 } 00689 00690 /* 00691 * @brief C custom defined QSUB8 for M3 and M0 processors 00692 */ 00693 static __INLINE q31_t __QSUB8( 00694 q31_t x, 00695 q31_t y) 00696 { 00697 00698 q31_t sum; 00699 q31_t r, s, t, u; 00700 00701 r = (q7_t) x; 00702 s = (q7_t) y; 00703 00704 r = __SSAT((r - s), 8); 00705 s = __SSAT(((q31_t) (((x << 16) >> 24) - ((y << 16) >> 24))), 8) << 8; 00706 t = __SSAT(((q31_t) (((x << 8) >> 24) - ((y << 8) >> 24))), 8) << 16; 00707 u = __SSAT(((q31_t) ((x >> 24) - (y >> 24))), 8) << 24; 00708 00709 sum = 00710 (u & 0xFF000000) | (t & 0x00FF0000) | (s & 0x0000FF00) | (r & 00711 0x000000FF); 00712 00713 return sum; 00714 } 00715 00716 /* 00717 * @brief C custom defined QADD16 for M3 and M0 processors 00718 */ 00719 00720 /* 00721 * @brief C custom defined QADD16 for M3 and M0 processors 00722 */ 00723 static __INLINE q31_t __QADD16( 00724 q31_t x, 00725 q31_t y) 00726 { 00727 00728 q31_t sum; 00729 q31_t r, s; 00730 00731 r = (short) x; 00732 s = (short) y; 00733 00734 r = __SSAT(r + s, 16); 00735 s = __SSAT(((q31_t) ((x >> 16) + (y >> 16))), 16) << 16; 00736 00737 sum = (s & 0xFFFF0000) | (r & 0x0000FFFF); 00738 00739 return sum; 00740 00741 } 00742 00743 /* 00744 * @brief C custom defined SHADD16 for M3 and M0 processors 00745 */ 00746 static __INLINE q31_t __SHADD16( 00747 q31_t x, 00748 q31_t y) 00749 { 00750 00751 q31_t sum; 00752 q31_t r, s; 00753 00754 r = (short) x; 00755 s = (short) y; 00756 00757 r = ((r >> 1) + (s >> 1)); 00758 s = ((q31_t) ((x >> 17) + (y >> 17))) << 16; 00759 00760 sum = (s & 0xFFFF0000) | (r & 0x0000FFFF); 00761 00762 return sum; 00763 00764 } 00765 00766 /* 00767 * @brief C custom defined QSUB16 for M3 and M0 processors 00768 */ 00769 static __INLINE q31_t __QSUB16( 00770 q31_t x, 00771 q31_t y) 00772 { 00773 00774 q31_t sum; 00775 q31_t r, s; 00776 00777 r = (short) x; 00778 s = (short) y; 00779 00780 r = __SSAT(r - s, 16); 00781 s = __SSAT(((q31_t) ((x >> 16) - (y >> 16))), 16) << 16; 00782 00783 sum = (s & 0xFFFF0000) | (r & 0x0000FFFF); 00784 00785 return sum; 00786 } 00787 00788 /* 00789 * @brief C custom defined SHSUB16 for M3 and M0 processors 00790 */ 00791 static __INLINE q31_t __SHSUB16( 00792 q31_t x, 00793 q31_t y) 00794 { 00795 00796 q31_t diff; 00797 q31_t r, s; 00798 00799 r = (short) x; 00800 s = (short) y; 00801 00802 r = ((r >> 1) - (s >> 1)); 00803 s = (((x >> 17) - (y >> 17)) << 16); 00804 00805 diff = (s & 0xFFFF0000) | (r & 0x0000FFFF); 00806 00807 return diff; 00808 } 00809 00810 /* 00811 * @brief C custom defined QASX for M3 and M0 processors 00812 */ 00813 static __INLINE q31_t __QASX( 00814 q31_t x, 00815 q31_t y) 00816 { 00817 00818 q31_t sum = 0; 00819 00820 sum = 00821 ((sum + 00822 clip_q31_to_q15((q31_t) ((short) (x >> 16) + (short) y))) << 16) + 00823 clip_q31_to_q15((q31_t) ((short) x - (short) (y >> 16))); 00824 00825 return sum; 00826 } 00827 00828 /* 00829 * @brief C custom defined SHASX for M3 and M0 processors 00830 */ 00831 static __INLINE q31_t __SHASX( 00832 q31_t x, 00833 q31_t y) 00834 { 00835 00836 q31_t sum; 00837 q31_t r, s; 00838 00839 r = (short) x; 00840 s = (short) y; 00841 00842 r = ((r >> 1) - (y >> 17)); 00843 s = (((x >> 17) + (s >> 1)) << 16); 00844 00845 sum = (s & 0xFFFF0000) | (r & 0x0000FFFF); 00846 00847 return sum; 00848 } 00849 00850 00851 /* 00852 * @brief C custom defined QSAX for M3 and M0 processors 00853 */ 00854 static __INLINE q31_t __QSAX( 00855 q31_t x, 00856 q31_t y) 00857 { 00858 00859 q31_t sum = 0; 00860 00861 sum = 00862 ((sum + 00863 clip_q31_to_q15((q31_t) ((short) (x >> 16) - (short) y))) << 16) + 00864 clip_q31_to_q15((q31_t) ((short) x + (short) (y >> 16))); 00865 00866 return sum; 00867 } 00868 00869 /* 00870 * @brief C custom defined SHSAX for M3 and M0 processors 00871 */ 00872 static __INLINE q31_t __SHSAX( 00873 q31_t x, 00874 q31_t y) 00875 { 00876 00877 q31_t sum; 00878 q31_t r, s; 00879 00880 r = (short) x; 00881 s = (short) y; 00882 00883 r = ((r >> 1) + (y >> 17)); 00884 s = (((x >> 17) - (s >> 1)) << 16); 00885 00886 sum = (s & 0xFFFF0000) | (r & 0x0000FFFF); 00887 00888 return sum; 00889 } 00890 00891 /* 00892 * @brief C custom defined SMUSDX for M3 and M0 processors 00893 */ 00894 static __INLINE q31_t __SMUSDX( 00895 q31_t x, 00896 q31_t y) 00897 { 00898 00899 return ((q31_t) (((short) x * (short) (y >> 16)) - 00900 ((short) (x >> 16) * (short) y))); 00901 } 00902 00903 /* 00904 * @brief C custom defined SMUADX for M3 and M0 processors 00905 */ 00906 static __INLINE q31_t __SMUADX( 00907 q31_t x, 00908 q31_t y) 00909 { 00910 00911 return ((q31_t) (((short) x * (short) (y >> 16)) + 00912 ((short) (x >> 16) * (short) y))); 00913 } 00914 00915 /* 00916 * @brief C custom defined QADD for M3 and M0 processors 00917 */ 00918 static __INLINE q31_t __QADD( 00919 q31_t x, 00920 q31_t y) 00921 { 00922 return clip_q63_to_q31((q63_t) x + y); 00923 } 00924 00925 /* 00926 * @brief C custom defined QSUB for M3 and M0 processors 00927 */ 00928 static __INLINE q31_t __QSUB( 00929 q31_t x, 00930 q31_t y) 00931 { 00932 return clip_q63_to_q31((q63_t) x - y); 00933 } 00934 00935 /* 00936 * @brief C custom defined SMLAD for M3 and M0 processors 00937 */ 00938 static __INLINE q31_t __SMLAD( 00939 q31_t x, 00940 q31_t y, 00941 q31_t sum) 00942 { 00943 00944 return (sum + ((short) (x >> 16) * (short) (y >> 16)) + 00945 ((short) x * (short) y)); 00946 } 00947 00948 /* 00949 * @brief C custom defined SMLADX for M3 and M0 processors 00950 */ 00951 static __INLINE q31_t __SMLADX( 00952 q31_t x, 00953 q31_t y, 00954 q31_t sum) 00955 { 00956 00957 return (sum + ((short) (x >> 16) * (short) (y)) + 00958 ((short) x * (short) (y >> 16))); 00959 } 00960 00961 /* 00962 * @brief C custom defined SMLSDX for M3 and M0 processors 00963 */ 00964 static __INLINE q31_t __SMLSDX( 00965 q31_t x, 00966 q31_t y, 00967 q31_t sum) 00968 { 00969 00970 return (sum - ((short) (x >> 16) * (short) (y)) + 00971 ((short) x * (short) (y >> 16))); 00972 } 00973 00974 /* 00975 * @brief C custom defined SMLALD for M3 and M0 processors 00976 */ 00977 static __INLINE q63_t __SMLALD( 00978 q31_t x, 00979 q31_t y, 00980 q63_t sum) 00981 { 00982 00983 return (sum + ((short) (x >> 16) * (short) (y >> 16)) + 00984 ((short) x * (short) y)); 00985 } 00986 00987 /* 00988 * @brief C custom defined SMLALDX for M3 and M0 processors 00989 */ 00990 static __INLINE q63_t __SMLALDX( 00991 q31_t x, 00992 q31_t y, 00993 q63_t sum) 00994 { 00995 00996 return (sum + ((short) (x >> 16) * (short) y)) + 00997 ((short) x * (short) (y >> 16)); 00998 } 00999 01000 /* 01001 * @brief C custom defined SMUAD for M3 and M0 processors 01002 */ 01003 static __INLINE q31_t __SMUAD( 01004 q31_t x, 01005 q31_t y) 01006 { 01007 01008 return (((x >> 16) * (y >> 16)) + 01009 (((x << 16) >> 16) * ((y << 16) >> 16))); 01010 } 01011 01012 /* 01013 * @brief C custom defined SMUSD for M3 and M0 processors 01014 */ 01015 static __INLINE q31_t __SMUSD( 01016 q31_t x, 01017 q31_t y) 01018 { 01019 01020 return (-((x >> 16) * (y >> 16)) + 01021 (((x << 16) >> 16) * ((y << 16) >> 16))); 01022 } 01023 01024 01025 /* 01026 * @brief C custom defined SXTB16 for M3 and M0 processors 01027 */ 01028 static __INLINE q31_t __SXTB16( 01029 q31_t x) 01030 { 01031 01032 return ((((x << 24) >> 24) & 0x0000FFFF) | 01033 (((x << 8) >> 8) & 0xFFFF0000)); 01034 } 01035 01036 01037 #endif /* defined (ARM_MATH_CM3) || defined (ARM_MATH_CM0_FAMILY) */ 01038 01039 01040 /** 01041 * @brief Instance structure for the Q7 FIR filter. 01042 */ 01043 typedef struct 01044 { 01045 uint16_t numTaps; /**< number of filter coefficients in the filter. */ 01046 q7_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 01047 q7_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 01048 } arm_fir_instance_q7; 01049 01050 /** 01051 * @brief Instance structure for the Q15 FIR filter. 01052 */ 01053 typedef struct 01054 { 01055 uint16_t numTaps; /**< number of filter coefficients in the filter. */ 01056 q15_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 01057 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 01058 } arm_fir_instance_q15; 01059 01060 /** 01061 * @brief Instance structure for the Q31 FIR filter. 01062 */ 01063 typedef struct 01064 { 01065 uint16_t numTaps; /**< number of filter coefficients in the filter. */ 01066 q31_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 01067 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 01068 } arm_fir_instance_q31; 01069 01070 /** 01071 * @brief Instance structure for the floating-point FIR filter. 01072 */ 01073 typedef struct 01074 { 01075 uint16_t numTaps; /**< number of filter coefficients in the filter. */ 01076 float32_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 01077 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 01078 } arm_fir_instance_f32; 01079 01080 01081 /** 01082 * @brief Processing function for the Q7 FIR filter. 01083 * @param[in] *S points to an instance of the Q7 FIR filter structure. 01084 * @param[in] *pSrc points to the block of input data. 01085 * @param[out] *pDst points to the block of output data. 01086 * @param[in] blockSize number of samples to process. 01087 * @return none. 01088 */ 01089 void arm_fir_q7( 01090 const arm_fir_instance_q7 * S, 01091 q7_t * pSrc, 01092 q7_t * pDst, 01093 uint32_t blockSize); 01094 01095 01096 /** 01097 * @brief Initialization function for the Q7 FIR filter. 01098 * @param[in,out] *S points to an instance of the Q7 FIR structure. 01099 * @param[in] numTaps Number of filter coefficients in the filter. 01100 * @param[in] *pCoeffs points to the filter coefficients. 01101 * @param[in] *pState points to the state buffer. 01102 * @param[in] blockSize number of samples that are processed. 01103 * @return none 01104 */ 01105 void arm_fir_init_q7( 01106 arm_fir_instance_q7 * S, 01107 uint16_t numTaps, 01108 q7_t * pCoeffs, 01109 q7_t * pState, 01110 uint32_t blockSize); 01111 01112 01113 /** 01114 * @brief Processing function for the Q15 FIR filter. 01115 * @param[in] *S points to an instance of the Q15 FIR structure. 01116 * @param[in] *pSrc points to the block of input data. 01117 * @param[out] *pDst points to the block of output data. 01118 * @param[in] blockSize number of samples to process. 01119 * @return none. 01120 */ 01121 void arm_fir_q15( 01122 const arm_fir_instance_q15 * S, 01123 q15_t * pSrc, 01124 q15_t * pDst, 01125 uint32_t blockSize); 01126 01127 /** 01128 * @brief Processing function for the fast Q15 FIR filter for Cortex-M3 and Cortex-M4. 01129 * @param[in] *S points to an instance of the Q15 FIR filter structure. 01130 * @param[in] *pSrc points to the block of input data. 01131 * @param[out] *pDst points to the block of output data. 01132 * @param[in] blockSize number of samples to process. 01133 * @return none. 01134 */ 01135 void arm_fir_fast_q15( 01136 const arm_fir_instance_q15 * S, 01137 q15_t * pSrc, 01138 q15_t * pDst, 01139 uint32_t blockSize); 01140 01141 /** 01142 * @brief Initialization function for the Q15 FIR filter. 01143 * @param[in,out] *S points to an instance of the Q15 FIR filter structure. 01144 * @param[in] numTaps Number of filter coefficients in the filter. Must be even and greater than or equal to 4. 01145 * @param[in] *pCoeffs points to the filter coefficients. 01146 * @param[in] *pState points to the state buffer. 01147 * @param[in] blockSize number of samples that are processed at a time. 01148 * @return The function returns ARM_MATH_SUCCESS if initialization was successful or ARM_MATH_ARGUMENT_ERROR if 01149 * <code>numTaps</code> is not a supported value. 01150 */ 01151 01152 arm_status arm_fir_init_q15( 01153 arm_fir_instance_q15 * S, 01154 uint16_t numTaps, 01155 q15_t * pCoeffs, 01156 q15_t * pState, 01157 uint32_t blockSize); 01158 01159 /** 01160 * @brief Processing function for the Q31 FIR filter. 01161 * @param[in] *S points to an instance of the Q31 FIR filter structure. 01162 * @param[in] *pSrc points to the block of input data. 01163 * @param[out] *pDst points to the block of output data. 01164 * @param[in] blockSize number of samples to process. 01165 * @return none. 01166 */ 01167 void arm_fir_q31( 01168 const arm_fir_instance_q31 * S, 01169 q31_t * pSrc, 01170 q31_t * pDst, 01171 uint32_t blockSize); 01172 01173 /** 01174 * @brief Processing function for the fast Q31 FIR filter for Cortex-M3 and Cortex-M4. 01175 * @param[in] *S points to an instance of the Q31 FIR structure. 01176 * @param[in] *pSrc points to the block of input data. 01177 * @param[out] *pDst points to the block of output data. 01178 * @param[in] blockSize number of samples to process. 01179 * @return none. 01180 */ 01181 void arm_fir_fast_q31( 01182 const arm_fir_instance_q31 * S, 01183 q31_t * pSrc, 01184 q31_t * pDst, 01185 uint32_t blockSize); 01186 01187 /** 01188 * @brief Initialization function for the Q31 FIR filter. 01189 * @param[in,out] *S points to an instance of the Q31 FIR structure. 01190 * @param[in] numTaps Number of filter coefficients in the filter. 01191 * @param[in] *pCoeffs points to the filter coefficients. 01192 * @param[in] *pState points to the state buffer. 01193 * @param[in] blockSize number of samples that are processed at a time. 01194 * @return none. 01195 */ 01196 void arm_fir_init_q31( 01197 arm_fir_instance_q31 * S, 01198 uint16_t numTaps, 01199 q31_t * pCoeffs, 01200 q31_t * pState, 01201 uint32_t blockSize); 01202 01203 /** 01204 * @brief Processing function for the floating-point FIR filter. 01205 * @param[in] *S points to an instance of the floating-point FIR structure. 01206 * @param[in] *pSrc points to the block of input data. 01207 * @param[out] *pDst points to the block of output data. 01208 * @param[in] blockSize number of samples to process. 01209 * @return none. 01210 */ 01211 void arm_fir_f32( 01212 const arm_fir_instance_f32 * S, 01213 float32_t * pSrc, 01214 float32_t * pDst, 01215 uint32_t blockSize); 01216 01217 /** 01218 * @brief Initialization function for the floating-point FIR filter. 01219 * @param[in,out] *S points to an instance of the floating-point FIR filter structure. 01220 * @param[in] numTaps Number of filter coefficients in the filter. 01221 * @param[in] *pCoeffs points to the filter coefficients. 01222 * @param[in] *pState points to the state buffer. 01223 * @param[in] blockSize number of samples that are processed at a time. 01224 * @return none. 01225 */ 01226 void arm_fir_init_f32( 01227 arm_fir_instance_f32 * S, 01228 uint16_t numTaps, 01229 float32_t * pCoeffs, 01230 float32_t * pState, 01231 uint32_t blockSize); 01232 01233 01234 /** 01235 * @brief Instance structure for the Q15 Biquad cascade filter. 01236 */ 01237 typedef struct 01238 { 01239 int8_t numStages; /**< number of 2nd order stages in the filter. Overall order is 2*numStages. */ 01240 q15_t *pState; /**< Points to the array of state coefficients. The array is of length 4*numStages. */ 01241 q15_t *pCoeffs; /**< Points to the array of coefficients. The array is of length 5*numStages. */ 01242 int8_t postShift; /**< Additional shift, in bits, applied to each output sample. */ 01243 01244 } arm_biquad_casd_df1_inst_q15; 01245 01246 01247 /** 01248 * @brief Instance structure for the Q31 Biquad cascade filter. 01249 */ 01250 typedef struct 01251 { 01252 uint32_t numStages; /**< number of 2nd order stages in the filter. Overall order is 2*numStages. */ 01253 q31_t *pState; /**< Points to the array of state coefficients. The array is of length 4*numStages. */ 01254 q31_t *pCoeffs; /**< Points to the array of coefficients. The array is of length 5*numStages. */ 01255 uint8_t postShift; /**< Additional shift, in bits, applied to each output sample. */ 01256 01257 } arm_biquad_casd_df1_inst_q31; 01258 01259 /** 01260 * @brief Instance structure for the floating-point Biquad cascade filter. 01261 */ 01262 typedef struct 01263 { 01264 uint32_t numStages; /**< number of 2nd order stages in the filter. Overall order is 2*numStages. */ 01265 float32_t *pState; /**< Points to the array of state coefficients. The array is of length 4*numStages. */ 01266 float32_t *pCoeffs; /**< Points to the array of coefficients. The array is of length 5*numStages. */ 01267 01268 01269 } arm_biquad_casd_df1_inst_f32; 01270 01271 01272 01273 /** 01274 * @brief Processing function for the Q15 Biquad cascade filter. 01275 * @param[in] *S points to an instance of the Q15 Biquad cascade structure. 01276 * @param[in] *pSrc points to the block of input data. 01277 * @param[out] *pDst points to the block of output data. 01278 * @param[in] blockSize number of samples to process. 01279 * @return none. 01280 */ 01281 01282 void arm_biquad_cascade_df1_q15( 01283 const arm_biquad_casd_df1_inst_q15 * S, 01284 q15_t * pSrc, 01285 q15_t * pDst, 01286 uint32_t blockSize); 01287 01288 /** 01289 * @brief Initialization function for the Q15 Biquad cascade filter. 01290 * @param[in,out] *S points to an instance of the Q15 Biquad cascade structure. 01291 * @param[in] numStages number of 2nd order stages in the filter. 01292 * @param[in] *pCoeffs points to the filter coefficients. 01293 * @param[in] *pState points to the state buffer. 01294 * @param[in] postShift Shift to be applied to the output. Varies according to the coefficients format 01295 * @return none 01296 */ 01297 01298 void arm_biquad_cascade_df1_init_q15( 01299 arm_biquad_casd_df1_inst_q15 * S, 01300 uint8_t numStages, 01301 q15_t * pCoeffs, 01302 q15_t * pState, 01303 int8_t postShift); 01304 01305 01306 /** 01307 * @brief Fast but less precise processing function for the Q15 Biquad cascade filter for Cortex-M3 and Cortex-M4. 01308 * @param[in] *S points to an instance of the Q15 Biquad cascade structure. 01309 * @param[in] *pSrc points to the block of input data. 01310 * @param[out] *pDst points to the block of output data. 01311 * @param[in] blockSize number of samples to process. 01312 * @return none. 01313 */ 01314 01315 void arm_biquad_cascade_df1_fast_q15( 01316 const arm_biquad_casd_df1_inst_q15 * S, 01317 q15_t * pSrc, 01318 q15_t * pDst, 01319 uint32_t blockSize); 01320 01321 01322 /** 01323 * @brief Processing function for the Q31 Biquad cascade filter 01324 * @param[in] *S points to an instance of the Q31 Biquad cascade structure. 01325 * @param[in] *pSrc points to the block of input data. 01326 * @param[out] *pDst points to the block of output data. 01327 * @param[in] blockSize number of samples to process. 01328 * @return none. 01329 */ 01330 01331 void arm_biquad_cascade_df1_q31( 01332 const arm_biquad_casd_df1_inst_q31 * S, 01333 q31_t * pSrc, 01334 q31_t * pDst, 01335 uint32_t blockSize); 01336 01337 /** 01338 * @brief Fast but less precise processing function for the Q31 Biquad cascade filter for Cortex-M3 and Cortex-M4. 01339 * @param[in] *S points to an instance of the Q31 Biquad cascade structure. 01340 * @param[in] *pSrc points to the block of input data. 01341 * @param[out] *pDst points to the block of output data. 01342 * @param[in] blockSize number of samples to process. 01343 * @return none. 01344 */ 01345 01346 void arm_biquad_cascade_df1_fast_q31( 01347 const arm_biquad_casd_df1_inst_q31 * S, 01348 q31_t * pSrc, 01349 q31_t * pDst, 01350 uint32_t blockSize); 01351 01352 /** 01353 * @brief Initialization function for the Q31 Biquad cascade filter. 01354 * @param[in,out] *S points to an instance of the Q31 Biquad cascade structure. 01355 * @param[in] numStages number of 2nd order stages in the filter. 01356 * @param[in] *pCoeffs points to the filter coefficients. 01357 * @param[in] *pState points to the state buffer. 01358 * @param[in] postShift Shift to be applied to the output. Varies according to the coefficients format 01359 * @return none 01360 */ 01361 01362 void arm_biquad_cascade_df1_init_q31( 01363 arm_biquad_casd_df1_inst_q31 * S, 01364 uint8_t numStages, 01365 q31_t * pCoeffs, 01366 q31_t * pState, 01367 int8_t postShift); 01368 01369 /** 01370 * @brief Processing function for the floating-point Biquad cascade filter. 01371 * @param[in] *S points to an instance of the floating-point Biquad cascade structure. 01372 * @param[in] *pSrc points to the block of input data. 01373 * @param[out] *pDst points to the block of output data. 01374 * @param[in] blockSize number of samples to process. 01375 * @return none. 01376 */ 01377 01378 void arm_biquad_cascade_df1_f32( 01379 const arm_biquad_casd_df1_inst_f32 * S, 01380 float32_t * pSrc, 01381 float32_t * pDst, 01382 uint32_t blockSize); 01383 01384 /** 01385 * @brief Initialization function for the floating-point Biquad cascade filter. 01386 * @param[in,out] *S points to an instance of the floating-point Biquad cascade structure. 01387 * @param[in] numStages number of 2nd order stages in the filter. 01388 * @param[in] *pCoeffs points to the filter coefficients. 01389 * @param[in] *pState points to the state buffer. 01390 * @return none 01391 */ 01392 01393 void arm_biquad_cascade_df1_init_f32( 01394 arm_biquad_casd_df1_inst_f32 * S, 01395 uint8_t numStages, 01396 float32_t * pCoeffs, 01397 float32_t * pState); 01398 01399 01400 /** 01401 * @brief Instance structure for the floating-point matrix structure. 01402 */ 01403 01404 typedef struct 01405 { 01406 uint16_t numRows; /**< number of rows of the matrix. */ 01407 uint16_t numCols; /**< number of columns of the matrix. */ 01408 float32_t *pData; /**< points to the data of the matrix. */ 01409 } arm_matrix_instance_f32; 01410 01411 /** 01412 * @brief Instance structure for the Q15 matrix structure. 01413 */ 01414 01415 typedef struct 01416 { 01417 uint16_t numRows; /**< number of rows of the matrix. */ 01418 uint16_t numCols; /**< number of columns of the matrix. */ 01419 q15_t *pData; /**< points to the data of the matrix. */ 01420 01421 } arm_matrix_instance_q15; 01422 01423 /** 01424 * @brief Instance structure for the Q31 matrix structure. 01425 */ 01426 01427 typedef struct 01428 { 01429 uint16_t numRows; /**< number of rows of the matrix. */ 01430 uint16_t numCols; /**< number of columns of the matrix. */ 01431 q31_t *pData; /**< points to the data of the matrix. */ 01432 01433 } arm_matrix_instance_q31; 01434 01435 01436 01437 /** 01438 * @brief Floating-point matrix addition. 01439 * @param[in] *pSrcA points to the first input matrix structure 01440 * @param[in] *pSrcB points to the second input matrix structure 01441 * @param[out] *pDst points to output matrix structure 01442 * @return The function returns either 01443 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01444 */ 01445 01446 arm_status arm_mat_add_f32( 01447 const arm_matrix_instance_f32 * pSrcA, 01448 const arm_matrix_instance_f32 * pSrcB, 01449 arm_matrix_instance_f32 * pDst); 01450 01451 /** 01452 * @brief Q15 matrix addition. 01453 * @param[in] *pSrcA points to the first input matrix structure 01454 * @param[in] *pSrcB points to the second input matrix structure 01455 * @param[out] *pDst points to output matrix structure 01456 * @return The function returns either 01457 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01458 */ 01459 01460 arm_status arm_mat_add_q15( 01461 const arm_matrix_instance_q15 * pSrcA, 01462 const arm_matrix_instance_q15 * pSrcB, 01463 arm_matrix_instance_q15 * pDst); 01464 01465 /** 01466 * @brief Q31 matrix addition. 01467 * @param[in] *pSrcA points to the first input matrix structure 01468 * @param[in] *pSrcB points to the second input matrix structure 01469 * @param[out] *pDst points to output matrix structure 01470 * @return The function returns either 01471 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01472 */ 01473 01474 arm_status arm_mat_add_q31( 01475 const arm_matrix_instance_q31 * pSrcA, 01476 const arm_matrix_instance_q31 * pSrcB, 01477 arm_matrix_instance_q31 * pDst); 01478 01479 01480 /** 01481 * @brief Floating-point matrix transpose. 01482 * @param[in] *pSrc points to the input matrix 01483 * @param[out] *pDst points to the output matrix 01484 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 01485 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01486 */ 01487 01488 arm_status arm_mat_trans_f32( 01489 const arm_matrix_instance_f32 * pSrc, 01490 arm_matrix_instance_f32 * pDst); 01491 01492 01493 /** 01494 * @brief Q15 matrix transpose. 01495 * @param[in] *pSrc points to the input matrix 01496 * @param[out] *pDst points to the output matrix 01497 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 01498 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01499 */ 01500 01501 arm_status arm_mat_trans_q15( 01502 const arm_matrix_instance_q15 * pSrc, 01503 arm_matrix_instance_q15 * pDst); 01504 01505 /** 01506 * @brief Q31 matrix transpose. 01507 * @param[in] *pSrc points to the input matrix 01508 * @param[out] *pDst points to the output matrix 01509 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 01510 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01511 */ 01512 01513 arm_status arm_mat_trans_q31( 01514 const arm_matrix_instance_q31 * pSrc, 01515 arm_matrix_instance_q31 * pDst); 01516 01517 01518 /** 01519 * @brief Floating-point matrix multiplication 01520 * @param[in] *pSrcA points to the first input matrix structure 01521 * @param[in] *pSrcB points to the second input matrix structure 01522 * @param[out] *pDst points to output matrix structure 01523 * @return The function returns either 01524 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01525 */ 01526 01527 arm_status arm_mat_mult_f32( 01528 const arm_matrix_instance_f32 * pSrcA, 01529 const arm_matrix_instance_f32 * pSrcB, 01530 arm_matrix_instance_f32 * pDst); 01531 01532 /** 01533 * @brief Q15 matrix multiplication 01534 * @param[in] *pSrcA points to the first input matrix structure 01535 * @param[in] *pSrcB points to the second input matrix structure 01536 * @param[out] *pDst points to output matrix structure 01537 * @param[in] *pState points to the array for storing intermediate results 01538 * @return The function returns either 01539 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01540 */ 01541 01542 arm_status arm_mat_mult_q15( 01543 const arm_matrix_instance_q15 * pSrcA, 01544 const arm_matrix_instance_q15 * pSrcB, 01545 arm_matrix_instance_q15 * pDst, 01546 q15_t * pState); 01547 01548 /** 01549 * @brief Q15 matrix multiplication (fast variant) for Cortex-M3 and Cortex-M4 01550 * @param[in] *pSrcA points to the first input matrix structure 01551 * @param[in] *pSrcB points to the second input matrix structure 01552 * @param[out] *pDst points to output matrix structure 01553 * @param[in] *pState points to the array for storing intermediate results 01554 * @return The function returns either 01555 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01556 */ 01557 01558 arm_status arm_mat_mult_fast_q15( 01559 const arm_matrix_instance_q15 * pSrcA, 01560 const arm_matrix_instance_q15 * pSrcB, 01561 arm_matrix_instance_q15 * pDst, 01562 q15_t * pState); 01563 01564 /** 01565 * @brief Q31 matrix multiplication 01566 * @param[in] *pSrcA points to the first input matrix structure 01567 * @param[in] *pSrcB points to the second input matrix structure 01568 * @param[out] *pDst points to output matrix structure 01569 * @return The function returns either 01570 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01571 */ 01572 01573 arm_status arm_mat_mult_q31( 01574 const arm_matrix_instance_q31 * pSrcA, 01575 const arm_matrix_instance_q31 * pSrcB, 01576 arm_matrix_instance_q31 * pDst); 01577 01578 /** 01579 * @brief Q31 matrix multiplication (fast variant) for Cortex-M3 and Cortex-M4 01580 * @param[in] *pSrcA points to the first input matrix structure 01581 * @param[in] *pSrcB points to the second input matrix structure 01582 * @param[out] *pDst points to output matrix structure 01583 * @return The function returns either 01584 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01585 */ 01586 01587 arm_status arm_mat_mult_fast_q31( 01588 const arm_matrix_instance_q31 * pSrcA, 01589 const arm_matrix_instance_q31 * pSrcB, 01590 arm_matrix_instance_q31 * pDst); 01591 01592 01593 /** 01594 * @brief Floating-point matrix subtraction 01595 * @param[in] *pSrcA points to the first input matrix structure 01596 * @param[in] *pSrcB points to the second input matrix structure 01597 * @param[out] *pDst points to output matrix structure 01598 * @return The function returns either 01599 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01600 */ 01601 01602 arm_status arm_mat_sub_f32( 01603 const arm_matrix_instance_f32 * pSrcA, 01604 const arm_matrix_instance_f32 * pSrcB, 01605 arm_matrix_instance_f32 * pDst); 01606 01607 /** 01608 * @brief Q15 matrix subtraction 01609 * @param[in] *pSrcA points to the first input matrix structure 01610 * @param[in] *pSrcB points to the second input matrix structure 01611 * @param[out] *pDst points to output matrix structure 01612 * @return The function returns either 01613 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01614 */ 01615 01616 arm_status arm_mat_sub_q15( 01617 const arm_matrix_instance_q15 * pSrcA, 01618 const arm_matrix_instance_q15 * pSrcB, 01619 arm_matrix_instance_q15 * pDst); 01620 01621 /** 01622 * @brief Q31 matrix subtraction 01623 * @param[in] *pSrcA points to the first input matrix structure 01624 * @param[in] *pSrcB points to the second input matrix structure 01625 * @param[out] *pDst points to output matrix structure 01626 * @return The function returns either 01627 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01628 */ 01629 01630 arm_status arm_mat_sub_q31( 01631 const arm_matrix_instance_q31 * pSrcA, 01632 const arm_matrix_instance_q31 * pSrcB, 01633 arm_matrix_instance_q31 * pDst); 01634 01635 /** 01636 * @brief Floating-point matrix scaling. 01637 * @param[in] *pSrc points to the input matrix 01638 * @param[in] scale scale factor 01639 * @param[out] *pDst points to the output matrix 01640 * @return The function returns either 01641 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01642 */ 01643 01644 arm_status arm_mat_scale_f32( 01645 const arm_matrix_instance_f32 * pSrc, 01646 float32_t scale, 01647 arm_matrix_instance_f32 * pDst); 01648 01649 /** 01650 * @brief Q15 matrix scaling. 01651 * @param[in] *pSrc points to input matrix 01652 * @param[in] scaleFract fractional portion of the scale factor 01653 * @param[in] shift number of bits to shift the result by 01654 * @param[out] *pDst points to output matrix 01655 * @return The function returns either 01656 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01657 */ 01658 01659 arm_status arm_mat_scale_q15( 01660 const arm_matrix_instance_q15 * pSrc, 01661 q15_t scaleFract, 01662 int32_t shift, 01663 arm_matrix_instance_q15 * pDst); 01664 01665 /** 01666 * @brief Q31 matrix scaling. 01667 * @param[in] *pSrc points to input matrix 01668 * @param[in] scaleFract fractional portion of the scale factor 01669 * @param[in] shift number of bits to shift the result by 01670 * @param[out] *pDst points to output matrix structure 01671 * @return The function returns either 01672 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 01673 */ 01674 01675 arm_status arm_mat_scale_q31( 01676 const arm_matrix_instance_q31 * pSrc, 01677 q31_t scaleFract, 01678 int32_t shift, 01679 arm_matrix_instance_q31 * pDst); 01680 01681 01682 /** 01683 * @brief Q31 matrix initialization. 01684 * @param[in,out] *S points to an instance of the floating-point matrix structure. 01685 * @param[in] nRows number of rows in the matrix. 01686 * @param[in] nColumns number of columns in the matrix. 01687 * @param[in] *pData points to the matrix data array. 01688 * @return none 01689 */ 01690 01691 void arm_mat_init_q31( 01692 arm_matrix_instance_q31 * S, 01693 uint16_t nRows, 01694 uint16_t nColumns, 01695 q31_t * pData); 01696 01697 /** 01698 * @brief Q15 matrix initialization. 01699 * @param[in,out] *S points to an instance of the floating-point matrix structure. 01700 * @param[in] nRows number of rows in the matrix. 01701 * @param[in] nColumns number of columns in the matrix. 01702 * @param[in] *pData points to the matrix data array. 01703 * @return none 01704 */ 01705 01706 void arm_mat_init_q15( 01707 arm_matrix_instance_q15 * S, 01708 uint16_t nRows, 01709 uint16_t nColumns, 01710 q15_t * pData); 01711 01712 /** 01713 * @brief Floating-point matrix initialization. 01714 * @param[in,out] *S points to an instance of the floating-point matrix structure. 01715 * @param[in] nRows number of rows in the matrix. 01716 * @param[in] nColumns number of columns in the matrix. 01717 * @param[in] *pData points to the matrix data array. 01718 * @return none 01719 */ 01720 01721 void arm_mat_init_f32( 01722 arm_matrix_instance_f32 * S, 01723 uint16_t nRows, 01724 uint16_t nColumns, 01725 float32_t * pData); 01726 01727 01728 01729 /** 01730 * @brief Instance structure for the Q15 PID Control. 01731 */ 01732 typedef struct 01733 { 01734 q15_t A0; /**< The derived gain, A0 = Kp + Ki + Kd . */ 01735 #ifdef ARM_MATH_CM0_FAMILY 01736 q15_t A1; 01737 q15_t A2; 01738 #else 01739 q31_t A1; /**< The derived gain A1 = -Kp - 2Kd | Kd.*/ 01740 #endif 01741 q15_t state[3]; /**< The state array of length 3. */ 01742 q15_t Kp; /**< The proportional gain. */ 01743 q15_t Ki; /**< The integral gain. */ 01744 q15_t Kd; /**< The derivative gain. */ 01745 } arm_pid_instance_q15; 01746 01747 /** 01748 * @brief Instance structure for the Q31 PID Control. 01749 */ 01750 typedef struct 01751 { 01752 q31_t A0; /**< The derived gain, A0 = Kp + Ki + Kd . */ 01753 q31_t A1; /**< The derived gain, A1 = -Kp - 2Kd. */ 01754 q31_t A2; /**< The derived gain, A2 = Kd . */ 01755 q31_t state[3]; /**< The state array of length 3. */ 01756 q31_t Kp; /**< The proportional gain. */ 01757 q31_t Ki; /**< The integral gain. */ 01758 q31_t Kd; /**< The derivative gain. */ 01759 01760 } arm_pid_instance_q31; 01761 01762 /** 01763 * @brief Instance structure for the floating-point PID Control. 01764 */ 01765 typedef struct 01766 { 01767 float32_t A0; /**< The derived gain, A0 = Kp + Ki + Kd . */ 01768 float32_t A1; /**< The derived gain, A1 = -Kp - 2Kd. */ 01769 float32_t A2; /**< The derived gain, A2 = Kd . */ 01770 float32_t state[3]; /**< The state array of length 3. */ 01771 float32_t Kp; /**< The proportional gain. */ 01772 float32_t Ki; /**< The integral gain. */ 01773 float32_t Kd; /**< The derivative gain. */ 01774 } arm_pid_instance_f32; 01775 01776 01777 01778 /** 01779 * @brief Initialization function for the floating-point PID Control. 01780 * @param[in,out] *S points to an instance of the PID structure. 01781 * @param[in] resetStateFlag flag to reset the state. 0 = no change in state 1 = reset the state. 01782 * @return none. 01783 */ 01784 void arm_pid_init_f32( 01785 arm_pid_instance_f32 * S, 01786 int32_t resetStateFlag); 01787 01788 /** 01789 * @brief Reset function for the floating-point PID Control. 01790 * @param[in,out] *S is an instance of the floating-point PID Control structure 01791 * @return none 01792 */ 01793 void arm_pid_reset_f32( 01794 arm_pid_instance_f32 * S); 01795 01796 01797 /** 01798 * @brief Initialization function for the Q31 PID Control. 01799 * @param[in,out] *S points to an instance of the Q15 PID structure. 01800 * @param[in] resetStateFlag flag to reset the state. 0 = no change in state 1 = reset the state. 01801 * @return none. 01802 */ 01803 void arm_pid_init_q31( 01804 arm_pid_instance_q31 * S, 01805 int32_t resetStateFlag); 01806 01807 01808 /** 01809 * @brief Reset function for the Q31 PID Control. 01810 * @param[in,out] *S points to an instance of the Q31 PID Control structure 01811 * @return none 01812 */ 01813 01814 void arm_pid_reset_q31( 01815 arm_pid_instance_q31 * S); 01816 01817 /** 01818 * @brief Initialization function for the Q15 PID Control. 01819 * @param[in,out] *S points to an instance of the Q15 PID structure. 01820 * @param[in] resetStateFlag flag to reset the state. 0 = no change in state 1 = reset the state. 01821 * @return none. 01822 */ 01823 void arm_pid_init_q15( 01824 arm_pid_instance_q15 * S, 01825 int32_t resetStateFlag); 01826 01827 /** 01828 * @brief Reset function for the Q15 PID Control. 01829 * @param[in,out] *S points to an instance of the q15 PID Control structure 01830 * @return none 01831 */ 01832 void arm_pid_reset_q15( 01833 arm_pid_instance_q15 * S); 01834 01835 01836 /** 01837 * @brief Instance structure for the floating-point Linear Interpolate function. 01838 */ 01839 typedef struct 01840 { 01841 uint32_t nValues; /**< nValues */ 01842 float32_t x1; /**< x1 */ 01843 float32_t xSpacing; /**< xSpacing */ 01844 float32_t *pYData; /**< pointer to the table of Y values */ 01845 } arm_linear_interp_instance_f32; 01846 01847 /** 01848 * @brief Instance structure for the floating-point bilinear interpolation function. 01849 */ 01850 01851 typedef struct 01852 { 01853 uint16_t numRows; /**< number of rows in the data table. */ 01854 uint16_t numCols; /**< number of columns in the data table. */ 01855 float32_t *pData; /**< points to the data table. */ 01856 } arm_bilinear_interp_instance_f32; 01857 01858 /** 01859 * @brief Instance structure for the Q31 bilinear interpolation function. 01860 */ 01861 01862 typedef struct 01863 { 01864 uint16_t numRows; /**< number of rows in the data table. */ 01865 uint16_t numCols; /**< number of columns in the data table. */ 01866 q31_t *pData; /**< points to the data table. */ 01867 } arm_bilinear_interp_instance_q31; 01868 01869 /** 01870 * @brief Instance structure for the Q15 bilinear interpolation function. 01871 */ 01872 01873 typedef struct 01874 { 01875 uint16_t numRows; /**< number of rows in the data table. */ 01876 uint16_t numCols; /**< number of columns in the data table. */ 01877 q15_t *pData; /**< points to the data table. */ 01878 } arm_bilinear_interp_instance_q15; 01879 01880 /** 01881 * @brief Instance structure for the Q15 bilinear interpolation function. 01882 */ 01883 01884 typedef struct 01885 { 01886 uint16_t numRows; /**< number of rows in the data table. */ 01887 uint16_t numCols; /**< number of columns in the data table. */ 01888 q7_t *pData; /**< points to the data table. */ 01889 } arm_bilinear_interp_instance_q7; 01890 01891 01892 /** 01893 * @brief Q7 vector multiplication. 01894 * @param[in] *pSrcA points to the first input vector 01895 * @param[in] *pSrcB points to the second input vector 01896 * @param[out] *pDst points to the output vector 01897 * @param[in] blockSize number of samples in each vector 01898 * @return none. 01899 */ 01900 01901 void arm_mult_q7( 01902 q7_t * pSrcA, 01903 q7_t * pSrcB, 01904 q7_t * pDst, 01905 uint32_t blockSize); 01906 01907 /** 01908 * @brief Q15 vector multiplication. 01909 * @param[in] *pSrcA points to the first input vector 01910 * @param[in] *pSrcB points to the second input vector 01911 * @param[out] *pDst points to the output vector 01912 * @param[in] blockSize number of samples in each vector 01913 * @return none. 01914 */ 01915 01916 void arm_mult_q15( 01917 q15_t * pSrcA, 01918 q15_t * pSrcB, 01919 q15_t * pDst, 01920 uint32_t blockSize); 01921 01922 /** 01923 * @brief Q31 vector multiplication. 01924 * @param[in] *pSrcA points to the first input vector 01925 * @param[in] *pSrcB points to the second input vector 01926 * @param[out] *pDst points to the output vector 01927 * @param[in] blockSize number of samples in each vector 01928 * @return none. 01929 */ 01930 01931 void arm_mult_q31( 01932 q31_t * pSrcA, 01933 q31_t * pSrcB, 01934 q31_t * pDst, 01935 uint32_t blockSize); 01936 01937 /** 01938 * @brief Floating-point vector multiplication. 01939 * @param[in] *pSrcA points to the first input vector 01940 * @param[in] *pSrcB points to the second input vector 01941 * @param[out] *pDst points to the output vector 01942 * @param[in] blockSize number of samples in each vector 01943 * @return none. 01944 */ 01945 01946 void arm_mult_f32( 01947 float32_t * pSrcA, 01948 float32_t * pSrcB, 01949 float32_t * pDst, 01950 uint32_t blockSize); 01951 01952 01953 01954 01955 01956 01957 /** 01958 * @brief Instance structure for the Q15 CFFT/CIFFT function. 01959 */ 01960 01961 typedef struct 01962 { 01963 uint16_t fftLen; /**< length of the FFT. */ 01964 uint8_t ifftFlag; /**< flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. */ 01965 uint8_t bitReverseFlag; /**< flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. */ 01966 q15_t *pTwiddle; /**< points to the Sin twiddle factor table. */ 01967 uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 01968 uint16_t twidCoefModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 01969 uint16_t bitRevFactor; /**< bit reversal modifier that supports different size FFTs with the same bit reversal table. */ 01970 } arm_cfft_radix2_instance_q15; 01971 01972 arm_status arm_cfft_radix2_init_q15( 01973 arm_cfft_radix2_instance_q15 * S, 01974 uint16_t fftLen, 01975 uint8_t ifftFlag, 01976 uint8_t bitReverseFlag); 01977 01978 void arm_cfft_radix2_q15( 01979 const arm_cfft_radix2_instance_q15 * S, 01980 q15_t * pSrc); 01981 01982 01983 01984 /** 01985 * @brief Instance structure for the Q15 CFFT/CIFFT function. 01986 */ 01987 01988 typedef struct 01989 { 01990 uint16_t fftLen; /**< length of the FFT. */ 01991 uint8_t ifftFlag; /**< flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. */ 01992 uint8_t bitReverseFlag; /**< flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. */ 01993 q15_t *pTwiddle; /**< points to the twiddle factor table. */ 01994 uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 01995 uint16_t twidCoefModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 01996 uint16_t bitRevFactor; /**< bit reversal modifier that supports different size FFTs with the same bit reversal table. */ 01997 } arm_cfft_radix4_instance_q15; 01998 01999 arm_status arm_cfft_radix4_init_q15( 02000 arm_cfft_radix4_instance_q15 * S, 02001 uint16_t fftLen, 02002 uint8_t ifftFlag, 02003 uint8_t bitReverseFlag); 02004 02005 void arm_cfft_radix4_q15( 02006 const arm_cfft_radix4_instance_q15 * S, 02007 q15_t * pSrc); 02008 02009 /** 02010 * @brief Instance structure for the Radix-2 Q31 CFFT/CIFFT function. 02011 */ 02012 02013 typedef struct 02014 { 02015 uint16_t fftLen; /**< length of the FFT. */ 02016 uint8_t ifftFlag; /**< flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. */ 02017 uint8_t bitReverseFlag; /**< flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. */ 02018 q31_t *pTwiddle; /**< points to the Twiddle factor table. */ 02019 uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 02020 uint16_t twidCoefModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02021 uint16_t bitRevFactor; /**< bit reversal modifier that supports different size FFTs with the same bit reversal table. */ 02022 } arm_cfft_radix2_instance_q31; 02023 02024 arm_status arm_cfft_radix2_init_q31( 02025 arm_cfft_radix2_instance_q31 * S, 02026 uint16_t fftLen, 02027 uint8_t ifftFlag, 02028 uint8_t bitReverseFlag); 02029 02030 void arm_cfft_radix2_q31( 02031 const arm_cfft_radix2_instance_q31 * S, 02032 q31_t * pSrc); 02033 02034 /** 02035 * @brief Instance structure for the Q31 CFFT/CIFFT function. 02036 */ 02037 02038 typedef struct 02039 { 02040 uint16_t fftLen; /**< length of the FFT. */ 02041 uint8_t ifftFlag; /**< flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. */ 02042 uint8_t bitReverseFlag; /**< flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. */ 02043 q31_t *pTwiddle; /**< points to the twiddle factor table. */ 02044 uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 02045 uint16_t twidCoefModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02046 uint16_t bitRevFactor; /**< bit reversal modifier that supports different size FFTs with the same bit reversal table. */ 02047 } arm_cfft_radix4_instance_q31; 02048 02049 02050 void arm_cfft_radix4_q31( 02051 const arm_cfft_radix4_instance_q31 * S, 02052 q31_t * pSrc); 02053 02054 arm_status arm_cfft_radix4_init_q31( 02055 arm_cfft_radix4_instance_q31 * S, 02056 uint16_t fftLen, 02057 uint8_t ifftFlag, 02058 uint8_t bitReverseFlag); 02059 02060 /** 02061 * @brief Instance structure for the floating-point CFFT/CIFFT function. 02062 */ 02063 02064 typedef struct 02065 { 02066 uint16_t fftLen; /**< length of the FFT. */ 02067 uint8_t ifftFlag; /**< flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. */ 02068 uint8_t bitReverseFlag; /**< flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. */ 02069 float32_t *pTwiddle; /**< points to the Twiddle factor table. */ 02070 uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 02071 uint16_t twidCoefModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02072 uint16_t bitRevFactor; /**< bit reversal modifier that supports different size FFTs with the same bit reversal table. */ 02073 float32_t onebyfftLen; /**< value of 1/fftLen. */ 02074 } arm_cfft_radix2_instance_f32; 02075 02076 /* Deprecated */ 02077 arm_status arm_cfft_radix2_init_f32( 02078 arm_cfft_radix2_instance_f32 * S, 02079 uint16_t fftLen, 02080 uint8_t ifftFlag, 02081 uint8_t bitReverseFlag); 02082 02083 /* Deprecated */ 02084 void arm_cfft_radix2_f32( 02085 const arm_cfft_radix2_instance_f32 * S, 02086 float32_t * pSrc); 02087 02088 /** 02089 * @brief Instance structure for the floating-point CFFT/CIFFT function. 02090 */ 02091 02092 typedef struct 02093 { 02094 uint16_t fftLen; /**< length of the FFT. */ 02095 uint8_t ifftFlag; /**< flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. */ 02096 uint8_t bitReverseFlag; /**< flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. */ 02097 float32_t *pTwiddle; /**< points to the Twiddle factor table. */ 02098 uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 02099 uint16_t twidCoefModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02100 uint16_t bitRevFactor; /**< bit reversal modifier that supports different size FFTs with the same bit reversal table. */ 02101 float32_t onebyfftLen; /**< value of 1/fftLen. */ 02102 } arm_cfft_radix4_instance_f32; 02103 02104 /* Deprecated */ 02105 arm_status arm_cfft_radix4_init_f32( 02106 arm_cfft_radix4_instance_f32 * S, 02107 uint16_t fftLen, 02108 uint8_t ifftFlag, 02109 uint8_t bitReverseFlag); 02110 02111 /* Deprecated */ 02112 void arm_cfft_radix4_f32( 02113 const arm_cfft_radix4_instance_f32 * S, 02114 float32_t * pSrc); 02115 02116 /** 02117 * @brief Instance structure for the floating-point CFFT/CIFFT function. 02118 */ 02119 02120 typedef struct 02121 { 02122 uint16_t fftLen; /**< length of the FFT. */ 02123 const float32_t *pTwiddle; /**< points to the Twiddle factor table. */ 02124 const uint16_t *pBitRevTable; /**< points to the bit reversal table. */ 02125 uint16_t bitRevLength; /**< bit reversal table length. */ 02126 } arm_cfft_instance_f32; 02127 02128 void arm_cfft_f32( 02129 const arm_cfft_instance_f32 * S, 02130 float32_t * p1, 02131 uint8_t ifftFlag, 02132 uint8_t bitReverseFlag); 02133 02134 /** 02135 * @brief Instance structure for the Q15 RFFT/RIFFT function. 02136 */ 02137 02138 typedef struct 02139 { 02140 uint32_t fftLenReal; /**< length of the real FFT. */ 02141 uint32_t fftLenBy2; /**< length of the complex FFT. */ 02142 uint8_t ifftFlagR; /**< flag that selects forward (ifftFlagR=0) or inverse (ifftFlagR=1) transform. */ 02143 uint8_t bitReverseFlagR; /**< flag that enables (bitReverseFlagR=1) or disables (bitReverseFlagR=0) bit reversal of output. */ 02144 uint32_t twidCoefRModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02145 q15_t *pTwiddleAReal; /**< points to the real twiddle factor table. */ 02146 q15_t *pTwiddleBReal; /**< points to the imag twiddle factor table. */ 02147 arm_cfft_radix4_instance_q15 *pCfft; /**< points to the complex FFT instance. */ 02148 } arm_rfft_instance_q15; 02149 02150 arm_status arm_rfft_init_q15( 02151 arm_rfft_instance_q15 * S, 02152 arm_cfft_radix4_instance_q15 * S_CFFT, 02153 uint32_t fftLenReal, 02154 uint32_t ifftFlagR, 02155 uint32_t bitReverseFlag); 02156 02157 void arm_rfft_q15( 02158 const arm_rfft_instance_q15 * S, 02159 q15_t * pSrc, 02160 q15_t * pDst); 02161 02162 /** 02163 * @brief Instance structure for the Q31 RFFT/RIFFT function. 02164 */ 02165 02166 typedef struct 02167 { 02168 uint32_t fftLenReal; /**< length of the real FFT. */ 02169 uint32_t fftLenBy2; /**< length of the complex FFT. */ 02170 uint8_t ifftFlagR; /**< flag that selects forward (ifftFlagR=0) or inverse (ifftFlagR=1) transform. */ 02171 uint8_t bitReverseFlagR; /**< flag that enables (bitReverseFlagR=1) or disables (bitReverseFlagR=0) bit reversal of output. */ 02172 uint32_t twidCoefRModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02173 q31_t *pTwiddleAReal; /**< points to the real twiddle factor table. */ 02174 q31_t *pTwiddleBReal; /**< points to the imag twiddle factor table. */ 02175 arm_cfft_radix4_instance_q31 *pCfft; /**< points to the complex FFT instance. */ 02176 } arm_rfft_instance_q31; 02177 02178 arm_status arm_rfft_init_q31( 02179 arm_rfft_instance_q31 * S, 02180 arm_cfft_radix4_instance_q31 * S_CFFT, 02181 uint32_t fftLenReal, 02182 uint32_t ifftFlagR, 02183 uint32_t bitReverseFlag); 02184 02185 void arm_rfft_q31( 02186 const arm_rfft_instance_q31 * S, 02187 q31_t * pSrc, 02188 q31_t * pDst); 02189 02190 /** 02191 * @brief Instance structure for the floating-point RFFT/RIFFT function. 02192 */ 02193 02194 typedef struct 02195 { 02196 uint32_t fftLenReal; /**< length of the real FFT. */ 02197 uint16_t fftLenBy2; /**< length of the complex FFT. */ 02198 uint8_t ifftFlagR; /**< flag that selects forward (ifftFlagR=0) or inverse (ifftFlagR=1) transform. */ 02199 uint8_t bitReverseFlagR; /**< flag that enables (bitReverseFlagR=1) or disables (bitReverseFlagR=0) bit reversal of output. */ 02200 uint32_t twidCoefRModifier; /**< twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. */ 02201 float32_t *pTwiddleAReal; /**< points to the real twiddle factor table. */ 02202 float32_t *pTwiddleBReal; /**< points to the imag twiddle factor table. */ 02203 arm_cfft_radix4_instance_f32 *pCfft; /**< points to the complex FFT instance. */ 02204 } arm_rfft_instance_f32; 02205 02206 arm_status arm_rfft_init_f32( 02207 arm_rfft_instance_f32 * S, 02208 arm_cfft_radix4_instance_f32 * S_CFFT, 02209 uint32_t fftLenReal, 02210 uint32_t ifftFlagR, 02211 uint32_t bitReverseFlag); 02212 02213 void arm_rfft_f32( 02214 const arm_rfft_instance_f32 * S, 02215 float32_t * pSrc, 02216 float32_t * pDst); 02217 02218 /** 02219 * @brief Instance structure for the floating-point RFFT/RIFFT function. 02220 */ 02221 02222 typedef struct 02223 { 02224 arm_cfft_instance_f32 Sint; /**< Internal CFFT structure. */ 02225 uint16_t fftLenRFFT; /**< length of the real sequence */ 02226 float32_t * pTwiddleRFFT; /**< Twiddle factors real stage */ 02227 } arm_rfft_fast_instance_f32 ; 02228 02229 arm_status arm_rfft_fast_init_f32 ( 02230 arm_rfft_fast_instance_f32 * S, 02231 uint16_t fftLen); 02232 02233 void arm_rfft_fast_f32( 02234 arm_rfft_fast_instance_f32 * S, 02235 float32_t * p, float32_t * pOut, 02236 uint8_t ifftFlag); 02237 02238 /** 02239 * @brief Instance structure for the floating-point DCT4/IDCT4 function. 02240 */ 02241 02242 typedef struct 02243 { 02244 uint16_t N; /**< length of the DCT4. */ 02245 uint16_t Nby2; /**< half of the length of the DCT4. */ 02246 float32_t normalize; /**< normalizing factor. */ 02247 float32_t *pTwiddle; /**< points to the twiddle factor table. */ 02248 float32_t *pCosFactor; /**< points to the cosFactor table. */ 02249 arm_rfft_instance_f32 *pRfft; /**< points to the real FFT instance. */ 02250 arm_cfft_radix4_instance_f32 *pCfft; /**< points to the complex FFT instance. */ 02251 } arm_dct4_instance_f32; 02252 02253 /** 02254 * @brief Initialization function for the floating-point DCT4/IDCT4. 02255 * @param[in,out] *S points to an instance of floating-point DCT4/IDCT4 structure. 02256 * @param[in] *S_RFFT points to an instance of floating-point RFFT/RIFFT structure. 02257 * @param[in] *S_CFFT points to an instance of floating-point CFFT/CIFFT structure. 02258 * @param[in] N length of the DCT4. 02259 * @param[in] Nby2 half of the length of the DCT4. 02260 * @param[in] normalize normalizing factor. 02261 * @return arm_status function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>fftLenReal</code> is not a supported transform length. 02262 */ 02263 02264 arm_status arm_dct4_init_f32( 02265 arm_dct4_instance_f32 * S, 02266 arm_rfft_instance_f32 * S_RFFT, 02267 arm_cfft_radix4_instance_f32 * S_CFFT, 02268 uint16_t N, 02269 uint16_t Nby2, 02270 float32_t normalize); 02271 02272 /** 02273 * @brief Processing function for the floating-point DCT4/IDCT4. 02274 * @param[in] *S points to an instance of the floating-point DCT4/IDCT4 structure. 02275 * @param[in] *pState points to state buffer. 02276 * @param[in,out] *pInlineBuffer points to the in-place input and output buffer. 02277 * @return none. 02278 */ 02279 02280 void arm_dct4_f32( 02281 const arm_dct4_instance_f32 * S, 02282 float32_t * pState, 02283 float32_t * pInlineBuffer); 02284 02285 /** 02286 * @brief Instance structure for the Q31 DCT4/IDCT4 function. 02287 */ 02288 02289 typedef struct 02290 { 02291 uint16_t N; /**< length of the DCT4. */ 02292 uint16_t Nby2; /**< half of the length of the DCT4. */ 02293 q31_t normalize; /**< normalizing factor. */ 02294 q31_t *pTwiddle; /**< points to the twiddle factor table. */ 02295 q31_t *pCosFactor; /**< points to the cosFactor table. */ 02296 arm_rfft_instance_q31 *pRfft; /**< points to the real FFT instance. */ 02297 arm_cfft_radix4_instance_q31 *pCfft; /**< points to the complex FFT instance. */ 02298 } arm_dct4_instance_q31; 02299 02300 /** 02301 * @brief Initialization function for the Q31 DCT4/IDCT4. 02302 * @param[in,out] *S points to an instance of Q31 DCT4/IDCT4 structure. 02303 * @param[in] *S_RFFT points to an instance of Q31 RFFT/RIFFT structure 02304 * @param[in] *S_CFFT points to an instance of Q31 CFFT/CIFFT structure 02305 * @param[in] N length of the DCT4. 02306 * @param[in] Nby2 half of the length of the DCT4. 02307 * @param[in] normalize normalizing factor. 02308 * @return arm_status function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>N</code> is not a supported transform length. 02309 */ 02310 02311 arm_status arm_dct4_init_q31( 02312 arm_dct4_instance_q31 * S, 02313 arm_rfft_instance_q31 * S_RFFT, 02314 arm_cfft_radix4_instance_q31 * S_CFFT, 02315 uint16_t N, 02316 uint16_t Nby2, 02317 q31_t normalize); 02318 02319 /** 02320 * @brief Processing function for the Q31 DCT4/IDCT4. 02321 * @param[in] *S points to an instance of the Q31 DCT4 structure. 02322 * @param[in] *pState points to state buffer. 02323 * @param[in,out] *pInlineBuffer points to the in-place input and output buffer. 02324 * @return none. 02325 */ 02326 02327 void arm_dct4_q31( 02328 const arm_dct4_instance_q31 * S, 02329 q31_t * pState, 02330 q31_t * pInlineBuffer); 02331 02332 /** 02333 * @brief Instance structure for the Q15 DCT4/IDCT4 function. 02334 */ 02335 02336 typedef struct 02337 { 02338 uint16_t N; /**< length of the DCT4. */ 02339 uint16_t Nby2; /**< half of the length of the DCT4. */ 02340 q15_t normalize; /**< normalizing factor. */ 02341 q15_t *pTwiddle; /**< points to the twiddle factor table. */ 02342 q15_t *pCosFactor; /**< points to the cosFactor table. */ 02343 arm_rfft_instance_q15 *pRfft; /**< points to the real FFT instance. */ 02344 arm_cfft_radix4_instance_q15 *pCfft; /**< points to the complex FFT instance. */ 02345 } arm_dct4_instance_q15; 02346 02347 /** 02348 * @brief Initialization function for the Q15 DCT4/IDCT4. 02349 * @param[in,out] *S points to an instance of Q15 DCT4/IDCT4 structure. 02350 * @param[in] *S_RFFT points to an instance of Q15 RFFT/RIFFT structure. 02351 * @param[in] *S_CFFT points to an instance of Q15 CFFT/CIFFT structure. 02352 * @param[in] N length of the DCT4. 02353 * @param[in] Nby2 half of the length of the DCT4. 02354 * @param[in] normalize normalizing factor. 02355 * @return arm_status function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>N</code> is not a supported transform length. 02356 */ 02357 02358 arm_status arm_dct4_init_q15( 02359 arm_dct4_instance_q15 * S, 02360 arm_rfft_instance_q15 * S_RFFT, 02361 arm_cfft_radix4_instance_q15 * S_CFFT, 02362 uint16_t N, 02363 uint16_t Nby2, 02364 q15_t normalize); 02365 02366 /** 02367 * @brief Processing function for the Q15 DCT4/IDCT4. 02368 * @param[in] *S points to an instance of the Q15 DCT4 structure. 02369 * @param[in] *pState points to state buffer. 02370 * @param[in,out] *pInlineBuffer points to the in-place input and output buffer. 02371 * @return none. 02372 */ 02373 02374 void arm_dct4_q15( 02375 const arm_dct4_instance_q15 * S, 02376 q15_t * pState, 02377 q15_t * pInlineBuffer); 02378 02379 /** 02380 * @brief Floating-point vector addition. 02381 * @param[in] *pSrcA points to the first input vector 02382 * @param[in] *pSrcB points to the second input vector 02383 * @param[out] *pDst points to the output vector 02384 * @param[in] blockSize number of samples in each vector 02385 * @return none. 02386 */ 02387 02388 void arm_add_f32( 02389 float32_t * pSrcA, 02390 float32_t * pSrcB, 02391 float32_t * pDst, 02392 uint32_t blockSize); 02393 02394 /** 02395 * @brief Q7 vector addition. 02396 * @param[in] *pSrcA points to the first input vector 02397 * @param[in] *pSrcB points to the second input vector 02398 * @param[out] *pDst points to the output vector 02399 * @param[in] blockSize number of samples in each vector 02400 * @return none. 02401 */ 02402 02403 void arm_add_q7( 02404 q7_t * pSrcA, 02405 q7_t * pSrcB, 02406 q7_t * pDst, 02407 uint32_t blockSize); 02408 02409 /** 02410 * @brief Q15 vector addition. 02411 * @param[in] *pSrcA points to the first input vector 02412 * @param[in] *pSrcB points to the second input vector 02413 * @param[out] *pDst points to the output vector 02414 * @param[in] blockSize number of samples in each vector 02415 * @return none. 02416 */ 02417 02418 void arm_add_q15( 02419 q15_t * pSrcA, 02420 q15_t * pSrcB, 02421 q15_t * pDst, 02422 uint32_t blockSize); 02423 02424 /** 02425 * @brief Q31 vector addition. 02426 * @param[in] *pSrcA points to the first input vector 02427 * @param[in] *pSrcB points to the second input vector 02428 * @param[out] *pDst points to the output vector 02429 * @param[in] blockSize number of samples in each vector 02430 * @return none. 02431 */ 02432 02433 void arm_add_q31( 02434 q31_t * pSrcA, 02435 q31_t * pSrcB, 02436 q31_t * pDst, 02437 uint32_t blockSize); 02438 02439 /** 02440 * @brief Floating-point vector subtraction. 02441 * @param[in] *pSrcA points to the first input vector 02442 * @param[in] *pSrcB points to the second input vector 02443 * @param[out] *pDst points to the output vector 02444 * @param[in] blockSize number of samples in each vector 02445 * @return none. 02446 */ 02447 02448 void arm_sub_f32( 02449 float32_t * pSrcA, 02450 float32_t * pSrcB, 02451 float32_t * pDst, 02452 uint32_t blockSize); 02453 02454 /** 02455 * @brief Q7 vector subtraction. 02456 * @param[in] *pSrcA points to the first input vector 02457 * @param[in] *pSrcB points to the second input vector 02458 * @param[out] *pDst points to the output vector 02459 * @param[in] blockSize number of samples in each vector 02460 * @return none. 02461 */ 02462 02463 void arm_sub_q7( 02464 q7_t * pSrcA, 02465 q7_t * pSrcB, 02466 q7_t * pDst, 02467 uint32_t blockSize); 02468 02469 /** 02470 * @brief Q15 vector subtraction. 02471 * @param[in] *pSrcA points to the first input vector 02472 * @param[in] *pSrcB points to the second input vector 02473 * @param[out] *pDst points to the output vector 02474 * @param[in] blockSize number of samples in each vector 02475 * @return none. 02476 */ 02477 02478 void arm_sub_q15( 02479 q15_t * pSrcA, 02480 q15_t * pSrcB, 02481 q15_t * pDst, 02482 uint32_t blockSize); 02483 02484 /** 02485 * @brief Q31 vector subtraction. 02486 * @param[in] *pSrcA points to the first input vector 02487 * @param[in] *pSrcB points to the second input vector 02488 * @param[out] *pDst points to the output vector 02489 * @param[in] blockSize number of samples in each vector 02490 * @return none. 02491 */ 02492 02493 void arm_sub_q31( 02494 q31_t * pSrcA, 02495 q31_t * pSrcB, 02496 q31_t * pDst, 02497 uint32_t blockSize); 02498 02499 /** 02500 * @brief Multiplies a floating-point vector by a scalar. 02501 * @param[in] *pSrc points to the input vector 02502 * @param[in] scale scale factor to be applied 02503 * @param[out] *pDst points to the output vector 02504 * @param[in] blockSize number of samples in the vector 02505 * @return none. 02506 */ 02507 02508 void arm_scale_f32( 02509 float32_t * pSrc, 02510 float32_t scale, 02511 float32_t * pDst, 02512 uint32_t blockSize); 02513 02514 /** 02515 * @brief Multiplies a Q7 vector by a scalar. 02516 * @param[in] *pSrc points to the input vector 02517 * @param[in] scaleFract fractional portion of the scale value 02518 * @param[in] shift number of bits to shift the result by 02519 * @param[out] *pDst points to the output vector 02520 * @param[in] blockSize number of samples in the vector 02521 * @return none. 02522 */ 02523 02524 void arm_scale_q7( 02525 q7_t * pSrc, 02526 q7_t scaleFract, 02527 int8_t shift, 02528 q7_t * pDst, 02529 uint32_t blockSize); 02530 02531 /** 02532 * @brief Multiplies a Q15 vector by a scalar. 02533 * @param[in] *pSrc points to the input vector 02534 * @param[in] scaleFract fractional portion of the scale value 02535 * @param[in] shift number of bits to shift the result by 02536 * @param[out] *pDst points to the output vector 02537 * @param[in] blockSize number of samples in the vector 02538 * @return none. 02539 */ 02540 02541 void arm_scale_q15( 02542 q15_t * pSrc, 02543 q15_t scaleFract, 02544 int8_t shift, 02545 q15_t * pDst, 02546 uint32_t blockSize); 02547 02548 /** 02549 * @brief Multiplies a Q31 vector by a scalar. 02550 * @param[in] *pSrc points to the input vector 02551 * @param[in] scaleFract fractional portion of the scale value 02552 * @param[in] shift number of bits to shift the result by 02553 * @param[out] *pDst points to the output vector 02554 * @param[in] blockSize number of samples in the vector 02555 * @return none. 02556 */ 02557 02558 void arm_scale_q31( 02559 q31_t * pSrc, 02560 q31_t scaleFract, 02561 int8_t shift, 02562 q31_t * pDst, 02563 uint32_t blockSize); 02564 02565 /** 02566 * @brief Q7 vector absolute value. 02567 * @param[in] *pSrc points to the input buffer 02568 * @param[out] *pDst points to the output buffer 02569 * @param[in] blockSize number of samples in each vector 02570 * @return none. 02571 */ 02572 02573 void arm_abs_q7( 02574 q7_t * pSrc, 02575 q7_t * pDst, 02576 uint32_t blockSize); 02577 02578 /** 02579 * @brief Floating-point vector absolute value. 02580 * @param[in] *pSrc points to the input buffer 02581 * @param[out] *pDst points to the output buffer 02582 * @param[in] blockSize number of samples in each vector 02583 * @return none. 02584 */ 02585 02586 void arm_abs_f32( 02587 float32_t * pSrc, 02588 float32_t * pDst, 02589 uint32_t blockSize); 02590 02591 /** 02592 * @brief Q15 vector absolute value. 02593 * @param[in] *pSrc points to the input buffer 02594 * @param[out] *pDst points to the output buffer 02595 * @param[in] blockSize number of samples in each vector 02596 * @return none. 02597 */ 02598 02599 void arm_abs_q15( 02600 q15_t * pSrc, 02601 q15_t * pDst, 02602 uint32_t blockSize); 02603 02604 /** 02605 * @brief Q31 vector absolute value. 02606 * @param[in] *pSrc points to the input buffer 02607 * @param[out] *pDst points to the output buffer 02608 * @param[in] blockSize number of samples in each vector 02609 * @return none. 02610 */ 02611 02612 void arm_abs_q31( 02613 q31_t * pSrc, 02614 q31_t * pDst, 02615 uint32_t blockSize); 02616 02617 /** 02618 * @brief Dot product of floating-point vectors. 02619 * @param[in] *pSrcA points to the first input vector 02620 * @param[in] *pSrcB points to the second input vector 02621 * @param[in] blockSize number of samples in each vector 02622 * @param[out] *result output result returned here 02623 * @return none. 02624 */ 02625 02626 void arm_dot_prod_f32( 02627 float32_t * pSrcA, 02628 float32_t * pSrcB, 02629 uint32_t blockSize, 02630 float32_t * result); 02631 02632 /** 02633 * @brief Dot product of Q7 vectors. 02634 * @param[in] *pSrcA points to the first input vector 02635 * @param[in] *pSrcB points to the second input vector 02636 * @param[in] blockSize number of samples in each vector 02637 * @param[out] *result output result returned here 02638 * @return none. 02639 */ 02640 02641 void arm_dot_prod_q7( 02642 q7_t * pSrcA, 02643 q7_t * pSrcB, 02644 uint32_t blockSize, 02645 q31_t * result); 02646 02647 /** 02648 * @brief Dot product of Q15 vectors. 02649 * @param[in] *pSrcA points to the first input vector 02650 * @param[in] *pSrcB points to the second input vector 02651 * @param[in] blockSize number of samples in each vector 02652 * @param[out] *result output result returned here 02653 * @return none. 02654 */ 02655 02656 void arm_dot_prod_q15( 02657 q15_t * pSrcA, 02658 q15_t * pSrcB, 02659 uint32_t blockSize, 02660 q63_t * result); 02661 02662 /** 02663 * @brief Dot product of Q31 vectors. 02664 * @param[in] *pSrcA points to the first input vector 02665 * @param[in] *pSrcB points to the second input vector 02666 * @param[in] blockSize number of samples in each vector 02667 * @param[out] *result output result returned here 02668 * @return none. 02669 */ 02670 02671 void arm_dot_prod_q31( 02672 q31_t * pSrcA, 02673 q31_t * pSrcB, 02674 uint32_t blockSize, 02675 q63_t * result); 02676 02677 /** 02678 * @brief Shifts the elements of a Q7 vector a specified number of bits. 02679 * @param[in] *pSrc points to the input vector 02680 * @param[in] shiftBits number of bits to shift. A positive value shifts left; a negative value shifts right. 02681 * @param[out] *pDst points to the output vector 02682 * @param[in] blockSize number of samples in the vector 02683 * @return none. 02684 */ 02685 02686 void arm_shift_q7( 02687 q7_t * pSrc, 02688 int8_t shiftBits, 02689 q7_t * pDst, 02690 uint32_t blockSize); 02691 02692 /** 02693 * @brief Shifts the elements of a Q15 vector a specified number of bits. 02694 * @param[in] *pSrc points to the input vector 02695 * @param[in] shiftBits number of bits to shift. A positive value shifts left; a negative value shifts right. 02696 * @param[out] *pDst points to the output vector 02697 * @param[in] blockSize number of samples in the vector 02698 * @return none. 02699 */ 02700 02701 void arm_shift_q15( 02702 q15_t * pSrc, 02703 int8_t shiftBits, 02704 q15_t * pDst, 02705 uint32_t blockSize); 02706 02707 /** 02708 * @brief Shifts the elements of a Q31 vector a specified number of bits. 02709 * @param[in] *pSrc points to the input vector 02710 * @param[in] shiftBits number of bits to shift. A positive value shifts left; a negative value shifts right. 02711 * @param[out] *pDst points to the output vector 02712 * @param[in] blockSize number of samples in the vector 02713 * @return none. 02714 */ 02715 02716 void arm_shift_q31( 02717 q31_t * pSrc, 02718 int8_t shiftBits, 02719 q31_t * pDst, 02720 uint32_t blockSize); 02721 02722 /** 02723 * @brief Adds a constant offset to a floating-point vector. 02724 * @param[in] *pSrc points to the input vector 02725 * @param[in] offset is the offset to be added 02726 * @param[out] *pDst points to the output vector 02727 * @param[in] blockSize number of samples in the vector 02728 * @return none. 02729 */ 02730 02731 void arm_offset_f32( 02732 float32_t * pSrc, 02733 float32_t offset, 02734 float32_t * pDst, 02735 uint32_t blockSize); 02736 02737 /** 02738 * @brief Adds a constant offset to a Q7 vector. 02739 * @param[in] *pSrc points to the input vector 02740 * @param[in] offset is the offset to be added 02741 * @param[out] *pDst points to the output vector 02742 * @param[in] blockSize number of samples in the vector 02743 * @return none. 02744 */ 02745 02746 void arm_offset_q7( 02747 q7_t * pSrc, 02748 q7_t offset, 02749 q7_t * pDst, 02750 uint32_t blockSize); 02751 02752 /** 02753 * @brief Adds a constant offset to a Q15 vector. 02754 * @param[in] *pSrc points to the input vector 02755 * @param[in] offset is the offset to be added 02756 * @param[out] *pDst points to the output vector 02757 * @param[in] blockSize number of samples in the vector 02758 * @return none. 02759 */ 02760 02761 void arm_offset_q15( 02762 q15_t * pSrc, 02763 q15_t offset, 02764 q15_t * pDst, 02765 uint32_t blockSize); 02766 02767 /** 02768 * @brief Adds a constant offset to a Q31 vector. 02769 * @param[in] *pSrc points to the input vector 02770 * @param[in] offset is the offset to be added 02771 * @param[out] *pDst points to the output vector 02772 * @param[in] blockSize number of samples in the vector 02773 * @return none. 02774 */ 02775 02776 void arm_offset_q31( 02777 q31_t * pSrc, 02778 q31_t offset, 02779 q31_t * pDst, 02780 uint32_t blockSize); 02781 02782 /** 02783 * @brief Negates the elements of a floating-point vector. 02784 * @param[in] *pSrc points to the input vector 02785 * @param[out] *pDst points to the output vector 02786 * @param[in] blockSize number of samples in the vector 02787 * @return none. 02788 */ 02789 02790 void arm_negate_f32( 02791 float32_t * pSrc, 02792 float32_t * pDst, 02793 uint32_t blockSize); 02794 02795 /** 02796 * @brief Negates the elements of a Q7 vector. 02797 * @param[in] *pSrc points to the input vector 02798 * @param[out] *pDst points to the output vector 02799 * @param[in] blockSize number of samples in the vector 02800 * @return none. 02801 */ 02802 02803 void arm_negate_q7( 02804 q7_t * pSrc, 02805 q7_t * pDst, 02806 uint32_t blockSize); 02807 02808 /** 02809 * @brief Negates the elements of a Q15 vector. 02810 * @param[in] *pSrc points to the input vector 02811 * @param[out] *pDst points to the output vector 02812 * @param[in] blockSize number of samples in the vector 02813 * @return none. 02814 */ 02815 02816 void arm_negate_q15( 02817 q15_t * pSrc, 02818 q15_t * pDst, 02819 uint32_t blockSize); 02820 02821 /** 02822 * @brief Negates the elements of a Q31 vector. 02823 * @param[in] *pSrc points to the input vector 02824 * @param[out] *pDst points to the output vector 02825 * @param[in] blockSize number of samples in the vector 02826 * @return none. 02827 */ 02828 02829 void arm_negate_q31( 02830 q31_t * pSrc, 02831 q31_t * pDst, 02832 uint32_t blockSize); 02833 /** 02834 * @brief Copies the elements of a floating-point vector. 02835 * @param[in] *pSrc input pointer 02836 * @param[out] *pDst output pointer 02837 * @param[in] blockSize number of samples to process 02838 * @return none. 02839 */ 02840 void arm_copy_f32( 02841 float32_t * pSrc, 02842 float32_t * pDst, 02843 uint32_t blockSize); 02844 02845 /** 02846 * @brief Copies the elements of a Q7 vector. 02847 * @param[in] *pSrc input pointer 02848 * @param[out] *pDst output pointer 02849 * @param[in] blockSize number of samples to process 02850 * @return none. 02851 */ 02852 void arm_copy_q7( 02853 q7_t * pSrc, 02854 q7_t * pDst, 02855 uint32_t blockSize); 02856 02857 /** 02858 * @brief Copies the elements of a Q15 vector. 02859 * @param[in] *pSrc input pointer 02860 * @param[out] *pDst output pointer 02861 * @param[in] blockSize number of samples to process 02862 * @return none. 02863 */ 02864 void arm_copy_q15( 02865 q15_t * pSrc, 02866 q15_t * pDst, 02867 uint32_t blockSize); 02868 02869 /** 02870 * @brief Copies the elements of a Q31 vector. 02871 * @param[in] *pSrc input pointer 02872 * @param[out] *pDst output pointer 02873 * @param[in] blockSize number of samples to process 02874 * @return none. 02875 */ 02876 void arm_copy_q31( 02877 q31_t * pSrc, 02878 q31_t * pDst, 02879 uint32_t blockSize); 02880 /** 02881 * @brief Fills a constant value into a floating-point vector. 02882 * @param[in] value input value to be filled 02883 * @param[out] *pDst output pointer 02884 * @param[in] blockSize number of samples to process 02885 * @return none. 02886 */ 02887 void arm_fill_f32( 02888 float32_t value, 02889 float32_t * pDst, 02890 uint32_t blockSize); 02891 02892 /** 02893 * @brief Fills a constant value into a Q7 vector. 02894 * @param[in] value input value to be filled 02895 * @param[out] *pDst output pointer 02896 * @param[in] blockSize number of samples to process 02897 * @return none. 02898 */ 02899 void arm_fill_q7( 02900 q7_t value, 02901 q7_t * pDst, 02902 uint32_t blockSize); 02903 02904 /** 02905 * @brief Fills a constant value into a Q15 vector. 02906 * @param[in] value input value to be filled 02907 * @param[out] *pDst output pointer 02908 * @param[in] blockSize number of samples to process 02909 * @return none. 02910 */ 02911 void arm_fill_q15( 02912 q15_t value, 02913 q15_t * pDst, 02914 uint32_t blockSize); 02915 02916 /** 02917 * @brief Fills a constant value into a Q31 vector. 02918 * @param[in] value input value to be filled 02919 * @param[out] *pDst output pointer 02920 * @param[in] blockSize number of samples to process 02921 * @return none. 02922 */ 02923 void arm_fill_q31( 02924 q31_t value, 02925 q31_t * pDst, 02926 uint32_t blockSize); 02927 02928 /** 02929 * @brief Convolution of floating-point sequences. 02930 * @param[in] *pSrcA points to the first input sequence. 02931 * @param[in] srcALen length of the first input sequence. 02932 * @param[in] *pSrcB points to the second input sequence. 02933 * @param[in] srcBLen length of the second input sequence. 02934 * @param[out] *pDst points to the location where the output result is written. Length srcALen+srcBLen-1. 02935 * @return none. 02936 */ 02937 02938 void arm_conv_f32( 02939 float32_t * pSrcA, 02940 uint32_t srcALen, 02941 float32_t * pSrcB, 02942 uint32_t srcBLen, 02943 float32_t * pDst); 02944 02945 02946 /** 02947 * @brief Convolution of Q15 sequences. 02948 * @param[in] *pSrcA points to the first input sequence. 02949 * @param[in] srcALen length of the first input sequence. 02950 * @param[in] *pSrcB points to the second input sequence. 02951 * @param[in] srcBLen length of the second input sequence. 02952 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 02953 * @param[in] *pScratch1 points to scratch buffer of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 02954 * @param[in] *pScratch2 points to scratch buffer of size min(srcALen, srcBLen). 02955 * @return none. 02956 */ 02957 02958 02959 void arm_conv_opt_q15( 02960 q15_t * pSrcA, 02961 uint32_t srcALen, 02962 q15_t * pSrcB, 02963 uint32_t srcBLen, 02964 q15_t * pDst, 02965 q15_t * pScratch1, 02966 q15_t * pScratch2); 02967 02968 02969 /** 02970 * @brief Convolution of Q15 sequences. 02971 * @param[in] *pSrcA points to the first input sequence. 02972 * @param[in] srcALen length of the first input sequence. 02973 * @param[in] *pSrcB points to the second input sequence. 02974 * @param[in] srcBLen length of the second input sequence. 02975 * @param[out] *pDst points to the location where the output result is written. Length srcALen+srcBLen-1. 02976 * @return none. 02977 */ 02978 02979 void arm_conv_q15( 02980 q15_t * pSrcA, 02981 uint32_t srcALen, 02982 q15_t * pSrcB, 02983 uint32_t srcBLen, 02984 q15_t * pDst); 02985 02986 /** 02987 * @brief Convolution of Q15 sequences (fast version) for Cortex-M3 and Cortex-M4 02988 * @param[in] *pSrcA points to the first input sequence. 02989 * @param[in] srcALen length of the first input sequence. 02990 * @param[in] *pSrcB points to the second input sequence. 02991 * @param[in] srcBLen length of the second input sequence. 02992 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 02993 * @return none. 02994 */ 02995 02996 void arm_conv_fast_q15( 02997 q15_t * pSrcA, 02998 uint32_t srcALen, 02999 q15_t * pSrcB, 03000 uint32_t srcBLen, 03001 q15_t * pDst); 03002 03003 /** 03004 * @brief Convolution of Q15 sequences (fast version) for Cortex-M3 and Cortex-M4 03005 * @param[in] *pSrcA points to the first input sequence. 03006 * @param[in] srcALen length of the first input sequence. 03007 * @param[in] *pSrcB points to the second input sequence. 03008 * @param[in] srcBLen length of the second input sequence. 03009 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 03010 * @param[in] *pScratch1 points to scratch buffer of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 03011 * @param[in] *pScratch2 points to scratch buffer of size min(srcALen, srcBLen). 03012 * @return none. 03013 */ 03014 03015 void arm_conv_fast_opt_q15( 03016 q15_t * pSrcA, 03017 uint32_t srcALen, 03018 q15_t * pSrcB, 03019 uint32_t srcBLen, 03020 q15_t * pDst, 03021 q15_t * pScratch1, 03022 q15_t * pScratch2); 03023 03024 03025 03026 /** 03027 * @brief Convolution of Q31 sequences. 03028 * @param[in] *pSrcA points to the first input sequence. 03029 * @param[in] srcALen length of the first input sequence. 03030 * @param[in] *pSrcB points to the second input sequence. 03031 * @param[in] srcBLen length of the second input sequence. 03032 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 03033 * @return none. 03034 */ 03035 03036 void arm_conv_q31( 03037 q31_t * pSrcA, 03038 uint32_t srcALen, 03039 q31_t * pSrcB, 03040 uint32_t srcBLen, 03041 q31_t * pDst); 03042 03043 /** 03044 * @brief Convolution of Q31 sequences (fast version) for Cortex-M3 and Cortex-M4 03045 * @param[in] *pSrcA points to the first input sequence. 03046 * @param[in] srcALen length of the first input sequence. 03047 * @param[in] *pSrcB points to the second input sequence. 03048 * @param[in] srcBLen length of the second input sequence. 03049 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 03050 * @return none. 03051 */ 03052 03053 void arm_conv_fast_q31( 03054 q31_t * pSrcA, 03055 uint32_t srcALen, 03056 q31_t * pSrcB, 03057 uint32_t srcBLen, 03058 q31_t * pDst); 03059 03060 03061 /** 03062 * @brief Convolution of Q7 sequences. 03063 * @param[in] *pSrcA points to the first input sequence. 03064 * @param[in] srcALen length of the first input sequence. 03065 * @param[in] *pSrcB points to the second input sequence. 03066 * @param[in] srcBLen length of the second input sequence. 03067 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 03068 * @param[in] *pScratch1 points to scratch buffer(of type q15_t) of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 03069 * @param[in] *pScratch2 points to scratch buffer (of type q15_t) of size min(srcALen, srcBLen). 03070 * @return none. 03071 */ 03072 03073 void arm_conv_opt_q7( 03074 q7_t * pSrcA, 03075 uint32_t srcALen, 03076 q7_t * pSrcB, 03077 uint32_t srcBLen, 03078 q7_t * pDst, 03079 q15_t * pScratch1, 03080 q15_t * pScratch2); 03081 03082 03083 03084 /** 03085 * @brief Convolution of Q7 sequences. 03086 * @param[in] *pSrcA points to the first input sequence. 03087 * @param[in] srcALen length of the first input sequence. 03088 * @param[in] *pSrcB points to the second input sequence. 03089 * @param[in] srcBLen length of the second input sequence. 03090 * @param[out] *pDst points to the block of output data Length srcALen+srcBLen-1. 03091 * @return none. 03092 */ 03093 03094 void arm_conv_q7( 03095 q7_t * pSrcA, 03096 uint32_t srcALen, 03097 q7_t * pSrcB, 03098 uint32_t srcBLen, 03099 q7_t * pDst); 03100 03101 03102 /** 03103 * @brief Partial convolution of floating-point sequences. 03104 * @param[in] *pSrcA points to the first input sequence. 03105 * @param[in] srcALen length of the first input sequence. 03106 * @param[in] *pSrcB points to the second input sequence. 03107 * @param[in] srcBLen length of the second input sequence. 03108 * @param[out] *pDst points to the block of output data 03109 * @param[in] firstIndex is the first output sample to start with. 03110 * @param[in] numPoints is the number of output points to be computed. 03111 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03112 */ 03113 03114 arm_status arm_conv_partial_f32( 03115 float32_t * pSrcA, 03116 uint32_t srcALen, 03117 float32_t * pSrcB, 03118 uint32_t srcBLen, 03119 float32_t * pDst, 03120 uint32_t firstIndex, 03121 uint32_t numPoints); 03122 03123 /** 03124 * @brief Partial convolution of Q15 sequences. 03125 * @param[in] *pSrcA points to the first input sequence. 03126 * @param[in] srcALen length of the first input sequence. 03127 * @param[in] *pSrcB points to the second input sequence. 03128 * @param[in] srcBLen length of the second input sequence. 03129 * @param[out] *pDst points to the block of output data 03130 * @param[in] firstIndex is the first output sample to start with. 03131 * @param[in] numPoints is the number of output points to be computed. 03132 * @param[in] * pScratch1 points to scratch buffer of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 03133 * @param[in] * pScratch2 points to scratch buffer of size min(srcALen, srcBLen). 03134 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03135 */ 03136 03137 arm_status arm_conv_partial_opt_q15( 03138 q15_t * pSrcA, 03139 uint32_t srcALen, 03140 q15_t * pSrcB, 03141 uint32_t srcBLen, 03142 q15_t * pDst, 03143 uint32_t firstIndex, 03144 uint32_t numPoints, 03145 q15_t * pScratch1, 03146 q15_t * pScratch2); 03147 03148 03149 /** 03150 * @brief Partial convolution of Q15 sequences. 03151 * @param[in] *pSrcA points to the first input sequence. 03152 * @param[in] srcALen length of the first input sequence. 03153 * @param[in] *pSrcB points to the second input sequence. 03154 * @param[in] srcBLen length of the second input sequence. 03155 * @param[out] *pDst points to the block of output data 03156 * @param[in] firstIndex is the first output sample to start with. 03157 * @param[in] numPoints is the number of output points to be computed. 03158 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03159 */ 03160 03161 arm_status arm_conv_partial_q15( 03162 q15_t * pSrcA, 03163 uint32_t srcALen, 03164 q15_t * pSrcB, 03165 uint32_t srcBLen, 03166 q15_t * pDst, 03167 uint32_t firstIndex, 03168 uint32_t numPoints); 03169 03170 /** 03171 * @brief Partial convolution of Q15 sequences (fast version) for Cortex-M3 and Cortex-M4 03172 * @param[in] *pSrcA points to the first input sequence. 03173 * @param[in] srcALen length of the first input sequence. 03174 * @param[in] *pSrcB points to the second input sequence. 03175 * @param[in] srcBLen length of the second input sequence. 03176 * @param[out] *pDst points to the block of output data 03177 * @param[in] firstIndex is the first output sample to start with. 03178 * @param[in] numPoints is the number of output points to be computed. 03179 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03180 */ 03181 03182 arm_status arm_conv_partial_fast_q15( 03183 q15_t * pSrcA, 03184 uint32_t srcALen, 03185 q15_t * pSrcB, 03186 uint32_t srcBLen, 03187 q15_t * pDst, 03188 uint32_t firstIndex, 03189 uint32_t numPoints); 03190 03191 03192 /** 03193 * @brief Partial convolution of Q15 sequences (fast version) for Cortex-M3 and Cortex-M4 03194 * @param[in] *pSrcA points to the first input sequence. 03195 * @param[in] srcALen length of the first input sequence. 03196 * @param[in] *pSrcB points to the second input sequence. 03197 * @param[in] srcBLen length of the second input sequence. 03198 * @param[out] *pDst points to the block of output data 03199 * @param[in] firstIndex is the first output sample to start with. 03200 * @param[in] numPoints is the number of output points to be computed. 03201 * @param[in] * pScratch1 points to scratch buffer of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 03202 * @param[in] * pScratch2 points to scratch buffer of size min(srcALen, srcBLen). 03203 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03204 */ 03205 03206 arm_status arm_conv_partial_fast_opt_q15( 03207 q15_t * pSrcA, 03208 uint32_t srcALen, 03209 q15_t * pSrcB, 03210 uint32_t srcBLen, 03211 q15_t * pDst, 03212 uint32_t firstIndex, 03213 uint32_t numPoints, 03214 q15_t * pScratch1, 03215 q15_t * pScratch2); 03216 03217 03218 /** 03219 * @brief Partial convolution of Q31 sequences. 03220 * @param[in] *pSrcA points to the first input sequence. 03221 * @param[in] srcALen length of the first input sequence. 03222 * @param[in] *pSrcB points to the second input sequence. 03223 * @param[in] srcBLen length of the second input sequence. 03224 * @param[out] *pDst points to the block of output data 03225 * @param[in] firstIndex is the first output sample to start with. 03226 * @param[in] numPoints is the number of output points to be computed. 03227 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03228 */ 03229 03230 arm_status arm_conv_partial_q31( 03231 q31_t * pSrcA, 03232 uint32_t srcALen, 03233 q31_t * pSrcB, 03234 uint32_t srcBLen, 03235 q31_t * pDst, 03236 uint32_t firstIndex, 03237 uint32_t numPoints); 03238 03239 03240 /** 03241 * @brief Partial convolution of Q31 sequences (fast version) for Cortex-M3 and Cortex-M4 03242 * @param[in] *pSrcA points to the first input sequence. 03243 * @param[in] srcALen length of the first input sequence. 03244 * @param[in] *pSrcB points to the second input sequence. 03245 * @param[in] srcBLen length of the second input sequence. 03246 * @param[out] *pDst points to the block of output data 03247 * @param[in] firstIndex is the first output sample to start with. 03248 * @param[in] numPoints is the number of output points to be computed. 03249 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03250 */ 03251 03252 arm_status arm_conv_partial_fast_q31( 03253 q31_t * pSrcA, 03254 uint32_t srcALen, 03255 q31_t * pSrcB, 03256 uint32_t srcBLen, 03257 q31_t * pDst, 03258 uint32_t firstIndex, 03259 uint32_t numPoints); 03260 03261 03262 /** 03263 * @brief Partial convolution of Q7 sequences 03264 * @param[in] *pSrcA points to the first input sequence. 03265 * @param[in] srcALen length of the first input sequence. 03266 * @param[in] *pSrcB points to the second input sequence. 03267 * @param[in] srcBLen length of the second input sequence. 03268 * @param[out] *pDst points to the block of output data 03269 * @param[in] firstIndex is the first output sample to start with. 03270 * @param[in] numPoints is the number of output points to be computed. 03271 * @param[in] *pScratch1 points to scratch buffer(of type q15_t) of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 03272 * @param[in] *pScratch2 points to scratch buffer (of type q15_t) of size min(srcALen, srcBLen). 03273 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03274 */ 03275 03276 arm_status arm_conv_partial_opt_q7( 03277 q7_t * pSrcA, 03278 uint32_t srcALen, 03279 q7_t * pSrcB, 03280 uint32_t srcBLen, 03281 q7_t * pDst, 03282 uint32_t firstIndex, 03283 uint32_t numPoints, 03284 q15_t * pScratch1, 03285 q15_t * pScratch2); 03286 03287 03288 /** 03289 * @brief Partial convolution of Q7 sequences. 03290 * @param[in] *pSrcA points to the first input sequence. 03291 * @param[in] srcALen length of the first input sequence. 03292 * @param[in] *pSrcB points to the second input sequence. 03293 * @param[in] srcBLen length of the second input sequence. 03294 * @param[out] *pDst points to the block of output data 03295 * @param[in] firstIndex is the first output sample to start with. 03296 * @param[in] numPoints is the number of output points to be computed. 03297 * @return Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2]. 03298 */ 03299 03300 arm_status arm_conv_partial_q7( 03301 q7_t * pSrcA, 03302 uint32_t srcALen, 03303 q7_t * pSrcB, 03304 uint32_t srcBLen, 03305 q7_t * pDst, 03306 uint32_t firstIndex, 03307 uint32_t numPoints); 03308 03309 03310 03311 /** 03312 * @brief Instance structure for the Q15 FIR decimator. 03313 */ 03314 03315 typedef struct 03316 { 03317 uint8_t M; /**< decimation factor. */ 03318 uint16_t numTaps; /**< number of coefficients in the filter. */ 03319 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 03320 q15_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 03321 } arm_fir_decimate_instance_q15; 03322 03323 /** 03324 * @brief Instance structure for the Q31 FIR decimator. 03325 */ 03326 03327 typedef struct 03328 { 03329 uint8_t M; /**< decimation factor. */ 03330 uint16_t numTaps; /**< number of coefficients in the filter. */ 03331 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 03332 q31_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 03333 03334 } arm_fir_decimate_instance_q31; 03335 03336 /** 03337 * @brief Instance structure for the floating-point FIR decimator. 03338 */ 03339 03340 typedef struct 03341 { 03342 uint8_t M; /**< decimation factor. */ 03343 uint16_t numTaps; /**< number of coefficients in the filter. */ 03344 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 03345 float32_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 03346 03347 } arm_fir_decimate_instance_f32; 03348 03349 03350 03351 /** 03352 * @brief Processing function for the floating-point FIR decimator. 03353 * @param[in] *S points to an instance of the floating-point FIR decimator structure. 03354 * @param[in] *pSrc points to the block of input data. 03355 * @param[out] *pDst points to the block of output data 03356 * @param[in] blockSize number of input samples to process per call. 03357 * @return none 03358 */ 03359 03360 void arm_fir_decimate_f32( 03361 const arm_fir_decimate_instance_f32 * S, 03362 float32_t * pSrc, 03363 float32_t * pDst, 03364 uint32_t blockSize); 03365 03366 03367 /** 03368 * @brief Initialization function for the floating-point FIR decimator. 03369 * @param[in,out] *S points to an instance of the floating-point FIR decimator structure. 03370 * @param[in] numTaps number of coefficients in the filter. 03371 * @param[in] M decimation factor. 03372 * @param[in] *pCoeffs points to the filter coefficients. 03373 * @param[in] *pState points to the state buffer. 03374 * @param[in] blockSize number of input samples to process per call. 03375 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_LENGTH_ERROR if 03376 * <code>blockSize</code> is not a multiple of <code>M</code>. 03377 */ 03378 03379 arm_status arm_fir_decimate_init_f32( 03380 arm_fir_decimate_instance_f32 * S, 03381 uint16_t numTaps, 03382 uint8_t M, 03383 float32_t * pCoeffs, 03384 float32_t * pState, 03385 uint32_t blockSize); 03386 03387 /** 03388 * @brief Processing function for the Q15 FIR decimator. 03389 * @param[in] *S points to an instance of the Q15 FIR decimator structure. 03390 * @param[in] *pSrc points to the block of input data. 03391 * @param[out] *pDst points to the block of output data 03392 * @param[in] blockSize number of input samples to process per call. 03393 * @return none 03394 */ 03395 03396 void arm_fir_decimate_q15( 03397 const arm_fir_decimate_instance_q15 * S, 03398 q15_t * pSrc, 03399 q15_t * pDst, 03400 uint32_t blockSize); 03401 03402 /** 03403 * @brief Processing function for the Q15 FIR decimator (fast variant) for Cortex-M3 and Cortex-M4. 03404 * @param[in] *S points to an instance of the Q15 FIR decimator structure. 03405 * @param[in] *pSrc points to the block of input data. 03406 * @param[out] *pDst points to the block of output data 03407 * @param[in] blockSize number of input samples to process per call. 03408 * @return none 03409 */ 03410 03411 void arm_fir_decimate_fast_q15( 03412 const arm_fir_decimate_instance_q15 * S, 03413 q15_t * pSrc, 03414 q15_t * pDst, 03415 uint32_t blockSize); 03416 03417 03418 03419 /** 03420 * @brief Initialization function for the Q15 FIR decimator. 03421 * @param[in,out] *S points to an instance of the Q15 FIR decimator structure. 03422 * @param[in] numTaps number of coefficients in the filter. 03423 * @param[in] M decimation factor. 03424 * @param[in] *pCoeffs points to the filter coefficients. 03425 * @param[in] *pState points to the state buffer. 03426 * @param[in] blockSize number of input samples to process per call. 03427 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_LENGTH_ERROR if 03428 * <code>blockSize</code> is not a multiple of <code>M</code>. 03429 */ 03430 03431 arm_status arm_fir_decimate_init_q15( 03432 arm_fir_decimate_instance_q15 * S, 03433 uint16_t numTaps, 03434 uint8_t M, 03435 q15_t * pCoeffs, 03436 q15_t * pState, 03437 uint32_t blockSize); 03438 03439 /** 03440 * @brief Processing function for the Q31 FIR decimator. 03441 * @param[in] *S points to an instance of the Q31 FIR decimator structure. 03442 * @param[in] *pSrc points to the block of input data. 03443 * @param[out] *pDst points to the block of output data 03444 * @param[in] blockSize number of input samples to process per call. 03445 * @return none 03446 */ 03447 03448 void arm_fir_decimate_q31( 03449 const arm_fir_decimate_instance_q31 * S, 03450 q31_t * pSrc, 03451 q31_t * pDst, 03452 uint32_t blockSize); 03453 03454 /** 03455 * @brief Processing function for the Q31 FIR decimator (fast variant) for Cortex-M3 and Cortex-M4. 03456 * @param[in] *S points to an instance of the Q31 FIR decimator structure. 03457 * @param[in] *pSrc points to the block of input data. 03458 * @param[out] *pDst points to the block of output data 03459 * @param[in] blockSize number of input samples to process per call. 03460 * @return none 03461 */ 03462 03463 void arm_fir_decimate_fast_q31( 03464 arm_fir_decimate_instance_q31 * S, 03465 q31_t * pSrc, 03466 q31_t * pDst, 03467 uint32_t blockSize); 03468 03469 03470 /** 03471 * @brief Initialization function for the Q31 FIR decimator. 03472 * @param[in,out] *S points to an instance of the Q31 FIR decimator structure. 03473 * @param[in] numTaps number of coefficients in the filter. 03474 * @param[in] M decimation factor. 03475 * @param[in] *pCoeffs points to the filter coefficients. 03476 * @param[in] *pState points to the state buffer. 03477 * @param[in] blockSize number of input samples to process per call. 03478 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_LENGTH_ERROR if 03479 * <code>blockSize</code> is not a multiple of <code>M</code>. 03480 */ 03481 03482 arm_status arm_fir_decimate_init_q31( 03483 arm_fir_decimate_instance_q31 * S, 03484 uint16_t numTaps, 03485 uint8_t M, 03486 q31_t * pCoeffs, 03487 q31_t * pState, 03488 uint32_t blockSize); 03489 03490 03491 03492 /** 03493 * @brief Instance structure for the Q15 FIR interpolator. 03494 */ 03495 03496 typedef struct 03497 { 03498 uint8_t L; /**< upsample factor. */ 03499 uint16_t phaseLength; /**< length of each polyphase filter component. */ 03500 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length L*phaseLength. */ 03501 q15_t *pState; /**< points to the state variable array. The array is of length blockSize+phaseLength-1. */ 03502 } arm_fir_interpolate_instance_q15; 03503 03504 /** 03505 * @brief Instance structure for the Q31 FIR interpolator. 03506 */ 03507 03508 typedef struct 03509 { 03510 uint8_t L; /**< upsample factor. */ 03511 uint16_t phaseLength; /**< length of each polyphase filter component. */ 03512 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length L*phaseLength. */ 03513 q31_t *pState; /**< points to the state variable array. The array is of length blockSize+phaseLength-1. */ 03514 } arm_fir_interpolate_instance_q31; 03515 03516 /** 03517 * @brief Instance structure for the floating-point FIR interpolator. 03518 */ 03519 03520 typedef struct 03521 { 03522 uint8_t L; /**< upsample factor. */ 03523 uint16_t phaseLength; /**< length of each polyphase filter component. */ 03524 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length L*phaseLength. */ 03525 float32_t *pState; /**< points to the state variable array. The array is of length phaseLength+numTaps-1. */ 03526 } arm_fir_interpolate_instance_f32; 03527 03528 03529 /** 03530 * @brief Processing function for the Q15 FIR interpolator. 03531 * @param[in] *S points to an instance of the Q15 FIR interpolator structure. 03532 * @param[in] *pSrc points to the block of input data. 03533 * @param[out] *pDst points to the block of output data. 03534 * @param[in] blockSize number of input samples to process per call. 03535 * @return none. 03536 */ 03537 03538 void arm_fir_interpolate_q15( 03539 const arm_fir_interpolate_instance_q15 * S, 03540 q15_t * pSrc, 03541 q15_t * pDst, 03542 uint32_t blockSize); 03543 03544 03545 /** 03546 * @brief Initialization function for the Q15 FIR interpolator. 03547 * @param[in,out] *S points to an instance of the Q15 FIR interpolator structure. 03548 * @param[in] L upsample factor. 03549 * @param[in] numTaps number of filter coefficients in the filter. 03550 * @param[in] *pCoeffs points to the filter coefficient buffer. 03551 * @param[in] *pState points to the state buffer. 03552 * @param[in] blockSize number of input samples to process per call. 03553 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_LENGTH_ERROR if 03554 * the filter length <code>numTaps</code> is not a multiple of the interpolation factor <code>L</code>. 03555 */ 03556 03557 arm_status arm_fir_interpolate_init_q15( 03558 arm_fir_interpolate_instance_q15 * S, 03559 uint8_t L, 03560 uint16_t numTaps, 03561 q15_t * pCoeffs, 03562 q15_t * pState, 03563 uint32_t blockSize); 03564 03565 /** 03566 * @brief Processing function for the Q31 FIR interpolator. 03567 * @param[in] *S points to an instance of the Q15 FIR interpolator structure. 03568 * @param[in] *pSrc points to the block of input data. 03569 * @param[out] *pDst points to the block of output data. 03570 * @param[in] blockSize number of input samples to process per call. 03571 * @return none. 03572 */ 03573 03574 void arm_fir_interpolate_q31( 03575 const arm_fir_interpolate_instance_q31 * S, 03576 q31_t * pSrc, 03577 q31_t * pDst, 03578 uint32_t blockSize); 03579 03580 /** 03581 * @brief Initialization function for the Q31 FIR interpolator. 03582 * @param[in,out] *S points to an instance of the Q31 FIR interpolator structure. 03583 * @param[in] L upsample factor. 03584 * @param[in] numTaps number of filter coefficients in the filter. 03585 * @param[in] *pCoeffs points to the filter coefficient buffer. 03586 * @param[in] *pState points to the state buffer. 03587 * @param[in] blockSize number of input samples to process per call. 03588 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_LENGTH_ERROR if 03589 * the filter length <code>numTaps</code> is not a multiple of the interpolation factor <code>L</code>. 03590 */ 03591 03592 arm_status arm_fir_interpolate_init_q31( 03593 arm_fir_interpolate_instance_q31 * S, 03594 uint8_t L, 03595 uint16_t numTaps, 03596 q31_t * pCoeffs, 03597 q31_t * pState, 03598 uint32_t blockSize); 03599 03600 03601 /** 03602 * @brief Processing function for the floating-point FIR interpolator. 03603 * @param[in] *S points to an instance of the floating-point FIR interpolator structure. 03604 * @param[in] *pSrc points to the block of input data. 03605 * @param[out] *pDst points to the block of output data. 03606 * @param[in] blockSize number of input samples to process per call. 03607 * @return none. 03608 */ 03609 03610 void arm_fir_interpolate_f32( 03611 const arm_fir_interpolate_instance_f32 * S, 03612 float32_t * pSrc, 03613 float32_t * pDst, 03614 uint32_t blockSize); 03615 03616 /** 03617 * @brief Initialization function for the floating-point FIR interpolator. 03618 * @param[in,out] *S points to an instance of the floating-point FIR interpolator structure. 03619 * @param[in] L upsample factor. 03620 * @param[in] numTaps number of filter coefficients in the filter. 03621 * @param[in] *pCoeffs points to the filter coefficient buffer. 03622 * @param[in] *pState points to the state buffer. 03623 * @param[in] blockSize number of input samples to process per call. 03624 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_LENGTH_ERROR if 03625 * the filter length <code>numTaps</code> is not a multiple of the interpolation factor <code>L</code>. 03626 */ 03627 03628 arm_status arm_fir_interpolate_init_f32( 03629 arm_fir_interpolate_instance_f32 * S, 03630 uint8_t L, 03631 uint16_t numTaps, 03632 float32_t * pCoeffs, 03633 float32_t * pState, 03634 uint32_t blockSize); 03635 03636 /** 03637 * @brief Instance structure for the high precision Q31 Biquad cascade filter. 03638 */ 03639 03640 typedef struct 03641 { 03642 uint8_t numStages; /**< number of 2nd order stages in the filter. Overall order is 2*numStages. */ 03643 q63_t *pState; /**< points to the array of state coefficients. The array is of length 4*numStages. */ 03644 q31_t *pCoeffs; /**< points to the array of coefficients. The array is of length 5*numStages. */ 03645 uint8_t postShift; /**< additional shift, in bits, applied to each output sample. */ 03646 03647 } arm_biquad_cas_df1_32x64_ins_q31; 03648 03649 03650 /** 03651 * @param[in] *S points to an instance of the high precision Q31 Biquad cascade filter structure. 03652 * @param[in] *pSrc points to the block of input data. 03653 * @param[out] *pDst points to the block of output data 03654 * @param[in] blockSize number of samples to process. 03655 * @return none. 03656 */ 03657 03658 void arm_biquad_cas_df1_32x64_q31 ( 03659 const arm_biquad_cas_df1_32x64_ins_q31 * S, 03660 q31_t * pSrc, 03661 q31_t * pDst, 03662 uint32_t blockSize); 03663 03664 03665 /** 03666 * @param[in,out] *S points to an instance of the high precision Q31 Biquad cascade filter structure. 03667 * @param[in] numStages number of 2nd order stages in the filter. 03668 * @param[in] *pCoeffs points to the filter coefficients. 03669 * @param[in] *pState points to the state buffer. 03670 * @param[in] postShift shift to be applied to the output. Varies according to the coefficients format 03671 * @return none 03672 */ 03673 03674 void arm_biquad_cas_df1_32x64_init_q31 ( 03675 arm_biquad_cas_df1_32x64_ins_q31 * S, 03676 uint8_t numStages, 03677 q31_t * pCoeffs, 03678 q63_t * pState, 03679 uint8_t postShift); 03680 03681 03682 03683 /** 03684 * @brief Instance structure for the floating-point transposed direct form II Biquad cascade filter. 03685 */ 03686 03687 typedef struct 03688 { 03689 uint8_t numStages; /**< number of 2nd order stages in the filter. Overall order is 2*numStages. */ 03690 float32_t *pState; /**< points to the array of state coefficients. The array is of length 2*numStages. */ 03691 float32_t *pCoeffs; /**< points to the array of coefficients. The array is of length 5*numStages. */ 03692 } arm_biquad_cascade_df2T_instance_f32; 03693 03694 03695 /** 03696 * @brief Processing function for the floating-point transposed direct form II Biquad cascade filter. 03697 * @param[in] *S points to an instance of the filter data structure. 03698 * @param[in] *pSrc points to the block of input data. 03699 * @param[out] *pDst points to the block of output data 03700 * @param[in] blockSize number of samples to process. 03701 * @return none. 03702 */ 03703 03704 void arm_biquad_cascade_df2T_f32( 03705 const arm_biquad_cascade_df2T_instance_f32 * S, 03706 float32_t * pSrc, 03707 float32_t * pDst, 03708 uint32_t blockSize); 03709 03710 03711 /** 03712 * @brief Initialization function for the floating-point transposed direct form II Biquad cascade filter. 03713 * @param[in,out] *S points to an instance of the filter data structure. 03714 * @param[in] numStages number of 2nd order stages in the filter. 03715 * @param[in] *pCoeffs points to the filter coefficients. 03716 * @param[in] *pState points to the state buffer. 03717 * @return none 03718 */ 03719 03720 void arm_biquad_cascade_df2T_init_f32( 03721 arm_biquad_cascade_df2T_instance_f32 * S, 03722 uint8_t numStages, 03723 float32_t * pCoeffs, 03724 float32_t * pState); 03725 03726 03727 03728 /** 03729 * @brief Instance structure for the Q15 FIR lattice filter. 03730 */ 03731 03732 typedef struct 03733 { 03734 uint16_t numStages; /**< number of filter stages. */ 03735 q15_t *pState; /**< points to the state variable array. The array is of length numStages. */ 03736 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length numStages. */ 03737 } arm_fir_lattice_instance_q15; 03738 03739 /** 03740 * @brief Instance structure for the Q31 FIR lattice filter. 03741 */ 03742 03743 typedef struct 03744 { 03745 uint16_t numStages; /**< number of filter stages. */ 03746 q31_t *pState; /**< points to the state variable array. The array is of length numStages. */ 03747 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length numStages. */ 03748 } arm_fir_lattice_instance_q31; 03749 03750 /** 03751 * @brief Instance structure for the floating-point FIR lattice filter. 03752 */ 03753 03754 typedef struct 03755 { 03756 uint16_t numStages; /**< number of filter stages. */ 03757 float32_t *pState; /**< points to the state variable array. The array is of length numStages. */ 03758 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length numStages. */ 03759 } arm_fir_lattice_instance_f32; 03760 03761 /** 03762 * @brief Initialization function for the Q15 FIR lattice filter. 03763 * @param[in] *S points to an instance of the Q15 FIR lattice structure. 03764 * @param[in] numStages number of filter stages. 03765 * @param[in] *pCoeffs points to the coefficient buffer. The array is of length numStages. 03766 * @param[in] *pState points to the state buffer. The array is of length numStages. 03767 * @return none. 03768 */ 03769 03770 void arm_fir_lattice_init_q15( 03771 arm_fir_lattice_instance_q15 * S, 03772 uint16_t numStages, 03773 q15_t * pCoeffs, 03774 q15_t * pState); 03775 03776 03777 /** 03778 * @brief Processing function for the Q15 FIR lattice filter. 03779 * @param[in] *S points to an instance of the Q15 FIR lattice structure. 03780 * @param[in] *pSrc points to the block of input data. 03781 * @param[out] *pDst points to the block of output data. 03782 * @param[in] blockSize number of samples to process. 03783 * @return none. 03784 */ 03785 void arm_fir_lattice_q15( 03786 const arm_fir_lattice_instance_q15 * S, 03787 q15_t * pSrc, 03788 q15_t * pDst, 03789 uint32_t blockSize); 03790 03791 /** 03792 * @brief Initialization function for the Q31 FIR lattice filter. 03793 * @param[in] *S points to an instance of the Q31 FIR lattice structure. 03794 * @param[in] numStages number of filter stages. 03795 * @param[in] *pCoeffs points to the coefficient buffer. The array is of length numStages. 03796 * @param[in] *pState points to the state buffer. The array is of length numStages. 03797 * @return none. 03798 */ 03799 03800 void arm_fir_lattice_init_q31( 03801 arm_fir_lattice_instance_q31 * S, 03802 uint16_t numStages, 03803 q31_t * pCoeffs, 03804 q31_t * pState); 03805 03806 03807 /** 03808 * @brief Processing function for the Q31 FIR lattice filter. 03809 * @param[in] *S points to an instance of the Q31 FIR lattice structure. 03810 * @param[in] *pSrc points to the block of input data. 03811 * @param[out] *pDst points to the block of output data 03812 * @param[in] blockSize number of samples to process. 03813 * @return none. 03814 */ 03815 03816 void arm_fir_lattice_q31( 03817 const arm_fir_lattice_instance_q31 * S, 03818 q31_t * pSrc, 03819 q31_t * pDst, 03820 uint32_t blockSize); 03821 03822 /** 03823 * @brief Initialization function for the floating-point FIR lattice filter. 03824 * @param[in] *S points to an instance of the floating-point FIR lattice structure. 03825 * @param[in] numStages number of filter stages. 03826 * @param[in] *pCoeffs points to the coefficient buffer. The array is of length numStages. 03827 * @param[in] *pState points to the state buffer. The array is of length numStages. 03828 * @return none. 03829 */ 03830 03831 void arm_fir_lattice_init_f32( 03832 arm_fir_lattice_instance_f32 * S, 03833 uint16_t numStages, 03834 float32_t * pCoeffs, 03835 float32_t * pState); 03836 03837 /** 03838 * @brief Processing function for the floating-point FIR lattice filter. 03839 * @param[in] *S points to an instance of the floating-point FIR lattice structure. 03840 * @param[in] *pSrc points to the block of input data. 03841 * @param[out] *pDst points to the block of output data 03842 * @param[in] blockSize number of samples to process. 03843 * @return none. 03844 */ 03845 03846 void arm_fir_lattice_f32( 03847 const arm_fir_lattice_instance_f32 * S, 03848 float32_t * pSrc, 03849 float32_t * pDst, 03850 uint32_t blockSize); 03851 03852 /** 03853 * @brief Instance structure for the Q15 IIR lattice filter. 03854 */ 03855 typedef struct 03856 { 03857 uint16_t numStages; /**< number of stages in the filter. */ 03858 q15_t *pState; /**< points to the state variable array. The array is of length numStages+blockSize. */ 03859 q15_t *pkCoeffs; /**< points to the reflection coefficient array. The array is of length numStages. */ 03860 q15_t *pvCoeffs; /**< points to the ladder coefficient array. The array is of length numStages+1. */ 03861 } arm_iir_lattice_instance_q15; 03862 03863 /** 03864 * @brief Instance structure for the Q31 IIR lattice filter. 03865 */ 03866 typedef struct 03867 { 03868 uint16_t numStages; /**< number of stages in the filter. */ 03869 q31_t *pState; /**< points to the state variable array. The array is of length numStages+blockSize. */ 03870 q31_t *pkCoeffs; /**< points to the reflection coefficient array. The array is of length numStages. */ 03871 q31_t *pvCoeffs; /**< points to the ladder coefficient array. The array is of length numStages+1. */ 03872 } arm_iir_lattice_instance_q31; 03873 03874 /** 03875 * @brief Instance structure for the floating-point IIR lattice filter. 03876 */ 03877 typedef struct 03878 { 03879 uint16_t numStages; /**< number of stages in the filter. */ 03880 float32_t *pState; /**< points to the state variable array. The array is of length numStages+blockSize. */ 03881 float32_t *pkCoeffs; /**< points to the reflection coefficient array. The array is of length numStages. */ 03882 float32_t *pvCoeffs; /**< points to the ladder coefficient array. The array is of length numStages+1. */ 03883 } arm_iir_lattice_instance_f32; 03884 03885 /** 03886 * @brief Processing function for the floating-point IIR lattice filter. 03887 * @param[in] *S points to an instance of the floating-point IIR lattice structure. 03888 * @param[in] *pSrc points to the block of input data. 03889 * @param[out] *pDst points to the block of output data. 03890 * @param[in] blockSize number of samples to process. 03891 * @return none. 03892 */ 03893 03894 void arm_iir_lattice_f32( 03895 const arm_iir_lattice_instance_f32 * S, 03896 float32_t * pSrc, 03897 float32_t * pDst, 03898 uint32_t blockSize); 03899 03900 /** 03901 * @brief Initialization function for the floating-point IIR lattice filter. 03902 * @param[in] *S points to an instance of the floating-point IIR lattice structure. 03903 * @param[in] numStages number of stages in the filter. 03904 * @param[in] *pkCoeffs points to the reflection coefficient buffer. The array is of length numStages. 03905 * @param[in] *pvCoeffs points to the ladder coefficient buffer. The array is of length numStages+1. 03906 * @param[in] *pState points to the state buffer. The array is of length numStages+blockSize-1. 03907 * @param[in] blockSize number of samples to process. 03908 * @return none. 03909 */ 03910 03911 void arm_iir_lattice_init_f32( 03912 arm_iir_lattice_instance_f32 * S, 03913 uint16_t numStages, 03914 float32_t * pkCoeffs, 03915 float32_t * pvCoeffs, 03916 float32_t * pState, 03917 uint32_t blockSize); 03918 03919 03920 /** 03921 * @brief Processing function for the Q31 IIR lattice filter. 03922 * @param[in] *S points to an instance of the Q31 IIR lattice structure. 03923 * @param[in] *pSrc points to the block of input data. 03924 * @param[out] *pDst points to the block of output data. 03925 * @param[in] blockSize number of samples to process. 03926 * @return none. 03927 */ 03928 03929 void arm_iir_lattice_q31( 03930 const arm_iir_lattice_instance_q31 * S, 03931 q31_t * pSrc, 03932 q31_t * pDst, 03933 uint32_t blockSize); 03934 03935 03936 /** 03937 * @brief Initialization function for the Q31 IIR lattice filter. 03938 * @param[in] *S points to an instance of the Q31 IIR lattice structure. 03939 * @param[in] numStages number of stages in the filter. 03940 * @param[in] *pkCoeffs points to the reflection coefficient buffer. The array is of length numStages. 03941 * @param[in] *pvCoeffs points to the ladder coefficient buffer. The array is of length numStages+1. 03942 * @param[in] *pState points to the state buffer. The array is of length numStages+blockSize. 03943 * @param[in] blockSize number of samples to process. 03944 * @return none. 03945 */ 03946 03947 void arm_iir_lattice_init_q31( 03948 arm_iir_lattice_instance_q31 * S, 03949 uint16_t numStages, 03950 q31_t * pkCoeffs, 03951 q31_t * pvCoeffs, 03952 q31_t * pState, 03953 uint32_t blockSize); 03954 03955 03956 /** 03957 * @brief Processing function for the Q15 IIR lattice filter. 03958 * @param[in] *S points to an instance of the Q15 IIR lattice structure. 03959 * @param[in] *pSrc points to the block of input data. 03960 * @param[out] *pDst points to the block of output data. 03961 * @param[in] blockSize number of samples to process. 03962 * @return none. 03963 */ 03964 03965 void arm_iir_lattice_q15( 03966 const arm_iir_lattice_instance_q15 * S, 03967 q15_t * pSrc, 03968 q15_t * pDst, 03969 uint32_t blockSize); 03970 03971 03972 /** 03973 * @brief Initialization function for the Q15 IIR lattice filter. 03974 * @param[in] *S points to an instance of the fixed-point Q15 IIR lattice structure. 03975 * @param[in] numStages number of stages in the filter. 03976 * @param[in] *pkCoeffs points to reflection coefficient buffer. The array is of length numStages. 03977 * @param[in] *pvCoeffs points to ladder coefficient buffer. The array is of length numStages+1. 03978 * @param[in] *pState points to state buffer. The array is of length numStages+blockSize. 03979 * @param[in] blockSize number of samples to process per call. 03980 * @return none. 03981 */ 03982 03983 void arm_iir_lattice_init_q15( 03984 arm_iir_lattice_instance_q15 * S, 03985 uint16_t numStages, 03986 q15_t * pkCoeffs, 03987 q15_t * pvCoeffs, 03988 q15_t * pState, 03989 uint32_t blockSize); 03990 03991 /** 03992 * @brief Instance structure for the floating-point LMS filter. 03993 */ 03994 03995 typedef struct 03996 { 03997 uint16_t numTaps; /**< number of coefficients in the filter. */ 03998 float32_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 03999 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 04000 float32_t mu; /**< step size that controls filter coefficient updates. */ 04001 } arm_lms_instance_f32; 04002 04003 /** 04004 * @brief Processing function for floating-point LMS filter. 04005 * @param[in] *S points to an instance of the floating-point LMS filter structure. 04006 * @param[in] *pSrc points to the block of input data. 04007 * @param[in] *pRef points to the block of reference data. 04008 * @param[out] *pOut points to the block of output data. 04009 * @param[out] *pErr points to the block of error data. 04010 * @param[in] blockSize number of samples to process. 04011 * @return none. 04012 */ 04013 04014 void arm_lms_f32( 04015 const arm_lms_instance_f32 * S, 04016 float32_t * pSrc, 04017 float32_t * pRef, 04018 float32_t * pOut, 04019 float32_t * pErr, 04020 uint32_t blockSize); 04021 04022 /** 04023 * @brief Initialization function for floating-point LMS filter. 04024 * @param[in] *S points to an instance of the floating-point LMS filter structure. 04025 * @param[in] numTaps number of filter coefficients. 04026 * @param[in] *pCoeffs points to the coefficient buffer. 04027 * @param[in] *pState points to state buffer. 04028 * @param[in] mu step size that controls filter coefficient updates. 04029 * @param[in] blockSize number of samples to process. 04030 * @return none. 04031 */ 04032 04033 void arm_lms_init_f32( 04034 arm_lms_instance_f32 * S, 04035 uint16_t numTaps, 04036 float32_t * pCoeffs, 04037 float32_t * pState, 04038 float32_t mu, 04039 uint32_t blockSize); 04040 04041 /** 04042 * @brief Instance structure for the Q15 LMS filter. 04043 */ 04044 04045 typedef struct 04046 { 04047 uint16_t numTaps; /**< number of coefficients in the filter. */ 04048 q15_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 04049 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 04050 q15_t mu; /**< step size that controls filter coefficient updates. */ 04051 uint32_t postShift; /**< bit shift applied to coefficients. */ 04052 } arm_lms_instance_q15; 04053 04054 04055 /** 04056 * @brief Initialization function for the Q15 LMS filter. 04057 * @param[in] *S points to an instance of the Q15 LMS filter structure. 04058 * @param[in] numTaps number of filter coefficients. 04059 * @param[in] *pCoeffs points to the coefficient buffer. 04060 * @param[in] *pState points to the state buffer. 04061 * @param[in] mu step size that controls filter coefficient updates. 04062 * @param[in] blockSize number of samples to process. 04063 * @param[in] postShift bit shift applied to coefficients. 04064 * @return none. 04065 */ 04066 04067 void arm_lms_init_q15( 04068 arm_lms_instance_q15 * S, 04069 uint16_t numTaps, 04070 q15_t * pCoeffs, 04071 q15_t * pState, 04072 q15_t mu, 04073 uint32_t blockSize, 04074 uint32_t postShift); 04075 04076 /** 04077 * @brief Processing function for Q15 LMS filter. 04078 * @param[in] *S points to an instance of the Q15 LMS filter structure. 04079 * @param[in] *pSrc points to the block of input data. 04080 * @param[in] *pRef points to the block of reference data. 04081 * @param[out] *pOut points to the block of output data. 04082 * @param[out] *pErr points to the block of error data. 04083 * @param[in] blockSize number of samples to process. 04084 * @return none. 04085 */ 04086 04087 void arm_lms_q15( 04088 const arm_lms_instance_q15 * S, 04089 q15_t * pSrc, 04090 q15_t * pRef, 04091 q15_t * pOut, 04092 q15_t * pErr, 04093 uint32_t blockSize); 04094 04095 04096 /** 04097 * @brief Instance structure for the Q31 LMS filter. 04098 */ 04099 04100 typedef struct 04101 { 04102 uint16_t numTaps; /**< number of coefficients in the filter. */ 04103 q31_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 04104 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 04105 q31_t mu; /**< step size that controls filter coefficient updates. */ 04106 uint32_t postShift; /**< bit shift applied to coefficients. */ 04107 04108 } arm_lms_instance_q31; 04109 04110 /** 04111 * @brief Processing function for Q31 LMS filter. 04112 * @param[in] *S points to an instance of the Q15 LMS filter structure. 04113 * @param[in] *pSrc points to the block of input data. 04114 * @param[in] *pRef points to the block of reference data. 04115 * @param[out] *pOut points to the block of output data. 04116 * @param[out] *pErr points to the block of error data. 04117 * @param[in] blockSize number of samples to process. 04118 * @return none. 04119 */ 04120 04121 void arm_lms_q31( 04122 const arm_lms_instance_q31 * S, 04123 q31_t * pSrc, 04124 q31_t * pRef, 04125 q31_t * pOut, 04126 q31_t * pErr, 04127 uint32_t blockSize); 04128 04129 /** 04130 * @brief Initialization function for Q31 LMS filter. 04131 * @param[in] *S points to an instance of the Q31 LMS filter structure. 04132 * @param[in] numTaps number of filter coefficients. 04133 * @param[in] *pCoeffs points to coefficient buffer. 04134 * @param[in] *pState points to state buffer. 04135 * @param[in] mu step size that controls filter coefficient updates. 04136 * @param[in] blockSize number of samples to process. 04137 * @param[in] postShift bit shift applied to coefficients. 04138 * @return none. 04139 */ 04140 04141 void arm_lms_init_q31( 04142 arm_lms_instance_q31 * S, 04143 uint16_t numTaps, 04144 q31_t * pCoeffs, 04145 q31_t * pState, 04146 q31_t mu, 04147 uint32_t blockSize, 04148 uint32_t postShift); 04149 04150 /** 04151 * @brief Instance structure for the floating-point normalized LMS filter. 04152 */ 04153 04154 typedef struct 04155 { 04156 uint16_t numTaps; /**< number of coefficients in the filter. */ 04157 float32_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 04158 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 04159 float32_t mu; /**< step size that control filter coefficient updates. */ 04160 float32_t energy; /**< saves previous frame energy. */ 04161 float32_t x0; /**< saves previous input sample. */ 04162 } arm_lms_norm_instance_f32; 04163 04164 /** 04165 * @brief Processing function for floating-point normalized LMS filter. 04166 * @param[in] *S points to an instance of the floating-point normalized LMS filter structure. 04167 * @param[in] *pSrc points to the block of input data. 04168 * @param[in] *pRef points to the block of reference data. 04169 * @param[out] *pOut points to the block of output data. 04170 * @param[out] *pErr points to the block of error data. 04171 * @param[in] blockSize number of samples to process. 04172 * @return none. 04173 */ 04174 04175 void arm_lms_norm_f32( 04176 arm_lms_norm_instance_f32 * S, 04177 float32_t * pSrc, 04178 float32_t * pRef, 04179 float32_t * pOut, 04180 float32_t * pErr, 04181 uint32_t blockSize); 04182 04183 /** 04184 * @brief Initialization function for floating-point normalized LMS filter. 04185 * @param[in] *S points to an instance of the floating-point LMS filter structure. 04186 * @param[in] numTaps number of filter coefficients. 04187 * @param[in] *pCoeffs points to coefficient buffer. 04188 * @param[in] *pState points to state buffer. 04189 * @param[in] mu step size that controls filter coefficient updates. 04190 * @param[in] blockSize number of samples to process. 04191 * @return none. 04192 */ 04193 04194 void arm_lms_norm_init_f32( 04195 arm_lms_norm_instance_f32 * S, 04196 uint16_t numTaps, 04197 float32_t * pCoeffs, 04198 float32_t * pState, 04199 float32_t mu, 04200 uint32_t blockSize); 04201 04202 04203 /** 04204 * @brief Instance structure for the Q31 normalized LMS filter. 04205 */ 04206 typedef struct 04207 { 04208 uint16_t numTaps; /**< number of coefficients in the filter. */ 04209 q31_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 04210 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 04211 q31_t mu; /**< step size that controls filter coefficient updates. */ 04212 uint8_t postShift; /**< bit shift applied to coefficients. */ 04213 q31_t *recipTable; /**< points to the reciprocal initial value table. */ 04214 q31_t energy; /**< saves previous frame energy. */ 04215 q31_t x0; /**< saves previous input sample. */ 04216 } arm_lms_norm_instance_q31; 04217 04218 /** 04219 * @brief Processing function for Q31 normalized LMS filter. 04220 * @param[in] *S points to an instance of the Q31 normalized LMS filter structure. 04221 * @param[in] *pSrc points to the block of input data. 04222 * @param[in] *pRef points to the block of reference data. 04223 * @param[out] *pOut points to the block of output data. 04224 * @param[out] *pErr points to the block of error data. 04225 * @param[in] blockSize number of samples to process. 04226 * @return none. 04227 */ 04228 04229 void arm_lms_norm_q31( 04230 arm_lms_norm_instance_q31 * S, 04231 q31_t * pSrc, 04232 q31_t * pRef, 04233 q31_t * pOut, 04234 q31_t * pErr, 04235 uint32_t blockSize); 04236 04237 /** 04238 * @brief Initialization function for Q31 normalized LMS filter. 04239 * @param[in] *S points to an instance of the Q31 normalized LMS filter structure. 04240 * @param[in] numTaps number of filter coefficients. 04241 * @param[in] *pCoeffs points to coefficient buffer. 04242 * @param[in] *pState points to state buffer. 04243 * @param[in] mu step size that controls filter coefficient updates. 04244 * @param[in] blockSize number of samples to process. 04245 * @param[in] postShift bit shift applied to coefficients. 04246 * @return none. 04247 */ 04248 04249 void arm_lms_norm_init_q31( 04250 arm_lms_norm_instance_q31 * S, 04251 uint16_t numTaps, 04252 q31_t * pCoeffs, 04253 q31_t * pState, 04254 q31_t mu, 04255 uint32_t blockSize, 04256 uint8_t postShift); 04257 04258 /** 04259 * @brief Instance structure for the Q15 normalized LMS filter. 04260 */ 04261 04262 typedef struct 04263 { 04264 uint16_t numTaps; /**< Number of coefficients in the filter. */ 04265 q15_t *pState; /**< points to the state variable array. The array is of length numTaps+blockSize-1. */ 04266 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps. */ 04267 q15_t mu; /**< step size that controls filter coefficient updates. */ 04268 uint8_t postShift; /**< bit shift applied to coefficients. */ 04269 q15_t *recipTable; /**< Points to the reciprocal initial value table. */ 04270 q15_t energy; /**< saves previous frame energy. */ 04271 q15_t x0; /**< saves previous input sample. */ 04272 } arm_lms_norm_instance_q15; 04273 04274 /** 04275 * @brief Processing function for Q15 normalized LMS filter. 04276 * @param[in] *S points to an instance of the Q15 normalized LMS filter structure. 04277 * @param[in] *pSrc points to the block of input data. 04278 * @param[in] *pRef points to the block of reference data. 04279 * @param[out] *pOut points to the block of output data. 04280 * @param[out] *pErr points to the block of error data. 04281 * @param[in] blockSize number of samples to process. 04282 * @return none. 04283 */ 04284 04285 void arm_lms_norm_q15( 04286 arm_lms_norm_instance_q15 * S, 04287 q15_t * pSrc, 04288 q15_t * pRef, 04289 q15_t * pOut, 04290 q15_t * pErr, 04291 uint32_t blockSize); 04292 04293 04294 /** 04295 * @brief Initialization function for Q15 normalized LMS filter. 04296 * @param[in] *S points to an instance of the Q15 normalized LMS filter structure. 04297 * @param[in] numTaps number of filter coefficients. 04298 * @param[in] *pCoeffs points to coefficient buffer. 04299 * @param[in] *pState points to state buffer. 04300 * @param[in] mu step size that controls filter coefficient updates. 04301 * @param[in] blockSize number of samples to process. 04302 * @param[in] postShift bit shift applied to coefficients. 04303 * @return none. 04304 */ 04305 04306 void arm_lms_norm_init_q15( 04307 arm_lms_norm_instance_q15 * S, 04308 uint16_t numTaps, 04309 q15_t * pCoeffs, 04310 q15_t * pState, 04311 q15_t mu, 04312 uint32_t blockSize, 04313 uint8_t postShift); 04314 04315 /** 04316 * @brief Correlation of floating-point sequences. 04317 * @param[in] *pSrcA points to the first input sequence. 04318 * @param[in] srcALen length of the first input sequence. 04319 * @param[in] *pSrcB points to the second input sequence. 04320 * @param[in] srcBLen length of the second input sequence. 04321 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04322 * @return none. 04323 */ 04324 04325 void arm_correlate_f32( 04326 float32_t * pSrcA, 04327 uint32_t srcALen, 04328 float32_t * pSrcB, 04329 uint32_t srcBLen, 04330 float32_t * pDst); 04331 04332 04333 /** 04334 * @brief Correlation of Q15 sequences 04335 * @param[in] *pSrcA points to the first input sequence. 04336 * @param[in] srcALen length of the first input sequence. 04337 * @param[in] *pSrcB points to the second input sequence. 04338 * @param[in] srcBLen length of the second input sequence. 04339 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04340 * @param[in] *pScratch points to scratch buffer of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 04341 * @return none. 04342 */ 04343 void arm_correlate_opt_q15( 04344 q15_t * pSrcA, 04345 uint32_t srcALen, 04346 q15_t * pSrcB, 04347 uint32_t srcBLen, 04348 q15_t * pDst, 04349 q15_t * pScratch); 04350 04351 04352 /** 04353 * @brief Correlation of Q15 sequences. 04354 * @param[in] *pSrcA points to the first input sequence. 04355 * @param[in] srcALen length of the first input sequence. 04356 * @param[in] *pSrcB points to the second input sequence. 04357 * @param[in] srcBLen length of the second input sequence. 04358 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04359 * @return none. 04360 */ 04361 04362 void arm_correlate_q15( 04363 q15_t * pSrcA, 04364 uint32_t srcALen, 04365 q15_t * pSrcB, 04366 uint32_t srcBLen, 04367 q15_t * pDst); 04368 04369 /** 04370 * @brief Correlation of Q15 sequences (fast version) for Cortex-M3 and Cortex-M4. 04371 * @param[in] *pSrcA points to the first input sequence. 04372 * @param[in] srcALen length of the first input sequence. 04373 * @param[in] *pSrcB points to the second input sequence. 04374 * @param[in] srcBLen length of the second input sequence. 04375 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04376 * @return none. 04377 */ 04378 04379 void arm_correlate_fast_q15( 04380 q15_t * pSrcA, 04381 uint32_t srcALen, 04382 q15_t * pSrcB, 04383 uint32_t srcBLen, 04384 q15_t * pDst); 04385 04386 04387 04388 /** 04389 * @brief Correlation of Q15 sequences (fast version) for Cortex-M3 and Cortex-M4. 04390 * @param[in] *pSrcA points to the first input sequence. 04391 * @param[in] srcALen length of the first input sequence. 04392 * @param[in] *pSrcB points to the second input sequence. 04393 * @param[in] srcBLen length of the second input sequence. 04394 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04395 * @param[in] *pScratch points to scratch buffer of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 04396 * @return none. 04397 */ 04398 04399 void arm_correlate_fast_opt_q15( 04400 q15_t * pSrcA, 04401 uint32_t srcALen, 04402 q15_t * pSrcB, 04403 uint32_t srcBLen, 04404 q15_t * pDst, 04405 q15_t * pScratch); 04406 04407 /** 04408 * @brief Correlation of Q31 sequences. 04409 * @param[in] *pSrcA points to the first input sequence. 04410 * @param[in] srcALen length of the first input sequence. 04411 * @param[in] *pSrcB points to the second input sequence. 04412 * @param[in] srcBLen length of the second input sequence. 04413 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04414 * @return none. 04415 */ 04416 04417 void arm_correlate_q31( 04418 q31_t * pSrcA, 04419 uint32_t srcALen, 04420 q31_t * pSrcB, 04421 uint32_t srcBLen, 04422 q31_t * pDst); 04423 04424 /** 04425 * @brief Correlation of Q31 sequences (fast version) for Cortex-M3 and Cortex-M4 04426 * @param[in] *pSrcA points to the first input sequence. 04427 * @param[in] srcALen length of the first input sequence. 04428 * @param[in] *pSrcB points to the second input sequence. 04429 * @param[in] srcBLen length of the second input sequence. 04430 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04431 * @return none. 04432 */ 04433 04434 void arm_correlate_fast_q31( 04435 q31_t * pSrcA, 04436 uint32_t srcALen, 04437 q31_t * pSrcB, 04438 uint32_t srcBLen, 04439 q31_t * pDst); 04440 04441 04442 04443 /** 04444 * @brief Correlation of Q7 sequences. 04445 * @param[in] *pSrcA points to the first input sequence. 04446 * @param[in] srcALen length of the first input sequence. 04447 * @param[in] *pSrcB points to the second input sequence. 04448 * @param[in] srcBLen length of the second input sequence. 04449 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04450 * @param[in] *pScratch1 points to scratch buffer(of type q15_t) of size max(srcALen, srcBLen) + 2*min(srcALen, srcBLen) - 2. 04451 * @param[in] *pScratch2 points to scratch buffer (of type q15_t) of size min(srcALen, srcBLen). 04452 * @return none. 04453 */ 04454 04455 void arm_correlate_opt_q7( 04456 q7_t * pSrcA, 04457 uint32_t srcALen, 04458 q7_t * pSrcB, 04459 uint32_t srcBLen, 04460 q7_t * pDst, 04461 q15_t * pScratch1, 04462 q15_t * pScratch2); 04463 04464 04465 /** 04466 * @brief Correlation of Q7 sequences. 04467 * @param[in] *pSrcA points to the first input sequence. 04468 * @param[in] srcALen length of the first input sequence. 04469 * @param[in] *pSrcB points to the second input sequence. 04470 * @param[in] srcBLen length of the second input sequence. 04471 * @param[out] *pDst points to the block of output data Length 2 * max(srcALen, srcBLen) - 1. 04472 * @return none. 04473 */ 04474 04475 void arm_correlate_q7( 04476 q7_t * pSrcA, 04477 uint32_t srcALen, 04478 q7_t * pSrcB, 04479 uint32_t srcBLen, 04480 q7_t * pDst); 04481 04482 04483 /** 04484 * @brief Instance structure for the floating-point sparse FIR filter. 04485 */ 04486 typedef struct 04487 { 04488 uint16_t numTaps; /**< number of coefficients in the filter. */ 04489 uint16_t stateIndex; /**< state buffer index. Points to the oldest sample in the state buffer. */ 04490 float32_t *pState; /**< points to the state buffer array. The array is of length maxDelay+blockSize-1. */ 04491 float32_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 04492 uint16_t maxDelay; /**< maximum offset specified by the pTapDelay array. */ 04493 int32_t *pTapDelay; /**< points to the array of delay values. The array is of length numTaps. */ 04494 } arm_fir_sparse_instance_f32; 04495 04496 /** 04497 * @brief Instance structure for the Q31 sparse FIR filter. 04498 */ 04499 04500 typedef struct 04501 { 04502 uint16_t numTaps; /**< number of coefficients in the filter. */ 04503 uint16_t stateIndex; /**< state buffer index. Points to the oldest sample in the state buffer. */ 04504 q31_t *pState; /**< points to the state buffer array. The array is of length maxDelay+blockSize-1. */ 04505 q31_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 04506 uint16_t maxDelay; /**< maximum offset specified by the pTapDelay array. */ 04507 int32_t *pTapDelay; /**< points to the array of delay values. The array is of length numTaps. */ 04508 } arm_fir_sparse_instance_q31; 04509 04510 /** 04511 * @brief Instance structure for the Q15 sparse FIR filter. 04512 */ 04513 04514 typedef struct 04515 { 04516 uint16_t numTaps; /**< number of coefficients in the filter. */ 04517 uint16_t stateIndex; /**< state buffer index. Points to the oldest sample in the state buffer. */ 04518 q15_t *pState; /**< points to the state buffer array. The array is of length maxDelay+blockSize-1. */ 04519 q15_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 04520 uint16_t maxDelay; /**< maximum offset specified by the pTapDelay array. */ 04521 int32_t *pTapDelay; /**< points to the array of delay values. The array is of length numTaps. */ 04522 } arm_fir_sparse_instance_q15; 04523 04524 /** 04525 * @brief Instance structure for the Q7 sparse FIR filter. 04526 */ 04527 04528 typedef struct 04529 { 04530 uint16_t numTaps; /**< number of coefficients in the filter. */ 04531 uint16_t stateIndex; /**< state buffer index. Points to the oldest sample in the state buffer. */ 04532 q7_t *pState; /**< points to the state buffer array. The array is of length maxDelay+blockSize-1. */ 04533 q7_t *pCoeffs; /**< points to the coefficient array. The array is of length numTaps.*/ 04534 uint16_t maxDelay; /**< maximum offset specified by the pTapDelay array. */ 04535 int32_t *pTapDelay; /**< points to the array of delay values. The array is of length numTaps. */ 04536 } arm_fir_sparse_instance_q7; 04537 04538 /** 04539 * @brief Processing function for the floating-point sparse FIR filter. 04540 * @param[in] *S points to an instance of the floating-point sparse FIR structure. 04541 * @param[in] *pSrc points to the block of input data. 04542 * @param[out] *pDst points to the block of output data 04543 * @param[in] *pScratchIn points to a temporary buffer of size blockSize. 04544 * @param[in] blockSize number of input samples to process per call. 04545 * @return none. 04546 */ 04547 04548 void arm_fir_sparse_f32( 04549 arm_fir_sparse_instance_f32 * S, 04550 float32_t * pSrc, 04551 float32_t * pDst, 04552 float32_t * pScratchIn, 04553 uint32_t blockSize); 04554 04555 /** 04556 * @brief Initialization function for the floating-point sparse FIR filter. 04557 * @param[in,out] *S points to an instance of the floating-point sparse FIR structure. 04558 * @param[in] numTaps number of nonzero coefficients in the filter. 04559 * @param[in] *pCoeffs points to the array of filter coefficients. 04560 * @param[in] *pState points to the state buffer. 04561 * @param[in] *pTapDelay points to the array of offset times. 04562 * @param[in] maxDelay maximum offset time supported. 04563 * @param[in] blockSize number of samples that will be processed per block. 04564 * @return none 04565 */ 04566 04567 void arm_fir_sparse_init_f32( 04568 arm_fir_sparse_instance_f32 * S, 04569 uint16_t numTaps, 04570 float32_t * pCoeffs, 04571 float32_t * pState, 04572 int32_t * pTapDelay, 04573 uint16_t maxDelay, 04574 uint32_t blockSize); 04575 04576 /** 04577 * @brief Processing function for the Q31 sparse FIR filter. 04578 * @param[in] *S points to an instance of the Q31 sparse FIR structure. 04579 * @param[in] *pSrc points to the block of input data. 04580 * @param[out] *pDst points to the block of output data 04581 * @param[in] *pScratchIn points to a temporary buffer of size blockSize. 04582 * @param[in] blockSize number of input samples to process per call. 04583 * @return none. 04584 */ 04585 04586 void arm_fir_sparse_q31( 04587 arm_fir_sparse_instance_q31 * S, 04588 q31_t * pSrc, 04589 q31_t * pDst, 04590 q31_t * pScratchIn, 04591 uint32_t blockSize); 04592 04593 /** 04594 * @brief Initialization function for the Q31 sparse FIR filter. 04595 * @param[in,out] *S points to an instance of the Q31 sparse FIR structure. 04596 * @param[in] numTaps number of nonzero coefficients in the filter. 04597 * @param[in] *pCoeffs points to the array of filter coefficients. 04598 * @param[in] *pState points to the state buffer. 04599 * @param[in] *pTapDelay points to the array of offset times. 04600 * @param[in] maxDelay maximum offset time supported. 04601 * @param[in] blockSize number of samples that will be processed per block. 04602 * @return none 04603 */ 04604 04605 void arm_fir_sparse_init_q31( 04606 arm_fir_sparse_instance_q31 * S, 04607 uint16_t numTaps, 04608 q31_t * pCoeffs, 04609 q31_t * pState, 04610 int32_t * pTapDelay, 04611 uint16_t maxDelay, 04612 uint32_t blockSize); 04613 04614 /** 04615 * @brief Processing function for the Q15 sparse FIR filter. 04616 * @param[in] *S points to an instance of the Q15 sparse FIR structure. 04617 * @param[in] *pSrc points to the block of input data. 04618 * @param[out] *pDst points to the block of output data 04619 * @param[in] *pScratchIn points to a temporary buffer of size blockSize. 04620 * @param[in] *pScratchOut points to a temporary buffer of size blockSize. 04621 * @param[in] blockSize number of input samples to process per call. 04622 * @return none. 04623 */ 04624 04625 void arm_fir_sparse_q15( 04626 arm_fir_sparse_instance_q15 * S, 04627 q15_t * pSrc, 04628 q15_t * pDst, 04629 q15_t * pScratchIn, 04630 q31_t * pScratchOut, 04631 uint32_t blockSize); 04632 04633 04634 /** 04635 * @brief Initialization function for the Q15 sparse FIR filter. 04636 * @param[in,out] *S points to an instance of the Q15 sparse FIR structure. 04637 * @param[in] numTaps number of nonzero coefficients in the filter. 04638 * @param[in] *pCoeffs points to the array of filter coefficients. 04639 * @param[in] *pState points to the state buffer. 04640 * @param[in] *pTapDelay points to the array of offset times. 04641 * @param[in] maxDelay maximum offset time supported. 04642 * @param[in] blockSize number of samples that will be processed per block. 04643 * @return none 04644 */ 04645 04646 void arm_fir_sparse_init_q15( 04647 arm_fir_sparse_instance_q15 * S, 04648 uint16_t numTaps, 04649 q15_t * pCoeffs, 04650 q15_t * pState, 04651 int32_t * pTapDelay, 04652 uint16_t maxDelay, 04653 uint32_t blockSize); 04654 04655 /** 04656 * @brief Processing function for the Q7 sparse FIR filter. 04657 * @param[in] *S points to an instance of the Q7 sparse FIR structure. 04658 * @param[in] *pSrc points to the block of input data. 04659 * @param[out] *pDst points to the block of output data 04660 * @param[in] *pScratchIn points to a temporary buffer of size blockSize. 04661 * @param[in] *pScratchOut points to a temporary buffer of size blockSize. 04662 * @param[in] blockSize number of input samples to process per call. 04663 * @return none. 04664 */ 04665 04666 void arm_fir_sparse_q7( 04667 arm_fir_sparse_instance_q7 * S, 04668 q7_t * pSrc, 04669 q7_t * pDst, 04670 q7_t * pScratchIn, 04671 q31_t * pScratchOut, 04672 uint32_t blockSize); 04673 04674 /** 04675 * @brief Initialization function for the Q7 sparse FIR filter. 04676 * @param[in,out] *S points to an instance of the Q7 sparse FIR structure. 04677 * @param[in] numTaps number of nonzero coefficients in the filter. 04678 * @param[in] *pCoeffs points to the array of filter coefficients. 04679 * @param[in] *pState points to the state buffer. 04680 * @param[in] *pTapDelay points to the array of offset times. 04681 * @param[in] maxDelay maximum offset time supported. 04682 * @param[in] blockSize number of samples that will be processed per block. 04683 * @return none 04684 */ 04685 04686 void arm_fir_sparse_init_q7( 04687 arm_fir_sparse_instance_q7 * S, 04688 uint16_t numTaps, 04689 q7_t * pCoeffs, 04690 q7_t * pState, 04691 int32_t * pTapDelay, 04692 uint16_t maxDelay, 04693 uint32_t blockSize); 04694 04695 04696 /* 04697 * @brief Floating-point sin_cos function. 04698 * @param[in] theta input value in degrees 04699 * @param[out] *pSinVal points to the processed sine output. 04700 * @param[out] *pCosVal points to the processed cos output. 04701 * @return none. 04702 */ 04703 04704 void arm_sin_cos_f32( 04705 float32_t theta, 04706 float32_t * pSinVal, 04707 float32_t * pCcosVal); 04708 04709 /* 04710 * @brief Q31 sin_cos function. 04711 * @param[in] theta scaled input value in degrees 04712 * @param[out] *pSinVal points to the processed sine output. 04713 * @param[out] *pCosVal points to the processed cosine output. 04714 * @return none. 04715 */ 04716 04717 void arm_sin_cos_q31( 04718 q31_t theta, 04719 q31_t * pSinVal, 04720 q31_t * pCosVal); 04721 04722 04723 /** 04724 * @brief Floating-point complex conjugate. 04725 * @param[in] *pSrc points to the input vector 04726 * @param[out] *pDst points to the output vector 04727 * @param[in] numSamples number of complex samples in each vector 04728 * @return none. 04729 */ 04730 04731 void arm_cmplx_conj_f32( 04732 float32_t * pSrc, 04733 float32_t * pDst, 04734 uint32_t numSamples); 04735 04736 /** 04737 * @brief Q31 complex conjugate. 04738 * @param[in] *pSrc points to the input vector 04739 * @param[out] *pDst points to the output vector 04740 * @param[in] numSamples number of complex samples in each vector 04741 * @return none. 04742 */ 04743 04744 void arm_cmplx_conj_q31( 04745 q31_t * pSrc, 04746 q31_t * pDst, 04747 uint32_t numSamples); 04748 04749 /** 04750 * @brief Q15 complex conjugate. 04751 * @param[in] *pSrc points to the input vector 04752 * @param[out] *pDst points to the output vector 04753 * @param[in] numSamples number of complex samples in each vector 04754 * @return none. 04755 */ 04756 04757 void arm_cmplx_conj_q15( 04758 q15_t * pSrc, 04759 q15_t * pDst, 04760 uint32_t numSamples); 04761 04762 04763 04764 /** 04765 * @brief Floating-point complex magnitude squared 04766 * @param[in] *pSrc points to the complex input vector 04767 * @param[out] *pDst points to the real output vector 04768 * @param[in] numSamples number of complex samples in the input vector 04769 * @return none. 04770 */ 04771 04772 void arm_cmplx_mag_squared_f32( 04773 float32_t * pSrc, 04774 float32_t * pDst, 04775 uint32_t numSamples); 04776 04777 /** 04778 * @brief Q31 complex magnitude squared 04779 * @param[in] *pSrc points to the complex input vector 04780 * @param[out] *pDst points to the real output vector 04781 * @param[in] numSamples number of complex samples in the input vector 04782 * @return none. 04783 */ 04784 04785 void arm_cmplx_mag_squared_q31( 04786 q31_t * pSrc, 04787 q31_t * pDst, 04788 uint32_t numSamples); 04789 04790 /** 04791 * @brief Q15 complex magnitude squared 04792 * @param[in] *pSrc points to the complex input vector 04793 * @param[out] *pDst points to the real output vector 04794 * @param[in] numSamples number of complex samples in the input vector 04795 * @return none. 04796 */ 04797 04798 void arm_cmplx_mag_squared_q15( 04799 q15_t * pSrc, 04800 q15_t * pDst, 04801 uint32_t numSamples); 04802 04803 04804 /** 04805 * @ingroup groupController 04806 */ 04807 04808 /** 04809 * @defgroup PID PID Motor Control 04810 * 04811 * A Proportional Integral Derivative (PID) controller is a generic feedback control 04812 * loop mechanism widely used in industrial control systems. 04813 * A PID controller is the most commonly used type of feedback controller. 04814 * 04815 * This set of functions implements (PID) controllers 04816 * for Q15, Q31, and floating-point data types. The functions operate on a single sample 04817 * of data and each call to the function returns a single processed value. 04818 * <code>S</code> points to an instance of the PID control data structure. <code>in</code> 04819 * is the input sample value. The functions return the output value. 04820 * 04821 * \par Algorithm: 04822 * <pre> 04823 * y[n] = y[n-1] + A0 * x[n] + A1 * x[n-1] + A2 * x[n-2] 04824 * A0 = Kp + Ki + Kd 04825 * A1 = (-Kp ) - (2 * Kd ) 04826 * A2 = Kd </pre> 04827 * 04828 * \par 04829 * where \c Kp is proportional constant, \c Ki is Integral constant and \c Kd is Derivative constant 04830 * 04831 * \par 04832 * \image html PID.gif "Proportional Integral Derivative Controller" 04833 * 04834 * \par 04835 * The PID controller calculates an "error" value as the difference between 04836 * the measured output and the reference input. 04837 * The controller attempts to minimize the error by adjusting the process control inputs. 04838 * The proportional value determines the reaction to the current error, 04839 * the integral value determines the reaction based on the sum of recent errors, 04840 * and the derivative value determines the reaction based on the rate at which the error has been changing. 04841 * 04842 * \par Instance Structure 04843 * The Gains A0, A1, A2 and state variables for a PID controller are stored together in an instance data structure. 04844 * A separate instance structure must be defined for each PID Controller. 04845 * There are separate instance structure declarations for each of the 3 supported data types. 04846 * 04847 * \par Reset Functions 04848 * There is also an associated reset function for each data type which clears the state array. 04849 * 04850 * \par Initialization Functions 04851 * There is also an associated initialization function for each data type. 04852 * The initialization function performs the following operations: 04853 * - Initializes the Gains A0, A1, A2 from Kp,Ki, Kd gains. 04854 * - Zeros out the values in the state buffer. 04855 * 04856 * \par 04857 * Instance structure cannot be placed into a const data section and it is recommended to use the initialization function. 04858 * 04859 * \par Fixed-Point Behavior 04860 * Care must be taken when using the fixed-point versions of the PID Controller functions. 04861 * In particular, the overflow and saturation behavior of the accumulator used in each function must be considered. 04862 * Refer to the function specific documentation below for usage guidelines. 04863 */ 04864 04865 /** 04866 * @addtogroup PID 04867 * @{ 04868 */ 04869 04870 /** 04871 * @brief Process function for the floating-point PID Control. 04872 * @param[in,out] *S is an instance of the floating-point PID Control structure 04873 * @param[in] in input sample to process 04874 * @return out processed output sample. 04875 */ 04876 04877 04878 static __INLINE float32_t arm_pid_f32( 04879 arm_pid_instance_f32 * S, 04880 float32_t in) 04881 { 04882 float32_t out; 04883 04884 /* y[n] = y[n-1] + A0 * x[n] + A1 * x[n-1] + A2 * x[n-2] */ 04885 out = (S->A0 * in) + 04886 (S->A1 * S->state[0]) + (S->A2 * S->state[1]) + (S->state[2]); 04887 04888 /* Update state */ 04889 S->state[1] = S->state[0]; 04890 S->state[0] = in; 04891 S->state[2] = out; 04892 04893 /* return to application */ 04894 return (out); 04895 04896 } 04897 04898 /** 04899 * @brief Process function for the Q31 PID Control. 04900 * @param[in,out] *S points to an instance of the Q31 PID Control structure 04901 * @param[in] in input sample to process 04902 * @return out processed output sample. 04903 * 04904 * <b>Scaling and Overflow Behavior:</b> 04905 * \par 04906 * The function is implemented using an internal 64-bit accumulator. 04907 * The accumulator has a 2.62 format and maintains full precision of the intermediate multiplication results but provides only a single guard bit. 04908 * Thus, if the accumulator result overflows it wraps around rather than clip. 04909 * In order to avoid overflows completely the input signal must be scaled down by 2 bits as there are four additions. 04910 * After all multiply-accumulates are performed, the 2.62 accumulator is truncated to 1.32 format and then saturated to 1.31 format. 04911 */ 04912 04913 static __INLINE q31_t arm_pid_q31( 04914 arm_pid_instance_q31 * S, 04915 q31_t in) 04916 { 04917 q63_t acc; 04918 q31_t out; 04919 04920 /* acc = A0 * x[n] */ 04921 acc = (q63_t) S->A0 * in; 04922 04923 /* acc += A1 * x[n-1] */ 04924 acc += (q63_t) S->A1 * S->state[0]; 04925 04926 /* acc += A2 * x[n-2] */ 04927 acc += (q63_t) S->A2 * S->state[1]; 04928 04929 /* convert output to 1.31 format to add y[n-1] */ 04930 out = (q31_t) (acc >> 31u); 04931 04932 /* out += y[n-1] */ 04933 out += S->state[2]; 04934 04935 /* Update state */ 04936 S->state[1] = S->state[0]; 04937 S->state[0] = in; 04938 S->state[2] = out; 04939 04940 /* return to application */ 04941 return (out); 04942 04943 } 04944 04945 /** 04946 * @brief Process function for the Q15 PID Control. 04947 * @param[in,out] *S points to an instance of the Q15 PID Control structure 04948 * @param[in] in input sample to process 04949 * @return out processed output sample. 04950 * 04951 * <b>Scaling and Overflow Behavior:</b> 04952 * \par 04953 * The function is implemented using a 64-bit internal accumulator. 04954 * Both Gains and state variables are represented in 1.15 format and multiplications yield a 2.30 result. 04955 * The 2.30 intermediate results are accumulated in a 64-bit accumulator in 34.30 format. 04956 * There is no risk of internal overflow with this approach and the full precision of intermediate multiplications is preserved. 04957 * After all additions have been performed, the accumulator is truncated to 34.15 format by discarding low 15 bits. 04958 * Lastly, the accumulator is saturated to yield a result in 1.15 format. 04959 */ 04960 04961 static __INLINE q15_t arm_pid_q15( 04962 arm_pid_instance_q15 * S, 04963 q15_t in) 04964 { 04965 q63_t acc; 04966 q15_t out; 04967 04968 #ifndef ARM_MATH_CM0_FAMILY 04969 __SIMD32_TYPE *vstate; 04970 04971 /* Implementation of PID controller */ 04972 04973 /* acc = A0 * x[n] */ 04974 acc = (q31_t) __SMUAD(S->A0, in); 04975 04976 /* acc += A1 * x[n-1] + A2 * x[n-2] */ 04977 vstate = __SIMD32_CONST(S->state); 04978 acc = __SMLALD(S->A1, (q31_t) *vstate, acc); 04979 04980 #else 04981 /* acc = A0 * x[n] */ 04982 acc = ((q31_t) S->A0) * in; 04983 04984 /* acc += A1 * x[n-1] + A2 * x[n-2] */ 04985 acc += (q31_t) S->A1 * S->state[0]; 04986 acc += (q31_t) S->A2 * S->state[1]; 04987 04988 #endif 04989 04990 /* acc += y[n-1] */ 04991 acc += (q31_t) S->state[2] << 15; 04992 04993 /* saturate the output */ 04994 out = (q15_t) (__SSAT((acc >> 15), 16)); 04995 04996 /* Update state */ 04997 S->state[1] = S->state[0]; 04998 S->state[0] = in; 04999 S->state[2] = out; 05000 05001 /* return to application */ 05002 return (out); 05003 05004 } 05005 05006 /** 05007 * @} end of PID group 05008 */ 05009 05010 05011 /** 05012 * @brief Floating-point matrix inverse. 05013 * @param[in] *src points to the instance of the input floating-point matrix structure. 05014 * @param[out] *dst points to the instance of the output floating-point matrix structure. 05015 * @return The function returns ARM_MATH_SIZE_MISMATCH, if the dimensions do not match. 05016 * If the input matrix is singular (does not have an inverse), then the algorithm terminates and returns error status ARM_MATH_SINGULAR. 05017 */ 05018 05019 arm_status arm_mat_inverse_f32( 05020 const arm_matrix_instance_f32 * src, 05021 arm_matrix_instance_f32 * dst); 05022 05023 05024 05025 /** 05026 * @ingroup groupController 05027 */ 05028 05029 05030 /** 05031 * @defgroup clarke Vector Clarke Transform 05032 * Forward Clarke transform converts the instantaneous stator phases into a two-coordinate time invariant vector. 05033 * Generally the Clarke transform uses three-phase currents <code>Ia, Ib and Ic</code> to calculate currents 05034 * in the two-phase orthogonal stator axis <code>Ialpha</code> and <code>Ibeta</code>. 05035 * When <code>Ialpha</code> is superposed with <code>Ia</code> as shown in the figure below 05036 * \image html clarke.gif Stator current space vector and its components in (a,b). 05037 * and <code>Ia + Ib + Ic = 0</code>, in this condition <code>Ialpha</code> and <code>Ibeta</code> 05038 * can be calculated using only <code>Ia</code> and <code>Ib</code>. 05039 * 05040 * The function operates on a single sample of data and each call to the function returns the processed output. 05041 * The library provides separate functions for Q31 and floating-point data types. 05042 * \par Algorithm 05043 * \image html clarkeFormula.gif 05044 * where <code>Ia</code> and <code>Ib</code> are the instantaneous stator phases and 05045 * <code>pIalpha</code> and <code>pIbeta</code> are the two coordinates of time invariant vector. 05046 * \par Fixed-Point Behavior 05047 * Care must be taken when using the Q31 version of the Clarke transform. 05048 * In particular, the overflow and saturation behavior of the accumulator used must be considered. 05049 * Refer to the function specific documentation below for usage guidelines. 05050 */ 05051 05052 /** 05053 * @addtogroup clarke 05054 * @{ 05055 */ 05056 05057 /** 05058 * 05059 * @brief Floating-point Clarke transform 05060 * @param[in] Ia input three-phase coordinate <code>a</code> 05061 * @param[in] Ib input three-phase coordinate <code>b</code> 05062 * @param[out] *pIalpha points to output two-phase orthogonal vector axis alpha 05063 * @param[out] *pIbeta points to output two-phase orthogonal vector axis beta 05064 * @return none. 05065 */ 05066 05067 static __INLINE void arm_clarke_f32( 05068 float32_t Ia, 05069 float32_t Ib, 05070 float32_t * pIalpha, 05071 float32_t * pIbeta) 05072 { 05073 /* Calculate pIalpha using the equation, pIalpha = Ia */ 05074 *pIalpha = Ia; 05075 05076 /* Calculate pIbeta using the equation, pIbeta = (1/sqrt(3)) * Ia + (2/sqrt(3)) * Ib */ 05077 *pIbeta = 05078 ((float32_t) 0.57735026919 * Ia + (float32_t) 1.15470053838 * Ib); 05079 05080 } 05081 05082 /** 05083 * @brief Clarke transform for Q31 version 05084 * @param[in] Ia input three-phase coordinate <code>a</code> 05085 * @param[in] Ib input three-phase coordinate <code>b</code> 05086 * @param[out] *pIalpha points to output two-phase orthogonal vector axis alpha 05087 * @param[out] *pIbeta points to output two-phase orthogonal vector axis beta 05088 * @return none. 05089 * 05090 * <b>Scaling and Overflow Behavior:</b> 05091 * \par 05092 * The function is implemented using an internal 32-bit accumulator. 05093 * The accumulator maintains 1.31 format by truncating lower 31 bits of the intermediate multiplication in 2.62 format. 05094 * There is saturation on the addition, hence there is no risk of overflow. 05095 */ 05096 05097 static __INLINE void arm_clarke_q31( 05098 q31_t Ia, 05099 q31_t Ib, 05100 q31_t * pIalpha, 05101 q31_t * pIbeta) 05102 { 05103 q31_t product1, product2; /* Temporary variables used to store intermediate results */ 05104 05105 /* Calculating pIalpha from Ia by equation pIalpha = Ia */ 05106 *pIalpha = Ia; 05107 05108 /* Intermediate product is calculated by (1/(sqrt(3)) * Ia) */ 05109 product1 = (q31_t) (((q63_t) Ia * 0x24F34E8B) >> 30); 05110 05111 /* Intermediate product is calculated by (2/sqrt(3) * Ib) */ 05112 product2 = (q31_t) (((q63_t) Ib * 0x49E69D16) >> 30); 05113 05114 /* pIbeta is calculated by adding the intermediate products */ 05115 *pIbeta = __QADD(product1, product2); 05116 } 05117 05118 /** 05119 * @} end of clarke group 05120 */ 05121 05122 /** 05123 * @brief Converts the elements of the Q7 vector to Q31 vector. 05124 * @param[in] *pSrc input pointer 05125 * @param[out] *pDst output pointer 05126 * @param[in] blockSize number of samples to process 05127 * @return none. 05128 */ 05129 void arm_q7_to_q31( 05130 q7_t * pSrc, 05131 q31_t * pDst, 05132 uint32_t blockSize); 05133 05134 05135 05136 05137 /** 05138 * @ingroup groupController 05139 */ 05140 05141 /** 05142 * @defgroup inv_clarke Vector Inverse Clarke Transform 05143 * Inverse Clarke transform converts the two-coordinate time invariant vector into instantaneous stator phases. 05144 * 05145 * The function operates on a single sample of data and each call to the function returns the processed output. 05146 * The library provides separate functions for Q31 and floating-point data types. 05147 * \par Algorithm 05148 * \image html clarkeInvFormula.gif 05149 * where <code>pIa</code> and <code>pIb</code> are the instantaneous stator phases and 05150 * <code>Ialpha</code> and <code>Ibeta</code> are the two coordinates of time invariant vector. 05151 * \par Fixed-Point Behavior 05152 * Care must be taken when using the Q31 version of the Clarke transform. 05153 * In particular, the overflow and saturation behavior of the accumulator used must be considered. 05154 * Refer to the function specific documentation below for usage guidelines. 05155 */ 05156 05157 /** 05158 * @addtogroup inv_clarke 05159 * @{ 05160 */ 05161 05162 /** 05163 * @brief Floating-point Inverse Clarke transform 05164 * @param[in] Ialpha input two-phase orthogonal vector axis alpha 05165 * @param[in] Ibeta input two-phase orthogonal vector axis beta 05166 * @param[out] *pIa points to output three-phase coordinate <code>a</code> 05167 * @param[out] *pIb points to output three-phase coordinate <code>b</code> 05168 * @return none. 05169 */ 05170 05171 05172 static __INLINE void arm_inv_clarke_f32( 05173 float32_t Ialpha, 05174 float32_t Ibeta, 05175 float32_t * pIa, 05176 float32_t * pIb) 05177 { 05178 /* Calculating pIa from Ialpha by equation pIa = Ialpha */ 05179 *pIa = Ialpha; 05180 05181 /* Calculating pIb from Ialpha and Ibeta by equation pIb = -(1/2) * Ialpha + (sqrt(3)/2) * Ibeta */ 05182 *pIb = -0.5 * Ialpha + (float32_t) 0.8660254039 *Ibeta; 05183 05184 } 05185 05186 /** 05187 * @brief Inverse Clarke transform for Q31 version 05188 * @param[in] Ialpha input two-phase orthogonal vector axis alpha 05189 * @param[in] Ibeta input two-phase orthogonal vector axis beta 05190 * @param[out] *pIa points to output three-phase coordinate <code>a</code> 05191 * @param[out] *pIb points to output three-phase coordinate <code>b</code> 05192 * @return none. 05193 * 05194 * <b>Scaling and Overflow Behavior:</b> 05195 * \par 05196 * The function is implemented using an internal 32-bit accumulator. 05197 * The accumulator maintains 1.31 format by truncating lower 31 bits of the intermediate multiplication in 2.62 format. 05198 * There is saturation on the subtraction, hence there is no risk of overflow. 05199 */ 05200 05201 static __INLINE void arm_inv_clarke_q31( 05202 q31_t Ialpha, 05203 q31_t Ibeta, 05204 q31_t * pIa, 05205 q31_t * pIb) 05206 { 05207 q31_t product1, product2; /* Temporary variables used to store intermediate results */ 05208 05209 /* Calculating pIa from Ialpha by equation pIa = Ialpha */ 05210 *pIa = Ialpha; 05211 05212 /* Intermediate product is calculated by (1/(2*sqrt(3)) * Ia) */ 05213 product1 = (q31_t) (((q63_t) (Ialpha) * (0x40000000)) >> 31); 05214 05215 /* Intermediate product is calculated by (1/sqrt(3) * pIb) */ 05216 product2 = (q31_t) (((q63_t) (Ibeta) * (0x6ED9EBA1)) >> 31); 05217 05218 /* pIb is calculated by subtracting the products */ 05219 *pIb = __QSUB(product2, product1); 05220 05221 } 05222 05223 /** 05224 * @} end of inv_clarke group 05225 */ 05226 05227 /** 05228 * @brief Converts the elements of the Q7 vector to Q15 vector. 05229 * @param[in] *pSrc input pointer 05230 * @param[out] *pDst output pointer 05231 * @param[in] blockSize number of samples to process 05232 * @return none. 05233 */ 05234 void arm_q7_to_q15( 05235 q7_t * pSrc, 05236 q15_t * pDst, 05237 uint32_t blockSize); 05238 05239 05240 05241 /** 05242 * @ingroup groupController 05243 */ 05244 05245 /** 05246 * @defgroup park Vector Park Transform 05247 * 05248 * Forward Park transform converts the input two-coordinate vector to flux and torque components. 05249 * The Park transform can be used to realize the transformation of the <code>Ialpha</code> and the <code>Ibeta</code> currents 05250 * from the stationary to the moving reference frame and control the spatial relationship between 05251 * the stator vector current and rotor flux vector. 05252 * If we consider the d axis aligned with the rotor flux, the diagram below shows the 05253 * current vector and the relationship from the two reference frames: 05254 * \image html park.gif "Stator current space vector and its component in (a,b) and in the d,q rotating reference frame" 05255 * 05256 * The function operates on a single sample of data and each call to the function returns the processed output. 05257 * The library provides separate functions for Q31 and floating-point data types. 05258 * \par Algorithm 05259 * \image html parkFormula.gif 05260 * where <code>Ialpha</code> and <code>Ibeta</code> are the stator vector components, 05261 * <code>pId</code> and <code>pIq</code> are rotor vector components and <code>cosVal</code> and <code>sinVal</code> are the 05262 * cosine and sine values of theta (rotor flux position). 05263 * \par Fixed-Point Behavior 05264 * Care must be taken when using the Q31 version of the Park transform. 05265 * In particular, the overflow and saturation behavior of the accumulator used must be considered. 05266 * Refer to the function specific documentation below for usage guidelines. 05267 */ 05268 05269 /** 05270 * @addtogroup park 05271 * @{ 05272 */ 05273 05274 /** 05275 * @brief Floating-point Park transform 05276 * @param[in] Ialpha input two-phase vector coordinate alpha 05277 * @param[in] Ibeta input two-phase vector coordinate beta 05278 * @param[out] *pId points to output rotor reference frame d 05279 * @param[out] *pIq points to output rotor reference frame q 05280 * @param[in] sinVal sine value of rotation angle theta 05281 * @param[in] cosVal cosine value of rotation angle theta 05282 * @return none. 05283 * 05284 * The function implements the forward Park transform. 05285 * 05286 */ 05287 05288 static __INLINE void arm_park_f32( 05289 float32_t Ialpha, 05290 float32_t Ibeta, 05291 float32_t * pId, 05292 float32_t * pIq, 05293 float32_t sinVal, 05294 float32_t cosVal) 05295 { 05296 /* Calculate pId using the equation, pId = Ialpha * cosVal + Ibeta * sinVal */ 05297 *pId = Ialpha * cosVal + Ibeta * sinVal; 05298 05299 /* Calculate pIq using the equation, pIq = - Ialpha * sinVal + Ibeta * cosVal */ 05300 *pIq = -Ialpha * sinVal + Ibeta * cosVal; 05301 05302 } 05303 05304 /** 05305 * @brief Park transform for Q31 version 05306 * @param[in] Ialpha input two-phase vector coordinate alpha 05307 * @param[in] Ibeta input two-phase vector coordinate beta 05308 * @param[out] *pId points to output rotor reference frame d 05309 * @param[out] *pIq points to output rotor reference frame q 05310 * @param[in] sinVal sine value of rotation angle theta 05311 * @param[in] cosVal cosine value of rotation angle theta 05312 * @return none. 05313 * 05314 * <b>Scaling and Overflow Behavior:</b> 05315 * \par 05316 * The function is implemented using an internal 32-bit accumulator. 05317 * The accumulator maintains 1.31 format by truncating lower 31 bits of the intermediate multiplication in 2.62 format. 05318 * There is saturation on the addition and subtraction, hence there is no risk of overflow. 05319 */ 05320 05321 05322 static __INLINE void arm_park_q31( 05323 q31_t Ialpha, 05324 q31_t Ibeta, 05325 q31_t * pId, 05326 q31_t * pIq, 05327 q31_t sinVal, 05328 q31_t cosVal) 05329 { 05330 q31_t product1, product2; /* Temporary variables used to store intermediate results */ 05331 q31_t product3, product4; /* Temporary variables used to store intermediate results */ 05332 05333 /* Intermediate product is calculated by (Ialpha * cosVal) */ 05334 product1 = (q31_t) (((q63_t) (Ialpha) * (cosVal)) >> 31); 05335 05336 /* Intermediate product is calculated by (Ibeta * sinVal) */ 05337 product2 = (q31_t) (((q63_t) (Ibeta) * (sinVal)) >> 31); 05338 05339 05340 /* Intermediate product is calculated by (Ialpha * sinVal) */ 05341 product3 = (q31_t) (((q63_t) (Ialpha) * (sinVal)) >> 31); 05342 05343 /* Intermediate product is calculated by (Ibeta * cosVal) */ 05344 product4 = (q31_t) (((q63_t) (Ibeta) * (cosVal)) >> 31); 05345 05346 /* Calculate pId by adding the two intermediate products 1 and 2 */ 05347 *pId = __QADD(product1, product2); 05348 05349 /* Calculate pIq by subtracting the two intermediate products 3 from 4 */ 05350 *pIq = __QSUB(product4, product3); 05351 } 05352 05353 /** 05354 * @} end of park group 05355 */ 05356 05357 /** 05358 * @brief Converts the elements of the Q7 vector to floating-point vector. 05359 * @param[in] *pSrc is input pointer 05360 * @param[out] *pDst is output pointer 05361 * @param[in] blockSize is the number of samples to process 05362 * @return none. 05363 */ 05364 void arm_q7_to_float( 05365 q7_t * pSrc, 05366 float32_t * pDst, 05367 uint32_t blockSize); 05368 05369 05370 /** 05371 * @ingroup groupController 05372 */ 05373 05374 /** 05375 * @defgroup inv_park Vector Inverse Park transform 05376 * Inverse Park transform converts the input flux and torque components to two-coordinate vector. 05377 * 05378 * The function operates on a single sample of data and each call to the function returns the processed output. 05379 * The library provides separate functions for Q31 and floating-point data types. 05380 * \par Algorithm 05381 * \image html parkInvFormula.gif 05382 * where <code>pIalpha</code> and <code>pIbeta</code> are the stator vector components, 05383 * <code>Id</code> and <code>Iq</code> are rotor vector components and <code>cosVal</code> and <code>sinVal</code> are the 05384 * cosine and sine values of theta (rotor flux position). 05385 * \par Fixed-Point Behavior 05386 * Care must be taken when using the Q31 version of the Park transform. 05387 * In particular, the overflow and saturation behavior of the accumulator used must be considered. 05388 * Refer to the function specific documentation below for usage guidelines. 05389 */ 05390 05391 /** 05392 * @addtogroup inv_park 05393 * @{ 05394 */ 05395 05396 /** 05397 * @brief Floating-point Inverse Park transform 05398 * @param[in] Id input coordinate of rotor reference frame d 05399 * @param[in] Iq input coordinate of rotor reference frame q 05400 * @param[out] *pIalpha points to output two-phase orthogonal vector axis alpha 05401 * @param[out] *pIbeta points to output two-phase orthogonal vector axis beta 05402 * @param[in] sinVal sine value of rotation angle theta 05403 * @param[in] cosVal cosine value of rotation angle theta 05404 * @return none. 05405 */ 05406 05407 static __INLINE void arm_inv_park_f32( 05408 float32_t Id, 05409 float32_t Iq, 05410 float32_t * pIalpha, 05411 float32_t * pIbeta, 05412 float32_t sinVal, 05413 float32_t cosVal) 05414 { 05415 /* Calculate pIalpha using the equation, pIalpha = Id * cosVal - Iq * sinVal */ 05416 *pIalpha = Id * cosVal - Iq * sinVal; 05417 05418 /* Calculate pIbeta using the equation, pIbeta = Id * sinVal + Iq * cosVal */ 05419 *pIbeta = Id * sinVal + Iq * cosVal; 05420 05421 } 05422 05423 05424 /** 05425 * @brief Inverse Park transform for Q31 version 05426 * @param[in] Id input coordinate of rotor reference frame d 05427 * @param[in] Iq input coordinate of rotor reference frame q 05428 * @param[out] *pIalpha points to output two-phase orthogonal vector axis alpha 05429 * @param[out] *pIbeta points to output two-phase orthogonal vector axis beta 05430 * @param[in] sinVal sine value of rotation angle theta 05431 * @param[in] cosVal cosine value of rotation angle theta 05432 * @return none. 05433 * 05434 * <b>Scaling and Overflow Behavior:</b> 05435 * \par 05436 * The function is implemented using an internal 32-bit accumulator. 05437 * The accumulator maintains 1.31 format by truncating lower 31 bits of the intermediate multiplication in 2.62 format. 05438 * There is saturation on the addition, hence there is no risk of overflow. 05439 */ 05440 05441 05442 static __INLINE void arm_inv_park_q31( 05443 q31_t Id, 05444 q31_t Iq, 05445 q31_t * pIalpha, 05446 q31_t * pIbeta, 05447 q31_t sinVal, 05448 q31_t cosVal) 05449 { 05450 q31_t product1, product2; /* Temporary variables used to store intermediate results */ 05451 q31_t product3, product4; /* Temporary variables used to store intermediate results */ 05452 05453 /* Intermediate product is calculated by (Id * cosVal) */ 05454 product1 = (q31_t) (((q63_t) (Id) * (cosVal)) >> 31); 05455 05456 /* Intermediate product is calculated by (Iq * sinVal) */ 05457 product2 = (q31_t) (((q63_t) (Iq) * (sinVal)) >> 31); 05458 05459 05460 /* Intermediate product is calculated by (Id * sinVal) */ 05461 product3 = (q31_t) (((q63_t) (Id) * (sinVal)) >> 31); 05462 05463 /* Intermediate product is calculated by (Iq * cosVal) */ 05464 product4 = (q31_t) (((q63_t) (Iq) * (cosVal)) >> 31); 05465 05466 /* Calculate pIalpha by using the two intermediate products 1 and 2 */ 05467 *pIalpha = __QSUB(product1, product2); 05468 05469 /* Calculate pIbeta by using the two intermediate products 3 and 4 */ 05470 *pIbeta = __QADD(product4, product3); 05471 05472 } 05473 05474 /** 05475 * @} end of Inverse park group 05476 */ 05477 05478 05479 /** 05480 * @brief Converts the elements of the Q31 vector to floating-point vector. 05481 * @param[in] *pSrc is input pointer 05482 * @param[out] *pDst is output pointer 05483 * @param[in] blockSize is the number of samples to process 05484 * @return none. 05485 */ 05486 void arm_q31_to_float( 05487 q31_t * pSrc, 05488 float32_t * pDst, 05489 uint32_t blockSize); 05490 05491 /** 05492 * @ingroup groupInterpolation 05493 */ 05494 05495 /** 05496 * @defgroup LinearInterpolate Linear Interpolation 05497 * 05498 * Linear interpolation is a method of curve fitting using linear polynomials. 05499 * Linear interpolation works by effectively drawing a straight line between two neighboring samples and returning the appropriate point along that line 05500 * 05501 * \par 05502 * \image html LinearInterp.gif "Linear interpolation" 05503 * 05504 * \par 05505 * A Linear Interpolate function calculates an output value(y), for the input(x) 05506 * using linear interpolation of the input values x0, x1( nearest input values) and the output values y0 and y1(nearest output values) 05507 * 05508 * \par Algorithm: 05509 * <pre> 05510 * y = y0 + (x - x0) * ((y1 - y0)/(x1-x0)) 05511 * where x0, x1 are nearest values of input x 05512 * y0, y1 are nearest values to output y 05513 * </pre> 05514 * 05515 * \par 05516 * This set of functions implements Linear interpolation process 05517 * for Q7, Q15, Q31, and floating-point data types. The functions operate on a single 05518 * sample of data and each call to the function returns a single processed value. 05519 * <code>S</code> points to an instance of the Linear Interpolate function data structure. 05520 * <code>x</code> is the input sample value. The functions returns the output value. 05521 * 05522 * \par 05523 * if x is outside of the table boundary, Linear interpolation returns first value of the table 05524 * if x is below input range and returns last value of table if x is above range. 05525 */ 05526 05527 /** 05528 * @addtogroup LinearInterpolate 05529 * @{ 05530 */ 05531 05532 /** 05533 * @brief Process function for the floating-point Linear Interpolation Function. 05534 * @param[in,out] *S is an instance of the floating-point Linear Interpolation structure 05535 * @param[in] x input sample to process 05536 * @return y processed output sample. 05537 * 05538 */ 05539 05540 static __INLINE float32_t arm_linear_interp_f32( 05541 arm_linear_interp_instance_f32 * S, 05542 float32_t x) 05543 { 05544 05545 float32_t y; 05546 float32_t x0, x1; /* Nearest input values */ 05547 float32_t y0, y1; /* Nearest output values */ 05548 float32_t xSpacing = S->xSpacing; /* spacing between input values */ 05549 int32_t i; /* Index variable */ 05550 float32_t *pYData = S->pYData; /* pointer to output table */ 05551 05552 /* Calculation of index */ 05553 i = (int32_t) ((x - S->x1) / xSpacing); 05554 05555 if(i < 0) 05556 { 05557 /* Iniatilize output for below specified range as least output value of table */ 05558 y = pYData[0]; 05559 } 05560 else if((uint32_t)i >= S->nValues) 05561 { 05562 /* Iniatilize output for above specified range as last output value of table */ 05563 y = pYData[S->nValues - 1]; 05564 } 05565 else 05566 { 05567 /* Calculation of nearest input values */ 05568 x0 = S->x1 + i * xSpacing; 05569 x1 = S->x1 + (i + 1) * xSpacing; 05570 05571 /* Read of nearest output values */ 05572 y0 = pYData[i]; 05573 y1 = pYData[i + 1]; 05574 05575 /* Calculation of output */ 05576 y = y0 + (x - x0) * ((y1 - y0) / (x1 - x0)); 05577 05578 } 05579 05580 /* returns output value */ 05581 return (y); 05582 } 05583 05584 /** 05585 * 05586 * @brief Process function for the Q31 Linear Interpolation Function. 05587 * @param[in] *pYData pointer to Q31 Linear Interpolation table 05588 * @param[in] x input sample to process 05589 * @param[in] nValues number of table values 05590 * @return y processed output sample. 05591 * 05592 * \par 05593 * Input sample <code>x</code> is in 12.20 format which contains 12 bits for table index and 20 bits for fractional part. 05594 * This function can support maximum of table size 2^12. 05595 * 05596 */ 05597 05598 05599 static __INLINE q31_t arm_linear_interp_q31( 05600 q31_t * pYData, 05601 q31_t x, 05602 uint32_t nValues) 05603 { 05604 q31_t y; /* output */ 05605 q31_t y0, y1; /* Nearest output values */ 05606 q31_t fract; /* fractional part */ 05607 int32_t index; /* Index to read nearest output values */ 05608 05609 /* Input is in 12.20 format */ 05610 /* 12 bits for the table index */ 05611 /* Index value calculation */ 05612 index = ((x & 0xFFF00000) >> 20); 05613 05614 if(index >= (int32_t)(nValues - 1)) 05615 { 05616 return (pYData[nValues - 1]); 05617 } 05618 else if(index < 0) 05619 { 05620 return (pYData[0]); 05621 } 05622 else 05623 { 05624 05625 /* 20 bits for the fractional part */ 05626 /* shift left by 11 to keep fract in 1.31 format */ 05627 fract = (x & 0x000FFFFF) << 11; 05628 05629 /* Read two nearest output values from the index in 1.31(q31) format */ 05630 y0 = pYData[index]; 05631 y1 = pYData[index + 1u]; 05632 05633 /* Calculation of y0 * (1-fract) and y is in 2.30 format */ 05634 y = ((q31_t) ((q63_t) y0 * (0x7FFFFFFF - fract) >> 32)); 05635 05636 /* Calculation of y0 * (1-fract) + y1 *fract and y is in 2.30 format */ 05637 y += ((q31_t) (((q63_t) y1 * fract) >> 32)); 05638 05639 /* Convert y to 1.31 format */ 05640 return (y << 1u); 05641 05642 } 05643 05644 } 05645 05646 /** 05647 * 05648 * @brief Process function for the Q15 Linear Interpolation Function. 05649 * @param[in] *pYData pointer to Q15 Linear Interpolation table 05650 * @param[in] x input sample to process 05651 * @param[in] nValues number of table values 05652 * @return y processed output sample. 05653 * 05654 * \par 05655 * Input sample <code>x</code> is in 12.20 format which contains 12 bits for table index and 20 bits for fractional part. 05656 * This function can support maximum of table size 2^12. 05657 * 05658 */ 05659 05660 05661 static __INLINE q15_t arm_linear_interp_q15( 05662 q15_t * pYData, 05663 q31_t x, 05664 uint32_t nValues) 05665 { 05666 q63_t y; /* output */ 05667 q15_t y0, y1; /* Nearest output values */ 05668 q31_t fract; /* fractional part */ 05669 int32_t index; /* Index to read nearest output values */ 05670 05671 /* Input is in 12.20 format */ 05672 /* 12 bits for the table index */ 05673 /* Index value calculation */ 05674 index = ((x & 0xFFF00000) >> 20u); 05675 05676 if(index >= (int32_t)(nValues - 1)) 05677 { 05678 return (pYData[nValues - 1]); 05679 } 05680 else if(index < 0) 05681 { 05682 return (pYData[0]); 05683 } 05684 else 05685 { 05686 /* 20 bits for the fractional part */ 05687 /* fract is in 12.20 format */ 05688 fract = (x & 0x000FFFFF); 05689 05690 /* Read two nearest output values from the index */ 05691 y0 = pYData[index]; 05692 y1 = pYData[index + 1u]; 05693 05694 /* Calculation of y0 * (1-fract) and y is in 13.35 format */ 05695 y = ((q63_t) y0 * (0xFFFFF - fract)); 05696 05697 /* Calculation of (y0 * (1-fract) + y1 * fract) and y is in 13.35 format */ 05698 y += ((q63_t) y1 * (fract)); 05699 05700 /* convert y to 1.15 format */ 05701 return (y >> 20); 05702 } 05703 05704 05705 } 05706 05707 /** 05708 * 05709 * @brief Process function for the Q7 Linear Interpolation Function. 05710 * @param[in] *pYData pointer to Q7 Linear Interpolation table 05711 * @param[in] x input sample to process 05712 * @param[in] nValues number of table values 05713 * @return y processed output sample. 05714 * 05715 * \par 05716 * Input sample <code>x</code> is in 12.20 format which contains 12 bits for table index and 20 bits for fractional part. 05717 * This function can support maximum of table size 2^12. 05718 */ 05719 05720 05721 static __INLINE q7_t arm_linear_interp_q7( 05722 q7_t * pYData, 05723 q31_t x, 05724 uint32_t nValues) 05725 { 05726 q31_t y; /* output */ 05727 q7_t y0, y1; /* Nearest output values */ 05728 q31_t fract; /* fractional part */ 05729 uint32_t index; /* Index to read nearest output values */ 05730 05731 /* Input is in 12.20 format */ 05732 /* 12 bits for the table index */ 05733 /* Index value calculation */ 05734 if (x < 0) 05735 { 05736 return (pYData[0]); 05737 } 05738 index = (x >> 20) & 0xfff; 05739 05740 05741 if(index >= (nValues - 1)) 05742 { 05743 return (pYData[nValues - 1]); 05744 } 05745 else 05746 { 05747 05748 /* 20 bits for the fractional part */ 05749 /* fract is in 12.20 format */ 05750 fract = (x & 0x000FFFFF); 05751 05752 /* Read two nearest output values from the index and are in 1.7(q7) format */ 05753 y0 = pYData[index]; 05754 y1 = pYData[index + 1u]; 05755 05756 /* Calculation of y0 * (1-fract ) and y is in 13.27(q27) format */ 05757 y = ((y0 * (0xFFFFF - fract))); 05758 05759 /* Calculation of y1 * fract + y0 * (1-fract) and y is in 13.27(q27) format */ 05760 y += (y1 * fract); 05761 05762 /* convert y to 1.7(q7) format */ 05763 return (y >> 20u); 05764 05765 } 05766 05767 } 05768 /** 05769 * @} end of LinearInterpolate group 05770 */ 05771 05772 /** 05773 * @brief Fast approximation to the trigonometric sine function for floating-point data. 05774 * @param[in] x input value in radians. 05775 * @return sin(x). 05776 */ 05777 05778 float32_t arm_sin_f32( 05779 float32_t x); 05780 05781 /** 05782 * @brief Fast approximation to the trigonometric sine function for Q31 data. 05783 * @param[in] x Scaled input value in radians. 05784 * @return sin(x). 05785 */ 05786 05787 q31_t arm_sin_q31( 05788 q31_t x); 05789 05790 /** 05791 * @brief Fast approximation to the trigonometric sine function for Q15 data. 05792 * @param[in] x Scaled input value in radians. 05793 * @return sin(x). 05794 */ 05795 05796 q15_t arm_sin_q15( 05797 q15_t x); 05798 05799 /** 05800 * @brief Fast approximation to the trigonometric cosine function for floating-point data. 05801 * @param[in] x input value in radians. 05802 * @return cos(x). 05803 */ 05804 05805 float32_t arm_cos_f32( 05806 float32_t x); 05807 05808 /** 05809 * @brief Fast approximation to the trigonometric cosine function for Q31 data. 05810 * @param[in] x Scaled input value in radians. 05811 * @return cos(x). 05812 */ 05813 05814 q31_t arm_cos_q31( 05815 q31_t x); 05816 05817 /** 05818 * @brief Fast approximation to the trigonometric cosine function for Q15 data. 05819 * @param[in] x Scaled input value in radians. 05820 * @return cos(x). 05821 */ 05822 05823 q15_t arm_cos_q15( 05824 q15_t x); 05825 05826 05827 /** 05828 * @ingroup groupFastMath 05829 */ 05830 05831 05832 /** 05833 * @defgroup SQRT Square Root 05834 * 05835 * Computes the square root of a number. 05836 * There are separate functions for Q15, Q31, and floating-point data types. 05837 * The square root function is computed using the Newton-Raphson algorithm. 05838 * This is an iterative algorithm of the form: 05839 * <pre> 05840 * x1 = x0 - f(x0)/f'(x0) 05841 * </pre> 05842 * where <code>x1</code> is the current estimate, 05843 * <code>x0</code> is the previous estimate, and 05844 * <code>f'(x0)</code> is the derivative of <code>f()</code> evaluated at <code>x0</code>. 05845 * For the square root function, the algorithm reduces to: 05846 * <pre> 05847 * x0 = in/2 [initial guess] 05848 * x1 = 1/2 * ( x0 + in / x0) [each iteration] 05849 * </pre> 05850 */ 05851 05852 05853 /** 05854 * @addtogroup SQRT 05855 * @{ 05856 */ 05857 05858 /** 05859 * @brief Floating-point square root function. 05860 * @param[in] in input value. 05861 * @param[out] *pOut square root of input value. 05862 * @return The function returns ARM_MATH_SUCCESS if input value is positive value or ARM_MATH_ARGUMENT_ERROR if 05863 * <code>in</code> is negative value and returns zero output for negative values. 05864 */ 05865 05866 static __INLINE arm_status arm_sqrt_f32( 05867 float32_t in, 05868 float32_t * pOut) 05869 { 05870 if(in > 0) 05871 { 05872 05873 // #if __FPU_USED 05874 #if (__FPU_USED == 1) && defined ( __CC_ARM ) 05875 *pOut = __sqrtf(in); 05876 #else 05877 *pOut = sqrtf(in); 05878 #endif 05879 05880 return (ARM_MATH_SUCCESS); 05881 } 05882 else 05883 { 05884 *pOut = 0.0f; 05885 return (ARM_MATH_ARGUMENT_ERROR); 05886 } 05887 05888 } 05889 05890 05891 /** 05892 * @brief Q31 square root function. 05893 * @param[in] in input value. The range of the input value is [0 +1) or 0x00000000 to 0x7FFFFFFF. 05894 * @param[out] *pOut square root of input value. 05895 * @return The function returns ARM_MATH_SUCCESS if input value is positive value or ARM_MATH_ARGUMENT_ERROR if 05896 * <code>in</code> is negative value and returns zero output for negative values. 05897 */ 05898 arm_status arm_sqrt_q31( 05899 q31_t in, 05900 q31_t * pOut); 05901 05902 /** 05903 * @brief Q15 square root function. 05904 * @param[in] in input value. The range of the input value is [0 +1) or 0x0000 to 0x7FFF. 05905 * @param[out] *pOut square root of input value. 05906 * @return The function returns ARM_MATH_SUCCESS if input value is positive value or ARM_MATH_ARGUMENT_ERROR if 05907 * <code>in</code> is negative value and returns zero output for negative values. 05908 */ 05909 arm_status arm_sqrt_q15( 05910 q15_t in, 05911 q15_t * pOut); 05912 05913 /** 05914 * @} end of SQRT group 05915 */ 05916 05917 05918 05919 05920 05921 05922 /** 05923 * @brief floating-point Circular write function. 05924 */ 05925 05926 static __INLINE void arm_circularWrite_f32( 05927 int32_t * circBuffer, 05928 int32_t L, 05929 uint16_t * writeOffset, 05930 int32_t bufferInc, 05931 const int32_t * src, 05932 int32_t srcInc, 05933 uint32_t blockSize) 05934 { 05935 uint32_t i = 0u; 05936 int32_t wOffset; 05937 05938 /* Copy the value of Index pointer that points 05939 * to the current location where the input samples to be copied */ 05940 wOffset = *writeOffset; 05941 05942 /* Loop over the blockSize */ 05943 i = blockSize; 05944 05945 while(i > 0u) 05946 { 05947 /* copy the input sample to the circular buffer */ 05948 circBuffer[wOffset] = *src; 05949 05950 /* Update the input pointer */ 05951 src += srcInc; 05952 05953 /* Circularly update wOffset. Watch out for positive and negative value */ 05954 wOffset += bufferInc; 05955 if(wOffset >= L) 05956 wOffset -= L; 05957 05958 /* Decrement the loop counter */ 05959 i--; 05960 } 05961 05962 /* Update the index pointer */ 05963 *writeOffset = wOffset; 05964 } 05965 05966 05967 05968 /** 05969 * @brief floating-point Circular Read function. 05970 */ 05971 static __INLINE void arm_circularRead_f32( 05972 int32_t * circBuffer, 05973 int32_t L, 05974 int32_t * readOffset, 05975 int32_t bufferInc, 05976 int32_t * dst, 05977 int32_t * dst_base, 05978 int32_t dst_length, 05979 int32_t dstInc, 05980 uint32_t blockSize) 05981 { 05982 uint32_t i = 0u; 05983 int32_t rOffset, dst_end; 05984 05985 /* Copy the value of Index pointer that points 05986 * to the current location from where the input samples to be read */ 05987 rOffset = *readOffset; 05988 dst_end = (int32_t) (dst_base + dst_length); 05989 05990 /* Loop over the blockSize */ 05991 i = blockSize; 05992 05993 while(i > 0u) 05994 { 05995 /* copy the sample from the circular buffer to the destination buffer */ 05996 *dst = circBuffer[rOffset]; 05997 05998 /* Update the input pointer */ 05999 dst += dstInc; 06000 06001 if(dst == (int32_t *) dst_end) 06002 { 06003 dst = dst_base; 06004 } 06005 06006 /* Circularly update rOffset. Watch out for positive and negative value */ 06007 rOffset += bufferInc; 06008 06009 if(rOffset >= L) 06010 { 06011 rOffset -= L; 06012 } 06013 06014 /* Decrement the loop counter */ 06015 i--; 06016 } 06017 06018 /* Update the index pointer */ 06019 *readOffset = rOffset; 06020 } 06021 06022 /** 06023 * @brief Q15 Circular write function. 06024 */ 06025 06026 static __INLINE void arm_circularWrite_q15( 06027 q15_t * circBuffer, 06028 int32_t L, 06029 uint16_t * writeOffset, 06030 int32_t bufferInc, 06031 const q15_t * src, 06032 int32_t srcInc, 06033 uint32_t blockSize) 06034 { 06035 uint32_t i = 0u; 06036 int32_t wOffset; 06037 06038 /* Copy the value of Index pointer that points 06039 * to the current location where the input samples to be copied */ 06040 wOffset = *writeOffset; 06041 06042 /* Loop over the blockSize */ 06043 i = blockSize; 06044 06045 while(i > 0u) 06046 { 06047 /* copy the input sample to the circular buffer */ 06048 circBuffer[wOffset] = *src; 06049 06050 /* Update the input pointer */ 06051 src += srcInc; 06052 06053 /* Circularly update wOffset. Watch out for positive and negative value */ 06054 wOffset += bufferInc; 06055 if(wOffset >= L) 06056 wOffset -= L; 06057 06058 /* Decrement the loop counter */ 06059 i--; 06060 } 06061 06062 /* Update the index pointer */ 06063 *writeOffset = wOffset; 06064 } 06065 06066 06067 06068 /** 06069 * @brief Q15 Circular Read function. 06070 */ 06071 static __INLINE void arm_circularRead_q15( 06072 q15_t * circBuffer, 06073 int32_t L, 06074 int32_t * readOffset, 06075 int32_t bufferInc, 06076 q15_t * dst, 06077 q15_t * dst_base, 06078 int32_t dst_length, 06079 int32_t dstInc, 06080 uint32_t blockSize) 06081 { 06082 uint32_t i = 0; 06083 int32_t rOffset, dst_end; 06084 06085 /* Copy the value of Index pointer that points 06086 * to the current location from where the input samples to be read */ 06087 rOffset = *readOffset; 06088 06089 dst_end = (int32_t) (dst_base + dst_length); 06090 06091 /* Loop over the blockSize */ 06092 i = blockSize; 06093 06094 while(i > 0u) 06095 { 06096 /* copy the sample from the circular buffer to the destination buffer */ 06097 *dst = circBuffer[rOffset]; 06098 06099 /* Update the input pointer */ 06100 dst += dstInc; 06101 06102 if(dst == (q15_t *) dst_end) 06103 { 06104 dst = dst_base; 06105 } 06106 06107 /* Circularly update wOffset. Watch out for positive and negative value */ 06108 rOffset += bufferInc; 06109 06110 if(rOffset >= L) 06111 { 06112 rOffset -= L; 06113 } 06114 06115 /* Decrement the loop counter */ 06116 i--; 06117 } 06118 06119 /* Update the index pointer */ 06120 *readOffset = rOffset; 06121 } 06122 06123 06124 /** 06125 * @brief Q7 Circular write function. 06126 */ 06127 06128 static __INLINE void arm_circularWrite_q7( 06129 q7_t * circBuffer, 06130 int32_t L, 06131 uint16_t * writeOffset, 06132 int32_t bufferInc, 06133 const q7_t * src, 06134 int32_t srcInc, 06135 uint32_t blockSize) 06136 { 06137 uint32_t i = 0u; 06138 int32_t wOffset; 06139 06140 /* Copy the value of Index pointer that points 06141 * to the current location where the input samples to be copied */ 06142 wOffset = *writeOffset; 06143 06144 /* Loop over the blockSize */ 06145 i = blockSize; 06146 06147 while(i > 0u) 06148 { 06149 /* copy the input sample to the circular buffer */ 06150 circBuffer[wOffset] = *src; 06151 06152 /* Update the input pointer */ 06153 src += srcInc; 06154 06155 /* Circularly update wOffset. Watch out for positive and negative value */ 06156 wOffset += bufferInc; 06157 if(wOffset >= L) 06158 wOffset -= L; 06159 06160 /* Decrement the loop counter */ 06161 i--; 06162 } 06163 06164 /* Update the index pointer */ 06165 *writeOffset = wOffset; 06166 } 06167 06168 06169 06170 /** 06171 * @brief Q7 Circular Read function. 06172 */ 06173 static __INLINE void arm_circularRead_q7( 06174 q7_t * circBuffer, 06175 int32_t L, 06176 int32_t * readOffset, 06177 int32_t bufferInc, 06178 q7_t * dst, 06179 q7_t * dst_base, 06180 int32_t dst_length, 06181 int32_t dstInc, 06182 uint32_t blockSize) 06183 { 06184 uint32_t i = 0; 06185 int32_t rOffset, dst_end; 06186 06187 /* Copy the value of Index pointer that points 06188 * to the current location from where the input samples to be read */ 06189 rOffset = *readOffset; 06190 06191 dst_end = (int32_t) (dst_base + dst_length); 06192 06193 /* Loop over the blockSize */ 06194 i = blockSize; 06195 06196 while(i > 0u) 06197 { 06198 /* copy the sample from the circular buffer to the destination buffer */ 06199 *dst = circBuffer[rOffset]; 06200 06201 /* Update the input pointer */ 06202 dst += dstInc; 06203 06204 if(dst == (q7_t *) dst_end) 06205 { 06206 dst = dst_base; 06207 } 06208 06209 /* Circularly update rOffset. Watch out for positive and negative value */ 06210 rOffset += bufferInc; 06211 06212 if(rOffset >= L) 06213 { 06214 rOffset -= L; 06215 } 06216 06217 /* Decrement the loop counter */ 06218 i--; 06219 } 06220 06221 /* Update the index pointer */ 06222 *readOffset = rOffset; 06223 } 06224 06225 06226 /** 06227 * @brief Sum of the squares of the elements of a Q31 vector. 06228 * @param[in] *pSrc is input pointer 06229 * @param[in] blockSize is the number of samples to process 06230 * @param[out] *pResult is output value. 06231 * @return none. 06232 */ 06233 06234 void arm_power_q31( 06235 q31_t * pSrc, 06236 uint32_t blockSize, 06237 q63_t * pResult); 06238 06239 /** 06240 * @brief Sum of the squares of the elements of a floating-point vector. 06241 * @param[in] *pSrc is input pointer 06242 * @param[in] blockSize is the number of samples to process 06243 * @param[out] *pResult is output value. 06244 * @return none. 06245 */ 06246 06247 void arm_power_f32( 06248 float32_t * pSrc, 06249 uint32_t blockSize, 06250 float32_t * pResult); 06251 06252 /** 06253 * @brief Sum of the squares of the elements of a Q15 vector. 06254 * @param[in] *pSrc is input pointer 06255 * @param[in] blockSize is the number of samples to process 06256 * @param[out] *pResult is output value. 06257 * @return none. 06258 */ 06259 06260 void arm_power_q15( 06261 q15_t * pSrc, 06262 uint32_t blockSize, 06263 q63_t * pResult); 06264 06265 /** 06266 * @brief Sum of the squares of the elements of a Q7 vector. 06267 * @param[in] *pSrc is input pointer 06268 * @param[in] blockSize is the number of samples to process 06269 * @param[out] *pResult is output value. 06270 * @return none. 06271 */ 06272 06273 void arm_power_q7( 06274 q7_t * pSrc, 06275 uint32_t blockSize, 06276 q31_t * pResult); 06277 06278 /** 06279 * @brief Mean value of a Q7 vector. 06280 * @param[in] *pSrc is input pointer 06281 * @param[in] blockSize is the number of samples to process 06282 * @param[out] *pResult is output value. 06283 * @return none. 06284 */ 06285 06286 void arm_mean_q7( 06287 q7_t * pSrc, 06288 uint32_t blockSize, 06289 q7_t * pResult); 06290 06291 /** 06292 * @brief Mean value of a Q15 vector. 06293 * @param[in] *pSrc is input pointer 06294 * @param[in] blockSize is the number of samples to process 06295 * @param[out] *pResult is output value. 06296 * @return none. 06297 */ 06298 void arm_mean_q15( 06299 q15_t * pSrc, 06300 uint32_t blockSize, 06301 q15_t * pResult); 06302 06303 /** 06304 * @brief Mean value of a Q31 vector. 06305 * @param[in] *pSrc is input pointer 06306 * @param[in] blockSize is the number of samples to process 06307 * @param[out] *pResult is output value. 06308 * @return none. 06309 */ 06310 void arm_mean_q31( 06311 q31_t * pSrc, 06312 uint32_t blockSize, 06313 q31_t * pResult); 06314 06315 /** 06316 * @brief Mean value of a floating-point vector. 06317 * @param[in] *pSrc is input pointer 06318 * @param[in] blockSize is the number of samples to process 06319 * @param[out] *pResult is output value. 06320 * @return none. 06321 */ 06322 void arm_mean_f32( 06323 float32_t * pSrc, 06324 uint32_t blockSize, 06325 float32_t * pResult); 06326 06327 /** 06328 * @brief Variance of the elements of a floating-point vector. 06329 * @param[in] *pSrc is input pointer 06330 * @param[in] blockSize is the number of samples to process 06331 * @param[out] *pResult is output value. 06332 * @return none. 06333 */ 06334 06335 void arm_var_f32( 06336 float32_t * pSrc, 06337 uint32_t blockSize, 06338 float32_t * pResult); 06339 06340 /** 06341 * @brief Variance of the elements of a Q31 vector. 06342 * @param[in] *pSrc is input pointer 06343 * @param[in] blockSize is the number of samples to process 06344 * @param[out] *pResult is output value. 06345 * @return none. 06346 */ 06347 06348 void arm_var_q31( 06349 q31_t * pSrc, 06350 uint32_t blockSize, 06351 q63_t * pResult); 06352 06353 /** 06354 * @brief Variance of the elements of a Q15 vector. 06355 * @param[in] *pSrc is input pointer 06356 * @param[in] blockSize is the number of samples to process 06357 * @param[out] *pResult is output value. 06358 * @return none. 06359 */ 06360 06361 void arm_var_q15( 06362 q15_t * pSrc, 06363 uint32_t blockSize, 06364 q31_t * pResult); 06365 06366 /** 06367 * @brief Root Mean Square of the elements of a floating-point vector. 06368 * @param[in] *pSrc is input pointer 06369 * @param[in] blockSize is the number of samples to process 06370 * @param[out] *pResult is output value. 06371 * @return none. 06372 */ 06373 06374 void arm_rms_f32( 06375 float32_t * pSrc, 06376 uint32_t blockSize, 06377 float32_t * pResult); 06378 06379 /** 06380 * @brief Root Mean Square of the elements of a Q31 vector. 06381 * @param[in] *pSrc is input pointer 06382 * @param[in] blockSize is the number of samples to process 06383 * @param[out] *pResult is output value. 06384 * @return none. 06385 */ 06386 06387 void arm_rms_q31( 06388 q31_t * pSrc, 06389 uint32_t blockSize, 06390 q31_t * pResult); 06391 06392 /** 06393 * @brief Root Mean Square of the elements of a Q15 vector. 06394 * @param[in] *pSrc is input pointer 06395 * @param[in] blockSize is the number of samples to process 06396 * @param[out] *pResult is output value. 06397 * @return none. 06398 */ 06399 06400 void arm_rms_q15( 06401 q15_t * pSrc, 06402 uint32_t blockSize, 06403 q15_t * pResult); 06404 06405 /** 06406 * @brief Standard deviation of the elements of a floating-point vector. 06407 * @param[in] *pSrc is input pointer 06408 * @param[in] blockSize is the number of samples to process 06409 * @param[out] *pResult is output value. 06410 * @return none. 06411 */ 06412 06413 void arm_std_f32( 06414 float32_t * pSrc, 06415 uint32_t blockSize, 06416 float32_t * pResult); 06417 06418 /** 06419 * @brief Standard deviation of the elements of a Q31 vector. 06420 * @param[in] *pSrc is input pointer 06421 * @param[in] blockSize is the number of samples to process 06422 * @param[out] *pResult is output value. 06423 * @return none. 06424 */ 06425 06426 void arm_std_q31( 06427 q31_t * pSrc, 06428 uint32_t blockSize, 06429 q31_t * pResult); 06430 06431 /** 06432 * @brief Standard deviation of the elements of a Q15 vector. 06433 * @param[in] *pSrc is input pointer 06434 * @param[in] blockSize is the number of samples to process 06435 * @param[out] *pResult is output value. 06436 * @return none. 06437 */ 06438 06439 void arm_std_q15( 06440 q15_t * pSrc, 06441 uint32_t blockSize, 06442 q15_t * pResult); 06443 06444 /** 06445 * @brief Floating-point complex magnitude 06446 * @param[in] *pSrc points to the complex input vector 06447 * @param[out] *pDst points to the real output vector 06448 * @param[in] numSamples number of complex samples in the input vector 06449 * @return none. 06450 */ 06451 06452 void arm_cmplx_mag_f32( 06453 float32_t * pSrc, 06454 float32_t * pDst, 06455 uint32_t numSamples); 06456 06457 /** 06458 * @brief Q31 complex magnitude 06459 * @param[in] *pSrc points to the complex input vector 06460 * @param[out] *pDst points to the real output vector 06461 * @param[in] numSamples number of complex samples in the input vector 06462 * @return none. 06463 */ 06464 06465 void arm_cmplx_mag_q31( 06466 q31_t * pSrc, 06467 q31_t * pDst, 06468 uint32_t numSamples); 06469 06470 /** 06471 * @brief Q15 complex magnitude 06472 * @param[in] *pSrc points to the complex input vector 06473 * @param[out] *pDst points to the real output vector 06474 * @param[in] numSamples number of complex samples in the input vector 06475 * @return none. 06476 */ 06477 06478 void arm_cmplx_mag_q15( 06479 q15_t * pSrc, 06480 q15_t * pDst, 06481 uint32_t numSamples); 06482 06483 /** 06484 * @brief Q15 complex dot product 06485 * @param[in] *pSrcA points to the first input vector 06486 * @param[in] *pSrcB points to the second input vector 06487 * @param[in] numSamples number of complex samples in each vector 06488 * @param[out] *realResult real part of the result returned here 06489 * @param[out] *imagResult imaginary part of the result returned here 06490 * @return none. 06491 */ 06492 06493 void arm_cmplx_dot_prod_q15( 06494 q15_t * pSrcA, 06495 q15_t * pSrcB, 06496 uint32_t numSamples, 06497 q31_t * realResult, 06498 q31_t * imagResult); 06499 06500 /** 06501 * @brief Q31 complex dot product 06502 * @param[in] *pSrcA points to the first input vector 06503 * @param[in] *pSrcB points to the second input vector 06504 * @param[in] numSamples number of complex samples in each vector 06505 * @param[out] *realResult real part of the result returned here 06506 * @param[out] *imagResult imaginary part of the result returned here 06507 * @return none. 06508 */ 06509 06510 void arm_cmplx_dot_prod_q31( 06511 q31_t * pSrcA, 06512 q31_t * pSrcB, 06513 uint32_t numSamples, 06514 q63_t * realResult, 06515 q63_t * imagResult); 06516 06517 /** 06518 * @brief Floating-point complex dot product 06519 * @param[in] *pSrcA points to the first input vector 06520 * @param[in] *pSrcB points to the second input vector 06521 * @param[in] numSamples number of complex samples in each vector 06522 * @param[out] *realResult real part of the result returned here 06523 * @param[out] *imagResult imaginary part of the result returned here 06524 * @return none. 06525 */ 06526 06527 void arm_cmplx_dot_prod_f32( 06528 float32_t * pSrcA, 06529 float32_t * pSrcB, 06530 uint32_t numSamples, 06531 float32_t * realResult, 06532 float32_t * imagResult); 06533 06534 /** 06535 * @brief Q15 complex-by-real multiplication 06536 * @param[in] *pSrcCmplx points to the complex input vector 06537 * @param[in] *pSrcReal points to the real input vector 06538 * @param[out] *pCmplxDst points to the complex output vector 06539 * @param[in] numSamples number of samples in each vector 06540 * @return none. 06541 */ 06542 06543 void arm_cmplx_mult_real_q15( 06544 q15_t * pSrcCmplx, 06545 q15_t * pSrcReal, 06546 q15_t * pCmplxDst, 06547 uint32_t numSamples); 06548 06549 /** 06550 * @brief Q31 complex-by-real multiplication 06551 * @param[in] *pSrcCmplx points to the complex input vector 06552 * @param[in] *pSrcReal points to the real input vector 06553 * @param[out] *pCmplxDst points to the complex output vector 06554 * @param[in] numSamples number of samples in each vector 06555 * @return none. 06556 */ 06557 06558 void arm_cmplx_mult_real_q31( 06559 q31_t * pSrcCmplx, 06560 q31_t * pSrcReal, 06561 q31_t * pCmplxDst, 06562 uint32_t numSamples); 06563 06564 /** 06565 * @brief Floating-point complex-by-real multiplication 06566 * @param[in] *pSrcCmplx points to the complex input vector 06567 * @param[in] *pSrcReal points to the real input vector 06568 * @param[out] *pCmplxDst points to the complex output vector 06569 * @param[in] numSamples number of samples in each vector 06570 * @return none. 06571 */ 06572 06573 void arm_cmplx_mult_real_f32( 06574 float32_t * pSrcCmplx, 06575 float32_t * pSrcReal, 06576 float32_t * pCmplxDst, 06577 uint32_t numSamples); 06578 06579 /** 06580 * @brief Minimum value of a Q7 vector. 06581 * @param[in] *pSrc is input pointer 06582 * @param[in] blockSize is the number of samples to process 06583 * @param[out] *result is output pointer 06584 * @param[in] index is the array index of the minimum value in the input buffer. 06585 * @return none. 06586 */ 06587 06588 void arm_min_q7( 06589 q7_t * pSrc, 06590 uint32_t blockSize, 06591 q7_t * result, 06592 uint32_t * index); 06593 06594 /** 06595 * @brief Minimum value of a Q15 vector. 06596 * @param[in] *pSrc is input pointer 06597 * @param[in] blockSize is the number of samples to process 06598 * @param[out] *pResult is output pointer 06599 * @param[in] *pIndex is the array index of the minimum value in the input buffer. 06600 * @return none. 06601 */ 06602 06603 void arm_min_q15( 06604 q15_t * pSrc, 06605 uint32_t blockSize, 06606 q15_t * pResult, 06607 uint32_t * pIndex); 06608 06609 /** 06610 * @brief Minimum value of a Q31 vector. 06611 * @param[in] *pSrc is input pointer 06612 * @param[in] blockSize is the number of samples to process 06613 * @param[out] *pResult is output pointer 06614 * @param[out] *pIndex is the array index of the minimum value in the input buffer. 06615 * @return none. 06616 */ 06617 void arm_min_q31( 06618 q31_t * pSrc, 06619 uint32_t blockSize, 06620 q31_t * pResult, 06621 uint32_t * pIndex); 06622 06623 /** 06624 * @brief Minimum value of a floating-point vector. 06625 * @param[in] *pSrc is input pointer 06626 * @param[in] blockSize is the number of samples to process 06627 * @param[out] *pResult is output pointer 06628 * @param[out] *pIndex is the array index of the minimum value in the input buffer. 06629 * @return none. 06630 */ 06631 06632 void arm_min_f32( 06633 float32_t * pSrc, 06634 uint32_t blockSize, 06635 float32_t * pResult, 06636 uint32_t * pIndex); 06637 06638 /** 06639 * @brief Maximum value of a Q7 vector. 06640 * @param[in] *pSrc points to the input buffer 06641 * @param[in] blockSize length of the input vector 06642 * @param[out] *pResult maximum value returned here 06643 * @param[out] *pIndex index of maximum value returned here 06644 * @return none. 06645 */ 06646 06647 void arm_max_q7( 06648 q7_t * pSrc, 06649 uint32_t blockSize, 06650 q7_t * pResult, 06651 uint32_t * pIndex); 06652 06653 /** 06654 * @brief Maximum value of a Q15 vector. 06655 * @param[in] *pSrc points to the input buffer 06656 * @param[in] blockSize length of the input vector 06657 * @param[out] *pResult maximum value returned here 06658 * @param[out] *pIndex index of maximum value returned here 06659 * @return none. 06660 */ 06661 06662 void arm_max_q15( 06663 q15_t * pSrc, 06664 uint32_t blockSize, 06665 q15_t * pResult, 06666 uint32_t * pIndex); 06667 06668 /** 06669 * @brief Maximum value of a Q31 vector. 06670 * @param[in] *pSrc points to the input buffer 06671 * @param[in] blockSize length of the input vector 06672 * @param[out] *pResult maximum value returned here 06673 * @param[out] *pIndex index of maximum value returned here 06674 * @return none. 06675 */ 06676 06677 void arm_max_q31( 06678 q31_t * pSrc, 06679 uint32_t blockSize, 06680 q31_t * pResult, 06681 uint32_t * pIndex); 06682 06683 /** 06684 * @brief Maximum value of a floating-point vector. 06685 * @param[in] *pSrc points to the input buffer 06686 * @param[in] blockSize length of the input vector 06687 * @param[out] *pResult maximum value returned here 06688 * @param[out] *pIndex index of maximum value returned here 06689 * @return none. 06690 */ 06691 06692 void arm_max_f32( 06693 float32_t * pSrc, 06694 uint32_t blockSize, 06695 float32_t * pResult, 06696 uint32_t * pIndex); 06697 06698 /** 06699 * @brief Q15 complex-by-complex multiplication 06700 * @param[in] *pSrcA points to the first input vector 06701 * @param[in] *pSrcB points to the second input vector 06702 * @param[out] *pDst points to the output vector 06703 * @param[in] numSamples number of complex samples in each vector 06704 * @return none. 06705 */ 06706 06707 void arm_cmplx_mult_cmplx_q15( 06708 q15_t * pSrcA, 06709 q15_t * pSrcB, 06710 q15_t * pDst, 06711 uint32_t numSamples); 06712 06713 /** 06714 * @brief Q31 complex-by-complex multiplication 06715 * @param[in] *pSrcA points to the first input vector 06716 * @param[in] *pSrcB points to the second input vector 06717 * @param[out] *pDst points to the output vector 06718 * @param[in] numSamples number of complex samples in each vector 06719 * @return none. 06720 */ 06721 06722 void arm_cmplx_mult_cmplx_q31( 06723 q31_t * pSrcA, 06724 q31_t * pSrcB, 06725 q31_t * pDst, 06726 uint32_t numSamples); 06727 06728 /** 06729 * @brief Floating-point complex-by-complex multiplication 06730 * @param[in] *pSrcA points to the first input vector 06731 * @param[in] *pSrcB points to the second input vector 06732 * @param[out] *pDst points to the output vector 06733 * @param[in] numSamples number of complex samples in each vector 06734 * @return none. 06735 */ 06736 06737 void arm_cmplx_mult_cmplx_f32( 06738 float32_t * pSrcA, 06739 float32_t * pSrcB, 06740 float32_t * pDst, 06741 uint32_t numSamples); 06742 06743 /** 06744 * @brief Converts the elements of the floating-point vector to Q31 vector. 06745 * @param[in] *pSrc points to the floating-point input vector 06746 * @param[out] *pDst points to the Q31 output vector 06747 * @param[in] blockSize length of the input vector 06748 * @return none. 06749 */ 06750 void arm_float_to_q31( 06751 float32_t * pSrc, 06752 q31_t * pDst, 06753 uint32_t blockSize); 06754 06755 /** 06756 * @brief Converts the elements of the floating-point vector to Q15 vector. 06757 * @param[in] *pSrc points to the floating-point input vector 06758 * @param[out] *pDst points to the Q15 output vector 06759 * @param[in] blockSize length of the input vector 06760 * @return none 06761 */ 06762 void arm_float_to_q15( 06763 float32_t * pSrc, 06764 q15_t * pDst, 06765 uint32_t blockSize); 06766 06767 /** 06768 * @brief Converts the elements of the floating-point vector to Q7 vector. 06769 * @param[in] *pSrc points to the floating-point input vector 06770 * @param[out] *pDst points to the Q7 output vector 06771 * @param[in] blockSize length of the input vector 06772 * @return none 06773 */ 06774 void arm_float_to_q7( 06775 float32_t * pSrc, 06776 q7_t * pDst, 06777 uint32_t blockSize); 06778 06779 06780 /** 06781 * @brief Converts the elements of the Q31 vector to Q15 vector. 06782 * @param[in] *pSrc is input pointer 06783 * @param[out] *pDst is output pointer 06784 * @param[in] blockSize is the number of samples to process 06785 * @return none. 06786 */ 06787 void arm_q31_to_q15( 06788 q31_t * pSrc, 06789 q15_t * pDst, 06790 uint32_t blockSize); 06791 06792 /** 06793 * @brief Converts the elements of the Q31 vector to Q7 vector. 06794 * @param[in] *pSrc is input pointer 06795 * @param[out] *pDst is output pointer 06796 * @param[in] blockSize is the number of samples to process 06797 * @return none. 06798 */ 06799 void arm_q31_to_q7( 06800 q31_t * pSrc, 06801 q7_t * pDst, 06802 uint32_t blockSize); 06803 06804 /** 06805 * @brief Converts the elements of the Q15 vector to floating-point vector. 06806 * @param[in] *pSrc is input pointer 06807 * @param[out] *pDst is output pointer 06808 * @param[in] blockSize is the number of samples to process 06809 * @return none. 06810 */ 06811 void arm_q15_to_float( 06812 q15_t * pSrc, 06813 float32_t * pDst, 06814 uint32_t blockSize); 06815 06816 06817 /** 06818 * @brief Converts the elements of the Q15 vector to Q31 vector. 06819 * @param[in] *pSrc is input pointer 06820 * @param[out] *pDst is output pointer 06821 * @param[in] blockSize is the number of samples to process 06822 * @return none. 06823 */ 06824 void arm_q15_to_q31( 06825 q15_t * pSrc, 06826 q31_t * pDst, 06827 uint32_t blockSize); 06828 06829 06830 /** 06831 * @brief Converts the elements of the Q15 vector to Q7 vector. 06832 * @param[in] *pSrc is input pointer 06833 * @param[out] *pDst is output pointer 06834 * @param[in] blockSize is the number of samples to process 06835 * @return none. 06836 */ 06837 void arm_q15_to_q7( 06838 q15_t * pSrc, 06839 q7_t * pDst, 06840 uint32_t blockSize); 06841 06842 06843 /** 06844 * @ingroup groupInterpolation 06845 */ 06846 06847 /** 06848 * @defgroup BilinearInterpolate Bilinear Interpolation 06849 * 06850 * Bilinear interpolation is an extension of linear interpolation applied to a two dimensional grid. 06851 * The underlying function <code>f(x, y)</code> is sampled on a regular grid and the interpolation process 06852 * determines values between the grid points. 06853 * Bilinear interpolation is equivalent to two step linear interpolation, first in the x-dimension and then in the y-dimension. 06854 * Bilinear interpolation is often used in image processing to rescale images. 06855 * The CMSIS DSP library provides bilinear interpolation functions for Q7, Q15, Q31, and floating-point data types. 06856 * 06857 * <b>Algorithm</b> 06858 * \par 06859 * The instance structure used by the bilinear interpolation functions describes a two dimensional data table. 06860 * For floating-point, the instance structure is defined as: 06861 * <pre> 06862 * typedef struct 06863 * { 06864 * uint16_t numRows; 06865 * uint16_t numCols; 06866 * float32_t *pData; 06867 * } arm_bilinear_interp_instance_f32; 06868 * </pre> 06869 * 06870 * \par 06871 * where <code>numRows</code> specifies the number of rows in the table; 06872 * <code>numCols</code> specifies the number of columns in the table; 06873 * and <code>pData</code> points to an array of size <code>numRows*numCols</code> values. 06874 * The data table <code>pTable</code> is organized in row order and the supplied data values fall on integer indexes. 06875 * That is, table element (x,y) is located at <code>pTable[x + y*numCols]</code> where x and y are integers. 06876 * 06877 * \par 06878 * Let <code>(x, y)</code> specify the desired interpolation point. Then define: 06879 * <pre> 06880 * XF = floor(x) 06881 * YF = floor(y) 06882 * </pre> 06883 * \par 06884 * The interpolated output point is computed as: 06885 * <pre> 06886 * f(x, y) = f(XF, YF) * (1-(x-XF)) * (1-(y-YF)) 06887 * + f(XF+1, YF) * (x-XF)*(1-(y-YF)) 06888 * + f(XF, YF+1) * (1-(x-XF))*(y-YF) 06889 * + f(XF+1, YF+1) * (x-XF)*(y-YF) 06890 * </pre> 06891 * Note that the coordinates (x, y) contain integer and fractional components. 06892 * The integer components specify which portion of the table to use while the 06893 * fractional components control the interpolation processor. 06894 * 06895 * \par 06896 * if (x,y) are outside of the table boundary, Bilinear interpolation returns zero output. 06897 */ 06898 06899 /** 06900 * @addtogroup BilinearInterpolate 06901 * @{ 06902 */ 06903 06904 /** 06905 * 06906 * @brief Floating-point bilinear interpolation. 06907 * @param[in,out] *S points to an instance of the interpolation structure. 06908 * @param[in] X interpolation coordinate. 06909 * @param[in] Y interpolation coordinate. 06910 * @return out interpolated value. 06911 */ 06912 06913 06914 static __INLINE float32_t arm_bilinear_interp_f32( 06915 const arm_bilinear_interp_instance_f32 * S, 06916 float32_t X, 06917 float32_t Y) 06918 { 06919 float32_t out; 06920 float32_t f00, f01, f10, f11; 06921 float32_t *pData = S->pData; 06922 int32_t xIndex, yIndex, index; 06923 float32_t xdiff, ydiff; 06924 float32_t b1, b2, b3, b4; 06925 06926 xIndex = (int32_t) X; 06927 yIndex = (int32_t) Y; 06928 06929 /* Care taken for table outside boundary */ 06930 /* Returns zero output when values are outside table boundary */ 06931 if(xIndex < 0 || xIndex > (S->numRows - 1) || yIndex < 0 06932 || yIndex > (S->numCols - 1)) 06933 { 06934 return (0); 06935 } 06936 06937 /* Calculation of index for two nearest points in X-direction */ 06938 index = (xIndex - 1) + (yIndex - 1) * S->numCols; 06939 06940 06941 /* Read two nearest points in X-direction */ 06942 f00 = pData[index]; 06943 f01 = pData[index + 1]; 06944 06945 /* Calculation of index for two nearest points in Y-direction */ 06946 index = (xIndex - 1) + (yIndex) * S->numCols; 06947 06948 06949 /* Read two nearest points in Y-direction */ 06950 f10 = pData[index]; 06951 f11 = pData[index + 1]; 06952 06953 /* Calculation of intermediate values */ 06954 b1 = f00; 06955 b2 = f01 - f00; 06956 b3 = f10 - f00; 06957 b4 = f00 - f01 - f10 + f11; 06958 06959 /* Calculation of fractional part in X */ 06960 xdiff = X - xIndex; 06961 06962 /* Calculation of fractional part in Y */ 06963 ydiff = Y - yIndex; 06964 06965 /* Calculation of bi-linear interpolated output */ 06966 out = b1 + b2 * xdiff + b3 * ydiff + b4 * xdiff * ydiff; 06967 06968 /* return to application */ 06969 return (out); 06970 06971 } 06972 06973 /** 06974 * 06975 * @brief Q31 bilinear interpolation. 06976 * @param[in,out] *S points to an instance of the interpolation structure. 06977 * @param[in] X interpolation coordinate in 12.20 format. 06978 * @param[in] Y interpolation coordinate in 12.20 format. 06979 * @return out interpolated value. 06980 */ 06981 06982 static __INLINE q31_t arm_bilinear_interp_q31( 06983 arm_bilinear_interp_instance_q31 * S, 06984 q31_t X, 06985 q31_t Y) 06986 { 06987 q31_t out; /* Temporary output */ 06988 q31_t acc = 0; /* output */ 06989 q31_t xfract, yfract; /* X, Y fractional parts */ 06990 q31_t x1, x2, y1, y2; /* Nearest output values */ 06991 int32_t rI, cI; /* Row and column indices */ 06992 q31_t *pYData = S->pData; /* pointer to output table values */ 06993 uint32_t nCols = S->numCols; /* num of rows */ 06994 06995 06996 /* Input is in 12.20 format */ 06997 /* 12 bits for the table index */ 06998 /* Index value calculation */ 06999 rI = ((X & 0xFFF00000) >> 20u); 07000 07001 /* Input is in 12.20 format */ 07002 /* 12 bits for the table index */ 07003 /* Index value calculation */ 07004 cI = ((Y & 0xFFF00000) >> 20u); 07005 07006 /* Care taken for table outside boundary */ 07007 /* Returns zero output when values are outside table boundary */ 07008 if(rI < 0 || rI > (S->numRows - 1) || cI < 0 || cI > (S->numCols - 1)) 07009 { 07010 return (0); 07011 } 07012 07013 /* 20 bits for the fractional part */ 07014 /* shift left xfract by 11 to keep 1.31 format */ 07015 xfract = (X & 0x000FFFFF) << 11u; 07016 07017 /* Read two nearest output values from the index */ 07018 x1 = pYData[(rI) + nCols * (cI)]; 07019 x2 = pYData[(rI) + nCols * (cI) + 1u]; 07020 07021 /* 20 bits for the fractional part */ 07022 /* shift left yfract by 11 to keep 1.31 format */ 07023 yfract = (Y & 0x000FFFFF) << 11u; 07024 07025 /* Read two nearest output values from the index */ 07026 y1 = pYData[(rI) + nCols * (cI + 1)]; 07027 y2 = pYData[(rI) + nCols * (cI + 1) + 1u]; 07028 07029 /* Calculation of x1 * (1-xfract ) * (1-yfract) and acc is in 3.29(q29) format */ 07030 out = ((q31_t) (((q63_t) x1 * (0x7FFFFFFF - xfract)) >> 32)); 07031 acc = ((q31_t) (((q63_t) out * (0x7FFFFFFF - yfract)) >> 32)); 07032 07033 /* x2 * (xfract) * (1-yfract) in 3.29(q29) and adding to acc */ 07034 out = ((q31_t) ((q63_t) x2 * (0x7FFFFFFF - yfract) >> 32)); 07035 acc += ((q31_t) ((q63_t) out * (xfract) >> 32)); 07036 07037 /* y1 * (1 - xfract) * (yfract) in 3.29(q29) and adding to acc */ 07038 out = ((q31_t) ((q63_t) y1 * (0x7FFFFFFF - xfract) >> 32)); 07039 acc += ((q31_t) ((q63_t) out * (yfract) >> 32)); 07040 07041 /* y2 * (xfract) * (yfract) in 3.29(q29) and adding to acc */ 07042 out = ((q31_t) ((q63_t) y2 * (xfract) >> 32)); 07043 acc += ((q31_t) ((q63_t) out * (yfract) >> 32)); 07044 07045 /* Convert acc to 1.31(q31) format */ 07046 return (acc << 2u); 07047 07048 } 07049 07050 /** 07051 * @brief Q15 bilinear interpolation. 07052 * @param[in,out] *S points to an instance of the interpolation structure. 07053 * @param[in] X interpolation coordinate in 12.20 format. 07054 * @param[in] Y interpolation coordinate in 12.20 format. 07055 * @return out interpolated value. 07056 */ 07057 07058 static __INLINE q15_t arm_bilinear_interp_q15( 07059 arm_bilinear_interp_instance_q15 * S, 07060 q31_t X, 07061 q31_t Y) 07062 { 07063 q63_t acc = 0; /* output */ 07064 q31_t out; /* Temporary output */ 07065 q15_t x1, x2, y1, y2; /* Nearest output values */ 07066 q31_t xfract, yfract; /* X, Y fractional parts */ 07067 int32_t rI, cI; /* Row and column indices */ 07068 q15_t *pYData = S->pData; /* pointer to output table values */ 07069 uint32_t nCols = S->numCols; /* num of rows */ 07070 07071 /* Input is in 12.20 format */ 07072 /* 12 bits for the table index */ 07073 /* Index value calculation */ 07074 rI = ((X & 0xFFF00000) >> 20); 07075 07076 /* Input is in 12.20 format */ 07077 /* 12 bits for the table index */ 07078 /* Index value calculation */ 07079 cI = ((Y & 0xFFF00000) >> 20); 07080 07081 /* Care taken for table outside boundary */ 07082 /* Returns zero output when values are outside table boundary */ 07083 if(rI < 0 || rI > (S->numRows - 1) || cI < 0 || cI > (S->numCols - 1)) 07084 { 07085 return (0); 07086 } 07087 07088 /* 20 bits for the fractional part */ 07089 /* xfract should be in 12.20 format */ 07090 xfract = (X & 0x000FFFFF); 07091 07092 /* Read two nearest output values from the index */ 07093 x1 = pYData[(rI) + nCols * (cI)]; 07094 x2 = pYData[(rI) + nCols * (cI) + 1u]; 07095 07096 07097 /* 20 bits for the fractional part */ 07098 /* yfract should be in 12.20 format */ 07099 yfract = (Y & 0x000FFFFF); 07100 07101 /* Read two nearest output values from the index */ 07102 y1 = pYData[(rI) + nCols * (cI + 1)]; 07103 y2 = pYData[(rI) + nCols * (cI + 1) + 1u]; 07104 07105 /* Calculation of x1 * (1-xfract ) * (1-yfract) and acc is in 13.51 format */ 07106 07107 /* x1 is in 1.15(q15), xfract in 12.20 format and out is in 13.35 format */ 07108 /* convert 13.35 to 13.31 by right shifting and out is in 1.31 */ 07109 out = (q31_t) (((q63_t) x1 * (0xFFFFF - xfract)) >> 4u); 07110 acc = ((q63_t) out * (0xFFFFF - yfract)); 07111 07112 /* x2 * (xfract) * (1-yfract) in 1.51 and adding to acc */ 07113 out = (q31_t) (((q63_t) x2 * (0xFFFFF - yfract)) >> 4u); 07114 acc += ((q63_t) out * (xfract)); 07115 07116 /* y1 * (1 - xfract) * (yfract) in 1.51 and adding to acc */ 07117 out = (q31_t) (((q63_t) y1 * (0xFFFFF - xfract)) >> 4u); 07118 acc += ((q63_t) out * (yfract)); 07119 07120 /* y2 * (xfract) * (yfract) in 1.51 and adding to acc */ 07121 out = (q31_t) (((q63_t) y2 * (xfract)) >> 4u); 07122 acc += ((q63_t) out * (yfract)); 07123 07124 /* acc is in 13.51 format and down shift acc by 36 times */ 07125 /* Convert out to 1.15 format */ 07126 return (acc >> 36); 07127 07128 } 07129 07130 /** 07131 * @brief Q7 bilinear interpolation. 07132 * @param[in,out] *S points to an instance of the interpolation structure. 07133 * @param[in] X interpolation coordinate in 12.20 format. 07134 * @param[in] Y interpolation coordinate in 12.20 format. 07135 * @return out interpolated value. 07136 */ 07137 07138 static __INLINE q7_t arm_bilinear_interp_q7( 07139 arm_bilinear_interp_instance_q7 * S, 07140 q31_t X, 07141 q31_t Y) 07142 { 07143 q63_t acc = 0; /* output */ 07144 q31_t out; /* Temporary output */ 07145 q31_t xfract, yfract; /* X, Y fractional parts */ 07146 q7_t x1, x2, y1, y2; /* Nearest output values */ 07147 int32_t rI, cI; /* Row and column indices */ 07148 q7_t *pYData = S->pData; /* pointer to output table values */ 07149 uint32_t nCols = S->numCols; /* num of rows */ 07150 07151 /* Input is in 12.20 format */ 07152 /* 12 bits for the table index */ 07153 /* Index value calculation */ 07154 rI = ((X & 0xFFF00000) >> 20); 07155 07156 /* Input is in 12.20 format */ 07157 /* 12 bits for the table index */ 07158 /* Index value calculation */ 07159 cI = ((Y & 0xFFF00000) >> 20); 07160 07161 /* Care taken for table outside boundary */ 07162 /* Returns zero output when values are outside table boundary */ 07163 if(rI < 0 || rI > (S->numRows - 1) || cI < 0 || cI > (S->numCols - 1)) 07164 { 07165 return (0); 07166 } 07167 07168 /* 20 bits for the fractional part */ 07169 /* xfract should be in 12.20 format */ 07170 xfract = (X & 0x000FFFFF); 07171 07172 /* Read two nearest output values from the index */ 07173 x1 = pYData[(rI) + nCols * (cI)]; 07174 x2 = pYData[(rI) + nCols * (cI) + 1u]; 07175 07176 07177 /* 20 bits for the fractional part */ 07178 /* yfract should be in 12.20 format */ 07179 yfract = (Y & 0x000FFFFF); 07180 07181 /* Read two nearest output values from the index */ 07182 y1 = pYData[(rI) + nCols * (cI + 1)]; 07183 y2 = pYData[(rI) + nCols * (cI + 1) + 1u]; 07184 07185 /* Calculation of x1 * (1-xfract ) * (1-yfract) and acc is in 16.47 format */ 07186 out = ((x1 * (0xFFFFF - xfract))); 07187 acc = (((q63_t) out * (0xFFFFF - yfract))); 07188 07189 /* x2 * (xfract) * (1-yfract) in 2.22 and adding to acc */ 07190 out = ((x2 * (0xFFFFF - yfract))); 07191 acc += (((q63_t) out * (xfract))); 07192 07193 /* y1 * (1 - xfract) * (yfract) in 2.22 and adding to acc */ 07194 out = ((y1 * (0xFFFFF - xfract))); 07195 acc += (((q63_t) out * (yfract))); 07196 07197 /* y2 * (xfract) * (yfract) in 2.22 and adding to acc */ 07198 out = ((y2 * (yfract))); 07199 acc += (((q63_t) out * (xfract))); 07200 07201 /* acc in 16.47 format and down shift by 40 to convert to 1.7 format */ 07202 return (acc >> 40); 07203 07204 } 07205 07206 /** 07207 * @} end of BilinearInterpolate group 07208 */ 07209 07210 07211 #if defined ( __CC_ARM ) //Keil 07212 //SMMLAR 07213 #define multAcc_32x32_keep32_R(a, x, y) \ 07214 a = (q31_t) (((((q63_t) a) << 32) + ((q63_t) x * y) + 0x80000000LL ) >> 32) 07215 07216 //SMMLSR 07217 #define multSub_32x32_keep32_R(a, x, y) \ 07218 a = (q31_t) (((((q63_t) a) << 32) - ((q63_t) x * y) + 0x80000000LL ) >> 32) 07219 07220 //SMMULR 07221 #define mult_32x32_keep32_R(a, x, y) \ 07222 a = (q31_t) (((q63_t) x * y + 0x80000000LL ) >> 32) 07223 07224 //Enter low optimization region - place directly above function definition 07225 #define LOW_OPTIMIZATION_ENTER \ 07226 _Pragma ("push") \ 07227 _Pragma ("O1") 07228 07229 //Exit low optimization region - place directly after end of function definition 07230 #define LOW_OPTIMIZATION_EXIT \ 07231 _Pragma ("pop") 07232 07233 //Enter low optimization region - place directly above function definition 07234 #define IAR_ONLY_LOW_OPTIMIZATION_ENTER 07235 07236 //Exit low optimization region - place directly after end of function definition 07237 #define IAR_ONLY_LOW_OPTIMIZATION_EXIT 07238 07239 #elif defined(__ICCARM__) //IAR 07240 //SMMLA 07241 #define multAcc_32x32_keep32_R(a, x, y) \ 07242 a += (q31_t) (((q63_t) x * y) >> 32) 07243 07244 //SMMLS 07245 #define multSub_32x32_keep32_R(a, x, y) \ 07246 a -= (q31_t) (((q63_t) x * y) >> 32) 07247 07248 //SMMUL 07249 #define mult_32x32_keep32_R(a, x, y) \ 07250 a = (q31_t) (((q63_t) x * y ) >> 32) 07251 07252 //Enter low optimization region - place directly above function definition 07253 #define LOW_OPTIMIZATION_ENTER \ 07254 _Pragma ("optimize=low") 07255 07256 //Exit low optimization region - place directly after end of function definition 07257 #define LOW_OPTIMIZATION_EXIT 07258 07259 //Enter low optimization region - place directly above function definition 07260 #define IAR_ONLY_LOW_OPTIMIZATION_ENTER \ 07261 _Pragma ("optimize=low") 07262 07263 //Exit low optimization region - place directly after end of function definition 07264 #define IAR_ONLY_LOW_OPTIMIZATION_EXIT 07265 07266 #elif defined(__GNUC__) 07267 //SMMLA 07268 #define multAcc_32x32_keep32_R(a, x, y) \ 07269 a += (q31_t) (((q63_t) x * y) >> 32) 07270 07271 //SMMLS 07272 #define multSub_32x32_keep32_R(a, x, y) \ 07273 a -= (q31_t) (((q63_t) x * y) >> 32) 07274 07275 //SMMUL 07276 #define mult_32x32_keep32_R(a, x, y) \ 07277 a = (q31_t) (((q63_t) x * y ) >> 32) 07278 07279 #define LOW_OPTIMIZATION_ENTER __attribute__(( optimize("-O1") )) 07280 07281 #define LOW_OPTIMIZATION_EXIT 07282 07283 #define IAR_ONLY_LOW_OPTIMIZATION_ENTER 07284 07285 #define IAR_ONLY_LOW_OPTIMIZATION_EXIT 07286 07287 #endif 07288 07289 07290 07291 07292 07293 #ifdef __cplusplus 07294 } 07295 #endif 07296 07297 07298 #endif /* _ARM_MATH_H */ 07299 07300 07301 /** 07302 * 07303 * End of file. 07304 */
Generated on Tue Jul 12 2022 12:36:56 by
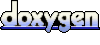