A library for talking to Multi-Tech's Cellular SocketModem Devices.
Dependents: M2X_dev axeda_wrapper_dev MTS_M2x_Example1 MTS_Cellular_Connect_Example ... more
Cellular Class Reference
This is a class for communicating with a Multi-Tech Systems SocketModem iCell. More...
#include <Cellular.h>
Inherits mts::IPStack.
Data Structures | |
struct | Sms |
This structure contains the data for an SMS message. More... | |
Public Types | |
enum | Registration |
An enumeration of radio registration states with a cell tower. More... | |
Public Member Functions | |
~Cellular () | |
Destructs a Cellular object and frees all related resources. | |
bool | init (MTSBufferedIO *io, PinName DCD=NC, PinName DTR=NC) |
This method initializes the object with the underlying radio interface to use. | |
virtual bool | connect () |
This method establishes a data connection on the cellular radio. | |
virtual void | disconnect () |
This method is used to stop a previously established cellular data connection. | |
virtual bool | isConnected () |
This method is used to check if the radio currently has a data connection established. | |
virtual bool | bind (unsigned int port) |
This method is used to set the local port for the UDP or TCP socket connection. | |
virtual bool | open (const std::string &address, unsigned int port, Mode mode) |
This method is used to open a socket connection with the given parameters. | |
virtual bool | isOpen () |
This method is used to determine if a socket connection is currently open. | |
virtual bool | close () |
This method is used to close a socket connection that is currently open. | |
virtual int | read (char *data, int max, int timeout=-1) |
This method is used to read data off of a socket, assuming a valid socket connection is already open. | |
virtual int | write (const char *data, int length, int timeout=-1) |
This method is used to write data to a socket, assuming a valid socket connection is already open. | |
virtual unsigned int | readable () |
This method is used to get the number of bytes available to read off the socket. | |
virtual unsigned int | writeable () |
This method is used to get the space available to write bytes to the socket. | |
virtual void | reset () |
A method to reset the Multi-Tech Socket Modem. | |
std::string | sendCommand (const std::string &command, unsigned int timeoutMillis, char esc=CR) |
A method for sending a generic AT command to the radio. | |
Code | sendBasicCommand (const std::string &command, unsigned int timeoutMillis, char esc=CR) |
A method for sending a basic AT command to the radio. | |
std::string | getDeviceIP () |
This method is used to get the IP address of the device, which is determined via DHCP by the cellular carrier. | |
Code | test () |
A method for testing command access to the radio. | |
Code | echo (bool state) |
A method for configuring command ehco capability on the radio. | |
int | getSignalStrength () |
A method for getting the signal strength of the radio. | |
Registration | getRegistration () |
This method is used to check the registration state of the radio with the cell tower. | |
Code | setApn (const std::string &apn) |
This method is used to set the radios APN if using a SIM card. | |
Code | setDns (const std::string &primary, const std::string &secondary="0.0.0.0") |
This method is used to set the DNS which enables the use of URLs instead of IP addresses when making a socket connection. | |
bool | ping (const std::string &address="8.8.8.8") |
This method is used test network connectivity by pinging a server. | |
Code | setSocketCloseable (bool enabled=true) |
This method can be used to trade socket functionality for performance. | |
Code | sendSMS (const std::string &phoneNumber, const std::string &message) |
This method is used to send an SMS message. | |
Code | sendSMS (const Sms &sms) |
This method is used to send an SMS message. | |
std::vector< Cellular::Sms > | getReceivedSms () |
This method retrieves all of the SMS messages currently available for this phone number. | |
Code | deleteAllReceivedSms () |
This method can be used to remove/delete all received SMS messages even if they have never been retrieved or read. | |
Code | deleteOnlyReceivedReadSms () |
This method can be used to remove/delete all received SMS messages that have been retrieved by the user through the getReceivedSms method. | |
Static Public Member Functions | |
static Cellular * | getInstance () |
This static function is used to create or get a reference to a Cellular object. | |
static std::string | getRegistrationNames (Registration registration) |
A static method for getting a string representation for the Registration enumeration. | |
Private Types | |
enum | Mode |
An enumeration for selecting the Socket Mode of TCP or UDP. More... |
Detailed Description
This is a class for communicating with a Multi-Tech Systems SocketModem iCell.
The SocketModem iCell is a family of carrier certified embedded cellular radio modules with a common hardware footprint and AT command set for built in IP-stack functionality. This class supports three main types of cellular radio interactions including: configuration and status AT command processing, SMS processing, and TCP Socket data connections. It should be noted that the radio can not process commands or SMS messages while having an open data connection at the same time. The concurrent capability may be added in a future release. This class also inherits from IPStack providing a common set of commands for communication devices that have an onboard IP Stack. It is also integrated with the standard mbed Sockets package and can therefore be used seamlessly with clients and services built on top of this interface already within the mbed library.
All of the following examples use the Pin Names for the Freedom KL46Z board coupled with the SocketModem Shield Arduino compatible board. Please chage Pin Names accordingly to match your hardware configuration. It also assumes the use of RTS/CTS hardware handshaking using GPIOs. To disable this you will need to change settings on the radio module and and use the MTSSerial class instead of MTSSerialFlowControl. The default baud rate for the cellular radio is 115200 bps.
The following set of example code demonstrates how to send and receive configuration and status AT commands with the radio, create a data connection and test it:
#include "mbed.h" #include "Cellular.h" #include "MTSSerialFlowControl.h" using namespace mts; main() { //Setup serial interface to radio MTSSerialFlowControl* serial = new MTSSerialFlowControl(PTD3, PTD2, PTA12, PTC8); serial->baud(115200); //Setup Cellular class Cellular* cellular = Cellular::getInstance(); cellular->init(serial, PTA4, PTC9); //DCD and DTR pins for KL46Z //Run status and configuration commands printf("\n\r////Start Status and Configuration Commands////\n\r"); printf("Command Test: %s\n\r", getCodeNames(cellular->test()).c_str()); printf("Signal Strength: %d\n\r", cellular->getSignalStrength()); printf("Registration State: %s\n\r", Cellular::getRegistrationNames(cellular->getRegistration()).c_str()); printf("Send Basic Command (AT): %s\n\r", getCodeNames(cellular->sendBasicCommand("AT", 1000)).c_str()); printf("Send Command (AT+CSQ): %s\n\r", cellular->sendCommand("AT+CSQ", 1000).c_str()); //Start Test printf("\n\r////Start Network Connectivity Test////\n\r"); printf("Set APN: %s\n\r", getCodeNames(cellular->setApn("wap.cingular")).c_str()); //Use APN from service provider!!! //Setup a data connection printf("Attempting to Connect, this may take some time...\n\r"); while (!cellular->connect()) { printf("Failed to connect... Trying again.\n\r"); wait(1); } printf("Connected to the Network!\n\r"); //Try pinging default server "8.8.8.8" printf("Ping Valid: %s\n\r", cellular->ping() ? "true" : "false"); printf("End Program\n\r"); }
The following set of example code demonstrates how process SMS messages:
#include "mbed.h" #include "Cellular.h" #include "MTSSerialFlowControl.h" using namespace mts; main() { //Setup serial interface to radio MTSSerialFlowControl* serial = new MTSSerialFlowControl(PTD3, PTD2, PTA12, PTC8); serial->baud(115200); //Setup Cellular class Cellular* cellular = Cellular::getInstance(); cellular->init(serial, PTA4, PTC9); //DCD and DTR pins for KL46Z //Start test printf("AT Test: %s\n\r", getCodeNames(cellular->test()).c_str()); //Waiting for network registration printf("Checking Network Registration, this may take some time...\n\r"); while (cellular->getRegistration() != Cellular::REGISTERED) { printf("Still waiting... Checking again.\n\r"); wait(1); } printf("Connected to the Network!\n\r"); //Send SMS Message Code code; std::string sMsg("Hello from Multi-Tech MBED!"); std::string sPhoneNum("16128675309"); //Put your phone number here or leave Jenny's... printf("Sending message [%s] to [%s]\r\n", sMsg.c_str(), sPhoneNum.c_str()); code = cellular->sendSMS(sPhoneNum, sMsg); if(code != SUCCESS) { printf("Error during SMS send [%s]\r\n", getCodeNames(code).c_str()); } else { printf("Success!\r\n"); } //Try and receive SMS messages //To determine your radio's phone number send yourself an SMS and check the received # printf("Checking Received Messages\r\n"); std::vector<Cellular::Sms> vSms = cellular->getReceivedSms(); printf("\r\n"); for(unsigned int i = 0; i < vSms.size(); i++) { printf("[%d][%s][%s][%s]\r\n", i, vSms[i].timestamp.c_str(), vSms[i].phoneNumber.c_str(), vSms[i].message.c_str()); } printf("End Program\n\r"); }
The following set of example code demonstrates how to setup and use a TCP socket connection using the native commands from this class:
#include "mbed.h" #include "Cellular.h" #include "MTSSerialFlowControl.h" using namespace mts; main() { //Define connection parameters Code code; const int TEST_PORT = 5798; const std::string TEST_SERVER("204.26.122.96"); //Setup serial interface to radio MTSSerialFlowControl* serial = new MTSSerialFlowControl(PTD3, PTD2, PTA12, PTC8); serial->baud(115200); //Setup Cellular class Cellular* cellular = Cellular::getInstance(); cellular->init(serial, PTA4, PTC9); //DCD and DTR pins for KL46Z //Start test printf("AT Test: %s\n\r", getCodeNames(cellular->test()).c_str()); printf("Setting APN\r\n"); code = cellular->setApn("wap.cingular"); // Use from your service provider! if(code == SUCCESS) { printf("Success!\r\n"); } else { printf("Error during APN setup [%s]\r\n", getCodeNames(code).c_str()); } //Setup a data connection printf("Attempting to Connect, this may take some time...\n\r"); while (!cellular->connect()) { printf("Failed to connect... Trying again.\n\r"); wait(1); } printf("Connected to the Network!\n\r"); printf("Opening a TCP Socket...\r\n"); if(cellular->open(TEST_SERVER, TEST_PORT, IPStack::TCP)) { printf("Success!\r\n"); } else { printf("Error during TCP socket open [%s:%d]\r\n", TEST_SERVER.c_str(), TEST_PORT); } char data[] = "My Test Echo Message"; int size = sizeof(data); printf("WRITE: [%d] [%s]\r\n", size, data); int bytesWritten = cellular->write(data, size, 10000); if(bytesWritten == size) { printf("Successfully wrote message!\r\n"); } else { printf("Failed to write message!\r\n"); } printf("Waiting 5 seconds\r\n"); wait(5); printf("Reading from socket for 10 seconds\r\n"); char response[size]; int bytesRead = cellular->read(response, size, 10000); response[size - 1] = '\0'; printf("READ: [%d] [%s]\r\n", bytesRead, response); //Check to see if echo was successful if (strcmp(data, response) == 0) { printf("Echo Successful!\n\r"); } else { printf("Echo failed!\n\r"); } //Cleaning up the connection printf("Closing socket: %s\r\n", cellular->close() ? "Success" : "Failure"); printf("Disconnecting...\r\n"); cellular->disconnect(); printf("End Program\n\r"); }
Definition at line 233 of file Cellular.h.
Member Enumeration Documentation
enum Registration |
An enumeration of radio registration states with a cell tower.
Definition at line 238 of file Cellular.h.
Constructor & Destructor Documentation
~Cellular | ( | ) |
Destructs a Cellular object and frees all related resources.
Definition at line 49 of file Cellular.cpp.
Member Function Documentation
bool bind | ( | unsigned int | port ) | [virtual] |
This method is used to set the local port for the UDP or TCP socket connection.
The connection can be made using the open method.
- Parameters:
-
port the local port of the socket as an int.
Implements IPStack.
Definition at line 211 of file Cellular.cpp.
bool close | ( | ) | [virtual] |
This method is used to close a socket connection that is currently open.
- Returns:
- true if successfully closed, otherwise returns false on an error.
Implements IPStack.
Definition at line 340 of file Cellular.cpp.
bool connect | ( | ) | [virtual] |
This method establishes a data connection on the cellular radio.
Note that before calling you must have an activated radio and if using a SIM card set the APN using the setApn method. The APN can be obtained from your cellular service provider.
- Returns:
- true if the connection was successfully established, otherwise false on an error.
Implements IPStack.
Definition at line 96 of file Cellular.cpp.
Code deleteAllReceivedSms | ( | ) |
This method can be used to remove/delete all received SMS messages even if they have never been retrieved or read.
- Returns:
- the standard AT Code enumeration.
Definition at line 797 of file Cellular.cpp.
Code deleteOnlyReceivedReadSms | ( | ) |
This method can be used to remove/delete all received SMS messages that have been retrieved by the user through the getReceivedSms method.
Messages that have not been retrieved yet will be unaffected.
- Returns:
- the standard AT Code enumeration.
Definition at line 792 of file Cellular.cpp.
void disconnect | ( | ) | [virtual] |
This method is used to stop a previously established cellular data connection.
Implements IPStack.
Definition at line 154 of file Cellular.cpp.
Code echo | ( | bool | state ) |
A method for configuring command ehco capability on the radio.
This command sets whether sent characters are echoed back from the radio, in which case you will receive back every command you send.
- Parameters:
-
state if true echo will be turned off, otherwise it will be turned on.
- Returns:
- the standard AT Code enumeration.
Definition at line 593 of file Cellular.cpp.
std::string getDeviceIP | ( | ) |
This method is used to get the IP address of the device, which is determined via DHCP by the cellular carrier.
- Returns:
- the devices IP address.
Definition at line 574 of file Cellular.cpp.
Cellular * getInstance | ( | ) | [static] |
This static function is used to create or get a reference to a Cellular object.
Cellular uses the singleton pattern, which means that you can only have one existing at a time. The first time you call getInstance this method creates a new uninitialized Cellular object and returns it. All future calls to this method will return a reference to the instance created during the first call. Note that you must call init on the returned instance before mnaking any other calls. If using this class's bindings to any of the Socket package classes like TCPSocketConnection, you must call this method and the init method on the returned object first, before even creating the other objects.
- Returns:
- a reference to the single Cellular obect that has been created.
Definition at line 26 of file Cellular.cpp.
std::vector< Cellular::Sms > getReceivedSms | ( | ) |
This method retrieves all of the SMS messages currently available for this phone number.
- Returns:
- a vector of existing SMS messages each as an Sms struct.
Definition at line 739 of file Cellular.cpp.
Cellular::Registration getRegistration | ( | ) |
This method is used to check the registration state of the radio with the cell tower.
If not appropriatley registered with the tower you cannot make a cellular connection.
- Returns:
- the registration state as an enumeration type.
Definition at line 620 of file Cellular.cpp.
std::string getRegistrationNames | ( | Registration | registration ) | [static] |
A static method for getting a string representation for the Registration enumeration.
- Parameters:
-
code a Registration enumeration.
- Returns:
- the enumeration name as a string.
Definition at line 890 of file Cellular.cpp.
int getSignalStrength | ( | ) |
A method for getting the signal strength of the radio.
This method allows you to get a value that maps to signal strength in dBm. Here 0-1 is Poor, 2-9 is Marginal, 10-14 is Ok, 15-19 is Good, and 20+ is Excellent. If you get a result of 99 the signal strength is not known or not detectable.
- Returns:
- an integer representing the signal strength.
Definition at line 606 of file Cellular.cpp.
bool init | ( | MTSBufferedIO * | io, |
PinName | DCD = NC , |
||
PinName | DTR = NC |
||
) |
This method initializes the object with the underlying radio interface to use.
Note that this function MUST be called before any other calls will function correctly on a Cellular object. Also note that MTSBufferedIO is abstract, so you must use one of its inherited classes like MTSSerial or MTSSerialFlowControl.
- Parameters:
-
io the buffered io interface that is attached to the cellular radio. DCD this is the dcd signal from the radio. If attached the the pin must be passed in here for this class to operate correctly. The default is not connected. DTR this is the dtr signal from the radio. If attached the the pin must be passed in here for this class to operate correctly. The default is not connected.
- Returns:
- true if the init was successful, otherwise false.
Definition at line 59 of file Cellular.cpp.
bool isConnected | ( | ) | [virtual] |
This method is used to check if the radio currently has a data connection established.
- Returns:
- true if a data connection exists, otherwise false.
Implements IPStack.
Definition at line 173 of file Cellular.cpp.
bool isOpen | ( | ) | [virtual] |
This method is used to determine if a socket connection is currently open.
- Returns:
- true if the socket is currently open, otherwise false.
Implements IPStack.
Definition at line 331 of file Cellular.cpp.
bool open | ( | const std::string & | address, |
unsigned int | port, | ||
Mode | mode | ||
) | [virtual] |
This method is used to open a socket connection with the given parameters.
This socket connection is established using the devices built in IP stack. Currently TCP is the only supported mode.
- Parameters:
-
address is the address you want to connect to in the form of xxx.xxx.xxx.xxx or a URL. If using a URL make sure the device supports DNS and is properly configured for that mode. port the remote port you want to connect to. mode an enum that specifies whether this socket connection is type TCP or UDP. Currently only TCP is supported.
- Returns:
- true if the connection was successfully opened, otherwise false.
Implements IPStack.
Definition at line 225 of file Cellular.cpp.
bool ping | ( | const std::string & | address = "8.8.8.8" ) |
This method is used test network connectivity by pinging a server.
- Parameters:
-
address the address of the server in format xxx.xxx.xxx.xxx.
- Returns:
- true if the ping was successful, otherwise false.
Definition at line 664 of file Cellular.cpp.
int read | ( | char * | data, |
int | max, | ||
int | timeout = -1 |
||
) | [virtual] |
This method is used to read data off of a socket, assuming a valid socket connection is already open.
- Parameters:
-
data a pointer to the data buffer that will be filled with the read data. max the maximum number of bytes to attempt to read, typically the same as the size of the passed in data buffer. timeout the amount of time in milliseconds to wait in trying to read the max number of bytes. If set to -1 the call blocks until it receives the max number of bytes or encounters and error.
- Returns:
- the number of bytes read and stored in the passed in data buffer. Returns -1 if there was an error in reading.
Implements IPStack.
Definition at line 382 of file Cellular.cpp.
unsigned int readable | ( | ) | [virtual] |
This method is used to get the number of bytes available to read off the socket.
- Returns:
- the number of bytes available, 0 if there are no bytes to read.
Implements IPStack.
Definition at line 536 of file Cellular.cpp.
void reset | ( | ) | [virtual] |
A method to reset the Multi-Tech Socket Modem.
This command brings down the PPP link if it is up. After this function is called, at least 30 seconds should be allowed for the cellular radio to come back up before any other Cellular functions are called.
Implements IPStack.
Definition at line 563 of file Cellular.cpp.
Code sendBasicCommand | ( | const std::string & | command, |
unsigned int | timeoutMillis, | ||
char | esc = CR |
||
) |
A method for sending a basic AT command to the radio.
A basic AT command is one that simply has a response of either OK or ERROR without any other information. Note that you cannot send commands and have a data connection at the same time.
- Parameters:
-
command the command to send to the radio without the escape character. timeoutMillis the time in millis to wait for a response before returning. esc escape character to add at the end of the command, defaults to carriage return (CR).
- Returns:
- the standard Code enumeration.
Definition at line 802 of file Cellular.cpp.
string sendCommand | ( | const std::string & | command, |
unsigned int | timeoutMillis, | ||
char | esc = CR |
||
) |
A method for sending a generic AT command to the radio.
Note that you cannot send commands and have a data connection at the same time.
- Parameters:
-
command the command to send to the radio without the escape character. timeoutMillis the time in millis to wait for a response before returning. esc escape character to add at the end of the command, defaults to carriage return (CR). Does not append any character if esc == 0.
- Returns:
- all data received from the radio after the command as a string.
Definition at line 821 of file Cellular.cpp.
Code sendSMS | ( | const Sms & | sms ) |
This method is used to send an SMS message.
Note that you cannot send an SMS message and have a data connection open at the same time.
- Parameters:
-
sms an Sms struct that contains all SMS transaction information.
- Returns:
- the standard AT Code enumeration.
Definition at line 712 of file Cellular.cpp.
Code sendSMS | ( | const std::string & | phoneNumber, |
const std::string & | message | ||
) |
This method is used to send an SMS message.
Note that you cannot send an SMS message and have a data connection open at the same time.
- Parameters:
-
phoneNumber the phone number to send the message to as a string. message the text message to be sent.
- Returns:
- the standard AT Code enumeration.
Definition at line 717 of file Cellular.cpp.
Code setApn | ( | const std::string & | apn ) |
This method is used to set the radios APN if using a SIM card.
Note that the APN must be set correctly before you can make a data connection. The APN for your SIM can be obtained by contacting your cellular service provider.
- Parameters:
-
the APN as a string.
- Returns:
- the standard AT Code enumeration.
Definition at line 648 of file Cellular.cpp.
Code setDns | ( | const std::string & | primary, |
const std::string & | secondary = "0.0.0.0" |
||
) |
This method is used to set the DNS which enables the use of URLs instead of IP addresses when making a socket connection.
- Parameters:
-
the DNS server address as a string in form xxx.xxx.xxx.xxx.
- Returns:
- the standard AT Code enumeration.
Definition at line 659 of file Cellular.cpp.
Code setSocketCloseable | ( | bool | enabled = true ) |
This method can be used to trade socket functionality for performance.
In order to enable a socket connection to be closed by the client side programtically, this class must process all read and write data on the socket to guard the special escape character used to close an open socket connection. It is recommened that you use the default of true unless the overhead of these operations is too significant.
- Parameters:
-
enabled set to true if you want the socket closeable, otherwise false. The default is true.
- Returns:
- the standard AT Code enumeration.
Definition at line 696 of file Cellular.cpp.
Code test | ( | ) |
A method for testing command access to the radio.
This method sends the command "AT" to the radio, which is a standard radio test to see if you have command access to the radio. The function returns when it receives the expected response from the radio.
- Returns:
- the standard AT Code enumeration.
Definition at line 579 of file Cellular.cpp.
int write | ( | const char * | data, |
int | length, | ||
int | timeout = -1 |
||
) | [virtual] |
This method is used to write data to a socket, assuming a valid socket connection is already open.
- Parameters:
-
data a pointer to the data buffer that will be written to the socket. length the size of the data buffer to be written. timeout the amount of time in milliseconds to wait in trying to write the entire number of bytes. If set to -1 the call blocks until it writes all of the bytes or encounters and error.
- Returns:
- the number of bytes written to the socket's write buffer. Returns -1 if there was an error in writing.
Implements IPStack.
Definition at line 448 of file Cellular.cpp.
unsigned int writeable | ( | ) | [virtual] |
This method is used to get the space available to write bytes to the socket.
- Returns:
- the number of bytes that can be written, 0 if unable to write.
Implements IPStack.
Definition at line 549 of file Cellular.cpp.
Generated on Tue Jul 12 2022 21:46:23 by
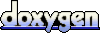