Free (GPLv2) TCP/IP stack developed by TASS Belgium
Dependents: lpc1768-picotcp-demo ZeroMQ_PicoTCP_Publisher_demo TCPSocket_HelloWorld_PicoTCP Pico_TCP_UDP_Test ... more
pico_protocol.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012-2015 Altran Intelligent Systems. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 *********************************************************************/ 00006 #ifndef INCLUDE_PICO_PROTOCOL 00007 #define INCLUDE_PICO_PROTOCOL 00008 #include "pico_config.h" 00009 #include "pico_queue.h" 00010 00011 #define PICO_LOOP_DIR_IN 1 00012 #define PICO_LOOP_DIR_OUT 2 00013 00014 enum pico_layer { 00015 PICO_LAYER_DATALINK = 2, /* Ethernet only. */ 00016 PICO_LAYER_NETWORK = 3, /* IPv4, IPv6, ARP. Arp is there because it communicates with L2 */ 00017 PICO_LAYER_TRANSPORT = 4, /* UDP, TCP, ICMP */ 00018 PICO_LAYER_SOCKET = 5 /* Socket management */ 00019 }; 00020 00021 enum pico_err_e { 00022 PICO_ERR_NOERR = 0, 00023 PICO_ERR_EPERM = 1, 00024 PICO_ERR_ENOENT = 2, 00025 /* ... */ 00026 PICO_ERR_EINTR = 4, 00027 PICO_ERR_EIO = 5, 00028 PICO_ERR_ENXIO = 6, 00029 /* ... */ 00030 PICO_ERR_EAGAIN = 11, 00031 PICO_ERR_ENOMEM = 12, 00032 PICO_ERR_EACCESS = 13, 00033 PICO_ERR_EFAULT = 14, 00034 /* ... */ 00035 PICO_ERR_EBUSY = 16, 00036 PICO_ERR_EEXIST = 17, 00037 /* ... */ 00038 PICO_ERR_EINVAL = 22, 00039 /* ... */ 00040 PICO_ERR_ENONET = 64, 00041 /* ... */ 00042 PICO_ERR_EPROTO = 71, 00043 /* ... */ 00044 PICO_ERR_ENOPROTOOPT = 92, 00045 PICO_ERR_EPROTONOSUPPORT = 93, 00046 /* ... */ 00047 PICO_ERR_EOPNOTSUPP = 95, 00048 PICO_ERR_EADDRINUSE = 98, 00049 PICO_ERR_EADDRNOTAVAIL = 99, 00050 PICO_ERR_ENETDOWN = 100, 00051 PICO_ERR_ENETUNREACH = 101, 00052 /* ... */ 00053 PICO_ERR_ECONNRESET = 104, 00054 /* ... */ 00055 PICO_ERR_EISCONN = 106, 00056 PICO_ERR_ENOTCONN = 107, 00057 PICO_ERR_ESHUTDOWN = 108, 00058 /* ... */ 00059 PICO_ERR_ETIMEDOUT = 110, 00060 PICO_ERR_ECONNREFUSED = 111, 00061 PICO_ERR_EHOSTDOWN = 112, 00062 PICO_ERR_EHOSTUNREACH = 113, 00063 }; 00064 00065 typedef enum pico_err_e pico_err_t; 00066 extern volatile pico_err_t pico_err; 00067 00068 #define IS_IPV6(f) (f && f->net_hdr && ((((uint8_t *)(f->net_hdr))[0] & 0xf0) == 0x60)) 00069 #define IS_IPV4(f) (f && f->net_hdr && ((((uint8_t *)(f->net_hdr))[0] & 0xf0) == 0x40)) 00070 00071 #define MAX_PROTOCOL_NAME 16 00072 00073 struct pico_protocol { 00074 char name[MAX_PROTOCOL_NAME]; 00075 uint32_t hash; 00076 enum pico_layer layer; 00077 uint16_t proto_number; 00078 struct pico_queue *q_in; 00079 struct pico_queue *q_out; 00080 struct pico_frame *(*alloc)(struct pico_protocol *self, uint16_t size); /* Frame allocation. */ 00081 int (*push)(struct pico_protocol *self, struct pico_frame *p); /* Push function, for active outgoing pkts from above */ 00082 int (*process_out)(struct pico_protocol *self, struct pico_frame *p); /* Send loop. */ 00083 int (*process_in)(struct pico_protocol *self, struct pico_frame *p); /* Recv loop. */ 00084 uint16_t (*get_mtu)(struct pico_protocol *self); 00085 }; 00086 00087 int pico_protocols_loop(int loop_score); 00088 void pico_protocol_init(struct pico_protocol *p); 00089 00090 int pico_protocol_datalink_loop(int loop_score, int direction); 00091 int pico_protocol_network_loop(int loop_score, int direction); 00092 int pico_protocol_transport_loop(int loop_score, int direction); 00093 int pico_protocol_socket_loop(int loop_score, int direction); 00094 00095 #endif
Generated on Tue Jul 12 2022 15:59:22 by
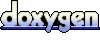