MODIFIED from mbed official WiflyInterface (interface for Roving Networks Wifly modules). Numerous performance and reliability improvements (see the detailed documentation). Also, tracking changes in mbed official version to retain functional parity.
Dependents: Smart-WiFly-WebServer PUB_WiflyInterface_Demo
Fork of WiflyInterface by
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "TCPSocketConnection.h" 00020 #include <algorithm> 00021 00022 TCPSocketConnection::TCPSocketConnection() {} 00023 00024 int TCPSocketConnection::connect(const char* host, const int port) 00025 { 00026 if (!wifi->connect(host, port)) 00027 return -1; 00028 wifi->flush(); 00029 return 0; 00030 } 00031 00032 bool TCPSocketConnection::is_connected(void) 00033 { 00034 return wifi->is_connected(); 00035 } 00036 00037 int TCPSocketConnection::send(const char* data, int length) 00038 { 00039 Timer tmr; 00040 00041 if (!_blocking) { 00042 tmr.start(); 00043 while (tmr.read_ms() < _timeout) { 00044 if (wifi->writeable()) 00045 break; 00046 } 00047 if (tmr.read_ms() >= _timeout) { 00048 return -1; 00049 } 00050 } 00051 return wifi->send(data, length); 00052 } 00053 00054 // -1 if unsuccessful, else number of bytes written 00055 int TCPSocketConnection::send_all(const char* data, int length) 00056 { 00057 Timer tmr; 00058 int idx = 0; 00059 tmr.start(); 00060 00061 while ((tmr.read_ms() < _timeout) || _blocking) { 00062 00063 idx += wifi->send(data, length); 00064 00065 if (idx == length) 00066 return idx; 00067 } 00068 return (idx == 0) ? -1 : idx; 00069 } 00070 00071 int TCPSocketConnection::receive(char* data, int length) 00072 { 00073 Timer tmr; 00074 int time = -1; 00075 00076 if (!_blocking) { 00077 tmr.start(); 00078 while (time < _timeout + 20) { 00079 if (wifi->readable()) { 00080 break; // go get the data. 00081 } 00082 time = tmr.read_ms(); 00083 } 00084 if (time >= _timeout + 20) { 00085 return -1; 00086 } 00087 } else { 00088 while(!wifi->readable()) 00089 ; 00090 } 00091 int nb_available = wifi->readable(); 00092 for (int i = 0; i < min(nb_available, length); i++) { 00093 data[i] = wifi->getc(); 00094 } 00095 return min(nb_available, length); 00096 } 00097 00098 int TCPSocketConnection::receive_all(char* data, int length) 00099 { 00100 Timer tmr; 00101 int idx = 0; 00102 int time = -1; 00103 00104 tmr.start(); 00105 while ((time < _timeout) || _blocking) { 00106 int nb_available = wifi->readable(); 00107 for (int i = 0; i < min(nb_available, length); i++) { 00108 data[idx++] = wifi->getc(); 00109 } 00110 if (idx == length) 00111 break; 00112 time = tmr.read_ms(); 00113 } 00114 return (idx == 0) ? -1 : idx; 00115 }
Generated on Tue Jul 12 2022 16:14:58 by
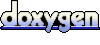