RTno is communicating library and framework which allows you to make your embedded device capable of communicating with RT-middleware world. RT-middleware is a platform software to realize Robotic system. In RTM, robots are developed by constructing robotics technologies\' elements (components) named RT-component. Therefore, the RTno helps you to create your own RT-component with your mbed and arduino. To know how to use your RTno device, visit here: http://ysuga.net/robot_e/rtm_e/rtc_e/1065?lang=en To know about RT-middleware and RT-component, visit http://www.openrtm.org
Dependents: RTnoV3_LED RTnoV3_Template RTnoV3_ADC RTnoV3_Timer ... more
ExecutionContext.cpp
00001 #define RTNO_SUBMODULE_DEFINE 00002 00003 #include <stdint.h> 00004 #include "RTno.h" 00005 #include "Packet.h" 00006 00007 #include "ExecutionContext.h" 00008 00009 static int8_t m_Type; 00010 static LifeCycleState m_Condition; 00011 00012 void EC_init(int8_t Type) { 00013 m_Type = Type; 00014 m_Condition = RTC_STATE_INACTIVE; 00015 } 00016 00017 int8_t EC_get_type() { 00018 return m_Type; 00019 } 00020 00021 LifeCycleState EC_get_component_state() { 00022 return m_Condition; 00023 } 00024 00025 ReturnValue_t EC_activate_component() { 00026 if(m_Condition != RTC_STATE_INACTIVE) { 00027 return RTC_PRECONDITION_NOT_MET; 00028 } 00029 00030 if(RTno::onActivated() == RTC_OK) { 00031 m_Condition = RTC_STATE_ACTIVE; 00032 return RTC_OK; 00033 } 00034 00035 m_Condition = RTC_STATE_ERROR; 00036 return RTC_ERROR; 00037 } 00038 00039 00040 ReturnValue_t EC_deactivate_component() { 00041 if(m_Condition != RTC_STATE_ACTIVE) { 00042 return RTC_PRECONDITION_NOT_MET; 00043 } 00044 00045 if(RTno::onDeactivated() == RTC_OK) { 00046 m_Condition = RTC_STATE_INACTIVE; 00047 return RTC_OK; 00048 } else { 00049 m_Condition = RTC_STATE_ERROR; 00050 return RTC_ERROR; 00051 } 00052 } 00053 00054 ReturnValue_t EC_execute() { 00055 if(m_Condition != RTC_STATE_ACTIVE) { 00056 return RTC_PRECONDITION_NOT_MET; 00057 } 00058 00059 if(RTno::onExecute() == RTC_OK) { 00060 return RTC_OK; 00061 } else { 00062 m_Condition = RTC_STATE_ERROR; 00063 return RTC_ERROR; 00064 } 00065 } 00066 00067 ReturnValue_t EC_error() { 00068 if(m_Condition != RTC_STATE_ERROR) { 00069 return RTC_PRECONDITION_NOT_MET; 00070 } 00071 00072 RTno::onError(); 00073 return RTC_OK; 00074 } 00075 00076 00077 #ifdef __cplusplus 00078 extern "C" { 00079 #endif 00080 00081 void (*EC_start)(); 00082 void (*EC_resume)(); 00083 void (*EC_suspend)(); 00084 00085 #ifdef __cplusplus 00086 } 00087 #endif
Generated on Tue Jul 12 2022 18:33:24 by
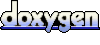