RTno is communicating library and framework which allows you to make your embedded device capable of communicating with RT-middleware world. RT-middleware is a platform software to realize Robotic system. In RTM, robots are developed by constructing robotics technologies\' elements (components) named RT-component. Therefore, the RTno helps you to create your own RT-component with your mbed and arduino. To know how to use your RTno device, visit here: http://ysuga.net/robot_e/rtm_e/rtc_e/1065?lang=en To know about RT-middleware and RT-component, visit http://www.openrtm.org
Dependents: RTnoV3_LED RTnoV3_Template RTnoV3_ADC RTnoV3_Timer ... more
BasicDataType.h
00001 /******************************************* 00002 * BasicDataType.h 00003 * @author Yuki Suga 00004 * @copyright Yuki Suga (ysuga.net) Nov, 10th, 2010. 00005 * @license LGPLv3 00006 *****************************************/ 00007 00008 #ifndef BASIC_DATA_TYPE_HEADER_INCLUDED 00009 #define BASIC_DATA_TYPE_HEADER_INCLUDED 00010 00011 #include <stdint.h> 00012 #include "Sequence.h" 00013 00014 #pragma pack(1) 00015 struct TimedBoolean { 00016 static const char typeCode = 'b'; 00017 char data; 00018 }; 00019 00020 struct TimedChar { 00021 static const char typeCode = 'c'; 00022 char data; 00023 }; 00024 00025 struct TimedOctet { 00026 static const char typeCode = 'o'; 00027 int8_t data; 00028 }; 00029 00030 struct TimedShort { 00031 static const char typeCode = 's'; 00032 int16_t data; 00033 00034 }; 00035 00036 struct TimedLong { 00037 static const char typeCode = 'l'; 00038 int32_t data; 00039 }; 00040 00041 struct TimedDouble { 00042 static const char typeCode = 'd'; 00043 double data; 00044 }; 00045 00046 struct TimedFloat { 00047 static const char typeCode = 'f'; 00048 float data; 00049 }; 00050 00051 00052 struct TimedOctetSeq { 00053 static const char typeCode = 'O'; 00054 Sequence<int8_t> data; 00055 }; 00056 00057 struct TimedCharSeq { 00058 static const char typeCode = 'C'; 00059 Sequence<char> data; 00060 }; 00061 00062 struct TimedBooleanSeq { 00063 static const char typeCode = 'B'; 00064 Sequence<char> data; 00065 }; 00066 00067 struct TimedShortSeq { 00068 static const char typeCode = 'S'; 00069 Sequence<int16_t> data; 00070 }; 00071 00072 struct TimedLongSeq { 00073 static const char typeCode = 'L'; 00074 Sequence<int32_t> data; 00075 }; 00076 00077 struct TimedFloatSeq { 00078 static const char typeCode = 'F'; 00079 Sequence<float> data; 00080 }; 00081 00082 struct TimedDoubleSeq { 00083 static const char typeCode = 'D'; 00084 Sequence<double> data; 00085 }; 00086 00087 00088 #endif
Generated on Tue Jul 12 2022 18:33:24 by
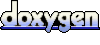