Library for Matrix Orbital VFD2041 display. Also useable for LCD2041 modules.
Embed:
(wiki syntax)
Show/hide line numbers
VFD.cpp
00001 #include "mbed.h" 00002 #include <string> 00003 #include "VFD.h" 00004 00005 VFD::VFD() : _i2c(SDA, SCL) { 00006 _i2c.frequency(100000); 00007 } 00008 00009 VFD::~VFD() { 00010 //Nothing to see here 00011 } 00012 00013 void VFD::start() { 00014 _i2c.start(); 00015 } 00016 00017 void VFD::stop() { 00018 _i2c.stop(); 00019 } 00020 00021 int VFD::send_byte(int byte) { 00022 return _i2c.write(byte); 00023 } 00024 00025 int VFD::read_byte(int ack) { 00026 return _i2c.read(ack); 00027 } 00028 00029 int VFD::init() { 00030 const char cmd[] = {254,160,0}; 00031 int length = 3; 00032 return write(cmd, length); 00033 } 00034 00035 int VFD::print(string msg) { 00036 return write(msg.data(), msg.size()); 00037 } 00038 00039 int VFD::autoScrollOn() { 00040 const char cmd[] = {254, 81}; 00041 int length = 2; 00042 return write(cmd, length); 00043 } 00044 00045 int VFD::autoScrollOff() { 00046 const char cmd[] = {254, 82}; 00047 int length = 2; 00048 return write(cmd, length); 00049 } 00050 00051 int VFD::lineWrapOn() { 00052 const char cmd[] = {254, 67}; 00053 int length = 2; 00054 return write(cmd, length); 00055 } 00056 00057 int VFD::lineWrapOff() { 00058 const char cmd[] = {254, 68}; 00059 int length = 2; 00060 return write(cmd, length); 00061 } 00062 00063 int VFD::setCursor(const int col, const int row) { 00064 const char cmd[] = {254, 71, col, row}; 00065 int length = 4; 00066 return write(cmd, length); 00067 } 00068 00069 int VFD::clearScreen() { 00070 const char cmd[] = {254, 88}; 00071 int length = 2; 00072 return write(cmd, length); 00073 } 00074 00075 int VFD::goHome() { 00076 const char cmd[] = {254, 72}; 00077 int length = 2; 00078 return write(cmd, length); 00079 } 00080 00081 int VFD::initLargeNumbers() { 00082 const char cmd[] = {254, 110}; 00083 int length = 2; 00084 return write(cmd, length); 00085 } 00086 00087 int VFD::setBrightness(const int val) { 00088 if (val < 0 || val > 3) 00089 return -1; 00090 const char cmd[] = {254, 89, val}; 00091 int length = 3; 00092 return write(cmd, length); 00093 } 00094 00095 int VFD::write(const char * data, int length) { 00096 int ret = _i2c.write(address, data, length); //Performs a complete write transaction 00097 wait_us(625); 00098 return ret; //Return I2C.write(...) return value 00099 } 00100 00101 int VFD::read(char * data, int length) { 00102 int ret = _i2c.read(address, data, length); //Performs a complete write transaction 00103 wait_us(625); 00104 return ret; //Return I2C.write(...) return value 00105 }
Generated on Wed Jul 27 2022 14:09:09 by
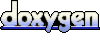